Brute-force Algorithm(hdu3221)
Brute-force Algorithm
Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 2740 Accepted Submission(s): 728
Brute is not good at algorithm design. Once he was asked to solve a
path finding problem. He worked on it for several days and finally came
up with the following algorithm:
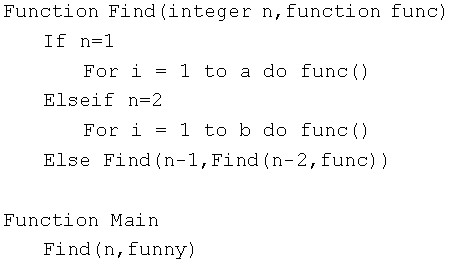
Any
fool but Brute knows that the function “funny” will be called too many
times. Brute wants to investigate the number of times the function will
be called, but he is too lazy to do it.
Now your task is to
calculate how many times the function “funny” will be called, for the
given a, b and n. Because the answer may be too large, you should output
the answer module by P.
For each test cases, there are four integers a, b, P and n in a single line.
You can assume that 1≤n≤1000000000, 1≤P≤1000000, 0≤a, b<1000000.
1 #include<stdio.h>
2 #include<algorithm>
3 #include<iostream>
4 #include<string.h>
5 #include<queue>
6 #include<set>
7 #include<math.h>
8 using namespace std;
9 typedef long long LL;
10 typedef struct node
11 {
12 LL m[4][4];
13 node()
14 {
15 memset(m,0,sizeof(m));
16 }
17 } maxtr;
18 int f1[1000];
19 int f2[1000];
20 maxtr E();
21 void Init(maxtr *q);
22 maxtr quick_m(maxtr ans,LL n,LL mod);
23 LL quick(LL n,LL m,LL mod);
24 bool prime[1000005];
25 int aa[1000005];
26 int oula[1000005];
27
28 int main(void)
29 {
30 int T;
31 int __ca = 0;
32 int i,j;
33 int k1,k2;
34 f1[2] = 0;
35 f1[3] = 1;
36 f2[2] = 1;
37 f2[3] = 1;
38 for(i = 2; i < 1000; i++)
39 {
40 for(j = i; (i*j) <= 1000000; j++)
41 {
42 prime[i*j] = true;
43 }
44 }
45 int cn = 0;
46 for(i = 2; i <= 1000000; i++)
47 {
48 oula[i] =i;
49 if(!prime[i])
50 aa[cn++] = i;
51 }
52 for(i = 0; i < cn; i++)
53 {
54 for(j = 1; (j*aa[i])<=1000000; j++)
55 {
56 oula[j*aa[i]]/=aa[i];
57 oula[j*aa[i]]*=(aa[i]-1);
58 }
59 }
60 for(i = 4;; i++)
61 {
62 f1[i] = f1[i-1]+f1[i-2];
63 if(f1[i] > 1000000)
64 {
65 k1 = i;
66 break;
67 }
68 }
69 for(i = 4;; i++)
70 {
71 f2[i] = f2[i-1] + f2[i-2];
72 if(f2[i] > 1000000)
73 {
74 k2 = i;
75 break;
76 }
77 }
78 k1=max(k1,k2);
79 scanf("%d",&T);
80 while(T--)
81 {
82 __ca++;
83 LL n,p,a,b;
84 scanf("%lld %lld %lld %lld",&a,&b,&p,&n);
85 printf("Case #%d: ",__ca);
86 if(n==1)
87 {
88 printf("%lld\n",a%p);
89 }
90 else if(n == 2)
91 {
92 printf("%lld\n",b%p);
93 }
94 else if(n == 3)
95 {
96 printf("%lld\n",a*b%p);
97 }
98 else if(n<=k1)
99 {
100 int x1 = f1[n];
101 int x2 = f2[n];
102 LL ask = quick(a,x1,p);
103 LL ack = quick(b,x2,p);
104 printf("%lld\n",ask*ack%p);
105 }
106 else
107 {
108 if(p==1)printf("0\n");
109 else
110 {
111 maxtr cc ;
112 Init(&cc);
113 cc = quick_m(cc,n-3,oula[p]);
114 LL xx = cc.m[0][0] +cc.m[0][1];
115 LL yy = cc.m[1][0] + cc.m[1][1];
116 LL ask = quick(a,yy+oula[p],p)*quick(b,xx+oula[p],p)%p;
117 printf("%lld\n",ask);
118 }
119 }
120 }
121 return 0;
122 }
123 maxtr E()
124 {
125 maxtr e;
126 for(int i = 0; i < 4; i++)
127 {
128 for(int j = 0; j < 4; j++)
129 {
130 if(i == j)
131 e.m[i][j] = 1;
132 }
133 }
134 return e;
135 }
136 void Init(maxtr *q)
137 {
138 q->m[0][0] = 1;
139 q->m[0][1] = 1;
140 q->m[1][0] = 1;
141 q->m[1][1] = 0;
142 }
143 maxtr quick_m(maxtr ans,LL n,LL mod)
144 {
145 int i,j,s;
146 maxtr ak = E();
147 while(n)
148 {
149 if(n&1)
150 {
151 maxtr c;
152 for(i = 0; i < 2; i++)
153 {
154 for(j = 0; j < 2; j++)
155 {
156 for(s = 0; s < 2; s++)
157 {
158 c.m[i][j] = c.m[i][j] + ans.m[i][s]*ak.m[s][j]%mod;
159 c.m[i][j]%=mod;
160 }
161 }
162 }
163 ak = c;
164 }
165 maxtr d;
166 for(i = 0; i < 2; i++)
167 {
168 for(j = 0; j < 2; j++)
169 {
170 for(s= 0; s < 2; s++)
171 {
172 d.m[i][j] = d.m[i][j] + ans.m[i][s]*ans.m[s][j]%mod;
173 d.m[i][j]%=mod;
174 }
175 }
176 }
177 ans = d;
178 n>>=1;
179 }
180 return ak;
181 }
182 LL quick(LL n,LL m,LL mod)
183 {
184 LL ak = 1;
185 while(m)
186 {
187 if(m&1)
188 ak = ak*n%mod;
189 n = n*n%mod;
190 m>>=1;
191 }
192 return ak;
193 }
Brute-force Algorithm(hdu3221)的更多相关文章
- SRM 582 Div II Level Three: ColorTheCells, Brute Force 算法
题目来源:http://community.topcoder.com/stat?c=problem_statement&pm=12581 Burte Force 算法,求解了所有了情况,注意 ...
- Test SRM Level Three: LargestCircle, Brute Force
题目来源:http://community.topcoder.com/stat?c=problem_statement&pm=3005&rd=5858 思路: 如果直接用Brute F ...
- 常用字符串匹配算法(brute force, kmp, sunday)
1. 暴力解法 // 暴力求解 int Idx(string S, string T){ // 返回第一个匹配元素的位置,若没有匹配的子串,则返回-1 int S_size = S.length(); ...
- 小白日记46:kali渗透测试之Web渗透-SqlMap自动注入(四)-sqlmap参数详解- Enumeration,Brute force,UDF injection,File system,OS,Windows Registry,General,Miscellaneous
sqlmap自动注入 Enumeration[数据枚举] --privileges -U username[CU 当前账号] -D dvwa -T users -C user --columns [ ...
- nginx 1.3.9/1.4.0 x86 Brute Force Remote Exploit
测试方法: 本站提供程序(方法)可能带有攻击性,仅供安全研究与教学之用,风险自负! #nginx 1.3.9/1.4.0 x86 brute force remote exploit # copyri ...
- 安全性测试入门:DVWA系列研究(一):Brute Force暴力破解攻击和防御
写在篇头: 随着国内的互联网产业日臻成熟,软件质量的要求越来越高,对测试团队和测试工程师提出了种种新的挑战. 传统的行业现象是90%的测试工程师被堆积在基本的功能.系统.黑盒测试,但是随着软件测试整体 ...
- HDU 6215 Brute Force Sorting(模拟链表 思维)
Brute Force Sorting Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 65535/32768 K (Java/Othe ...
- hdu6215 Brute Force Sorting
地址:http://acm.split.hdu.edu.cn/showproblem.php?pid=6215 题目: Brute Force Sorting Time Limit: 1000/100 ...
- HDU - 6215 2017 ACM/ICPC Asia Regional Qingdao Online J - Brute Force Sorting
Brute Force Sorting Time Limit: 1 Sec Memory Limit: 128 MB 题目连接 http://acm.hdu.edu.cn/showproblem.p ...
- 「暑期训练」「Brute Force」 Restoring Painting (CFR353D2B)
题意 给定一定条件,问符合的矩阵有几种. 分析 见了鬼了,这破题谁加的brute force的标签,素质极差.因为范围是1e5,那你平方(枚举算法)的复杂度必然爆. 然后你就会思考其中奥妙无穷的数学规 ...
随机推荐
- Linux学习 - 正则表达式
一.正则表达式与通配符 正则表达式:在文件中匹配符合条件的字符串,正则是包含匹配 通配符:用来匹配符合条件的文件名,通配符是完全匹配 二.基础正则表达式 元字符 作用 a* a有0个或任意多个 . 除 ...
- zabbix之模板制作(memcache redis)
#:找一台主机安装redis和memcached(记得安装zabbix-agent) root@ubuntu:~# apt install redis root@ubuntu:~# apt insta ...
- 类型类 && .class 与 .getClass() 的区别
一. 什么是类型类 Java 中的每一个类(.java 文件)被编译成 .class 文件的时候,Java虚拟机(JVM)会为这个类生成一个类对象(我们姑且认为就是 .class 文件),这个对象包含 ...
- html标签设置contenteditable时,去除粘贴文本自带样式
在一个div标签里面加了可编辑的属性,从别的地方复制了一串文本,只想把文本内容存到接口里面,结果发现文本自带的标签和样式都会存进去. $(".session-new-name"). ...
- MyEclipse配置Spring框架(基础篇)
一.新建项目,添加spring的相关jar包等 二.创建相关类以及属性和方法 Student.java package com.yh; public class Student implements ...
- 【HarmonyOS】【DevEco Studio】NOTE05:PageAbility生命周期的呈现
NOTE05:PageAbility生命周期的呈现 基本界面设置 创建Slice与对应xml BarAbilitySlice package com.example.myapplication.sli ...
- C# 温故知新 第二篇 C# 程序的通用结构
C# 程序由一个或多个文件组成. 每个文件均包含零个或多个命名空间. 一个命名空间包含类.结构.接口.枚举.委托等类型或其他命名空间. 以下示例是包含所有这些元素的 C# 程序主干. 主要包括 1. ...
- 热部署详细步骤---·> 小热身!
IDEA 2018.1.5 4版本 热部署 网址:https://www.jb51.net/softjc/629271.html
- Mit6.830 - simpledb - 总览
总览 github 地址: https://github.com/CreatorsStack/CreatorDB 在开始 simpledb 旅途之前, 我们先从整体上来看看 SimpleDb 是一个 ...
- 如何实现 range 函数的参数?
关于 range 函数 Python内置的range函数可以接收三个参数: class range(stop): ... class range(start, stop[, step]): ... 标 ...