UVA - 12130 Summits
Description
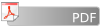
Problem G - Summits
Time limit: 8 seconds
You recently started working for the largest map drawing company in theNetherlands. Part of your job is to determine what the summits in aparticular landscape are. Unfortunately, it is not so easy to determinewhich points are summits and which are not, because
we do not want tocall a small hump a summit. For example look at the landscape given bythe sample input.
We call the points of height 3 summits, since there are no higherpoints. But although the points of height 2, which are to theleft of the summit of height 3, are all higher than or equal totheir immediate neighbours, we do notwant to call them summits, because
we can reach a higher point fromthem without going to low (the summits of height 3). In contrast,we do want to call the area of height 2 on the right a summit, sinceif we would want to walk to the summit of height 3, we first have todescend to a point with
height 0.
After the above example, we introduce the concept of a d-summit. Apoint, with height
h, is a d-summit if and only if it isimpossible to reach a higher point without going through an area withheight smaller than or equal to
h-d.
The problem is, given a rectangular grid of integer heights and aninteger
d, to find the number of d-summits.
Input
On the first line one positive number: the number of testcases, atmost 100. After that per testcase:
- One line with three integers 1 ≤ h ≤ 500, 1 &le w ≤ 500 and 1 ≤
d ≤ 1000000000. h and w are the dimensions of the map.
d is as defined in the text. - h lines with w integers, where the xth integer on the
yth line denotes the height 0 ≤ h ≤ 1000000000 of the point (x,
y).
Output
Per testcase:
- One line with the number of summits.
Sample Input
1
6 10 2
0 0 0 0 0 0 0 0 0 0
0 1 2 1 1 1 1 0 1 0
0 2 1 2 1 3 1 0 0 0
0 1 2 1 3 3 1 1 0 0
0 2 1 2 1 1 1 0 2 0
0 0 0 0 0 0 0 0 0 0
Sample Output
4
题意:多么费解的题目啊,找顶点,假设一个点是最高的话那么就是顶点。假设不是的话,可是它到比它到的点的路径中假设有<=h-d(h为该点的高度)那么就不能去,那么它就是顶点
思路:首先找个性质:假设A->B,C->B,假设HA>HC,由于HA-d>HC-d,那么C->A,所以我们先按高度排序,然后逐个BFS,假设它的周围能找到跟它一样高的点。那么这些点都是顶点。假设遇到已经被较高找到的点。那么它就也能够到那个较高的点。那么它就不是顶点
#include <iostream>
#include <cstring>
#include <cstdio>
#include <vector>
#include <algorithm>
#include <queue>
using namespace std;
const int MAXN = 510; struct node {
int x, y, h;
node(int _x = 0, int _y = 0, int _h = 0) {
x = _x;
y = _y;
h = _h;
}
} arr[MAXN*MAXN];
int map[MAXN][MAXN];
int vis[MAXN][MAXN];
int n, m, d;
int cnt;
int dx[4]={1, -1, 0, 0};
int dy[4]={0, 0, 1, -1};
queue<node> q; int cmp(node a, node b) {
return a.h > b.h;
} void cal() {
memset(vis, -1, sizeof(vis));
int ans = 0;
while (!q.empty())
q.pop();
for (int i = 0; i < cnt; i++) {
node cur = arr[i];
if (vis[cur.x][cur.y] != -1)
continue;
int flag = 1;
int bound = cur.h - d;
int top = cur.h;
q.push(cur);
int tot = 1;
while (!q.empty()) {
cur = q.front();
q.pop();
vis[cur.x][cur.y] = top;
for (int i = 0; i < 4; i++) {
int nx = cur.x + dx[i];
int ny = cur.y + dy[i];
if (nx < 1 || ny < 1 || nx > n || ny > m)
continue;
if (map[nx][ny] <= bound)
continue;
if (vis[nx][ny] != -1) {
if (vis[nx][ny] != top)
flag = 0;
continue;
}
node tmp;
tmp.x = nx, tmp.y = ny, tmp.h = map[nx][ny];
vis[nx][ny] = top;
if (tmp.h == top)
tot++;
q.push(tmp);
}
}
if (flag)
ans += tot;
}
printf("%d\n", ans);
} int main() {
int t;
scanf("%d", &t);
while (t--) {
scanf("%d%d%d", &n, &m, &d);
cnt = 0;
for (int i = 1; i <= n; i++)
for (int j = 1; j <= m; j++) {
scanf("%d", &map[i][j]);
arr[cnt++] = node(i, j, map[i][j]);
}
sort(arr, arr+cnt, cmp);
cal();
}
return 0;
}
版权声明:本文博客原创文章,博客,未经同意,不得转载。
UVA - 12130 Summits的更多相关文章
- UVA 12130 - Summits(BFS+贪心)
UVA 12130 - Summits 题目链接 题意:给定一个h * w的图,每一个位置有一个值.如今要求出这个图上的峰顶有多少个.峰顶是这样定义的.有一个d值,假设一个位置是峰顶.那么它不能走到不 ...
- uva 1354 Mobile Computing ——yhx
aaarticlea/png;base64,iVBORw0KGgoAAAANSUhEUgAABGcAAANuCAYAAAC7f2QuAAAgAElEQVR4nOy9XUhjWbo3vu72RRgkF5
- UVA 10564 Paths through the Hourglass[DP 打印]
UVA - 10564 Paths through the Hourglass 题意: 要求从第一层走到最下面一层,只能往左下或右下走 问有多少条路径之和刚好等于S? 如果有的话,输出字典序最小的路径 ...
- UVA 11404 Palindromic Subsequence[DP LCS 打印]
UVA - 11404 Palindromic Subsequence 题意:一个字符串,删去0个或多个字符,输出字典序最小且最长的回文字符串 不要求路径区间DP都可以做 然而要字典序最小 倒过来求L ...
- UVA&&POJ离散概率与数学期望入门练习[4]
POJ3869 Headshot 题意:给出左轮手枪的子弹序列,打了一枪没子弹,要使下一枪也没子弹概率最大应该rotate还是shoot 条件概率,|00|/(|00|+|01|)和|0|/n谁大的问 ...
- UVA计数方法练习[3]
UVA - 11538 Chess Queen 题意:n*m放置两个互相攻击的后的方案数 分开讨论行 列 两条对角线 一个求和式 可以化简后计算 // // main.cpp // uva11538 ...
- UVA数学入门训练Round1[6]
UVA - 11388 GCD LCM 题意:输入g和l,找到a和b,gcd(a,b)=g,lacm(a,b)=l,a<b且a最小 g不能整除l时无解,否则一定g,l最小 #include &l ...
- UVA - 1625 Color Length[序列DP 代价计算技巧]
UVA - 1625 Color Length 白书 很明显f[i][j]表示第一个取到i第二个取到j的代价 问题在于代价的计算,并不知道每种颜色的开始和结束 和模拟赛那道环形DP很想,计算这 ...
- UVA - 10375 Choose and divide[唯一分解定理]
UVA - 10375 Choose and divide Choose and divide Time Limit: 1000MS Memory Limit: 65536K Total Subm ...
随机推荐
- OCP读书笔记(3) - 使用RMAN恢复目录
创建恢复目录 在hndx上创建恢复目录:[oracle@oracle admin]$ export ORACLE_SID=hndx[oracle@oracle admin]$ sqlplus / as ...
- Android真机网络adb联机调试初探
新项目是一个基于android4.2设备.刚拿到demo板时就对联机互调感兴趣了.处于以前在S3c2440上对linux的移植使用经验.心里猜测对于android设备应该也这样.所以通过搜索资料整理如 ...
- PowerDesigner中SQL文件、数据库表反向生成PDM
1 反向生成PDM 1) 创建一个空的PDM模型(选择相应的DBMS): 2) 选择[Database]--[Update Model from Database ...
- Java设置的读书笔记和集合框架Collection API
一个.CollectionAPI 集合是一系列对象的聚集(Collection). 集合在程序设计中是一种重要的数据接口.Java中提供了有关集合的类库称为CollectionAPI. 集合实际上是用 ...
- hdu1114(完全背包)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1114 分析:很裸的一道完全背包题,只是这里求装满背包后使得价值最少,只需初始化数组dp为inf:dp[ ...
- sar使用说明
sar这东西,一开始还以为是内部有的,原来是外部的工具,可以到 http://pagesperso-orange.fr/sebastien.godard/download.html 去下载 1 安装 ...
- java解析String类型t复杂xml,多级节点,最好的例子
需要用jar包 dom4j-1.6.1.jar 字符串xml如下: <root> <flw> <name>aa</name> <age>22 ...
- jeecg 3.5.2 新版本号4种首页风格 【经典风格,shortcut风格,ACE bootstrap风格,云桌面风格】
[1]经典风格: [2]Shortcut风格: [3]ACE bootsrap风格: [4]云桌面风格: [5]自己定义图表 watermark/2/text/aHR0cDovL2Jsb2cuY3Nk ...
- python面向对象具体解释(上)
创建类 Python 类使用 class 关键字来创建.简单的类的声明能够是关键字后紧跟类名: class ClassName(bases): 'class documentation string' ...
- (转载)浅析error LNK2001: unresolved external symbol "public: __thisc...
学习VC++时经常会遇到链接错误LNK2001,该错误非常讨厌,因为对于 编程者来说,最好改的错误莫过于编译错误,而一般说来发生连接错误时, 编译都已通过.产生连接错误的原因非常多 ...