Maximum repetition substring 后缀数组
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 7578 | Accepted: 2281 |
Description
The repetition number of a string is defined as the maximum number R such that the string can be partitioned into R same consecutive substrings. For example, the repetition number of "ababab" is 3 and "ababa" is 1.
Given a string containing lowercase letters, you are to find a substring of it with maximum repetition number.
Input
The input consists of multiple test cases. Each test case contains exactly one line, which gives a non-empty string consisting of lowercase letters. The length of the string will not be greater than 100,000.
The last test case is followed by a line containing a '#'.
Output
For each test case, print a line containing the test case number( beginning with 1) followed by the substring of maximum repetition number. If there are multiple substrings of maximum repetition number, print the lexicographically smallest one.
Sample Input
- ccabababc
- daabbccaa
- #
Sample Output
- Case 1: ababab
- Case 2: aa
Source


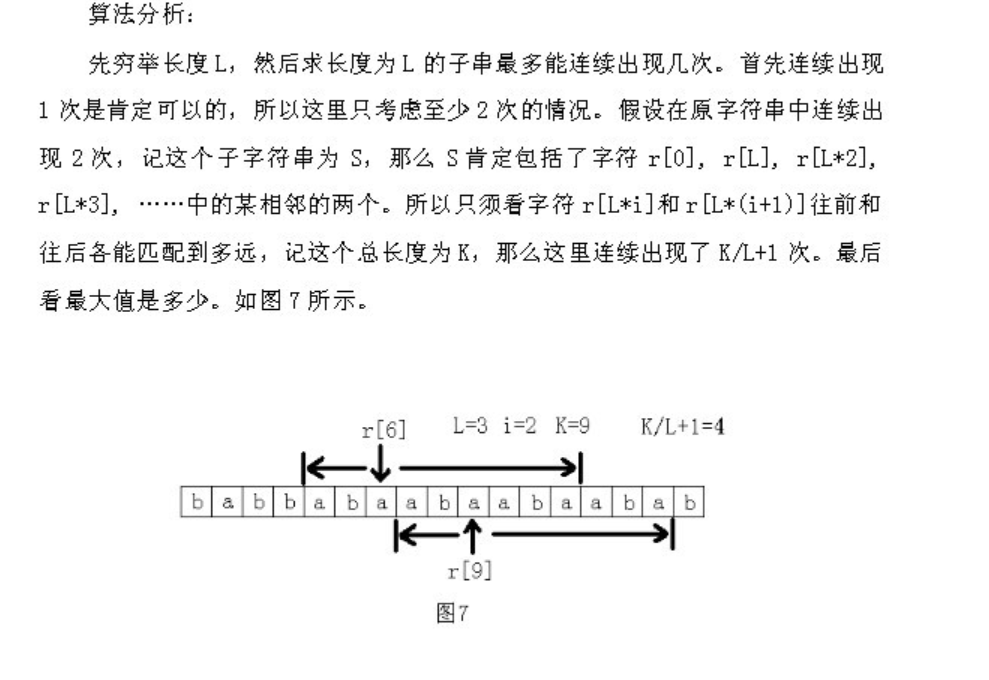
- #include <iostream>
- #include <cstdio>
- #include <cstring>
- #include <cmath>
- #include <algorithm>
- #include <string>
- #include <vector>
- #include <stack>
- #include <queue>
- #include <set>
- #include <map>
- #include <list>
- #include <iomanip>
- #include <cstdlib>
- using namespace std;
- const int INF=0x5fffffff;
- const double EXP=1e-;
- const int MS=;
- // KMP TRIE DFA SUFFIX
- int dp[MS][]; // RMQ
- int t1[MS],t2[MS],c[MS],v[MS];
- int rank[MS],sa[MS],height[MS];
- char str[MS],str1[MS];
- int s[MS];
- int cmp(int *r,int a,int b,int k)
- {
- return r[a]==r[b]&&r[a+k]==r[b+k];
- }
- void get_sa(int *r,int *sa,int n,int m)
- {
- int i,j,p,*x=t1,*y=t2;
- for(i=;i<m;i++)
- c[i]=;
- for(i=;i<n;i++)
- c[x[i]=r[i]]++;
- for(i=;i<m;i++)
- c[i]+=c[i-];
- for(i=n-;i>=;i--)
- sa[--c[x[i]]]=i;
- p=;j=;
- for(;p<n;j*=,m=p)
- {
- for(p=,i=n-j;i<n;i++)
- y[p++]=i;
- for(i=;i<n;i++)
- if(sa[i]>=j)
- y[p++]=sa[i]-j;
- for(i=;i<n;i++)
- v[i]=x[y[i]];
- for(i=;i<m;i++)
- c[i]=;
- for(i=;i<n;i++)
- c[v[i]]++;
- for(i=;i<m;i++)
- c[i]+=c[i-];
- for(i=n-;i>=;i--)
- sa[--c[v[i]]]=y[i];
- swap(x,y);
- x[sa[]]=;
- for(p=,i=;i<n;i++)
- x[sa[i]]=cmp(y,sa[i-],sa[i],j)?p-:p++;
- }
- }
- void get_height(int *r,int n)
- {
- int i,j,k=;
- for(i=;i<=n;i++)
- rank[sa[i]]=i;
- //height[i]>=height[i-1]-1;
- for(i=;i<n;i++)
- {
- if(k)
- k--;
- else
- k=;
- j=sa[rank[i]-];
- while(r[i+k]==r[j+k])
- k++;
- height[rank[i]]=k;
- }
- }
- void rmq_init(int n)
- {
- for(int i=;i<=n;i++) dp[i][]=height[i];
- for(int j=;(<<j)<=n;j++)
- for(int i=;i+(<<j)-<=n;i++)
- dp[i][j]=min(dp[i][j-],dp[i+(<<(j-))][j-]);
- }
- int rmq(int ll,int rr)
- {
- int k=;
- ll=rank[ll];
- rr=rank[rr];
- if(ll>rr)
- {
- int tmp=ll;
- ll=rr;
- rr=tmp;
- }
- ll++;
- while((<<(k+))<=rr-ll+) k++;
- return min(dp[ll][k],dp[rr-(<<k)+][k]);
- }
- int main()
- {
- int text=;
- while(scanf("%s",str)>)
- {
- if(str[]=='#')
- break;
- int len=strlen(str);
- for(int i=;i<len;i++)
- s[i]=str[i]-'a'+;
- s[len]=;
- get_sa(s,sa,len+,);
- get_height(s,len);
- rmq_init(len);
- int ans=,pos=,lenn;
- for(int i=;i<=len/;i++)
- {
- for(int j=;j<len-i;j+=i)
- {
- if(str[j]!=str[j+i])
- continue;
- int k=rmq(j,j+i);
- int tol=k/i+;
- //printf("%d\n",tol);
- int r=i-k%i;
- int p=j;
- int cnt=;
- for(int m=j-;m>j-i&&str[m]==str[m+i]&&m>=;m--)
- {
- cnt++;
- if(cnt==r)
- {
- tol++;
- p=m;
- }
- else if(rank[p]>rank[m])
- {
- p=m;
- }
- }
- if(ans<tol)
- {
- ans=tol;
- pos=p;
- lenn=tol*i;
- }
- else if(ans==tol&&rank[pos]>rank[p])
- {
- pos=p;
- lenn=tol*i;
- }
- }
- }
- printf("Case %d: ",++text);
- // printf("%d %d %d\n",ans,pos,lenn);
- if(ans<) //这里,如果字符总长度小于2,那么就在原串中找出一个最小的字符就好
- {
- char ch='z';
- for(int i=;i<len;i++)
- if(str[i]<ch)
- ch=str[i];
- printf("%c\n",ch);
- continue;
- }
- for(int i=pos;i<pos+lenn;i++)
- printf("%c",str[i]);
- printf("\n");
- }
- return ;
- }
Maximum repetition substring 后缀数组的更多相关文章
- POJ3693 Maximum repetition substring [后缀数组 ST表]
Maximum repetition substring Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 9458 Acc ...
- POJ3693 Maximum repetition substring 后缀数组
POJ - 3693 Maximum repetition substring 题意 输入一个串,求重复次数最多的连续重复字串,如果有次数相同的,则输出字典序最小的 Sample input ccab ...
- POJ3693 Maximum repetition substring —— 后缀数组 重复次数最多的连续重复子串
题目链接:https://vjudge.net/problem/POJ-3693 Maximum repetition substring Time Limit: 1000MS Memory Li ...
- poj3693 Maximum repetition substring (后缀数组+rmq)
Description The repetition number of a string is defined as the maximum number R such that the strin ...
- POJ 3693 Maximum repetition substring ——后缀数组
重复次数最多的字串,我们可以枚举循环节的长度. 然后正反两次LCP,然后发现如果长度%L有剩余的情况时,答案是在一个区间内的. 所以需要找到区间内最小的rk值. 两个后缀数组,四个ST表,$\Thet ...
- 【Poj-3693】Maximum repetition substring 后缀数组 连续重复子串
POJ - 3693 题意 SPOJ - REPEATS的进阶版,在这题的基础上输出字典序最小的重复字串. 思路 跟上题一样,先求出最长的重复次数,在求的过程中顺便纪录最多次数可能的长度. 因为sa数 ...
- poj 3693 Maximum repetition substring (后缀数组)
其实是论文题.. 题意:求一个字符串中,能由单位串repeat得到的子串中,单位串重复次数最多的子串.若有多个重复次数相同的,输出字典序最小的那个. 解题思路:其实跟论文差不多,我看了很久没看懂,后来 ...
- POJ 3693 Maximum repetition substring (后缀数组+RMQ)
题意:给定一个字符串,求其中一个由循环子串构成且循环次数最多的一个子串,有多个就输出最小字典序的. 析:枚举循环串的长度ll,然后如果它出现了两次,那么它一定会覆盖s[0],s[ll],s[ll*2] ...
- POJ-3693-Maximum repetition substring(后缀数组-重复次数最多的连续重复子串)
题意: 给出一个串,求重复次数最多的连续重复子串 分析: 比较容易理解的部分就是枚举长度为L,然后看长度为L的字符串最多连续出现几次. 既然长度为L的串重复出现,那么str[0],str[l],str ...
随机推荐
- cocos2d-x 3.2 例子文件工程的位置
更新到3.2后突然想要看看官方的例子,忽然发现在test中的cpp工程下面没有了工程的启动配置.奇怪,难道只有代码吗?重新查找后原来启动的工程文件都移动到了build文件夹下面,具体的路径就是 coc ...
- GWT+CodeTemplate+TableCreate快速开发
刚进一家新公司,公司表示让我们几个新人写页面联系熟悉 怎么快速开发,进入正题: 1.根据设计规范设计页面excel 2.CodeTemplate根据excel生成属性类和对应方法(文本框,下拉框等等单 ...
- windows 7 获取SYSTEM权限
当Adobe Reader 9.0卸载之后,你会发现原来的C:\Program Files\Adobe\Reader 9.0\Resource\CMap文件夹下的一些文件无法删除,提示你需要SYSTE ...
- Ubuntu之系统交换分区Swap增加与优化
http://os.51cto.com/art/201212/372860.htm http://blog.csdn.net/xingyu15/article/details/5570225 ...
- LoadRunner执行自动化以及报告自动化的方法
There are three major articles KB articles on Automating LR: 1. Command line arguments for the LoadR ...
- C++拓扑排序
安利一篇比较写的比较好的的博客... 拓扑排序的原理及其实现 我本来以为我看懂了原理就会打了,没想到因为没有手动实践过...原理实际上也没记清楚.... 一题HDU的拓扑裸题HDU 3342 我的拓扑 ...
- Apache开启伪静态后报500错误.
参考:http://blog.163.com/lgh_2002/blog/static/44017526201051452939761/ 加载Rewrite模块: 在conf目录下httpd.conf ...
- HTTP(一) 连接管理
・HTTP是如何使用TCP连接的 HTTP传送一条报文时,以流的形式将报文数据内容通过一条打开的TCP连接按序传输. TCP收到数据流之后,由TCP/IP软件将数据流砍成被称作段的小数据块,并将段封装 ...
- Generate the Jobs script from msdb Database
前两周,由于数据库简繁体的转换,大量的数据库需要转到新的服务器. 在转其中的一台的时候,原先导出来的JOBS脚本不翼而飞(原因至今未明),而恰巧这一台服务器有90多个JOB(看下图恢复后的,注意滚动条 ...
- How to log in to Amazon EC2 using PEM format from SecureCRT
SecureCRT requires both a private and a public key. Use the supplied key.pem file from EC2 here as y ...