数据库CRUD操作以及MyBatis的配置使用
• 业务字段设计

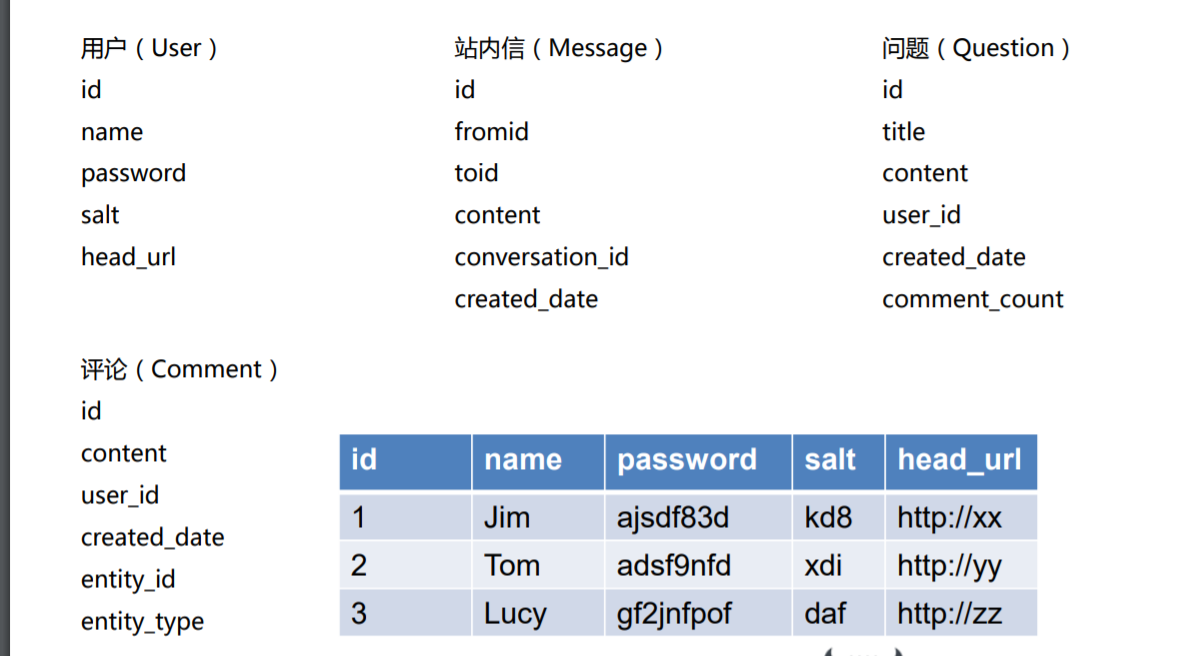
name:logub_ticket
lable:
id
user_id
ticket
expired
status

//xml配置,在相应的resources下建立DAO相应目录的的xml;
数据库CRUD操作以及MyBatis的配置使用的更多相关文章
- 【转】数据库CRUD操作
数据库CRUD操作 一.删除表 drop table 表名称 二.修改表 alter table 表名称 add 列名 数据类型 (add表示添加一列) alter table 表名 ...
- Mybatis基于代理Dao实现CRUD操作 及 Mybatis的参数深入
Mybatis基于代理Dao实现CRUD操作 使用要求: 1.持久层接口和持久层接口的映射配置必须在相同的包下 2.持久层映射配置中mapper标签的namespace属性取值必须是持久层接口的全限定 ...
- 05 Mybatis的CRUD操作和Mybatis连接池
1.CRUD的含义 CRUD是指在做计算处理时的增加(Create).读取(Retrieve)(重新得到数据).更新(Update)和删除(Delete)几个单词的首字母简写.主要被用在描述软件系统中 ...
- 10月16日下午MySQL数据库CRUD操作(增加、删除、修改、查询)
1.MySQL注释语法--,# 2.2.后缀是.sql的文件是数据库查询文件. 3.保存查询. 关闭查询时会弹出提示是否保存,保存的是这段文字,不是表格(只要是执行成功了表格已经建立了).保存以后下次 ...
- 数据库CRUD操作:C:create创建(添加)、R:read读取、U:update:修改、D:delete删除;高级查询
1.注释语法:--,#2.后缀是.sql的文件是数据库查询文件3.保存查询4.在数据库里面 列有个名字叫字段 行有个名字叫记录5.一条数据即为表的一行 CRUD操作:create 创建(添加)re ...
- 数据库CRUD操作
CRUD操作: C:create 增加数据: insert into 表名 values('N001','汉族') 普通 insert into 表名 values('','','') 如果有自增长列 ...
- django notes 六:数据库 CRUD 操作
CRUD 也没什么可说的,django 提供了完善的 orm api, 直接用就行了. 我只贴几个列子,一看就明白了,自己再用用就熟了. # create b = Blog(name='Beatle ...
- 使用node_redis进行redis数据库crud操作
正在学习使用pomelo开发游戏服务器,碰到node.js操作redis,记录一下 假设应用场景是操作一个用户表的数据 引入node_redis库,创建客户端 var redis = require( ...
- 【Mybatis】MyBatis对表执行CRUD操作(三)
本例在[Mybatis]MyBatis配置文件的使用(二)基础上继续学习对表执行CRUD操作 使用MyBatis对表执行CRUD操作 1.定义sql映射xml文件(EmployeeMapper.xml ...
随机推荐
- [Swift]LeetCode665. 非递减数列 | Non-decreasing Array
Given an array with n integers, your task is to check if it could become non-decreasing by modifying ...
- [Swift]LeetCode871. 最低加油次数 | Minimum Number of Refueling Stops
A car travels from a starting position to a destination which is target miles east of the starting p ...
- Nginx学习系列四默认负载均衡轮询及Ip_hash等常用指令介绍
一.简介 Upstream模块是Nginx中一个核心模块,当客户端访问Nginx服务器的时候,Nginx会从服务器列表中选取压力小的服务器,然后分配给客户端进行访问.这个过程,Nginx通过轮询算法轮 ...
- C/C++读写二进制文件
C++读写二进制文件 最近在给android层提供支持,因此代码都是用标准库库函数写出来的,好多windows和第三方的库不能或者很难使用,下面有我在读写二进制文件时候的一些心得,也算是一种总结吧 1 ...
- 关于ML.NET v0.6的发布说明
ML.NET 0.6版本提供了几项令人兴奋的新增功能: 用于构建和使用机器学习模型的新API 我们主要关注的是发布用于构建和使用模型的新ML.NET API的第一次迭代.这些新的,更灵活的API支持新 ...
- Unity资源 ----加载最好需要做哪些事
先上图解 一.基本关键词 1)AssetBundle:一种保存“一个或多个资源的转变为某种利于传输等的特殊格式(二进制之类)”的文件.(我这边是使用Unity制作手游的角度来说明) 简称AB. 2)对 ...
- Python爬虫入门教程 33-100 《海王》评论数据抓取 scrapy
1. 海王评论数据爬取前分析 海王上映了,然后口碑炸了,对咱来说,多了一个可爬可分析的电影,美哉~ 摘录一个评论 零点场刚看完,温导的电影一直很不错,无论是速7,电锯惊魂还是招魂都很棒.打斗和音效方面 ...
- 【Python3爬虫】12306爬虫
此次要实现的目标是登录12306网站和查看火车票信息. 具体步骤 一.登录 登录功能是通过使用selenium实现的,用到了超级鹰来识别验证码.没有超级鹰账号的先注册一个账号,充值一点题分,然后把下载 ...
- List-ArrayList集合基础增强底层源码分析
List集合基础增强底层源码分析 作者:Stanley 罗昊 [转载请注明出处和署名,谢谢!] 集合分为三个系列,分别为:List.set.map List系列 特点:元素有序可重复 有序指的是元素的 ...
- 【深度学习系列】用PaddlePaddle进行车牌识别(二)
上节我们讲了第一部分,如何用生成简易的车牌,这节课中我们会用PaddlePaddle来识别生成的车牌. 数据读取 在上一节生成车牌时,我们可以分别生成训练数据和测试数据,方法如下(完整代码在这里): ...