AtCoder Regular Contest 069 D
D - Menagerie
Time limit : 2sec / Memory limit : 256MB
Score : 500 points
Problem Statement
Snuke, who loves animals, built a zoo.
There are N animals in this zoo. They are conveniently numbered 1 through N, and arranged in a circle. The animal numbered i(2≤i≤N−1) is adjacent to the animals numbered i−1 and i+1. Also, the animal numbered 1 is adjacent to the animals numbered 2 and N, and the animal numbered N is adjacent to the animals numbered N−1 and 1.
There are two kinds of animals in this zoo: honest sheep that only speak the truth, and lying wolves that only tell lies.
Snuke cannot tell the difference between these two species, and asked each animal the following question: "Are your neighbors of the same species?" The animal numbered i answered si. Here, if si is o
, the animal said that the two neighboring animals are of the same species, and if si is x
, the animal said that the two neighboring animals are of different species.
More formally, a sheep answered o
if the two neighboring animals are both sheep or both wolves, and answered x
otherwise. Similarly, a wolf answered x
if the two neighboring animals are both sheep or both wolves, and answered o
otherwise.
Snuke is wondering whether there is a valid assignment of species to the animals that is consistent with these responses. If there is such an assignment, show one such assignment. Otherwise, print -1
.
Constraints
- 3≤N≤105
- s is a string of length N consisting of
o
andx
.
Input
The input is given from Standard Input in the following format:
- N
- s
Output
If there does not exist an valid assignment that is consistent with s, print -1
. Otherwise, print an string t in the following format. The output is considered correct if the assignment described by t is consistent with s.
- t is a string of length N consisting of
S
andW
. - If ti is
S
, it indicates that the animal numbered i is a sheep. If ti isW
, it indicates that the animal numbered i is a wolf.
Sample Input 1
- 6
- ooxoox
Sample Output 1
- SSSWWS
For example, if the animals numbered 1, 2, 3, 4, 5 and 6 are respectively a sheep, sheep, sheep, wolf, wolf, and sheep, it is consistent with their responses. Besides, there is another valid assignment of species: a wolf, sheep, wolf, sheep, wolf and wolf.
Let us remind you: if the neiboring animals are of the same species, a sheep answers o
and a wolf answers x
. If the neiboring animals are of different species, a sheep answers x
and a wolf answers o
.
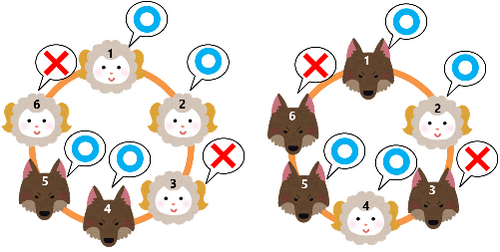
Sample Input 2
- 3
- oox
Sample Output 2
- -1
Print -1
if there is no valid assignment of species.
Sample Input 3
- 10
- oxooxoxoox
- #include <bits/stdc++.h>
- using namespace std;
- const int N=;
- int gh(char *str,char *ch,int n) {
- for(int i=; i<n; i++) {
- if(i<n-) {
- if(str[i]=='o') {
- if(ch[i]=='S') {
- ch[i+]=ch[i-];
- } else {
- if(ch[i-]=='S') ch[i+]='W';
- else ch[i+]='S';
- }
- } else {
- if(ch[i]=='S') {
- if(ch[i-]=='S') ch[i+]='W';
- else ch[i+]='S';
- } else {
- ch[i+]=ch[i-];
- }
- }
- }
- else if(i==n-) {
- if(str[i]=='o') {
- if(ch[i]=='S') {
- if(ch[i+]!=ch[i-]) {
- return ;
- }
- }
- else {
- if(ch[i+]==ch[i-]){
- return ;
- }
- }
- }
- else{
- if(ch[i]=='S'){
- if(ch[i+]==ch[i-]){
- return ;
- }
- }
- else{
- if(ch[i+]!=ch[i-]) {
- return ;
- }
- }
- }
- }
- else if(i==n-){
- if(str[i]=='x'){
- if(ch[i]=='S') {
- if(ch[n-]==ch[]) return ;
- }
- else{
- if(ch[n-]!=ch[]) return ;
- }
- }
- else{
- if(ch[i]=='S'){
- if(ch[n-]!=ch[]) return ;
- }
- else{
- if(ch[n-]==ch[]) return ;
- }
- }
- }
- }
- return ;
- }
- int main() {
- char str[N];
- char ch[N];
- int n;
- int flag=;
- scanf("%d",&n);
- scanf("%s",str);
- ch[]='S';
- if(str[]=='o') {
- memset(ch,,sizeof(ch));
- ch[]='S';
- ch[]='S';
- ch[n-]='S';
- flag=gh(str,ch,n);
- if(flag==) {
- for(int i=; i<n; i++) printf("%c",ch[i]);
- puts("");
- return ;
- } else {
- memset(ch,,sizeof(ch));
- ch[]='S';
- ch[]='W';
- ch[n-]='W';
- flag=gh(str,ch,n);
- if(flag==){
- for(int i=;i<n;i++) printf("%c",ch[i]);
- puts("");
- return ;
- }
- }
- }
- else{
- memset(ch,,sizeof(ch));
- ch[]='S';
- ch[]='S';
- ch[n-]='W';
- flag=gh(str,ch,n);
- if(flag==){
- for(int i=;i<n;i++) printf("%c",ch[i]);
- puts("");
- return ;
- }
- else{
- memset(ch,,sizeof(ch));
- ch[]='S';
- ch[]='W';
- ch[n-]='S';
- flag=gh(str,ch,n);
- if(flag==){
- for(int i=;i<n;i++) printf("%c",ch[i]);
- puts("");
- return ;
- }
- }
- }
- ch[]='W';
- if(str[]=='o'){
- memset(ch,,sizeof(ch));
- ch[]='W';
- ch[]='S';
- ch[n-]='W';
- flag=gh(str,ch,n);
- if(flag==){
- for(int i=;i<n;i++) printf("%c",ch[i]);
- puts("");
- return ;
- }
- else{
- ch[]='W';
- ch[]='W';
- ch[n-]='S';
- flag=gh(str,ch,n);
- if(flag==){
- for(int i=;i<n;i++) printf("%c",ch[i]);
- puts("");
- return ;
- }
- }
- }
- else{
- memset(ch,,sizeof(ch));
- ch[]='W';
- ch[]='S';
- ch[n-]='S';
- flag=gh(str,ch,n);
- if(flag==){
- for(int i=;i<n;i++) printf("%c",ch[i]);
- puts("");
- }
- else{
- ch[]='W';
- ch[]='W';
- ch[n-]='W';
- flag=gh(str,ch,n);
- if(flag==){
- for(int i=;i<n;i++) printf("%c",ch[i]);
- puts("");
- return ;
- }
- }
- }
- puts("-1");
- return ;
- }
AtCoder Regular Contest 069 D的更多相关文章
- AtCoder Regular Contest 069 F Flags 二分,2-sat,线段树优化建图
AtCoder Regular Contest 069 F Flags 二分,2-sat,线段树优化建图 链接 AtCoder 大意 在数轴上放上n个点,点i可能的位置有\(x_i\)或者\(y_i\ ...
- AtCoder Regular Contest 069 D - Menagerie 枚举起点 模拟递推
arc069.contest.atcoder.jp/tasks/arc069_b 题意:一堆不明身份的动物排成一圈,身份可能是羊或狼,羊一定说实话,狼一定说假话.大家各自报自己的两边是同类还是不同类, ...
- AtCoder Regular Contest 069 F - Flags
题意: 有n个点需要摆在一个数轴上,每个点需要摆在ai这个位置或者bi上,问怎么摆能使数轴上相邻两个点之间的距离的最小值最大. 二分答案后显然是个2-sat判定问题,因为边很多而连边的又是一个区间,所 ...
- AtCoder Regular Contest 069
1. C - Scc Puzzle 计算scc的个数,先判断s个数需要多少个cc,多的cc,每四个可以组成一个scc.注意数据范围,使用long long. #include<bits/stdc ...
- AtCoder Regular Contest 061
AtCoder Regular Contest 061 C.Many Formulas 题意 给长度不超过\(10\)且由\(0\)到\(9\)数字组成的串S. 可以在两数字间放\(+\)号. 求所有 ...
- AtCoder Regular Contest 094 (ARC094) CDE题解
原文链接http://www.cnblogs.com/zhouzhendong/p/8735114.html $AtCoder\ Regular\ Contest\ 094(ARC094)\ CDE$ ...
- AtCoder Regular Contest 092
AtCoder Regular Contest 092 C - 2D Plane 2N Points 题意: 二维平面上给了\(2N\)个点,其中\(N\)个是\(A\)类点,\(N\)个是\(B\) ...
- AtCoder Regular Contest 093
AtCoder Regular Contest 093 C - Traveling Plan 题意: 给定n个点,求出删去i号点时,按顺序从起点到一号点走到n号点最后回到起点所走的路程是多少. \(n ...
- AtCoder Regular Contest 094
AtCoder Regular Contest 094 C - Same Integers 题意: 给定\(a,b,c\)三个数,可以进行两个操作:1.把一个数+2:2.把任意两个数+1.求最少需要几 ...
随机推荐
- iOS 蓝牙开发资料记录
一.蓝牙基础认识: 1.iOS蓝牙开发: iOS蓝牙开发:蓝牙连接和数据读写 iOS蓝牙后台运行 iOS关于app连接已配对设备的问题(ancs协议的锅) iOS蓝牙空中 ...
- Hybris 项目工程配置
1.控制台页面进入platform目录 cd F:\hybris640\hybris\bin\platform 并运行 setantenv.bat 生成对应的ant. 2.运行 ant moduleg ...
- xamarin android布局
xamarin android布局练习(1) xamarin android布局练习,基础非常重要,首先要学习的就是android的布局练习,xamarin也一样,做了几个xamarin androi ...
- 英雄联盟LOL用什么语言写的?
是用openGL开发的 开发语言是c/c++ 客户端是一个.net的web界面
- 活动倒计时-兼容ios
最近要做一个活动,需要用倒计时,写好之后再IOS上无效,经过百度知道了,原来IOS不能识别格式"2017-11-09 --",所以要进行转换才有效 直接上代码了: <!DOC ...
- 点击按钮显示隐藏DIV,点击DIV外面隐藏DIV
点击按钮显示隐藏DIV,点击DIV外面隐藏DIV 注意:此方法对touch事件不行,因为stopPropagation并不能阻止touchend的冒泡 <style type="tex ...
- 使用sed,grep 批量修改文件内容
使用sed命令可以进行字符串的批量替换操作,以节省大量的时间及人力: 使用的格式如下: sed -i "s/oldstring/newstring/g" `grep oldstri ...
- 房上的猫:了解java与学习java前的准备
一.java 概述: 1.通常指完成某些事情的一种既定方式和过程 2.程序可以看做对一系列动作执行过程的描述 3.计算机按照某种顺序完成一系列指令的集合称为程序 4.计算机仅识别二进制低级语言 ...
- View学习(四)-View的绘制(draw)过程
View的draw过程相比之于measrue过程,也是比较简单的.并且在我们自定义View时,也经常需要重写onDraw方法,来绘制出我们要实现的效果. 如之前的文章所说,绘制的流程也是起始于View ...
- IndentationError: unexpected indent
都知道python是对格式要求很严格的,写了一些python但是也没发现他严格在哪里,今天遇到了IndentationError: unexpected indent错误我才知道他是多么的严格. ...