1.tTensorboard
Windows下坑太多......
在启动TensorBoard的过程,还是遇到了一些问题。接下来简单的总结一下我遇到的坑。
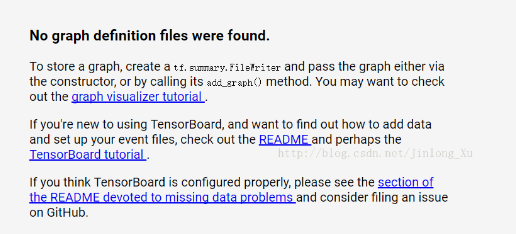
查看指定端口并kill
也可以使用lsof命令:
lsof -i:8888
若要关闭使用这个端口的程序,使用kill + 对应的pid
kill -9 PID号

启动jupyter notebook
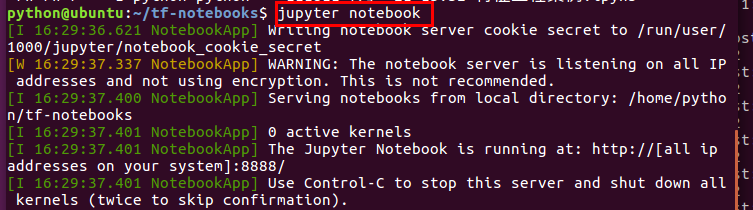
通过windows远程调用写一个Demo
启动tensorboard并通过web访问:
sudo tensorboard --logdir='.' --port=8811
- """
- Please note, this code is only for python 3+. If you are using python 2+, please modify the code accordingly.
- """
- #-*-coding:utf8-*-
- import tensorflow as tf
- import numpy as np
- def add_layer(inputs, in_size, out_size, n_layer, activation_function=None):
- # add one more layer and return the output of this layer
- layer_name = 'layer%s' % n_layer
- with tf.name_scope(layer_name):
- with tf.name_scope('weights'):
- Weights = tf.Variable(tf.random_normal([in_size, out_size]), name='W')
- tf.summary.histogram(layer_name + '/weights', Weights)
- with tf.name_scope('biases'):
- biases = tf.Variable(tf.zeros([1, out_size]) + 0.1, name='b')
- tf.summary.histogram(layer_name + '/biases', biases)
- with tf.name_scope('Wx_plus_b'):
- Wx_plus_b = tf.add(tf.matmul(inputs, Weights), biases)
- if activation_function is None:
- outputs = Wx_plus_b
- else:
- outputs = activation_function(Wx_plus_b, )
- tf.summary.histogram(layer_name + '/outputs', outputs)
- return outputs
- # Make up some real data
- x_data = np.linspace(-1, 1, 300)[:, np.newaxis]
- noise = np.random.normal(0, 0.05, x_data.shape)
- y_data = np.square(x_data) - 0.5 + noise
- # define placeholder for inputs to network
- with tf.name_scope('inputs'):
- xs = tf.placeholder(tf.float32, [None, 1], name='x_input')
- ys = tf.placeholder(tf.float32, [None, 1], name='y_input')
- # add hidden layer
- l1 = add_layer(xs, 1, 10, n_layer=1, activation_function=tf.nn.relu)
- # add output layer
- prediction = add_layer(l1, 10, 1, n_layer=2, activation_function=None)
- # the error between prediciton and real data
- with tf.name_scope('loss'):
- loss = tf.reduce_mean(tf.reduce_sum(tf.square(ys - prediction),
- reduction_indices=[1]))
- tf.summary.scalar('loss', loss)
- with tf.name_scope('train'):
- train_step = tf.train.GradientDescentOptimizer(0.1).minimize(loss)
- sess = tf.Session()
- merged = tf.summary.merge_all()
- writer = tf.summary.FileWriter("./tensorlogs/", sess.graph)
- init = tf.global_variables_initializer()
- sess.run(init)
下面代码在python3中正常,在python2中需要更改
- for i in range(1000):
- sess.run(train_step, feed_dict={xs: x_data, ys: y_data})
- if i % 50 == 0:
- result = sess.run(merged,feed_dict={xs: x_data, ys: y_data})
- writer.add_summary(result, i)
- # direct to the local dir and run this in terminal:
- # $ tensorboard --logdir logs
web页面显示
1.tTensorboard的更多相关文章
随机推荐
- ajax之cache血与泪~~
场景:项目以ie5渲染页面,点击导出列表数据(Excel形式),点击导出发送get请求,后台生成Excel文件,返回文件地址信息 异常:ie第一次返回的信息正常,之后返回的都是第一次的结果,googl ...
- Android ViewDragHelper全然解析 自己定义ViewGroup神器
转载请标明出处: http://blog.csdn.net/lmj623565791/article/details/46858663. 本文出自:[张鸿洋的博客] 一.概述 在自己定义ViewGro ...
- 3. Oracle数据库逻辑备份与恢复
一. Oracle逻辑备份介绍 Oracle逻辑备份的核心就是复制数据:Oracle提供的逻辑备份与恢复的命令有exp/imp,expdp/impdp.当然像表级复制(create table tab ...
- PHP代码审计笔记--变量覆盖漏洞
变量覆盖指的是用我们自定义的参数值替换程序原有的变量值,一般变量覆盖漏洞需要结合程序的其它功能来实现完整的攻击. 经常导致变量覆盖漏洞场景有:$$,extract()函数,parse_str()函数, ...
- iOS开发-- 设置UIButton的文字显示位置、字体的大小、字体的颜色
btn.frame = CGRectMake(x, y, width, height); [btn setTitle: @"search" forState: UIControlS ...
- SpringBoot(十一)-- 动态数据源
SpringBoot中使用动态数据源可以实现分布式中的分库技术,比如查询用户 就在用户库中查询,查询订单 就在订单库中查询. 一.配置文件application.properties # 默认数据源 ...
- 导入贴图操作:处理贴图MaxSize和Format
using UnityEngine; using System.Collections; using UnityEditor; public class ImportModflyTextures : ...
- redis 缓存类型为map
// 获取分类列表,以及同类品牌 public Map<String, List> getCatalogInfo(Product product) { String key = Cache ...
- HttpClient 通信工具类
package com.taotao.web.service; import java.util.ArrayList; import java.util.List; import java.util. ...
- CoreData 数据库更新,数据迁移
本文转载至 http://blog.163.com/djx421@126/blog/static/48855136201411381212985/ 一般程序app升级时,数据库有可能发生改变,如增 ...