UVA 1665 Islands
题意:输入一个n*m矩阵,每一个格子都有一个正整数,再输入T个整数ti,对于每一个ti,输出大于ti的正整数组成多少个四连快
思路:正着做的话事实上相当于删除连通块,而假设反着做的话就相当于变成添加连通块,把格子都编号然后排序。用并查集
#include<bits/stdc++.h>
using namespace std;
const int maxn = 1005;
const int maxq = 1e5+1;
int pre[maxn*maxn];
int Find(int x)
{
return x==pre[x]?x:pre[x]=Find(pre[x]);
}
struct Node
{
int x,y;
int val;
}nodes[1005*1005];
int mapp[maxn][maxn];
int qq[maxq];
int n,m;
int dir[4][2]={{0,1},{0,-1},{1,0},{-1,0}};
bool cmp(Node a,Node b)
{
return a.val<b.val;
}
int main()
{
int T;
scanf("%d",&T);
while (T--)
{
scanf("%d%d",&n,&m);
for (int i = 0;i<n;i++)
for (int j = 0;j<m;j++)
{
scanf("%d",&mapp[i][j]);
int pos = i*m+j;
nodes[pos].x=i;
nodes[pos].y=j;
nodes[pos].val=mapp[i][j];
}
int ans = 0;
int q;
scanf("%d",&q);
for (int i = 0;i<q;i++)
scanf("%d",&qq[i]);
memset(pre,-1,sizeof(pre));
sort(nodes,nodes+n*m,cmp);
int k = n*m-1;
for (int i = q-1;i>=0;i--)
{
if (qq[i]< nodes[k].val)
{
while (k>=0 && qq[i]<nodes[k].val)
{
int pos = nodes[k].x*m+nodes[k].y;
if (!~pre[pos])
pre[pos]=pos,ans++;
for (int di=0;di<4;di++)
{
int dx = nodes[k].x+dir[di][0];
int dy = nodes[k].y+dir[di][1];
if (dx>=0 && dx<n&&dy>=0&&dy<m&&mapp[dx][dy]>qq[i])
{
int ppos = dx*m+dy;
if (~pre[ppos])
{
int u = Find(ppos);
int v = Find(pos);
if (u!=v)
pre[u]=v,ans--;
}
}
}
k--;
}
if (k<0)
{
for (;i>=0;i--)
{
qq[i]=ans;
}
break;
}
}
qq[i]=ans;
}
for (int i = 0;i<q;i++)
printf("%d ",qq[i]);
printf("\n");
}
}
Description
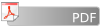
Deep in the Carribean, there is an island even stranger than the Monkey Island, dwelled by Horatio Torquemada Marley. Not only it has a rectangular shape, but is also divided into an nxm grid.
Each grid field has a certain height. Unfortunately, the sea level started to raise and in year i, the level is i meters. Another strange feature of the island is that it is made of sponge,
and the water can freely flow through it. Thus, a grid field whose height is at most the current sea level is considered flooded. Adjacent unflooded fields (i.e., sharing common edge) create unflooded areas. Sailors are interested
in the number of unflooded areas in a given year.
An example of a 4 x 5 island is given below. Numbers denote the heights of respective fields in meters. Unflooded fields are darker; there are two unflooded areas in the first
year and three areas in the second year.
Input
The input contains several test cases. The first line of the input contains a positive integer Z20,
denoting the number of test cases. ThenZ test cases follow, each conforming to the format described below.
The first line contains two numbers n and m separated by a single space, the dimensions of the island, where 1n, m
1000.
Next nlines contain m integers from the range [1, 109] separated by single spaces, denoting the heights of the respective fields. Next line contains
an integer T(1T
105).
The last line contains T integers tj, separated by single spaces, such that 0t1
t2
...
tT-1
tT
109.
Output
For each test case, your program has to write an output conforming to the format described below.
Your program should output a single line consisting of T numbers rj separated by single spaces, where rj is the number of unflooded
areas in year tj.
cid=113332" style="color:blue; text-decoration:none">Sample Input
1
4 5
1 2 3 3 1
1 3 2 2 1
2 1 3 4 3
1 2 2 2 2
5
1 2 3 4 5
cid=113332" style="color:blue; text-decoration:none">Sample Output
2 3 1 0 0
UVA 1665 Islands的更多相关文章
- 紫书 习题 11-12 UVa 1665 (并查集维护联通分量)
这道题要逆向思维 反过来从大到小枚举, 就是在矩阵中一点一点加进去数字,这样比较 好操作, 如果正着做就要一点一点删除数字, 不好做. 我们需要在这个过程中维护联通块的个数, 这里用到了并查集. 首先 ...
- UVA 572 油田连通块-并查集解决
题意:8个方向如果能够连成一块就算是一个连通块,求一共有几个连通块. 分析:网上的题解一般都是dfs,但是今天发现并查集也可以解决,为了方便我自己理解大神的模板,便尝试解这道题目,没想到过了... # ...
- [LeetCode] Number of Islands II 岛屿的数量之二
A 2d grid map of m rows and n columns is initially filled with water. We may perform an addLand oper ...
- [LeetCode] Number of Islands 岛屿的数量
Given a 2d grid map of '1's (land) and '0's (water), count the number of islands. An island is surro ...
- uva 1354 Mobile Computing ——yhx
aaarticlea/png;base64,iVBORw0KGgoAAAANSUhEUgAABGcAAANuCAYAAAC7f2QuAAAgAElEQVR4nOy9XUhjWbo3vu72RRgkF5
- UVA 10564 Paths through the Hourglass[DP 打印]
UVA - 10564 Paths through the Hourglass 题意: 要求从第一层走到最下面一层,只能往左下或右下走 问有多少条路径之和刚好等于S? 如果有的话,输出字典序最小的路径 ...
- UVA 11404 Palindromic Subsequence[DP LCS 打印]
UVA - 11404 Palindromic Subsequence 题意:一个字符串,删去0个或多个字符,输出字典序最小且最长的回文字符串 不要求路径区间DP都可以做 然而要字典序最小 倒过来求L ...
- UVA&&POJ离散概率与数学期望入门练习[4]
POJ3869 Headshot 题意:给出左轮手枪的子弹序列,打了一枪没子弹,要使下一枪也没子弹概率最大应该rotate还是shoot 条件概率,|00|/(|00|+|01|)和|0|/n谁大的问 ...
- UVA计数方法练习[3]
UVA - 11538 Chess Queen 题意:n*m放置两个互相攻击的后的方案数 分开讨论行 列 两条对角线 一个求和式 可以化简后计算 // // main.cpp // uva11538 ...
随机推荐
- 静态区间第k大 树套树解法
然而过不去你谷的模板 思路: 值域线段树\([l,r]\)代表一棵值域在\([l,r]\)范围内的点构成的一颗平衡树 平衡树的\(BST\)权值为点在序列中的位置 查询区间第\(k\)大值时 左区间在 ...
- 大型C++项目必须注意的几个小问题
大型C++项目必须注意的几个小问题 有些问题对于小型的C++项目来说可能无关紧要,但对于大中型C++项目来讲,这些问题却成了大问题.什么样的项目算是小型项目呢,什么样的算是大中型项目呢,我认为10万L ...
- JavaScript各变量类型的判断方法
我们很容易被漂亮的代码吸引,也不知不觉的在自己的代码库中加入这些.却没有冷静的想过它们的优劣.这不,我就收集了一系列形如 "是否为……?" 的判断的boolean函数. isNul ...
- 3.Docker与LXC、虚拟化技术的区别——虚拟化技术本质上是在模拟硬件,Docker底层是LXC,本质都是cgroups是在直接操作硬件
先说和虚拟化技术的区别 难道虚拟技术就做不到吗? 不不不,虚拟技术也可以做到,但是会有一定程度的性能损失,灵活度也会下降.容器技术不是模仿硬件层次,而是 在Linux内核里使用cgroup和names ...
- 转:ListView中getView的工作原理
ListView中getView的工作原理: [1]ListView asks adapter “give me a view” (getView) for each item of the list ...
- Mysql性能优化【转】
mysql的性能优化无法一蹴而就,必须一步一步慢慢来,从各个方面进行优化,最终性能就会有大的提升. Mysql数据库的优化技术 对mysql优化是一个综合性的技术,主要包括 表的设计合理化(符合3NF ...
- switch与if 性能测试
测试结果:switch性能更高. 测试过程:新建一个Win32 Console Application, 在cpp文件中添加下面代码 #include "stdafx.h" #in ...
- msvc交叉编译:使用vcvarsall.bat设置命令行编译环境
一直以来我只知道vc设置命令行编译环境的批处理命令是%VS140COMNTOOLS%/Common7/Tools下的vsvars32.bat,(%VS140COMNTOOLS%为定义vs2015公共工 ...
- python tips;matplotlib 显示中文
import numpy as npimport matplotlib.pyplot as pltimport matplotlib as mpl mpl.rcParams['axes.unicode ...
- hdu 5109(构造数+对取模的理解程度)
Alexandra and A*B Problem Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Jav ...