Android中如何使用JUnit进行单元测试 eclipse
Android中如何使用JUnit进行单元测试
<instrumentation android:name="android.test.InstrumentationTestRunner"
android:targetPackage="com.example.junittest" android:label="@string/app_name"
></instrumentation>
|
<?xml version="1.0" encoding="utf-8"?>
<manifest xmlns:android="http://schemas.android.com/apk/res/android"
package="com.example.junittest"
android:versionCode="1"
android:versionName="1.0" >
<uses-sdk
android:minSdkVersion="8"
android:targetSdkVersion="18" />
<instrumentation android:name="android.test.InstrumentationTestRunner"
android:targetPackage="com.example.junittest" android:label="@string/app_name"
></instrumentation>
<application
android:allowBackup="true"
android:icon="@drawable/ic_launcher"
android:label="@string/app_name"
android:theme="@style/AppTheme" >
<uses-library android:name="android.test.runner"/>
<activity
android:name="com.example.junittest.MainActivity"
android:label="@string/app_name" >
<intent-filter>
<action android:name="android.intent.action.MAIN" />
<category android:name="android.intent.category.LAUNCHER" />
</intent-filter>
</activity>
</application>
</manifest>
|
package com.example.junittest;
public class Calculator {
public int add(int x,int y){
return x+y;
}
public int sub(int x,int y){
return x-y;
}
public int divide(int x,int y){
return x/y;
}
public int multiply(int x,int y){
return x*y;
}
}
|

package com.example.test;
import junit.framework.Assert;
import com.example.junittest.Calculator;
import android.test.AndroidTestCase;
import android.util.Log;
public class CalculatorTester extends AndroidTestCase {
private static final String TAG = CalculatorTester.class.getSimpleName();
private Calculator calculator;
/**
* This method is invoked before any of the test methods in the class.
* Use it to set up the environment for the test (the test fixture. You can use setUp() to instantiate a new Intent with the action ACTION_MAIN. You can then use this intent to start the Activity under test.
*/
@Override
protected void setUp() throws Exception {
Log.e(TAG, "setUp");
calculator = new Calculator();
super.setUp();
}
/**
* 测试Calculator的add(int x, int y)方法
* 把异常抛给测试框架
* @throws Exception
*/
public void testAdd() throws Exception{
int result = calculator.add(3, 5);
Assert.assertEquals(8, result);
}
/**
* 测试Calculator的divide(int x, int y)方法
* 把异常抛给测试框架
* @throws Exception
*/
public void testDivide() throws Exception{
int result = calculator.divide(10, 0);
Assert.assertEquals(10, result);
}
/**
* This method is invoked after all the test methods in the class.
* Use it to do garbage collection and to reset the test fixture.
*/
@Override
protected void tearDown() throws Exception {
Log.e(TAG, "tearDown");
calculator = null;
super.tearDown();
}
}
|
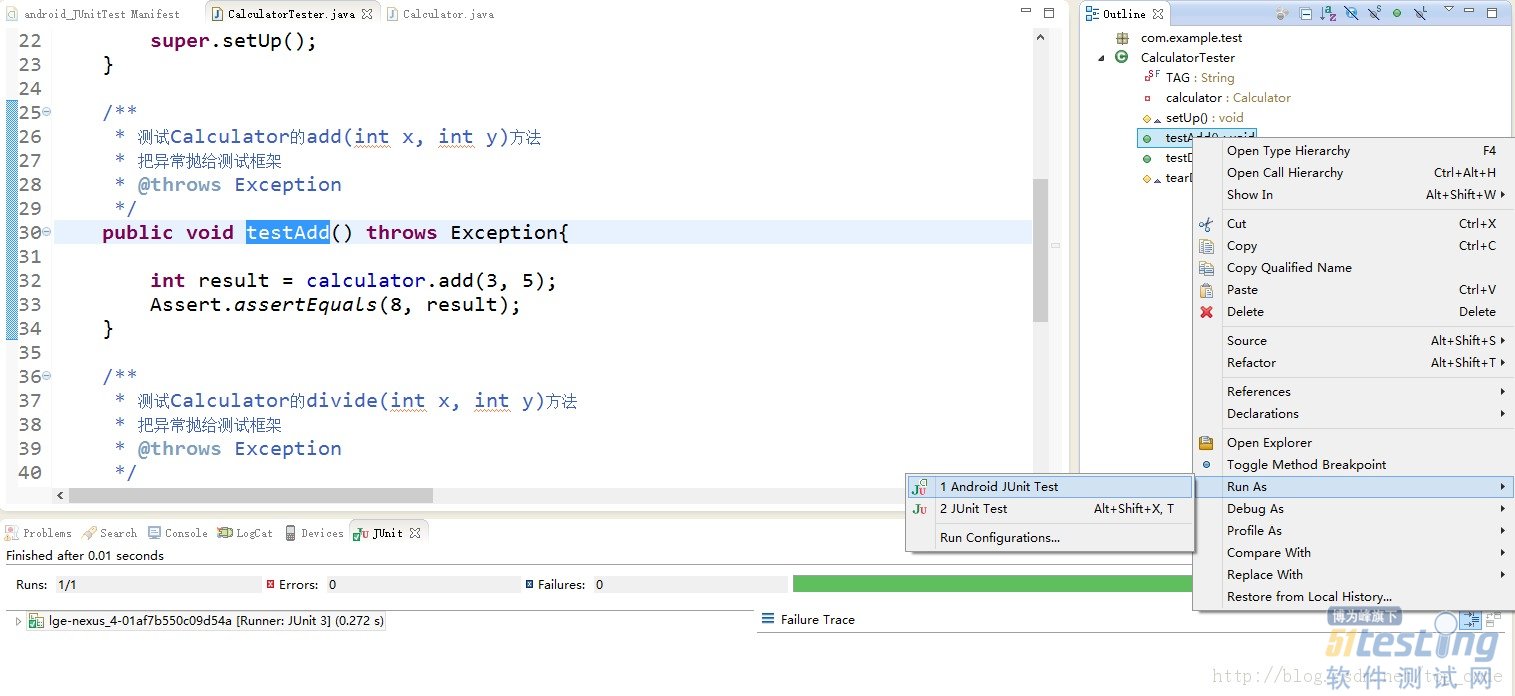



Android中如何使用JUnit进行单元测试 eclipse的更多相关文章
- Android之如何使用JUnit进行单元测试
转的:http://www.blogjava.net/qileilove/archive/2014/05/19/413824.html Android中如何使用JUnit进行单元测试 在我们日常开发a ...
- SpringBoot重点详解--使用Junit进行单元测试
目录 添加依赖与配置 ApplicationContext测试 Environment测试 MockBean测试 Controller测试 情况一 情况二 方法一 方法二 本文将对在Springboo ...
- 在Android中进行单元测试遇到的问题
问题1.Cannot connect to VM socket closed 在使用JUnit进行测试的时候,遇到这个问题.网上的解释是:使用Eclipse对Java代码进行调试,无论是远程JVM还 ...
- 软件工程第二次作业(Android Studio利用Junit进行单元测试)
一.开发工具的安装和运行 1.安装 由于我的电脑之前就安装好了Android Studio,就不再重装了.在这里就给出几条安装过程中需要注意的地方吧: 安装包最好在官网下载已经带有Android SD ...
- Android使用JUnit进行单元测试
前言:为什么要进行单元测试?单元测试能快速是开发者,找到代码中的问题所在,因为是单元测试,所以代码只执行响应的测试单元,执行快解决问题的效率高,同时提高代码的质量. Android中的单元测试可简单分 ...
- Android中使用自身携带的Junit新建一个测试工程
1.新建立一个Android工程 package com.shellway.junit; public class Service { public int divide(int a,int b){ ...
- 如何将Android默认的Camra程序导入到eclipse中
由于工作需要将camera源码导入到Eclipse中,找了很多的方法,现将自己的整理发出来.... 由于开发的要求,需要将Android默认的Camra程序导入到eclipse中,进行修改和再开发. ...
- Android中的单元测试
2015年5月19日 23:10 在Android中,已经内置了Junit所以不需要在导包.只要继承AndroidTestCase类就可以了. 首先需要修改AndroidManifes ...
- Android 开发之开发插件使用:Eclipse 插件 SQLiteManger eclipse中查看数据内容--翻译
最近研究了一段时间Android开发后发现,google自带的ADT工具,缺失一些开发常用的东西,希望可以构建一个类似使用JAVA EE开发体系一样开发的工具包集合,包括前台开发,调试,到后台数据库的 ...
随机推荐
- OpenCV_火焰检测——完整代码
转:http://blog.csdn.net/xiao_lxl/article/details/43307993 火焰检测小程序 前几天,偶然看到了An Early Fire-Detection Me ...
- FZU 2280 Magic(字符串Hash)题解
题意:给你n个字符串,每个字符串有一个值w,有q次询问,一共两种操作:一是“1 x y”表示把第x个串的w变为y:二是“2 x”,输出第x个串能放几次魔法.放魔法的条件是这样:用串x放魔法,如果在1~ ...
- Mininet实验 自定义拓扑结构
参考:MiniNet实验2 通过Mininet学习可视化操作,可以在界面上方便的构建拓扑结构. 最新的Mininet 2.2.0内置了一个mininet可视化工具miniedit.miniedit在/ ...
- python 单向链表
import sys import random class employee: def __init__(self): self.num= self.salary= self.name='' sel ...
- [原][osg][osgearth]我眼中的osgearth
看了一下,OE生成的可执行文件 除了osg库和第三方库 OE生产最多的dll就是 osgdb_osgearth_XXXX.dll了 这些都是为了通过osgDB机制加载earth的数据用的. 所以,我觉 ...
- 前端基础3:js篇(基础及算法)
1.js闭包相关: 题1: for (var i = 0; i < 5; i++) { setTimeout(function() { console.log(i); }, 1000 * i); ...
- Android之扫描二维码和根据输入信息生成名片二维码
开发中常常遇到二维码扫码操作,前段时间做项目要实现该功能,于是网上查找资料实现了,现在把他做出来给各位分享一下,主要包含了二维码扫描和生成二维码名片. 先来看看效果图: 生成的二维码,打开微信扫一 ...
- jxl将list导入到Excel中供下载
jxl操作excel /** * 分隔符 */ private final static String SEPARATOR = "|"; /** * 由List导出至指定的Shee ...
- 平衡二叉树,AVL树之代码篇
看完了第一篇博客,相信大家对于平衡二叉树的插入调整以及删除调整已经有了一定的了解,下面,我们开始介绍代码部分. 首先,再次提一下使用的结构定义 typedef char KeyType; //关键字 ...
- activity+fragment+listview+adapter+bean在同一个类中的套路
1.xml activity_main.xml <?xml version="1.0" encoding="utf-8"?><FrameLay ...