iOS:UITableViewCell自定义单元格
UITableViewCell:自定义的单元格,可以在xib中创建单元格,也可以在storyBorad中创建单元格。有四种创建方式
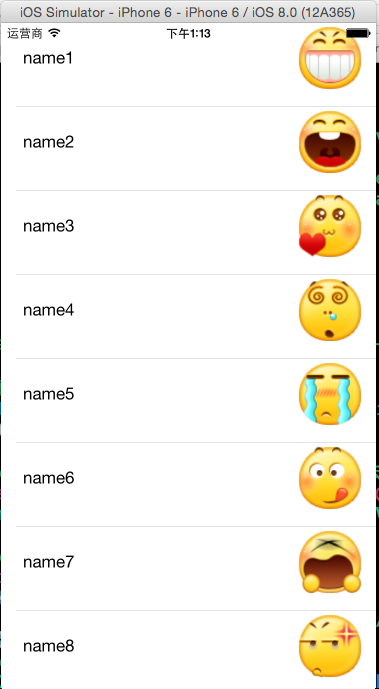
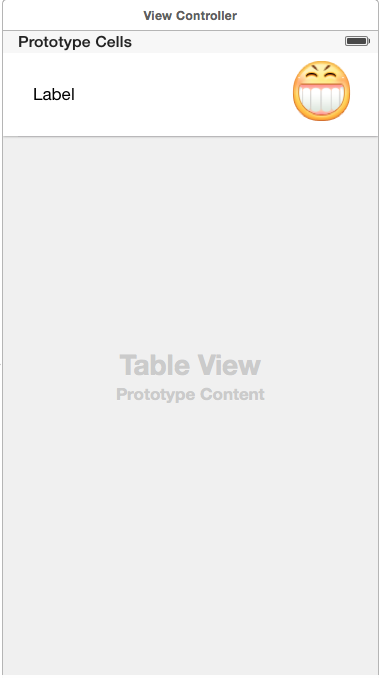

#import <Foundation/Foundation.h> @interface Contact : NSObject
@property (copy,nonatomic)NSString *name;
@property (copy,nonatomic)NSString *faceName;
-(instancetype)initWithName:(NSString*)name andFaceName:(NSString*) faceName;
@end
#import "Contact.h" @implementation Contact
-(instancetype)initWithName:(NSString*)name andFaceName:(NSString*) faceName
{
self = [super init];
if(self)
{
_name = [name copy];
_faceName = [faceName copy];
}
return self;
}
@end
在视图控制器中完成代码:(需要用tag获取单元格的属性控件)
#import "ViewController.h"
#import "Contact.h"
@interface ViewController ()<UITableViewDataSource>
@property (weak, nonatomic) IBOutlet UITableView *tableView;
@property (strong,nonatomic)NSMutableArray *contacts;
@end @implementation ViewController - (void)viewDidLoad {
[super viewDidLoad];
//初始化数据
self.contacts = [NSMutableArray arrayWithCapacity:];
for(int i=; i<; i++)
{
Contact *conatct = [[Contact alloc]initWithName:[NSString stringWithFormat:@"name%d",i+] andFaceName:[NSString stringWithFormat:@"%d.png",i]];
[self.contacts addObject:conatct];
} //设置tableView的数据源
self.tableView.dataSource = self;
} #pragma mark -tableView的数据源方法
//每一组多少行
-(NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return self.contacts.count;
}
//设置每一个单元格的内容
-(UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
//1.根据reuseIdentifier,先到对象池中去找重用的单元格对象
static NSString *reuseIdentifier = @"myCell";
UITableViewCell *cell = [self.tableView dequeueReusableCellWithIdentifier:reuseIdentifier];
//2.设置单元格对象的内容
Contact *contact = [self.contacts objectAtIndex:indexPath.row];
UILabel *label = (UILabel*)[cell viewWithTag:];
label.text = contact.name;
UIImageView *imageView = (UIImageView*)[cell viewWithTag:];
[imageView setImage:[UIImage imageNamed:contact.faceName]];
return cell;
} @end
方法二:直接在storyBoard中创建单元格并关联自定义的类并直接加载,自定义的单元格位置一个UITableView的上面
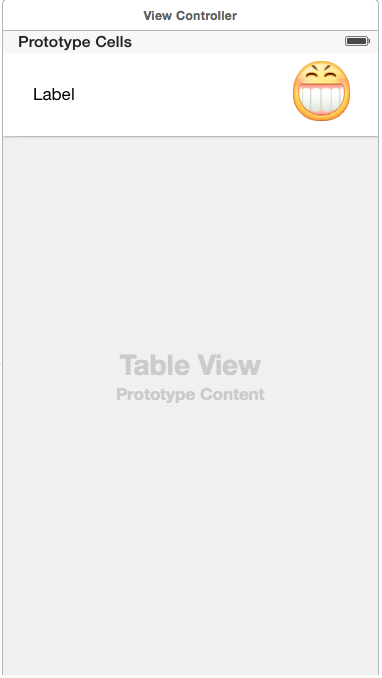
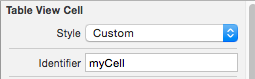
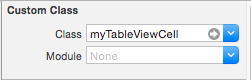
#import <Foundation/Foundation.h> @interface Contact : NSObject
@property (copy,nonatomic)NSString *name;
@property (copy,nonatomic)NSString *faceName;
-(instancetype)initWithName:(NSString*)name andFaceName:(NSString*) faceName;
@end #import "Contact.h" @implementation Contact
-(instancetype)initWithName:(NSString*)name andFaceName:(NSString*) faceName
{
self = [super init];
if(self)
{
_name = [name copy];
_faceName = [faceName copy];
}
return self;
}
@end
与单元格关联的自定义的类,关联单元格的属性控件(不需要再用tag获取了,直接用self.获取)
还是在视图控制器中完成加载:
#import "ViewController.h"
#import "Contact.h"
#import "myTableViewCell.h"
@interface ViewController ()<UITableViewDataSource>
@property (weak, nonatomic) IBOutlet UITableView *tableView;
@property (strong,nonatomic)NSMutableArray *contacts;
@end @implementation ViewController - (void)viewDidLoad {
[super viewDidLoad];
//初始化数据
self.contacts = [NSMutableArray arrayWithCapacity:];
for(int i=; i<; i++)
{
Contact *conatct = [[Contact alloc]initWithName:[NSString stringWithFormat:@"name%d",i+] andFaceName:[NSString stringWithFormat:@"%d.png",i]];
[self.contacts addObject:conatct];
} //设置tableView的数据源
self.tableView.dataSource = self;
} #pragma mark -tableView的数据源方法
//每一组多少行
-(NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return self.contacts.count;
}
//设置每一个单元格的内容
-(UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
//1.根据reuseIdentifier,先到对象池中去找重用的单元格对象
static NSString *reuseIdentifier = @"myCell";
myTableViewCell *cell = [self.tableView dequeueReusableCellWithIdentifier:reuseIdentifier];
//2.设置单元格对象的内容
Contact *contact = [self.contacts objectAtIndex:indexPath.row];
cell.label.text = contact.name;
[cell.imgView setImage:[UIImage imageNamed:contact.faceName]];
return cell;
} @end
方法三:在xib文件中创建单元格,然后再视图控制器中直接加载使用
首先在storyBoard中添加一个UITableView
然后在已经创建好的MyCell.xib中创建自定义的单元格为:
设置该单元格的重用标识符identifier:
创建一个联系人初始化的类:
#import <Foundation/Foundation.h> @interface Contact : NSObject
@property (copy,nonatomic)NSString *name;
@property (copy,nonatomic)NSString *faceName;
-(instancetype)initWithName:(NSString*)name andFaceName:(NSString*) faceName;
@end #import "Contact.h" @implementation Contact
-(instancetype)initWithName:(NSString*)name andFaceName:(NSString*) faceName
{
self = [super init];
if(self)
{
_name = [name copy];
_faceName = [faceName copy];
}
return self;
}
@end
还是在视图控制器中完成加载:
#import "ViewController.h"
#import "Contact.h"
#import "myTableViewCell.h"
@interface ViewController ()<UITableViewDataSource>
@property (weak, nonatomic) IBOutlet UITableView *tableView;
@property (strong,nonatomic)NSMutableArray *contacts;
@end @implementation ViewController - (void)viewDidLoad {
[super viewDidLoad];
//初始化数据
self.contacts = [NSMutableArray arrayWithCapacity:];
for(int i=; i<; i++)
{
Contact *conatct = [[Contact alloc]initWithName:[NSString stringWithFormat:@"name%d",i+] andFaceName:[NSString stringWithFormat:@"%d.png",i]];
[self.contacts addObject:conatct];
} //设置tableView的数据源
self.tableView.dataSource = self;
} #pragma mark -tableView的数据源方法
//每一组多少行
-(NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return self.contacts.count;
} //直接从xib文件中加载 //设置每一个单元格的内容
-(UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
//1.根据reuseIdentifier,先到对象池中去找重用的单元格对象
static NSString *reuseIdentifier = @"myCell";
UITableViewCell *cell = [self.tableView dequeueReusableCellWithIdentifier:reuseIdentifier];
//2.如果没找到,就自己创建cell
if(!cell)
{
//从xib文件中加载视图
NSArray *views = [[NSBundle mainBundle]loadNibNamed:@"MyCell" owner:nil options:nil];
cell = (UITableViewCell*)[views lastObject];
}
//3.设置单元格对象的内容
Contact *contact = [self.contacts objectAtIndex:indexPath.row];
UILabel *label = (UILabel*)[cell viewWithTag:];
label.text = contact.name;
UIImageView *imgView = (UIImageView*)[cell viewWithTag:];
[imgView setImage:[UIImage imageNamed:contact.faceName]]; return cell;
}
方法四:在xib文件中创建单元格,并创建与之关联的的类,然后将加载过程封装到它的类中帮助初始化完成,同时该类提供类方法,最后再视图控制器中通过这个类方法获取单元格。
首先在storyBoard中添加一个UITableView
然后在已经创建好的MyCell.xib中创建自定义的单元格为:
给单元格设置重用标识符identifier
将单元格与自定义的类关联
创建一个联系人初始化的类:
#import <Foundation/Foundation.h> @interface Contact : NSObject
@property (copy,nonatomic)NSString *name;
@property (copy,nonatomic)NSString *faceName;
-(instancetype)initWithName:(NSString*)name andFaceName:(NSString*) faceName;
@end #import "Contact.h" @implementation Contact
-(instancetype)initWithName:(NSString*)name andFaceName:(NSString*) faceName
{
self = [super init];
if(self)
{
_name = [name copy];
_faceName = [faceName copy];
}
return self;
}
@end
创建一个与单元格关联的类:(将加载单元格的过程和属性封装起来)
在视图控制器中通过上面的类方法获取单元格
#import "ViewController.h"
#import "Contact.h"
#import "myTableViewCell.h"
@interface ViewController ()<UITableViewDataSource>
@property (weak, nonatomic) IBOutlet UITableView *tableView;
@property (strong,nonatomic)NSMutableArray *contacts;
@end @implementation ViewController - (void)viewDidLoad {
[super viewDidLoad];
//初始化数据
self.contacts = [NSMutableArray arrayWithCapacity:];
for(int i=; i<; i++)
{
Contact *conatct = [[Contact alloc]initWithName:[NSString stringWithFormat:@"name%d",i+] andFaceName:[NSString stringWithFormat:@"%d.png",i]];
[self.contacts addObject:conatct];
} //设置tableView的数据源
self.tableView.dataSource = self;
} #pragma mark -tableView的数据源方法
//每一组多少行
-(NSInteger)tableView:(UITableView *)tableView numberOfRowsInSection:(NSInteger)section
{
return self.contacts.count;
}
//在与xib关联的类中加载xib文件(其实就是封装了一下而已) //设置每一个单元格的内容
-(UITableViewCell *)tableView:(UITableView *)tableView cellForRowAtIndexPath:(NSIndexPath *)indexPath
{
//1.根据reuseIdentifier,先到对象池中去找重用的单元格对象
static NSString *reuseIdentifier = @"myCell";
myTableViewCell *cell = [self.tableView dequeueReusableCellWithIdentifier:reuseIdentifier];
//2.如果没找到,就自己创建cell
if(!cell)
{
cell = [myTableViewCell cell];//调用类方法
}
//3.设置单元格对象的内容
Contact *contact = [self.contacts objectAtIndex:indexPath.row];
[cell setContact:contact];//调用实例方法 return cell;
} @end
iOS:UITableViewCell自定义单元格的更多相关文章
- Swift - 自定义单元格实现微信聊天界面
1,下面是一个放微信聊天界面的消息展示列表,实现的功能有: (1)消息可以是文本消息也可以是图片消息 (2)消息背景为气泡状图片,同时消息气泡可根据内容自适应大小 (3)每条消息旁边有头像,在左边表示 ...
- 浅谈DevExpress<五>:TreeList简单的美化——自定义单元格,加注释以及行序号
今天就以昨天的列表为例,实现以下效果:预算大于110万的单元格突出显示,加上行序号以及注释,如下图:
- jQuery MiniUI自定义单元格
监听处理"drawcell"事件 使用"drawcell"事件,可以自定义单元格内容.样式.行样式等. grid.on("drawcell" ...
- 使用VUE组件创建SpreadJS自定义单元格(一)
作为近五年都冲在热门框架排行榜首的Vue,大家一定会学到的一部分就是组件的使用.前端开发的模块化,可以让代码逻辑更加简单清晰,项目的扩展性大大加强.对于Vue而言,模块化的体现集中在组件之上,以组件为 ...
- 使用VUE组件创建SpreadJS自定义单元格(二)
在上篇中,我们介绍了如何通过设置runtimeCompiler为true,在Vue中实现了动态创建电子表格组件.想了解具体内容可看点击查看使用VUE组件创建SpreadJS自定义单元格(一). 但是在 ...
- 自己的自定义单元格(IOS)
定义自己的单位格有三种方法 - 代码 - xib - storyboard(推荐) 操作方法故事板 1.在TableView财产Prototype Cells至1.莫感觉1: 2.须要创建自己定义的单 ...
- IOS 取消表格单元格 TableViewCell 去掉高亮状态 点击Cell取消选择状态
以下是两种实现效果 1. 自定义cell 继承UITableViewCell 重写 -(void)setSelected:(BOOL)selected animated:(BOOL)animated ...
- UITableView自定义单元格
随手笔记: RootViewController代码 #import "RootViewController.h" #import "AddressContact.h&q ...
- NPOI 自定义单元格背景颜色-Excel
NPOI针对office2003使用HSSFWorkbook,对于offce2007及以上使用XSSFWorkbook:今天我以HSSFWorkbook自定义颜色为例说明,Office2007的未研究 ...
随机推荐
- 本机ip和127.0.0.1的区别
简介 连接上因特网的每一台计算机都会有一个IP地址, 在linux下可以使用命令ifconfig来查看本机的ip地址(windows为ipconfig), 比如我当前电脑的ip地址为192.168.0 ...
- mysql 函数substring_index()
函数: 1.从左开始截取字符串 left(str, length) 说明:left(被截取字段,截取长度) 例:select left(content,200) as abstract from my ...
- 远程连接mysql root账号报错:2003-can't connect to MYSQL serve(转)
远程连接mysql root账号报错:2003-can't connect to MYSQL serve 1.远程连接Linux系统,登录数据库:mysql -uroot -p(密码) 2.修改roo ...
- Python并发编程系列之多进程(multiprocessing)
1 引言 本篇博文主要对Python中并发编程中的多进程相关内容展开详细介绍,Python进程主要在multiprocessing模块中,本博文以multiprocessing种Process类为中心 ...
- 监控cpu、内存 <shell>
获取cpu.内存结果 pid=$1 #获取进程pid echo $pid interval=1 #设置采集间隔 while true do echo $(date +"%y-%m-%d %H ...
- 【POJ】1419:Graph Coloring【普通图最大点独立集】【最大团】
Graph Coloring Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 5775 Accepted: 2678 ...
- pygame系列_pygame安装
在接下来的blog中,会有一系列的文章来介绍关于pygame的内容,所以把标题设置为pygame系列 在这篇blog中,主要描述一下我们怎样来安装pygame 可能很多人像我一样,发现了pygame是 ...
- flask 中 session的源码解析
1.首先请求上下文和应用上下文中已经知道session是一个LocalProxy()对象 2.然后需要了解整个请求流程, 3.客户端的请求进来时,会调用app.wsgi_app(),于此此时,会生成一 ...
- GIT(6)----fork和clone的区别,fetch与pull的区别
参考资料: [1].Git学习笔记:fork和clone的区别,fetch与pull的区别 [2].在Github和Git上fork之简单指南
- ROS知识(8)----CMakeLists.txt文件编写的理解
ROS(Indigo)编程必须要理解CMakeList.txt的编写规则,教程地址:catkin/CMakeLists.txt,官网有相关的教程,中文的翻译版本写的很不错,教程地址:ROS中的CMak ...