POJ 1681 Painter's Problem 【高斯消元 二进制枚举】
任意门:http://poj.org/problem?id=1681
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 7667 | Accepted: 3624 |
Description
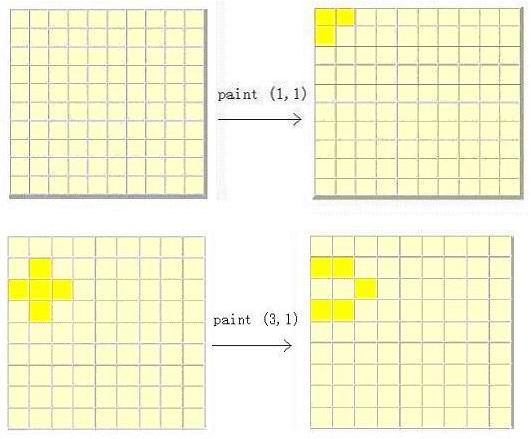
Input
Output
Sample Input
2
3
yyy
yyy
yyy
5
wwwww
wwwww
wwwww
wwwww
wwwww
Sample Output
0
15
Source
题意概括:
一个二维矩阵, 输入每个格子的初始颜色,可以进行的操作是 粉刷一个格子则相邻的上下左右四个各自颜色都会取反(只有两种颜色);
问最后把全部各自涂成黄色的最小操作数;
解题思路:
根据题意,每个各自都是一个变元,根据各自之间的相邻关系构造增广矩阵;
高斯消元求出自由元个数 sum;
二进制枚举方案,找出最小的操作数;
AC code:
#include <cstdio>
#include <iostream>
#include <algorithm>
#include <cstring>
#define INF 0x3f3f3f3f
#define LL long long
using namespace std;
const int MAXN = ; int equ, var;
int a[MAXN][MAXN];
char str[MAXN][MAXN];
int x[MAXN];
int free_x[MAXN];
int free_num;
int N; int Gauss()
{
int maxRow, col, k;
free_num = ;
for(k = , col = ; k < equ && col < var; k++, col++){
maxRow = k;
for(int i = k+; i <equ; i++){
if(abs(a[i][col]) > abs(a[maxRow][col]))
maxRow = i;
}
if(a[maxRow][col] == ){ //最大的都为0说明该列下面全是 0
k--;
free_x[free_num++] = col; //说明col是自由元
continue;
}
if(maxRow != k){ //交换行
for(int j = col; j < var+; j++)
swap(a[k][j], a[maxRow][j]);
}
for(int i = k+; i < equ; i++){ //消元
if(a[i][col] != ){
for(int j = col; j < var+; j++){
a[i][j] ^= a[k][j];
}
}
}
}
for(int i = k; i< equ; i++){
if(a[i][col] != ) return -; //无解
}
if(k < var) return var-k; //返回自由元个数 for(int i = var-; i >= ; i--){ //唯一解,回代
x[i] = a[i][var];
for(int j = i+; j < var; j++){
x[i] ^= (a[i][j] && x[j]);
}
}
return ;
} void init()
{
memset(a, , sizeof(a));
memset(x, , sizeof(x));
equ = N*N;
var = N*N;
for(int i = ; i < N; i++){ //构造增广矩阵
for(int j = -; j < N; j++){
int t = i*N+j;
a[t][t] = ;
if(i > ) a[(i-)*N+j][t] = ;
if(i < N-) a[(i+)*N+j][t] = ;
if(j > ) a[i*N+j-][t] = ;
if(j < N-) a[i*N+j+][t] = ;
}
}
} void solve()
{
int t = Gauss();
if(t == -){ //无解
printf("inf\n");
return;
}
else if(t == ){ //唯一解
int ans = ;
for(int i = ; i < N*N; i++){
ans += x[i];
}
printf("%d\n", ans);
return;
}
else{ //多解,需要枚举自由元
int ans = INF;
int tot = <<t;
int cnt = ;
for(int i = ; i < tot; i++){
cnt = ;
for(int j = ; j < t; j++){
if(i&(<<j)){
x[free_x[j]] = ;
cnt++;
}
else x[free_x[j]] = ;
} for(int j = var-t-; j >= ; j--){
int index;
for(index = j; index < var; index++){
if(a[j][index]) break;
}
x[index] = a[j][var]; for(int s = index+; s < var; s++)
if(a[j][s]) x[index] ^= x[s]; cnt+=x[index];
}
ans = min(ans, cnt);
}
printf("%d\n", ans);
}
} int main()
{
int T_case;
int tpx, tpy;
scanf("%d", &T_case);
while(T_case--){
scanf("%d", &N);
init();
for(int i = ; i < N; i++){
scanf("%s", str[i]);
for(int j = ; j < N; j++){
tpx = i*N+j, tpy = N*N;
if(str[i][j] == 'y') a[tpx][tpy] = ;
else a[tpx][tpy] = ;
}
}
solve();
}
return ;
}
POJ 1681 Painter's Problem 【高斯消元 二进制枚举】的更多相关文章
- POJ 1681 Painter's Problem (高斯消元)
题目链接 题意:有一面墙每个格子有黄白两种颜色,刷墙每次刷一格会将上下左右中五个格子变色,求最少的刷方法使得所有的格子都变成yellow. 题解:通过打表我们可以得知4*4的一共有4个自由变元,那么我 ...
- POJ 1681 Painter's Problem [高斯消元XOR]
同上题 需要判断无解 需要求最小按几次,正确做法是枚举自由元的所有取值来遍历变量的所有取值取合法的最小值,然而听说数据太弱自由元全0就可以就水过去吧.... #include <iostream ...
- POJ 1222【异或高斯消元|二进制状态枚举】
题目链接:[http://poj.org/problem?id=1222] 题意:Light Out,给出一个5 * 6的0,1矩阵,0表示灯熄灭,反之为灯亮.输出一种方案,使得所有的等都被熄灭. 题 ...
- POJ 1681 Painter's Problem(高斯消元+枚举自由变元)
http://poj.org/problem?id=1681 题意:有一块只有黄白颜色的n*n的板子,每次刷一块格子时,上下左右都会改变颜色,求最少刷几次可以使得全部变成黄色. 思路: 这道题目也就是 ...
- poj 1681 Painter's Problem(高斯消元)
id=1681">http://poj.org/problem? id=1681 求最少经过的步数使得输入的矩阵全变为y. 思路:高斯消元求出自由变元.然后枚举自由变元,求出最优值. ...
- poj 1681 Painter's Problem
Painter's Problem 题意:给一个n*n(1 <= n <= 15)具有初始颜色(颜色只有yellow&white两种,即01矩阵)的square染色,每次对一个方格 ...
- POJ 2947 Widget Factory(高斯消元)
Description The widget factory produces several different kinds of widgets. Each widget is carefully ...
- POJ 1830 开关问题(高斯消元)题解
思路:乍一看好像和线性代数没什么关系.我们用一个数组B表示第i个位置的灯变了没有,然后假设我用u[i] = 1表示动开关i,mp[i][j] = 1表示动了i之后j也会跟着动,那么第i个开关的最终状态 ...
- POJ 1830 开关问题(高斯消元求解的情况)
开关问题 Time Limit: 1000MS Memory Limit: 30000K Total Submissions: 8714 Accepted: 3424 Description ...
随机推荐
- 企业DevOps构建 (一)
一,环境: tomcat 7.0.92 jenkins 1.658 maven mysql 5.5.23 mongodb 2.6.11 redis 4.0.12 01, 安装jenkins wge ...
- shell的常用脚本一
批量创建用户名脚本: ######################################################################### # File Name: cr ...
- java多态的具体表现实例和理解
Java的多态性 面向对象编程有三个特征,即封装.继承和多态. 封装隐藏了类的内部实现机制,从而可以在不影响使用者的前提下改变类的内部结构,同时保护了数据. 继承是为了重用父类代码,同时为实现多态性作 ...
- Beam概念学习系列之PTransform数据处理
不多说,直接上干货! PTransform数据处理 PTransform对PCollection进行并行处理,每次处理1条,例如Filter过滤.Groupby分组.Combine统计.Join关联等 ...
- hdu 5242——Game——————【树链剖分思想】
Game Time Limit:1500MS Memory Limit:32768KB 64bit IO Format:%I64d & %I64u Submit Status ...
- 深入理解理解 JavaScript 的 async/await
原文地址:https://segmentfault.com/a/1190000007535316,首先感谢原文作者对该知识的总结与分享.本文是在自己理解的基础上略作修改所写,主要为了加深对该知识点的理 ...
- javaweb九大个内置对象,四大域
9个内置对象如下: 1.session对象:会话对象 当客户端第一次访问服务器的页面时,web服务器会自动为该客户端创建一个session对象并分配一个唯一的id号 常常用它来在多个页面间共享数据,如 ...
- 针对在webview模式中,小米魅族手机不支持html5原生video的control的解决办法![原创]
其实,解决办法就是,重新写个control控制功能,.同样用流行的video.js可以实现 第一步就是增加个播放的图片..要不然没有按钮多难看! <div class="videoDi ...
- Html-知识总结
1.Html概述 1.1什么是Html Html:超文本标记语言(hyperText Markup Language) “超文本”加上指页面内可以包含图片,链接等非文字内容. “标记”就是使用标签的方 ...
- Sass学习笔记(三)
一.Sass的控制命令 二.Sass的函数功能 sass中除了可以定义变量,还自备了一系列函数功能,主要包括:字符串函数.数字函数.列表函数.颜色函数.Instrospection函数.三元函数等.当 ...