Chrome 开发工具之 Application
{
"name": "testApp",
"short_name": "tApp",
"display": "fullscreen",
"background_color": "#000",
"start_url": "/",
"description": "A test web app for manifest",
"icons": [
{
"src": "https://developers.google.com/web/images/web-fundamentals-icon192x192.png",
"type": "image/png",
"sizes": "192x192"
}
]
}
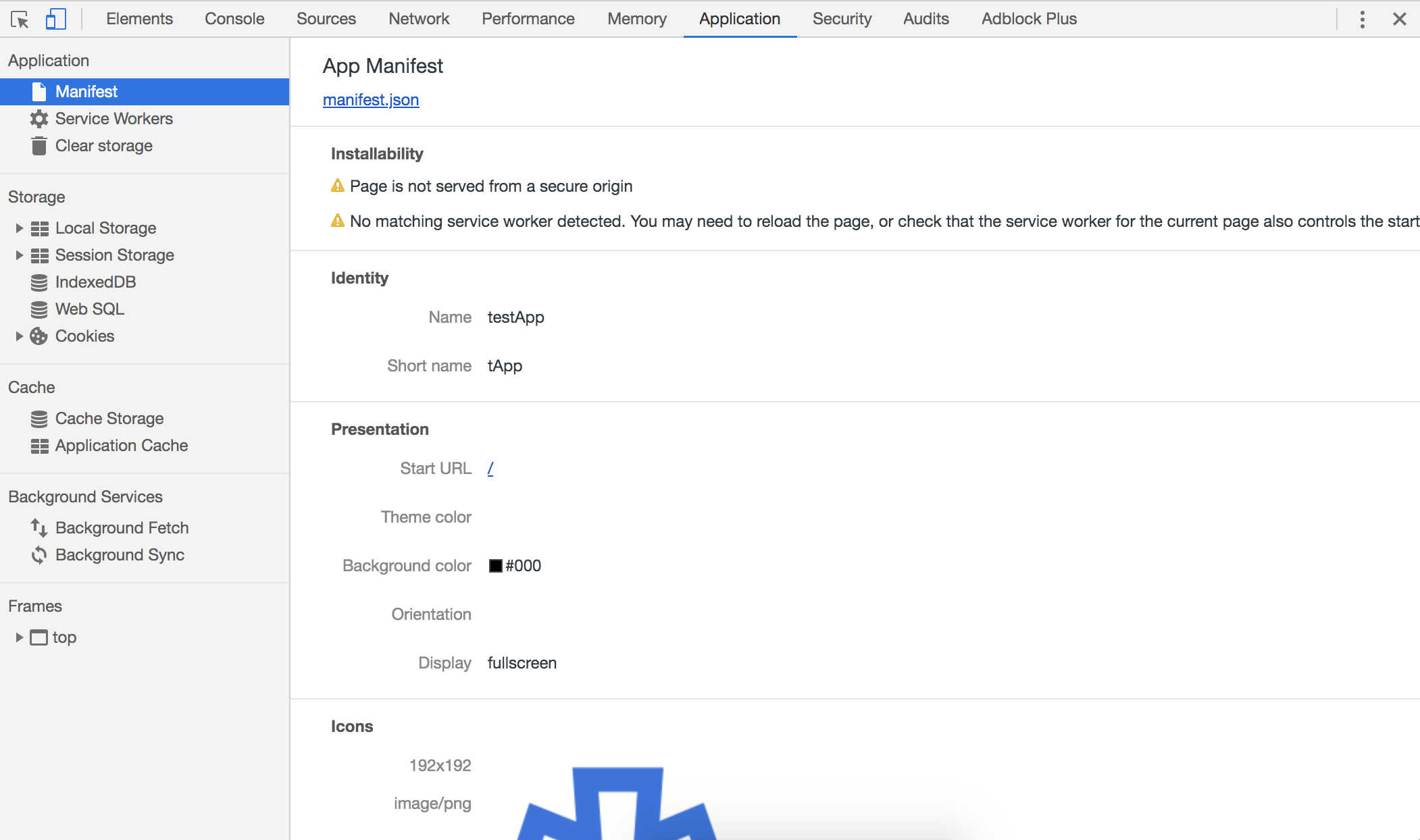
if ('serviceWorker' in navigator) {
const sw = navigator.serviceWorker
.register('/testhtml/sw.js', { scope: '/testhtml/' })
.then(function(reg) {
if (reg.installing) {
console.log('Service worker installing')
} else if (reg.waiting) {
console.log('Service worker installed')
} else if (reg.active) {
console.log('Service worker active')
}
reg.update()
})
.catch(function(error) {
console.log('Registration failed with ' + error)
}) navigator.serviceWorker.ready.then(sw => {
return sw.sync.register('hello-sync')
}) navigator.serviceWorker.ready.then(async sw => {
const bgFetch = await sw.backgroundFetch.fetch('custom-fetch', ['1.jpg'], {
title: 'download 1.jpg',
icons: [
{
sizes: '300x300',
src: '2.jpg',
type: 'image/jpg'
}
]
})
})
}
self.addEventListener('install', event => {
event.waitUntil(
caches.open('v1').then(cache => {
return cache.addAll([
'/testhtml/test.html',
'/testhtml/test.css',
'/testhtml/test.js',
'/testhtml/2.jpg'
])
})
)
}) self.addEventListener('sync', event => {
if (event.tag == 'hello-sync') {
console.log('receive hello-sync')
}
}) self.addEventListener('fetch', event => {
event.respondWith(
caches.match(event.request).then(response => {
if (response !== undefined) {
return response
} else {
return fetch(event.request)
.then(response => {
let responseClone = response.clone()
caches.open('cache_1').then(cache => {
cache.put(event.request, responseClone)
})
return response
})
.catch(() => {})
}
})
)
})
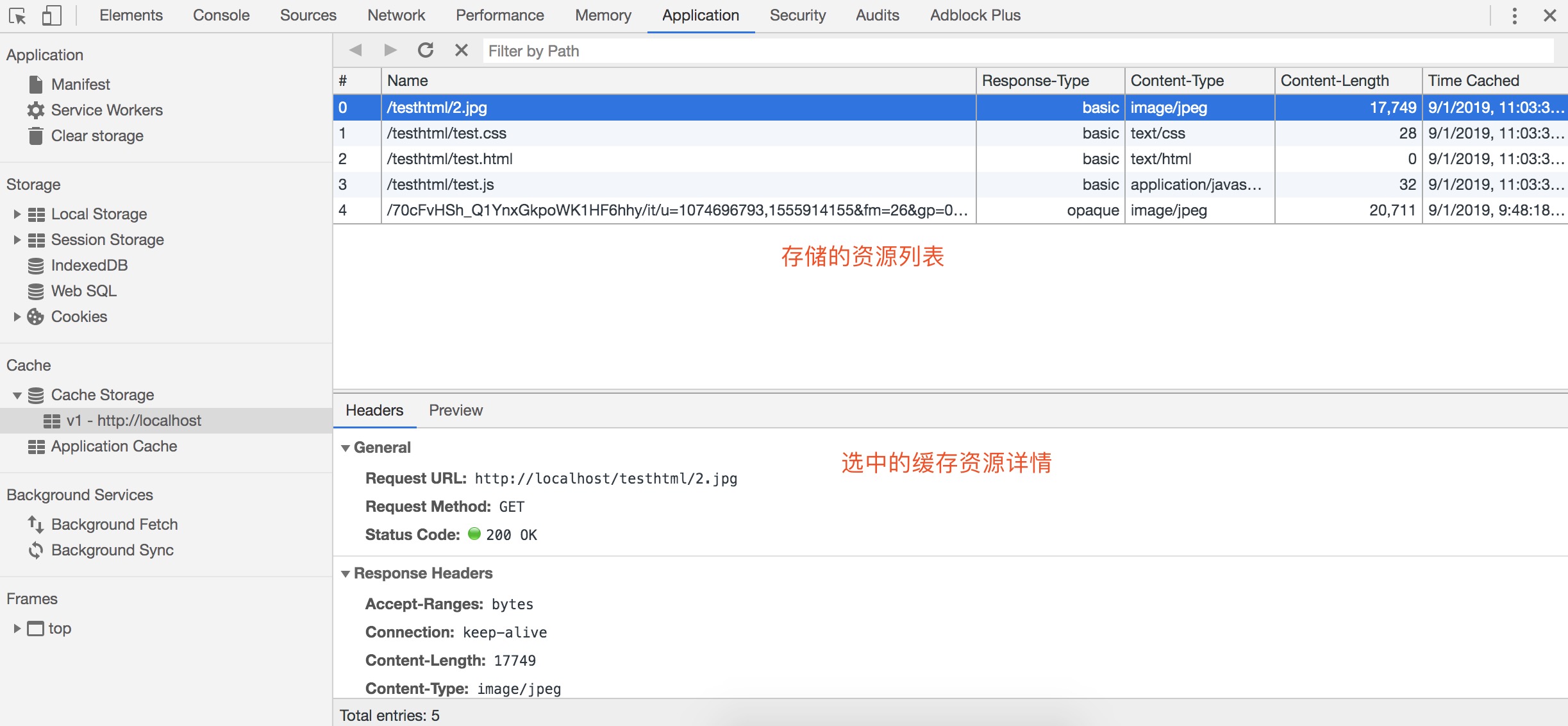
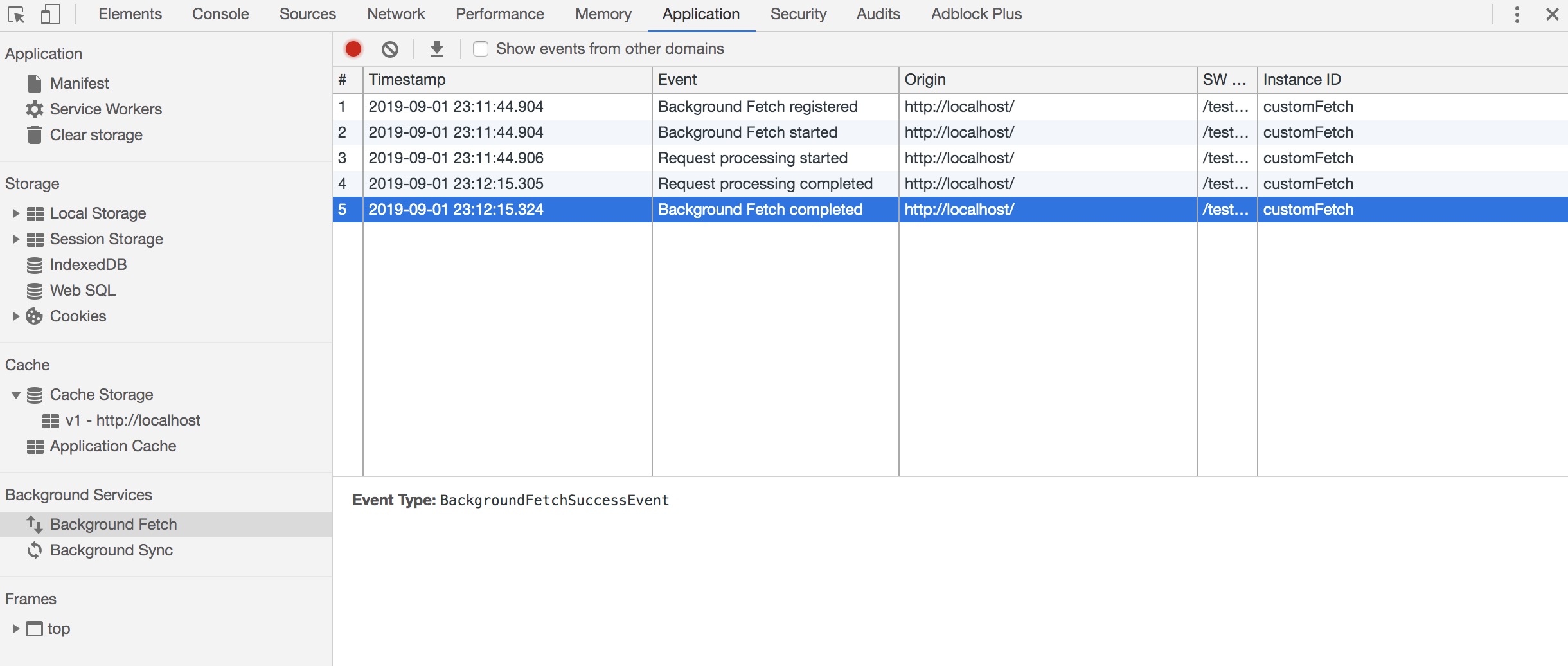
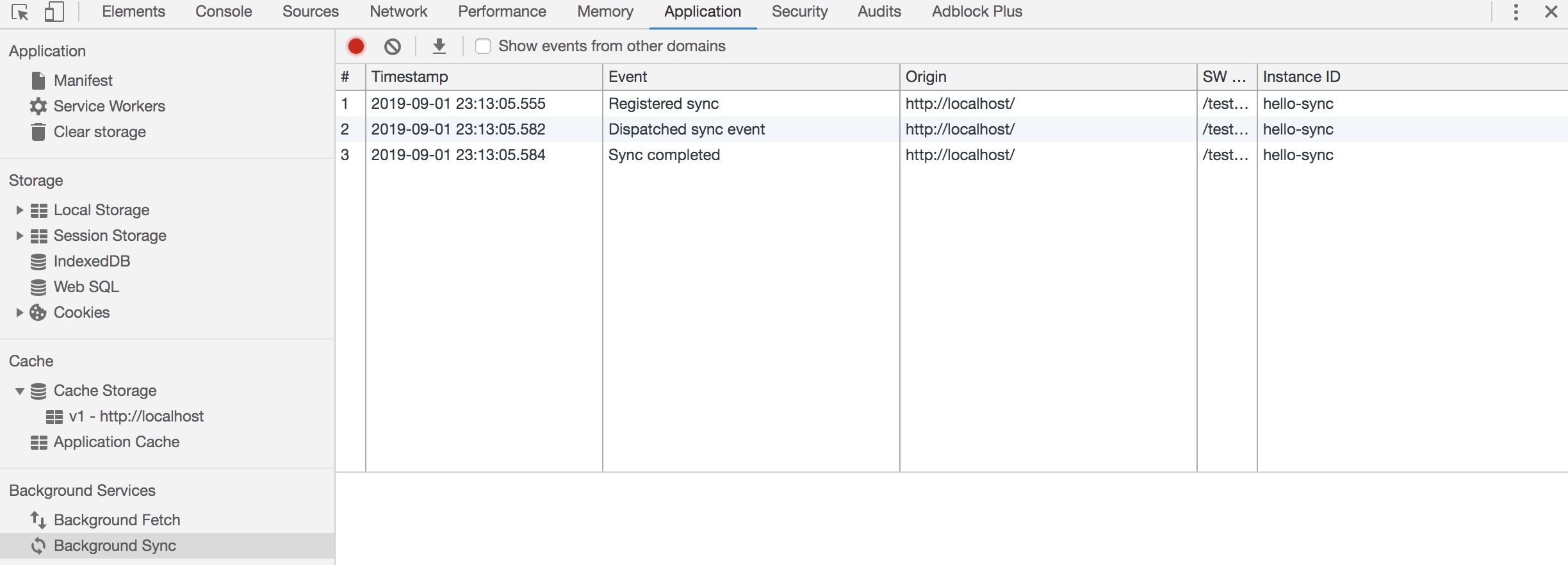
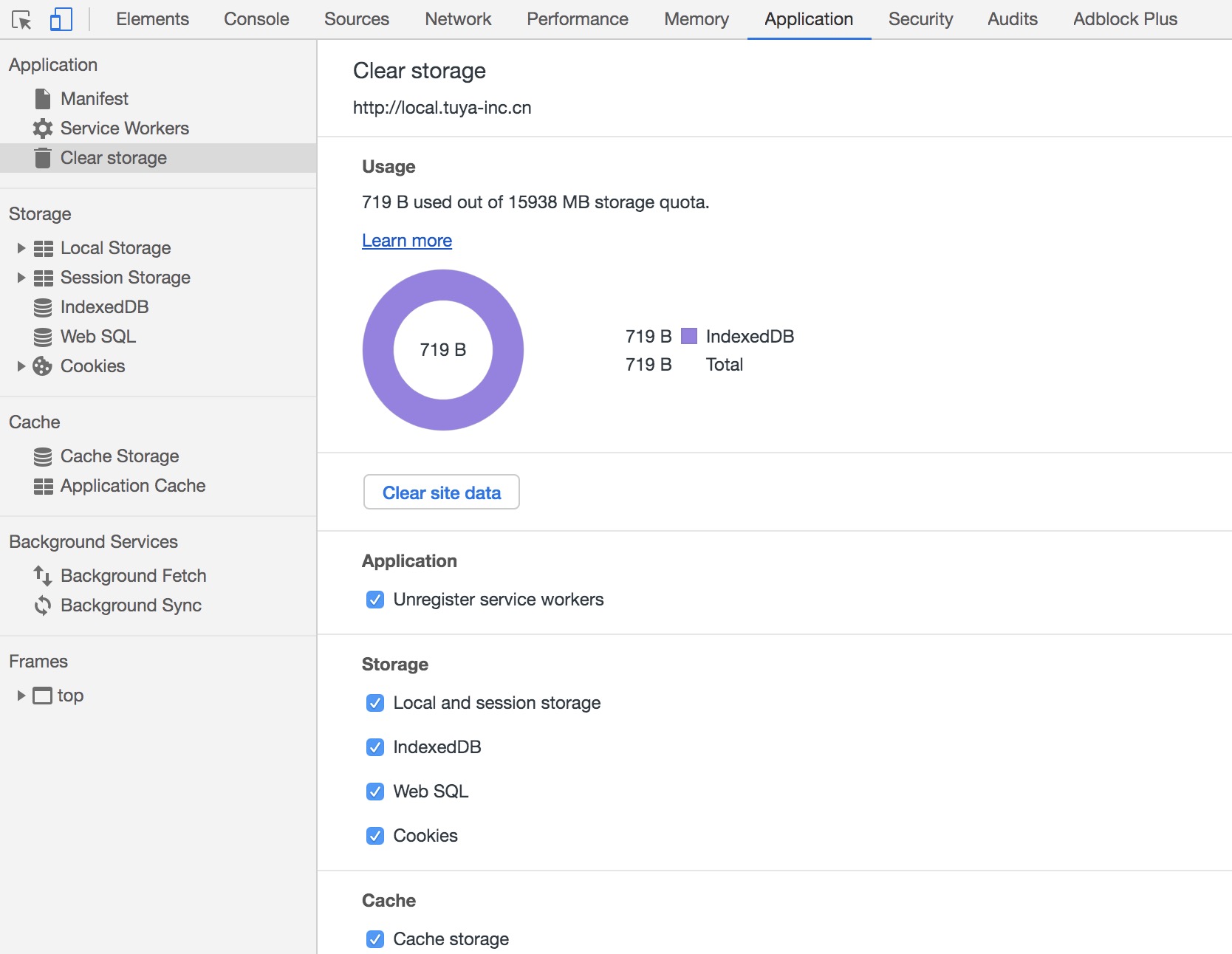
- 卸载 services workers
- Local storage 和 Session storage
- IndexedDB 数据
- Web SQL 数据
- Cookies
- Cache storage
- 图中底下还有 Application Cache 的选项未能截全...
localStorage.setItem('astring', 'This is an apple')
localStorage.setItem('aobject', { say: 'hello' })
localStorage.setItem('aboolean', false)
const IDBOpenDBRequest = indexedDB.open('testDB', 1)
IDBOpenDBRequest.onsuccess = e => {
const db = IDBOpenDBRequest.result
const transaction = db.transaction(['User', 'Book'], 'readwrite')
const objStore = transaction.objectStore('User')
const objBookStore = transaction.objectStore('Book')
// User 表加2条数据
objStore.put({
name: 'xiaoming',
age: 18,
sex: 1
})
objStore.put({
name: 'xiaohong',
age: 18,
sex: 2
})
// Book 表加一条数据
objBookStore.put({
bookName: '< hello world >',
price: 29,
status: 1
})
}
IDBOpenDBRequest.onupgradeneeded = e => {
const db = IDBOpenDBRequest.result
const store = db.createObjectStore('User', {
keyPath: 'name'
})
store.createIndex('name', 'name')
store.createIndex('age', 'age')
store.createIndex('sex', 'sex')
const bookStore = db.createObjectStore('Book', {
keyPath: 'id',
autoIncrement: true
})
bookStore.createIndex('bookName', 'bookName')
bookStore.createIndex('price', 'price')
bookStore.createIndex('status', 'status')
}
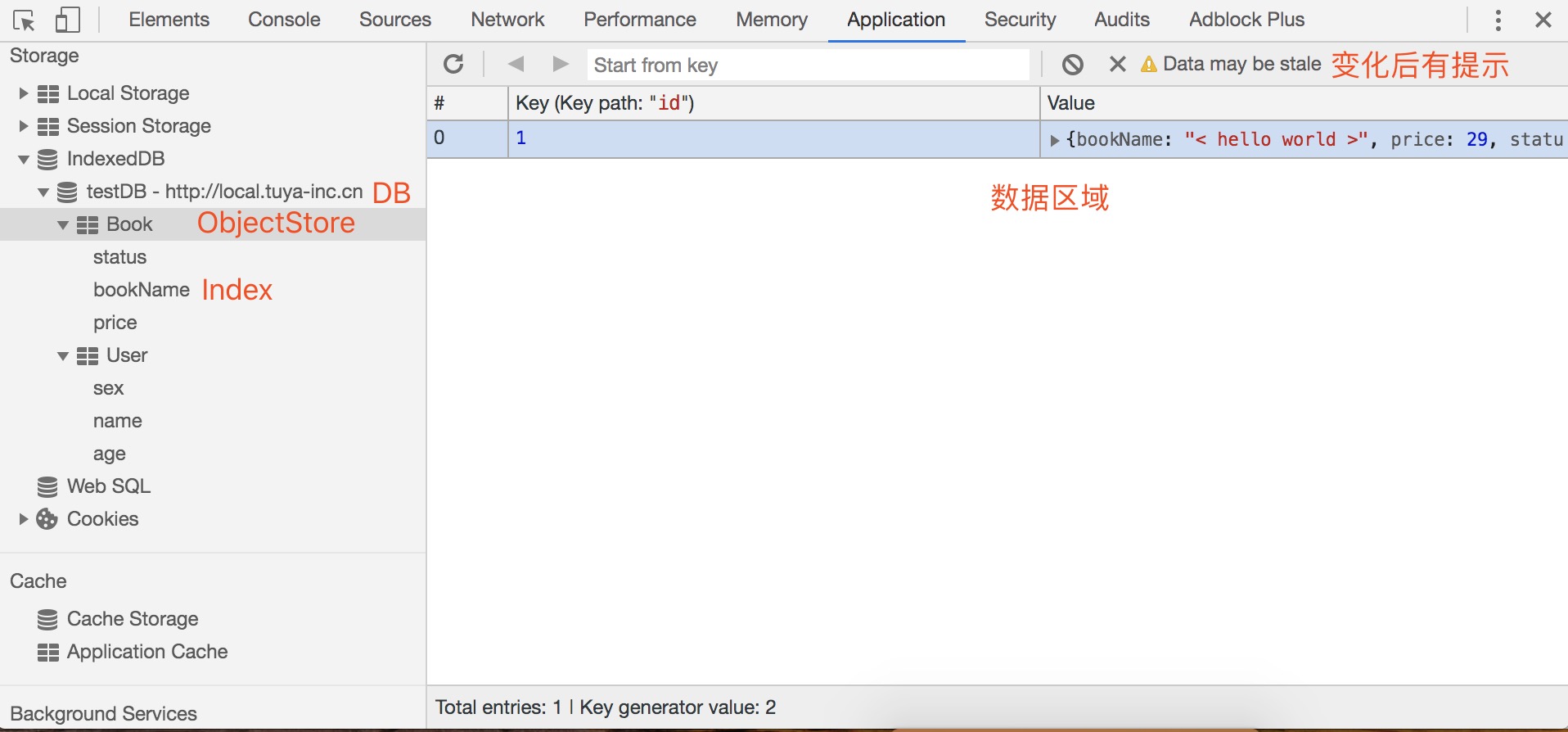
add_header Set-Cookie "say=hello;Domain=local.tuya-inc.cn;Path=/;Max-Age=10000";
Chrome 开发工具之 Application的更多相关文章
- Chrome 开发工具之Timeline
之前有说到Element,Console,Sources大多运用于debug,Network可用于debug和查看性能,今天的主角Timeline更多的是用在性能优化方面,它的作用就是记录与分析应用程 ...
- Chrome 开发工具之Sources
Sources面板主要用于查看web站点的资源列表及javascript代码的debug 熟悉面板 了解完面板之后,下面来试试这些功能都是如何使用的. 文件列表 展示当前页面内所引用资源的列表,和平常 ...
- Chrome 开发工具之Console
前段时间看git的相关,记的笔记也大致写到了博客上,还有些因为运用不熟,或者还有一些疑惑点,暂时也不做过多纠缠,之后在实践中多运用得出结论再整理分享吧. 工欲善其事,必先利其器.要想做好前端的工作,也 ...
- Chrome开发工具之Console
Chrome开发工具-Console 看了别人的博客,才发现在百度主页用开发工具“Console”可以看到百度的招聘信息 前端调试工具可以按F12打开,谷歌的开发工具中的Console面板可以查看错误 ...
- Chrome 开发工具指南
Chrome 开发工具指南 谷歌 Chrome 开发工具,是基于谷歌浏览器内含的一套网页制作和调试工具.开发者工具允许网页开发者深入浏览器和网页应用程序的内部.该工具可以有效地追踪布局问题,设置 Ja ...
- Chrome 开发工具之Timeline/Performance
之前有说到Element,Console,Sources大多运用于debug,Network可用于debug和查看性能,今天的主角Timeline(现已更名Performance)更多的是用在性能优化 ...
- Chrome 开发工具之 Memory
开发过程中难免会遇到内存问题,emmm... 本文主要记录一下Chrome排查内存问题的面板,官网也有,但有些说明和例子跟不上新的版本了,也不够详细... !!! 多图预警!!! 简单的内存 ...
- Chrome开发工具Elements面板(编辑DOM和CSS样式)详解
Element 译为“元素”,Element 面板可以让我们动态查看和编辑DOM节点和CSS样式表,并且立即生效,避免了频繁切换浏览器和编辑器的麻烦. 我们可以使用Element面板来查看源代码,它不 ...
- Chrome 开发工具 Javascript 调试技巧
http://www.w3cplus.com/tools/dev-tips.html 一.Sources 面板介绍: Sources 面板分为左中右 3 部分左:Sources 当前页面加载的资源列表 ...
随机推荐
- 《css的总结》
一.span标签:能让某几个文字或者某个词语凸显出来 <p> 今天是11月份的<span>第一天</span>,地铁卡不打折了 </p> 二.字体风格 ...
- JSP使用分层实现业务处理
在Java开发中,使用JDBC操作数据库的四个步骤如下: ①加载数据库驱动程序(Class.forName("数据库驱动类");) ②连接数据库(Connection co ...
- Divide and Conquer
1 2 218 The Skyline Problem 最大堆 遍历节点 public List<int[]> getSkyline(int[][] buildings) { ...
- 深入理解JVM-java字节码文件结构剖析(1)
public class MyTest1 { private int a = 1; public int getA() { return a; } public void setA(int a) { ...
- ipv6的连接
基础知识不说了,网上一大堆! 基本内容不说了,写字太累了! 只说三点细节,记住就行: 1.ff开头的是多播地址,只能用于udp多播 2.fe80开头的是本地link地址,不管ping也好,connec ...
- Spring MVC浅入浅出——不吹牛逼不装逼
Spring MVC浅入浅出——不吹牛逼不装逼 前言 上文书说了Spring相关的知识,对Spring来了个浅入浅出,大家应该了解到,Spring在三层架构中主做Service层,那还有Web层,也就 ...
- java 遍历map的四种方法
16:21:42 Map.entrySet() 这个方法返回的是一个Set<Map.Entry<K,V>>,Map.Entry 是Map中的一个接口,他的用途是表示一个映射项( ...
- unity 四叉树管理场景
当场景元素过多时,需要实时的显示及隐藏物体使得性能提示,但是物体那么多,怎么知道哪些物体需要显示,哪些物体不需要显示的.当然,遍历物体判断该物体是否可以显示是最容易想到的方法,但是每次更新要遍历所有物 ...
- HlpViewer.exe 单独打开
1.在桌面新建一个快捷键 2.添加HlpViewer.exe 的本地地址 3.在添加的地址后面添加 /catalogName VisualStudio12 4.保存快捷键即可 列: 桌面右键-> ...
- Python模拟登录淘宝
最近想爬取淘宝的一些商品,但是发现如果要使用搜索等一些功能时基本都需要登录,所以就想出一篇模拟登录淘宝的文章!看了下网上有很多关于模拟登录淘宝,但是基本都是使用scrapy.pyppeteer.sel ...