hdu1541 Stars
the distribution of the levels of the stars.
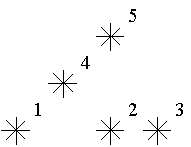
For example, look at the map shown on the figure above. Level of the star number 5 is equal to 3 (it's formed by three stars with a numbers 1, 2 and 4). And the levels of the stars numbered by 2 and 4 are 1. At this map there are only one star of the level
0, two stars of the level 1, one star of the level 2, and one star of the level 3.
You are to write a program that will count the amounts of the stars of each level on a given map.
are listed in ascending order of Y coordinate. Stars with equal Y coordinates are listed in ascending order of X coordinate.
1 1
5 1
7 1
3 3
5 5
2
1
10
这题是简单的线段树应用,好像也可以用树状数组做,以后学了再做一次(笑)。因为题目中的坐标是先按纵坐标大小,再按横坐标大小排列的,所以一个左边的值就是比它横坐标小的值再加上它下面的坐标。可以用线段树维护区间的小于等于区间右端点的横坐标的个数,这里查询和更新放在了一起,从根节点到叶子节点是查询,再从叶子节点递归上来是询问。
#include<iostream>
#include<stdio.h>
#include<string.h>
#include<math.h>
#include<vector>
#include<map>
#include<queue>
#include<stack>
#include<string>
#include<algorithm>
using namespace std;
#define maxn 32005
int num,vis[15005];
struct node{
int l,r,num;
}b[8*maxn];
void build(int l,int r,int i)
{
int mid;
b[i].l=l;b[i].r=r;b[i].num=0;
if(l==r)return;
mid=(l+r)/2;
build(l,mid,i*2);
build(mid+1,r,i*2+1);
}
void update(int id,int i)
{
int mid;
if(b[i].l==b[i].r){
num+=b[i].num;
b[i].num++;return;
}
if(b[i*2].r>=id){
update(id,i*2);
}
else{
num+=b[i*2].num;
update(id,i*2+1);
}
b[i].num=b[i*2].num+b[i*2+1].num;
}
int main()
{
int n,m,i,j,c,d;
while(scanf("%d",&n)!=EOF)
{
memset(vis,0,sizeof(vis));
build(0,32005,1);
for(i=1;i<=n;i++){
scanf("%d%d",&c,&d);
num=0;
update(c,1);
vis[num]++;
}
for(i=0;i<=n-1;i++){
printf("%d\n",vis[i]);
}
}
return 0;
}
hdu1541 Stars的更多相关文章
- HDU-1541 Stars 树状数组
题目链接:https://cn.vjudge.net/problem/HDU-1541 题意 天上有许多星星 现给天空一个平面坐标轴,统计每个星星的level, level是指某一颗星星的左下角(x& ...
- hdu1541 Stars 树状数组
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1541 题目大意就是统计其左上位置的星星的个数 由于y已经按升序排列,因此只用按照x坐标生成一维树状数组 ...
- 树状数组入门 hdu1541 Stars
树状数组 树状数组(Binary Indexed Tree(B.I.T), Fenwick Tree)是一个查询和修改复杂度都为log(n)的数据结构.主要用于查询任意两位之间的所有元素之和,但是每次 ...
- [学习笔记]CDQ分治和整体二分
序言 \(CDQ\) 分治和整体二分都是基于分治的思想,把复杂的问题拆分成许多可以简单求的解子问题.但是这两种算法必须离线处理,不能解决一些强制在线的题目.不过如果题目允许离线的话,这两种算法能把在线 ...
- poj 2352 Stars 数星星 详解
题目: poj 2352 Stars 数星星 题意:已知n个星星的坐标.每个星星都有一个等级,数值等于坐标系内纵坐标和横坐标皆不大于它的星星的个数.星星的坐标按照纵坐标从小到大的顺序给出,纵坐标相同时 ...
- HDU1541 树状数组
Stars Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others)Total Submi ...
- POJ 2352 Stars(树状数组)
Stars Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 30496 Accepted: 13316 Descripti ...
- 【POJ-2482】Stars in your window 线段树 + 扫描线
Stars in Your Window Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 11706 Accepted: ...
- Java基础之在窗口中绘图——填充星型(StarApplet 2 filled stars)
Applet程序. import javax.swing.*; import java.awt.*; import java.awt.geom.GeneralPath; @SuppressWarnin ...
随机推荐
- LeetCode222 判断是否为完全二叉树并求节点个数
给出一个完全二叉树,求出该树的节点个数. 说明: 完全二叉树的定义如下:在完全二叉树中,除了最底层节点可能没填满外,其余每层节点数都达到最大值,并且最下面一层的节点都集中在该层最左边的若干位置.若最底 ...
- Count PAT's (25) PAT甲级真题
题目分析: 由于本题字符串长度有10^5所以直接暴力是不可取的,猜测最后的算法应该是先预处理一下再走一层循环就能得到答案,所以本题的关键就在于这个预处理的过程,由于本题字符串匹配的内容的固定的PAT, ...
- (十六)re模块
正则表达式并不是Python的一部分,本质而言,正则表达式(或 RE)是一种小型的.高度专业化的编程语言.正则表达式是用于处理字符串的强大工具,很多编程语言都支持正则表达式的语法. 字符匹配分为普通字 ...
- uni-app 微信小程序 picker 三级联动
之前做过一个picker的三级联动功能,这里分享代码给大家 具体代码: // An highlighted block <template> <view> <picker ...
- Android事件分发机制五:面试官你坐啊
前言 很高兴遇见你~ 事件分发系列文章已经到最后一篇了,先来回顾一下前面四篇,也当个目录: Android事件分发机制一:事件是如何到达activity的? : 从window机制出发分析了事件分发的 ...
- centos6-centos7防火墙(iptables-firewalld)设置端口nat转发
背景: 将本机的 8080端口转发至其他主机,主机 IP:192.168.1.162,目标主机 IP和端口192.168.1.163:80,方法如下: centos6系统iptables环境下: ip ...
- VMware中安装Ubuntu后,安装VMwareTools提示“Not enough free space to extract VMwareTools-10.3.10-13959562.tar.gz”的解决办法
将加载后的Vmware Tools中的*.tar.gz文件复制到桌面后提取,否则会报错:
- 避免重复提交?分布式服务的幂等性设计! 架构文摘 今天 点击蓝色“架构文摘”关注我哟 加个“星标”,每天上午 09:25,干货推送! 来源:https://www.cnblogs.com/QG-whz/p/10372458.html 作者:melonstreet
避免重复提交?分布式服务的幂等性设计! 架构文摘 今天 点击蓝色"架构文摘"关注我哟 加个"星标",每天上午 09:25,干货推送! 来源:h ...
- Most basic operations in Go are not synchronized. In other words, they are not concurrency-safe.
Most basic operations in Go are not synchronized. In other words, they are not concurrency-safe. htt ...
- Coded UI
Coded UI Test是Visual Studio 2010对于Testing Project(测试工程)提供的关于UI自动化测试的框架,支持Win32,Web,WPF等UI的自动化测试,是一个非 ...