poj1470 Closest Common Ancestors [ 离线LCA tarjan ]
Time Limit: 2000MS | Memory Limit: 10000K | |
Total Submissions: 14915 | Accepted: 4745 |
Description
Input
nr_of_vertices
vertex:(nr_of_successors) successor1 successor2 ... successorn
...
where vertices are represented as integers from 1 to n ( n <= 900 ). The tree description is followed by a list of pairs of vertices, in the form:
nr_of_pairs
(u v) (x y) ...
The input file contents several data sets (at least one).
Note that white-spaces (tabs, spaces and line breaks) can be used freely in the input.
Output
For example, for the following tree:
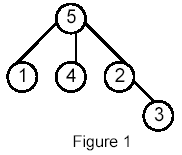
Sample Input
5
5:(3) 1 4 2
1:(0)
4:(0)
2:(1) 3
3:(0)
6
(1 5) (1 4) (4 2)
(2 3)
(1 3) (4 3)
Sample Output
2:1
5:5
Hint
Source
思路:离线lca ,利用tarjan算法。
tarjan算法的步骤是(当dfs到节点u时):
1. 在并查集中建立仅有u的集合,设置该集合的祖先为u
2. 对u的每个孩子v:
2.1 tarjan之
2.2 合并v到父节点u的集合,确保集合的祖先是u
3. 设置u为已遍历
4. 处理关于u的查询,若查询(u,v)中的v已遍历过,则LCA(u,v)=v所在的集合的祖先
14006525 | njczy2010 | 1470 | Accepted | 2992K | 516MS | G++ | 1979B | 2015-03-25 19:29:20 |
#include <cstdio>
#include <cstring>
#include <stack>
#include <vector>
#include <algorithm> #define ll long long
int const N = ;
int const M = ;
int const inf = ;
ll const mod = ; using namespace std; int n,m;
vector<int> bian[N];
vector<int> query[N];
int cnt[N];
int fa[N];
int vis[N];
int degree[N]; int findfa(int x)
{
return fa[x] == x ? fa[x] : fa[x] = findfa(fa[x]);
} void ini()
{
int i,j;
int k,u,v;
memset(cnt,,sizeof(cnt));
memset(vis,,sizeof(vis));
memset(degree,,sizeof(degree));
for(i=;i<=n;i++){
bian[i].clear();
query[i].clear();
fa[i]=i;
}
for(i=;i<=n;i++){
scanf("%d:(%d)",&u,&k);
for(j=;j<k;j++){
scanf("%d",&v);
bian[u].push_back(v);
degree[v]++;
}
}
scanf("%d",&m);
for(i=;i<=m;i++){
while(getchar()!='(') ;
scanf("%d%d",&u,&v);
while(getchar()!=')') ;
query[u].push_back(v);
query[v].push_back(u);
}
} void tarjan(int u,int f)
{
vector<int>::iterator it;
int v;
for(it=bian[u].begin();it!=bian[u].end();it++){
v=*it;
tarjan(v,u);
} for(it=query[u].begin();it!=query[u].end();it++){
v=*it;
if(vis[v]==) continue;
cnt[ findfa(v) ]++;
}
vis[u]=;
fa[u]=f;
} void solve()
{
int i;
for(i=;i<=n;i++){
if(degree[i]==){
tarjan(i,-);
}
}
} void out()
{
int i;
for(i=;i<=n;i++){
// printf("%d:%d\n",i,cnt[i]);
if(cnt[i]!=){
printf("%d:%d\n",i,cnt[i]);
}
}
} int main()
{
//freopen("data.in","r",stdin);
//scanf("%d",&T);
// for(cnt=1;cnt<=T;cnt++)
//while(T--)
while(scanf("%d",&n)!=EOF)
{
ini();
solve();
out();
}
}
poj1470 Closest Common Ancestors [ 离线LCA tarjan ]的更多相关文章
- POJ 1470 Closest Common Ancestors【LCA Tarjan】
题目链接: http://poj.org/problem?id=1470 题意: 给定若干有向边,构成有根数,给定若干查询,求每个查询的结点的LCA出现次数. 分析: 还是很裸的tarjan的LCA. ...
- POJ 1470 Closest Common Ancestors (LCA,离线Tarjan算法)
Closest Common Ancestors Time Limit: 2000MS Memory Limit: 10000K Total Submissions: 13372 Accept ...
- POJ 1470 Closest Common Ancestors (LCA, dfs+ST在线算法)
Closest Common Ancestors Time Limit: 2000MS Memory Limit: 10000K Total Submissions: 13370 Accept ...
- POJ 1470 Closest Common Ancestors 【LCA】
任意门:http://poj.org/problem?id=1470 Closest Common Ancestors Time Limit: 2000MS Memory Limit: 10000 ...
- poj----(1470)Closest Common Ancestors(LCA)
Closest Common Ancestors Time Limit: 2000MS Memory Limit: 10000K Total Submissions: 15446 Accept ...
- ZOJ 1141:Closest Common Ancestors(LCA)
Closest Common Ancestors Time Limit: 10 Seconds Memory Limit: 32768 KB Write a program that tak ...
- POJ1470 Closest Common Ancestors
LCA问题,用了离线的tarjan算法.输入输出参考了博客http://www.cnblogs.com/rainydays/archive/2011/06/20/2085503.htmltarjan算 ...
- POJ 1470 Closest Common Ancestors (模板题)(Tarjan离线)【LCA】
<题目链接> 题目大意:给你一棵树,然后进行q次询问,然后要你统计这q次询问中指定的两个节点最近公共祖先出现的次数. 解题分析:LCA模板题,下面用的是离线Tarjan来解决.并且为了代码 ...
- POJ1470 Closest Common Ancestors 【Tarjan的LCA】
非常裸的模版题,只是Tarjan要好好多拿出来玩味几次 非常有点巧妙呢,tarjan,大概就是当前结点和它儿子结点的羁绊 WA了俩小时,,,原因是,这个题是多数据的(还没告诉你T,用scanf!=EO ...
随机推荐
- VS2015调用低版本lib库出现“无法解析的外部符号 __snprintf ”问题的解决
VS2015在调用低版本lib库出现有时会出现“无法解析的外部符号 __snprintf ”的问题,解决方法是加入lib库“legacy_stdio_definitions.lib”到工程.
- HTML的历史与历史遗留问题
1. <style type="text/css"> 从前,HTML的设计者认为以后应该还会有其他样式,不过如今我们已经醒悟,事实表明,完全可以只使用<style ...
- Windows 2016 安装Sharepoint 2016 预装组件失败
Windows 2016 安装Sharepoint 2016 预装组件失败 日志如下: -- :: - Request for install time of Web 服务器(IIS)角色 -- :: ...
- Android iconfont字体图标的使用
1.首先,进入阿里的矢量图标库,在这个图标库里面可以找到很多图片资源,当然了需要登录才能下载或者使用,用GitHub账号或者新浪微博账号登录都可以 2.登录以后,可以搜索自己需要的资源,然后直接下载使 ...
- 【java基础】Java锁机制
在读很多并发文章中,会提及各种各样锁如公平锁,乐观锁等等,这篇文章介绍各种锁的分类.介绍的内容如下: 公平锁/非公平锁 可重入锁 独享锁/共享锁(广义) 互斥锁/读写锁(独享锁/共享锁的实现) 乐观锁 ...
- EMAC IP 核
在有线连接的世界里,以太网(Ethernet)无所不在.以太网具有各种速度模式.接口方式.以及灵活的配置方式.现在的以太网卡都是10/100/1000Mbps自适应网卡.以太网的物理层(PHY)通常使 ...
- HYSBZ 1503 郁闷的出纳员 (Splay树)
题意: 作为一名出纳员,我的任务之一便是统计每位员工的工资.但是我们的老板反复无常,经常调整员工的工资.如果他心情好,就可能把每位员工的工资加上一个相同的量.反之,如果心情不好,就可能把他们的工资扣除 ...
- TCP socket如何判断连接断开
http://blog.csdn.net/zzhongcy/article/details/21992123 SO_KEEPALIVE是系统底层的机制,用于系统维护每一个tcp连接的. 心跳线程属于应 ...
- 并发3-Volatile
Volatile关键字实现原理 1.认识volatile关键字 程序举例 用一个线程读数据,一个线程改数据 存在数据的不一致性 2.机器硬件CPU与JMM (1)CPU Cache模 (2)CPU缓存 ...
- strong&weak
copy:建立一个索引计数为1的对象,然后释放旧对象 对NSString对NSString 它指出,在赋值时使用传入值的一份拷贝.拷贝工作由copy方法执行,此属性只对那些实行了NSCopying协议 ...