[MIT Intro. to algo]Lecture 1: 课程介绍,算法优势,插入算法和归并算法分析,渐近符号
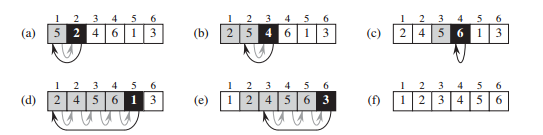
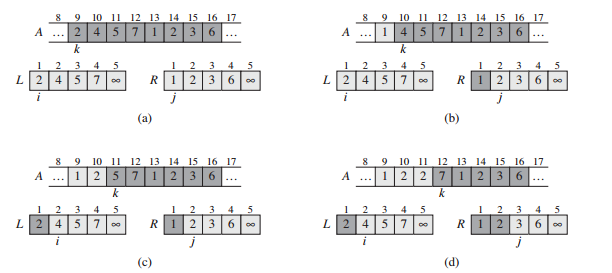
[MIT Intro. to algo]Lecture 1: 课程介绍,算法优势,插入算法和归并算法分析,渐近符号的更多相关文章
- [学习笔记] CS131 Computer Vision: Foundations and Applications:Lecture 1 课程介绍
课程大纲:http://vision.stanford.edu/teaching/cs131_fall1718/syllabus.html 课程定位: 课程交叉: what is (computer) ...
- MIT 6.828 课程介绍
MIT 6.828 课程介绍 本文是对MIT 6.828操作系统课程介绍的简单摘录,详细介绍见6.828: Learning by doing以及朱佳顺的推荐一门课:6.828.学习资源均可以在课程主 ...
- 优雅智慧女性课程班 - 公开课程 - 课程介绍 - 中国人民大学商学院EDP中心
优雅智慧女性课程班 - 公开课程 - 课程介绍 - 中国人民大学商学院EDP中心 优雅智慧女性课程班 课程总览 思想睿智成熟,外表美丽自信,气质优雅端庄,是魅力女性所应具备的特性.在当今不确定环境下, ...
- Vue+koa2开发一款全栈小程序(1.课程介绍+2.ES6入门)
1.课程介绍 1.课程概述 1.做什么? Vue+koa2开发一款全栈小程序 2.哪些功能? 个人中心.图书列表.图书详情.图书评论.个人评论列表 3.技术栈 小程序.Vue.js.koa2.koa- ...
- 爬虫--Scrapy框架课程介绍
Scrapy框架课程介绍: 框架的简介和基础使用 持久化存储 代理和cookie 日志等级和请求传参 CrawlSpider 基于redis的分布式爬虫 一scrapy框架的简介和基础使用 a) ...
- web安全之SQL注入--第一章 课程介绍
课程介绍1.什么是SQL注入?2.如何寻找SQL注入漏洞?3.如何进行sql注入攻击?4.如何预防sql注入5.课程总结
- python入门课程 第一章 课程介绍
1-1 Python入门课程介绍特点: 优雅.明确.简单适合领域: web网站和各种网络服务 系统工具和脚本 作为"胶水"语言把其他语言开发的模块包装起来方 ...
- 01.课程介绍 & 02.最小可行化产品MVP
01.课程介绍 02.最小可行化产品MVP 产品开发过程 最小化和可用之间找到一个平衡点
- JS--- part6课程介绍 & part5复习
part6 课程介绍 scroll系列:-----重点,每个属性是什么意思 封装scroll系列的相关的属性,固定导航栏案例---事件浏览器的滚动条事件--能够写出来 封装动画函数---缓动动画--- ...
随机推荐
- async/await actor promise 异步编程
Python协程:从yield/send到async/await http://blog.guoyb.com/2016/07/03/python-coroutine/ Async/Await替代Pro ...
- Docker实战(五)之端口映射与容器互联
除了网络访问外,Docker还提供了两个很方便的功能来满足服务访问的基本需求:一个是允许映射容器内应用的服务端口到本地宿主主机;另一个是互联机制实现多个容器间通过容器名来快速访问. 1.端口映射实现访 ...
- 为什么 token可以防止 csrf?
Token被用户端放在Cookie中(不设置HttpOnly),同源页面每次发请求都在请求头或者参数中加入Cookie中读取的Token来完成验证.CSRF只能通过浏览器自己带上Cookie,不能操作 ...
- Python中乘法
1.numpy乘法运算中"*"或multiply(),是数组元素逐个计算,具体代码如下: import numpy as np # 2-D array: 2 x 3 two_dim ...
- sqoop-1.4.7 搭建
sqoop搭建环境: jdk1.8 hadoop分布式集群(HDFS) HIVE(看使用情况) 下载网址:http://sqoop.apache.org/ 建议: sqoop1.4. ...
- 一条SQL语句执行得很慢原因有哪些
一条SQL语句执行得很慢,要分两种情况: 1.大多数情况是正常,偶尔很慢 数据库在处理数据忙时候,更新或新增数据都会暂时记录到redo log日志,等空闲时把数据同步到磁盘.假设数据库一直很忙,更新又 ...
- RS485接口为什么要接地
RS485接口为什么要接地 RS485接口有三根线,分别是A.B和GND线.因为RS485是差分传输的,所以很多工程师以为GND地线不重要,经常不接,甚至有些工程为了节约成本用两芯线或者用视频线来传输 ...
- #leetcode刷题之路33-搜索旋转排序数组
假设按照升序排序的数组在预先未知的某个点上进行了旋转.( 例如,数组 [0,1,2,4,5,6,7] 可能变为 [4,5,6,7,0,1,2] ).搜索一个给定的目标值,如果数组中存在这个目标值,则返 ...
- maven tomcat plugin 踩坑记
今天在自己家里的电脑上改一个项目,安装的是社区版的 IntelliJ Idea, 没有办法安装 Tomcat 插件来启动项目.尝试用 jettry runer 启动,结果报 java.lang.Inc ...
- C语言学习记录_2019.02.07
C99开始,可以用变量来定义数组的大小:例如,利用键盘输入的变量来定义数组大小: 赋值号左边的值叫做左值: 关于数组:编译器和运行环境不会检查数组下标是否越界,无论读还是写. 越界数组可能造成的问题提 ...