UVa——400Unix ls(字典序文本处理输出iomanip)
Time Limit: 3000MS | Memory Limit: Unknown | 64bit IO Format: %lld & %llu |
Description
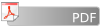
The computer company you work for is introducing a brand new computer line and is developing a new Unix-like operating system to be introduced along with the new computer. Your assignment is to write the formatter for thels function.
Your program will eventually read input from a pipe (although for now your program will read from the input file). Input to your program will consist of a list of (F) filenames that you will sort (ascending based on the ASCII character values) and format into (C) columns based on the length (L) of the longest filename. Filenames will be between 1 and 60 (inclusive) characters in length and will be formatted into left-justified columns. The rightmost column will be the width of the longest filename and all other columns will be the width of the longest filename plus 2. There will be as many columns as will fit in 60 characters. Your program should use as few rows (R) as possible with rows being filled to capacity from left to right.
Input
The input file will contain an indefinite number of lists of filenames. Each list will begin with a line containing a single integer ( ). There will then beN lines each containing one left-justified filename and the entire line's contents (between 1 and 60 characters) are considered to be part of the filename. Allowable characters are alphanumeric (a toz, A to Z, and 0 to 9) and from the following set
{ ._- }
(not including the curly braces). There will be no illegal characters in any of the filenames and no line will be completely empty.
Immediately following the last filename will be the N for the next set or the end of file. You should read and format all sets in the input file.
Output
For each set of filenames you should print a line of exactly 60 dashes (-) followed by the formatted columns of filenames. The sorted filenames 1 toR will be listed down column 1; filenames R+1 to 2R listed down column 2; etc.
Sample Input
10
tiny
2short4me
very_long_file_name
shorter
size-1
size2
size3
much_longer_name
12345678.123
mid_size_name
12
Weaser
Alfalfa
Stimey
Buckwheat
Porky
Joe
Darla
Cotton
Butch
Froggy
Mrs_Crabapple
P.D.
19
Mr._French
Jody
Buffy
Sissy
Keith
Danny
Lori
Chris
Shirley
Marsha
Jan
Cindy
Carol
Mike
Greg
Peter
Bobby
Alice
Ruben
Sample Output
------------------------------------------------------------
12345678.123 size-1
2short4me size2
mid_size_name size3
much_longer_name tiny
shorter very_long_file_name
------------------------------------------------------------
Alfalfa Cotton Joe Porky
Buckwheat Darla Mrs_Crabapple Stimey
Butch Froggy P.D. Weaser
------------------------------------------------------------
Alice Chris Jan Marsha Ruben
Bobby Cindy Jody Mike Shirley
Buffy Danny Keith Mr._French Sissy
Carol Greg Lori Peter
题意:输入N后有N段字符串,读入进行按字典序排序,然后按尽量少行数输出,每行最多60个字符,因此最大列数colum=(60-maxlen)/(maxlen+2)+1;当然maxlen最长字符串的长度,行数row=(int)(ceil( (double)t/colum) )。然后最后一列的每个字符串输出占位数为maxlen,其他为maxlen+2。题意刚开始看不懂,直接百度了。
代码:
#include<iostream>
#include<algorithm>
#include<string>
#include<cmath>
#include<cstdio>
#include<set>
#include<sstream>
#include<map>
#include<vector>
#include<iomanip>
using namespace std;
bool cmp(const string &a,const string &b)
{
return a<b;
}
int main(void)
{
int t,maxlen,colum,row;
while (cin>>t)
{
string list[1000];
maxlen=0;
for (int q=0; q<t; q++)
{
cin>>list[q];
maxlen=max(maxlen,(int)list[q].size());//计算字符串最大长度
}
sort(list,list+t,cmp);//字典序排序
colum=(60-maxlen)/(maxlen+2)+1;//计算列数
row=(int)(ceil((double)t/colum));//计算行数
cout<<"------------------------------------------------------------"<<endl;
for (int i=0; i<row; i++)//行外循环
{
for (int j=0; j<colum; j++)//列内循环
{
if(j<colum-1)
cout<<left<<setw(maxlen)<<list[j*row+i]<<" ";//不是最后一列,maxlen再多输俩空格
else
cout<<left<<setw(maxlen)<<list[j*row+i];//按maxlen输出
}
cout<<endl;
}
}
return 0;
}
UVa——400Unix ls(字典序文本处理输出iomanip)的更多相关文章
- ls按时间排序输出文件列表
文件转自:http://www.2cto.com/os/201303/197829.html ls按时间排序输出文件列表 首先,ls --help查看ls相关的与时间排序相关的参数: > ...
- 【HTML5游戏开发小技巧】RPG情景对话中,令文本逐字输出
以前用JAVAscript实现过令文本逐字输出的效果,今天我来用html5中的canvas实现一下.canvas里的内容可不像<p>那样好操作,首先,你需要懂得一些html5的API才能操 ...
- 2019.11.13课堂实验之用Linux下的shell脚本完成两文本交替输出
有两个文本如下,实际中并不知道两文本各有多少行: 文本1.txt aaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaaa ccccccccccccccccccccccccccc ...
- Linux终端和win32控制台文本颜色输出
在使用putty.secureCRT.XShell等终端仿真器连接linux系统时,ls.vim等工具的输出都含有各种颜色,这些颜色的输出大大地增强了文本的可读性. 通常我们可以使用echo命令加-e ...
- 【Windows编程】系列第三篇:文本字符输出
上一篇我们展示了如何使用Windows SDK创建基本控件,本篇来讨论如何输出文本字符. 在使用Win32编程时,我们常常要输出文本到窗口上,Windows所有的文本字符或者图形输出都是通过图形设备接 ...
- 转:MVC2表单验证失败后,直接返回View,已填写的内容就会清空,可以这样做;MVC2输出文本;MVC2输出PDF文件
ViewData.ModelState.AddModelError("FormValidator", message); foreach (string field in Requ ...
- api (三)文本字符输出 (转)
在使用Win32编程时,我们常常要输出文本到窗口上,Windows所有的文本字符或者图形输出都是通过图形设备接口(GDI)进行的,Windows的三大核心组件之一的GDI32.dll封装了所有的文本和 ...
- SAM I AM UVA - 11419 最小点集覆盖 要输出具体覆盖的行和列。
/** 题目:SAM I AM UVA - 11419 链接:https://vjudge.net/problem/UVA-11419 题意:给定n*n的矩阵,'X'表示障碍物,'.'表示空格;你有一 ...
- Log4net系列一:Log4net搭建之文本格式输出【转】
前言 项目开发中,记录项目日志是必须的,如果非要说日志的重要性(日志可看做,飞机的黑匣子,或者汽车的行车记录仪),根据等级进行记录,方便我们排查相关问题,以后项目运维中,也方便很多.基本上我们进入一家 ...
随机推荐
- SAP数据中心概述
文章目录 SAP数据中心内部的组成部分 SAP数据中心的安全性 SAP数据中心的绿色运营 SAP云平台编程环境 Jerry的前一篇文章企业数字化转型与SAP云平台介绍了SAP云平台在企业数字化转型中的 ...
- UVALive 4329 Ping pong (BIT)
枚举中间的人,只要知道在这个人前面的技能值比他小的人数和后面技能值比他小的人数就能计算方案数了,技能值大的可有小的推出. 因此可以利用树状数组,从左到右往树上插点,每个点询问sum(a[i]-1)就是 ...
- setTimeout,clearTimeout的一些好用法
if(hidden != 1){ $.ui.showMask(); var _aaa = setTimeout(function(){ $.ui.hideMask(); },5000); } //be ...
- window.addEventListener介绍说明
原型 public override function addEventListener(type:String, listener:Function, useCapture:Boolean = fa ...
- dht 分布式hash 一致性hash区别
先有一致性hash :一致性哈希,似乎最早提出是在分布式缓存里面的,让节点震荡的时候,影响最小.不过现在已经应用在分布式存储和p2p系统里面. dht 是p2p领域的概念,内有三大概念是由keyspa ...
- shell脚本,awk取中间列的方法。
解释 1.$(int(NF/2)+1) 中int(NF/2)等于3,然后加1,就得到中间的4了. 2.$(NF/2+0.5) 相当于得出的是整数.NF/2是3.5,再由3.5+0.5,所以就是4了,也 ...
- Oracle11g 数据库的导入导出
导出: 全部: exp imagesys/imagesys@orcl file=/icms/20170116.dmp full=y 用户: exp imagesys/imagesys @orcl fi ...
- 03_6_package和import语句
03_6_package和import语句 1. package和import语句 为便于管理大型软件系统中数目众多的类,解决类的命名冲突问题,Java引入包(package)机制,提供类的多重命名空 ...
- presenting view controller
Present ViewController详解 Present ViewController Modally 一.主要用途 弹出模态ViewController是IOS变成中很有用的一个技术,UIK ...
- stataic 变量
static 是静态变量的的类型说明符 静态变量属于静态存储方式,(外部变量也是静态存储方式) 静态的局部变量 静态局部变量属于静态存储方式,它具有以下特点: (1)静态局部变量在函数内定义 它的生存 ...