Risk(最短路)
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 2915 | Accepted: 1352 |
Description
During the course of play, a player often engages in a sequence of conquests with the goal of transferring a large mass of armies from some starting country to a destination country. Typically, one chooses the intervening countries so as to minimize the total number of countries that need to be conquered. Given a description of the gameboard with 20 countries each with between 1 and 19 connections to other countries, your task is to write a function that takes a starting country and a destination country and computes the minimum number of countries that must be conquered to reach the destination. You do not need to output the sequence of countries, just the number of countries to be conquered including the destination. For example, if starting and destination countries are neighbors, then your program should return one.
The following connection diagram illustrates the first sample input.
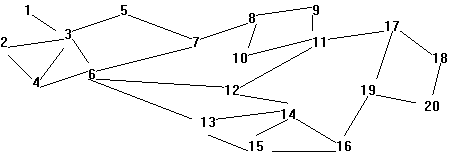
Input
There can be multiple test sets in the input file; your program should continue reading and processing until reaching the end of file. There will be at least one path between any two given countries in every country configuration.
Output
Sample Input
1 3
2 3 4
3 4 5 6
1 6
1 7
2 12 13
1 8
2 9 10
1 11
1 11
2 12 17
1 14
2 14 15
2 15 16
1 16
1 19
2 18 19
1 20
1 20
5
1 20
2 9
19 5
18 19
16 20
Sample Output
Test Set #1
1 to 20: 7
2 to 9: 5
19 to 5: 6
18 to 19: 2
16 to 20: 2
题解:很水的一道题,就是个最简单的最短路;
代码:
#include<iostream>
#include<cstdio>
#include<cstring>
#include<cmath>
#include<algorithm>
using namespace std;
const int INF=0x3f3f3f3f;
#define SI(x) scanf("%d",&x)
#define PI(x) printf("%d",x)
#define P_ printf(" ")
#define mem(x,y) memset(x,y,sizeof(x))
const int MAXN=;
int mp[MAXN][MAXN],d[MAXN],vis[MAXN];
void dijkscra(int u){
mem(vis,);
mem(d,INF);
d[u]=;
while(true){
int k=-;
for(int i=;i<=;i++){
if(!vis[i])if(k==-||d[i]<d[k])k=i;
}
if(k==-)break;
vis[k]=;
for(int i=;i<=;i++)
d[i]=min(d[i],d[k]+mp[k][i]);
}
}
int main(){
int kase=;
while(){
mem(mp,INF);
int x,b,a;
for(int i=;i<=;i++){
if(!~scanf("%d",&x))exit();
while(x--){
scanf("%d",&b);
mp[i][b]=mp[b][i]=;
}
}
if(!~scanf("%d",&x))exit();
printf("Test Set #%d\n",++kase);
while(x--){
scanf("%d%d",&a,&b);
dijkscra(a);
printf("%d to %d: %d\n",a,b,d[b]);
}
puts("");
}
return ;
}
Risk(最短路)的更多相关文章
- UVa567_Risk(最短路)(小白书图论专题)
解题报告 option=com_onlinejudge&Itemid=8&category=7&page=show_problem&problem=508"& ...
- 任意两点之间的最短路(floyed)
F.Moving On Firdaws and Fatinah are living in a country with nn cities, numbered from 11 to nn. Each ...
- bzoj1001--最大流转最短路
http://www.lydsy.com/JudgeOnline/problem.php?id=1001 思路:这应该算是经典的最大流求最小割吧.不过题目中n,m<=1000,用最大流会TLE, ...
- 【USACO 3.2】Sweet Butter(最短路)
题意 一个联通图里给定若干个点,求他们到某点距离之和的最小值. 题解 枚举到的某点,然后优先队列优化的dijkstra求最短路,把给定的点到其的最短路加起来,更新最小值.复杂度是\(O(NElogE) ...
- Sicily 1031: Campus (最短路)
这是一道典型的最短路问题,直接用Dijkstra算法便可求解,主要是需要考虑输入的点是不是在已给出的地图中,具体看代码 #include<bits/stdc++.h> #define MA ...
- 最短路(Floyd)
关于最短的先记下了 Floyd算法: 1.比较精简准确的关于Floyd思想的表达:从任意节点A到任意节点B的最短路径不外乎2种可能,1是直接从A到B,2是从A经过若干个节点X到B.所以,我们假设maz ...
- bzoj1266最短路+最小割
本来写了spfa wa了 看到网上有人写Floyd过了 表示不开心 ̄へ ̄ 改成Floyd试试... 还是wa ヾ(。`Д´。)原来是建图错了(样例怎么过的) 结果T了 于是把Floyd改回spfa 还 ...
- HDU2433 BFS最短路
Travel Time Limit: 10000/2000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)Total Sub ...
- 最短路(代码来源于kuangbin和百度)
最短路 最短路有多种算法,常见的有一下几种:Dijstra.Floyd.Bellman-Ford,其中Dijstra和Bellman-Ford还有优化:Dijstra可以用优先队列(或者堆)优化,Be ...
随机推荐
- Oracle EBS-SQL (INV-6):检查监督帐户别名处理.sql
select MSI.SEGMENT1 项目编码, MSI.DESCRIPTION ...
- C语言的本质(18)——函数的可变参数
一般而言,在设计函数时会遇到许多数学和逻辑操作,是需要一些可变功能.例如,计算数字串的总和.字符串的联接或其它操作过程. 实现一个函数,要求在函数中计算传入的所有参数之和,并输出到屏幕上.这个函数实现 ...
- [Leetcode][Python]48: Rotate Image
# -*- coding: utf8 -*-'''__author__ = 'dabay.wang@gmail.com' 48: Rotate Imagehttps://leetcode.com/pr ...
- linux grep练习
1.显示/proc/meminfo文件中以不区分大小的s开头的行: 2.显示/etc/passwd中以nologin结尾的行; 3.显示/etc/inittab中以#开头,且后面跟一个或多个空白字符, ...
- DB操作用法总结。
用到了慢慢总结.用到了随时更新. 其实可以看手册了.但是看了完了手册之后,还是记不住. 1. mysql select * from table where id in(1,2,3,3,4) 怎么能显 ...
- word2vec 入门(三)模型介绍
两种模型,两种方法 模型:CBOW和Skip-Gram 方法:Hierarchical Softmax和Negative Sampling CBOW模型Hierarchical Softmax方法 C ...
- SpringNote01.基于SpringMVC-Hibernate的Blog系统
最近,在学习Spring,做这样一个简单的blog系统,主要是让自己动手练习使用Spring,熟练的使用才干进一步的深入学习.该项目使用Maven构建,使用git进行代码管理,通过这样一个小项目,熟悉 ...
- android典型监听事件实
public class MainActivity extends Activity { int counter; Button add, sub; TextView display; @Overri ...
- vs2010编译live555源码
最近加入了公司的C++视频小组,利用中秋这个假期将研究了一些live555的源码,现在先将如何编译使用vs2010编译live555,整理出来,对以后分析代码有很大帮助. 1.下载live555源码, ...
- XSD (xml Schema Definition)
.xsd文件是定义DataSet的XML文件,利用XML文件的结构优势容易可视化地设计DataSet,设计完它会生成相应的.cs文件,里面的内容就是对应的类型化的DataSet.你的代码里的DataA ...