Fiber Network ZOJ 1967(Floyd+二进制状态压缩)
Description
Now, service providers, who want to send data from node A to node B are curious, which company is able to provide the necessary connections. Help the providers by answering their queries.
Input
After the list of connections, each test case is completed by a list of queries. Each query consists of two numbers A, B. The list (and with it the test case) is terminated by A=B=0. Otherwise, 1<=A,B<=n, and they denote the start and the endpoint of the query. You may assume that no connection and no query contains identical start and end nodes.
Output
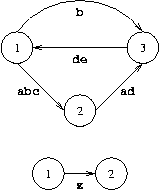
Sample Input
3
1 2 abc
2 3 ad
1 3 b
3 1 de
0 0
1 3
2 1
3 2
0 0
2
1 2 z
0 0
1 2
2 1
0 0
0
Sample Output
ab
d
- z
-
题目意思:n个路由器,编号1-n,26个公司,编号a-z,路由器之间有一些有向边,边权为一个字符串,字符串由小写字母组成,表示字符串对应的公司能使这条边连通。现在给若干个查询,查询能使任意2个路由器连通的公司。输出公司号,若不存在公司则输出‘-’。
解题思路:本题并不是求最短路,但是却要用到Floyd算法求解最短路的思想。题目就是要求能使任意2个路由器连通的公司的集合,所以可以使用Floyd传递闭包建立联系。另外,在本题中需要很巧妙地处理公司集合,公司是一个小写字母标识,最多只有26个公司,这样可以使用整数二进制位代表每个公司实现集合的状态压缩。
例如,站点1到站点2连接的公司有集合{ ‘a’ , 'b' , 'c' },可以用“00000000000000000000000111”表示,
站点2到站点3连接的公司有集合{ ‘a’ , 'd' } ,可以用“00000000000000000000001001”表示,
这两个整数进行二进制与运算后得到“00000000000000000000000001”表示通过中间站点2,站点1到站点3的连接的公司是集合{ ‘ a ’ }。
floyd的本质是枚举中间节点k,使节点i到j的距离最大或最小。针对本题,是要求一个集合,使从i到j连通的公司,那么枚举k的时候,就要求保证i->k和k->j同时连通的公司,状态压缩的话,直接将dis[i][k]和dis[k][j]相与便是结果,这个结果要加到dis[i][j]上去,所以再和dis[i][j]相或。所以总的方程就是:
dis[i][j] = dis[i][j] | (dis[i][k]&dis[k][j])。
#include<cstdio>
#include<cstring>
#include<algorithm>
using namespace std;
int dis[][];
int n,m;
void Floyd()
{
int i,j,k;
for(k=; k<=n; k++)
{
for(i=; i<=n; i++)
{
for(j=; j<=n; j++)
{
dis[i][j]|=(dis[i][k]&dis[k][j]);
}
}
}
}
int main()
{
int a,b,i;
int len;
char s[];
while(scanf("%d",&n)!=EOF)
{
if(n==)
{
break;
}
memset(dis,,sizeof(dis));
while(scanf("%d%d",&a,&b)!=EOF)
{
if(a==&&b==)
{
break;
}
scanf("%s",s);
len=strlen(s);
for(i=; i<len; i++)
{
dis[a][b]|=(<<(s[i]-'a'));///逻辑左移s[i]-'a'位
}
}
Floyd();
while(scanf("%d%d",&a,&b)!=EOF)
{
if(a==&&b==)
{
break;
}
if(dis[a][b])
{
for(i=; i<; i++)
{
if(dis[a][b]&(<<i))///如果dis[a][b]&(1<<i)!=0,说明dis[a][b]所代表的集合中包含公司'a'+i
{
putchar('a'+i);
}
}
}
else
{
putchar('-');
}
putchar('\n');
}
putchar('\n');
}
return ;
}
Fiber Network ZOJ 1967(Floyd+二进制状态压缩)的更多相关文章
- POJ 2777.Count Color-线段树(区间染色+区间查询颜色数量二进制状态压缩)-若干年之前的一道题目。。。
Count Color Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 53312 Accepted: 16050 Des ...
- hdu 1429 bfs+二进制状态压缩
开始时候只用了BFS,显然超时啊,必然在结构体里加一个数组什么的判重啊,开始用的一个BOOL数组,显然还是不行,复杂度高,每次都要遍历数组来判重:后百度之,学习了二进制状态压缩,其实就用一个二进制数来 ...
- # 最短Hamilton路径(二进制状态压缩)
最短Hamilton路径(二进制状态压缩) 题目描述:n个点的带权无向图,从0-n-1,求从起点0到终点n-1的最短Hamilton路径(Hamilton路径:从0-n-1不重不漏的每个点恰好进过一次 ...
- POJ 2570 Fiber Network(最短路 二进制处理)
题目翻译 一些公司决定搭建一个更快的网络.称为"光纤网". 他们已经在全世界建立了很多网站.这 些网站的作用类似于路由器.不幸的是,这些公司在关于网站之间的接线问题上存在争论,这样 ...
- 二进制状态压缩dp(旅行商TSP)POJ3311
http://poj.org/problem?id=3311 Hie with the Pie Time Limit: 2000MS Memory Limit: 65536K Total Subm ...
- POJ-3279.Fliptile(二进制状态压缩 + dfs) 子集生成
昨天晚上12点刷到的这个题,一开始一位是BFS,但是一直没有思路.后来推了一下发现只需要依次枚举第一行的所有翻转状态然后再对每个情况的其它田地翻转进行暴力dfs就可以,但是由于二进制压缩学的不是很透, ...
- HDU_1429——胜利大逃亡续,十位二进制状态压缩,状态判重
Problem Description Ignatius再次被魔王抓走了(搞不懂他咋这么讨魔王喜欢)……这次魔王汲取了上次的教训,把Ignatius关在一个n*m的地牢里,并在地牢的某些地方安装了带锁 ...
- BFS+二进制状态压缩 hdu-1429
好久没写搜索题了,就当练手吧. vis[][][1025]第三个维度用来维护不同key持有状态的访问情况. 对于只有钥匙没有对应门的位置,置为'.',避免不必要的状态分支. // // main.cp ...
- ZOJ - 3471 Most Powerful (状态压缩)
题目大意:有n种原子,两种原子相碰撞的话就会产生能量,当中的一种原子会消失. 问这n种原子能产生的能量最大是多少 解题思路:用0表示该原子还没消失.1表示该原子已经消失.那么就能够得到状态转移方程了 ...
随机推荐
- generate failed: Cannot resolve classpath entry: mysql-connector-java-5.1.38.jar
详细错误及处理方法如下: [ERROR] Failed to execute goal org.mybatis.generator:mybatis-generator-maven-plugin:1.3 ...
- HTML中放置CSS的三种方式和CSS选择器
(一)在HTML中使用CSS样式的方式一般有三种: 1 内联引用 2 内部引用 3 外部引用. 第一种:内联引用(也叫行内引用) 就是把CSS样式直接作用在HTML标签中. <p style ...
- Python绘制奥运五环
绘制奥运五环主要涉及到Python中的turtle绘图库运用: turtle.forward(distance) 向当前画笔方向移动distance像素长度 turtle.backward(dista ...
- 傻瓜式搭建php+nginx+mysql服务器环境
1.安装nginx 1.安装yum源 rpm -Uvh http://nginx.org/packages/centos/7/noarch/RPMS/nginx-release-centos-7-0. ...
- C# 数组集合分页 Skip Take
var input=new input(); var personList= new List<Person>(); //一个查询集合 var Total = personList.Cou ...
- 将select的默认小三角替换成别的图片,且实现点击图片出现下拉框选择option
最近做项目,要求修改select下拉框的默认三角样式,因为它在不同浏览器的样式不同且有点丑,找找网上也没什么详细修改方法,我就总结一下自己的吧. 目标是做成下图效果: 图一:将默认小三角换成红圈的三角 ...
- Redis的特性以及优势(附官网)
NoSQL:一类新出现的数据库(not only sql) 泛指非关系型的数据库 不支持SQL语法 存储结构跟传统关系型数据库中的那种关系表完全不同,nosql中存储的数据都是KV形式 NoSQL的世 ...
- JVM,JMM,虚拟机栈,本地方法栈
JVM 虚拟机栈 本地方法栈:本地方法(使用native关键词修饰的方法,是由JVM底层用C,C++实现的),运行这部份代码使用的栈就是本地方法栈
- unix文件共享
UNIX系统支持在不同的进程间共享打开文件.内核使用3种数据结构表示打开文件,他们之间的关系决定了在文件共享方面一个进程对另一个进程产生的影响. (1)每个进程在进程表中都有一个记录项,记录项中包含一 ...
- postgres 输出数据集的自定义函数
定义一个可输出数据集自定义函数有多种方法 1,先定义结构,再使用结构输出结果 CREATE TYPE compfoo AS (f1 int, f2 text); CREATE FUNCTION get ...