【线段树】Atlantis
Time Limit: 1000MS | Memory Limit: 10000K | |
Total Submissions: 23181 | Accepted: 8644 |
Description
Input
The input file is terminated by a line containing a single 0. Don't process it.
Output
Output a blank line after each test case.
Sample Input
2
10 10 20 20
15 15 25 25.5
0
Sample Output
Test case #1
Total explored area: 180.00
Source
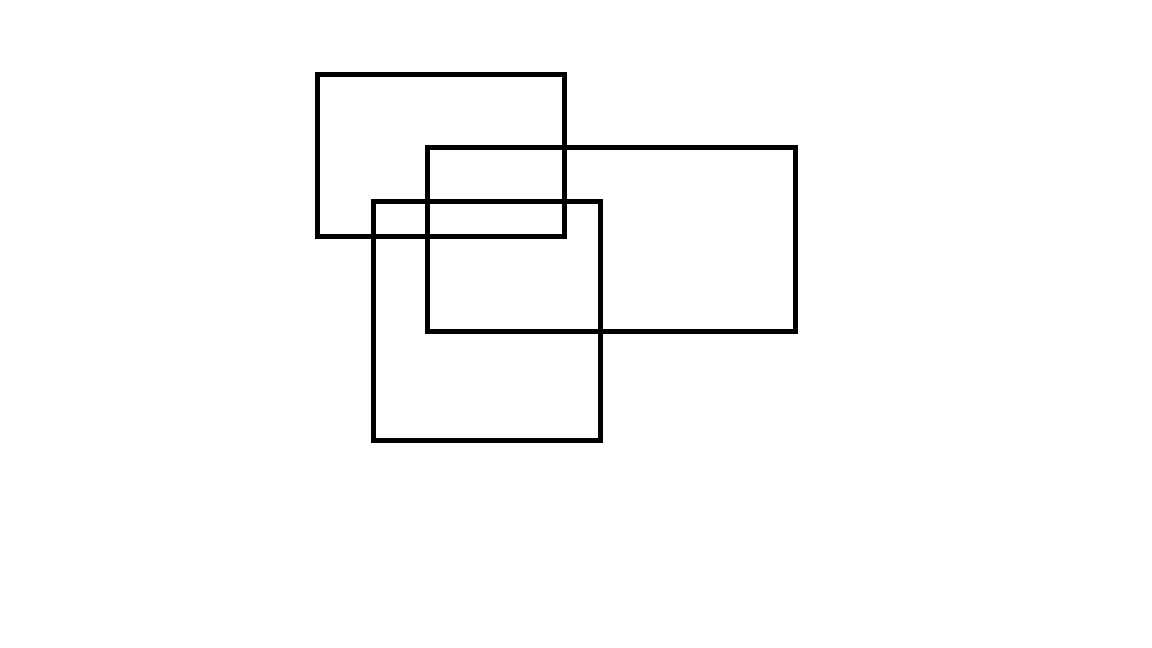
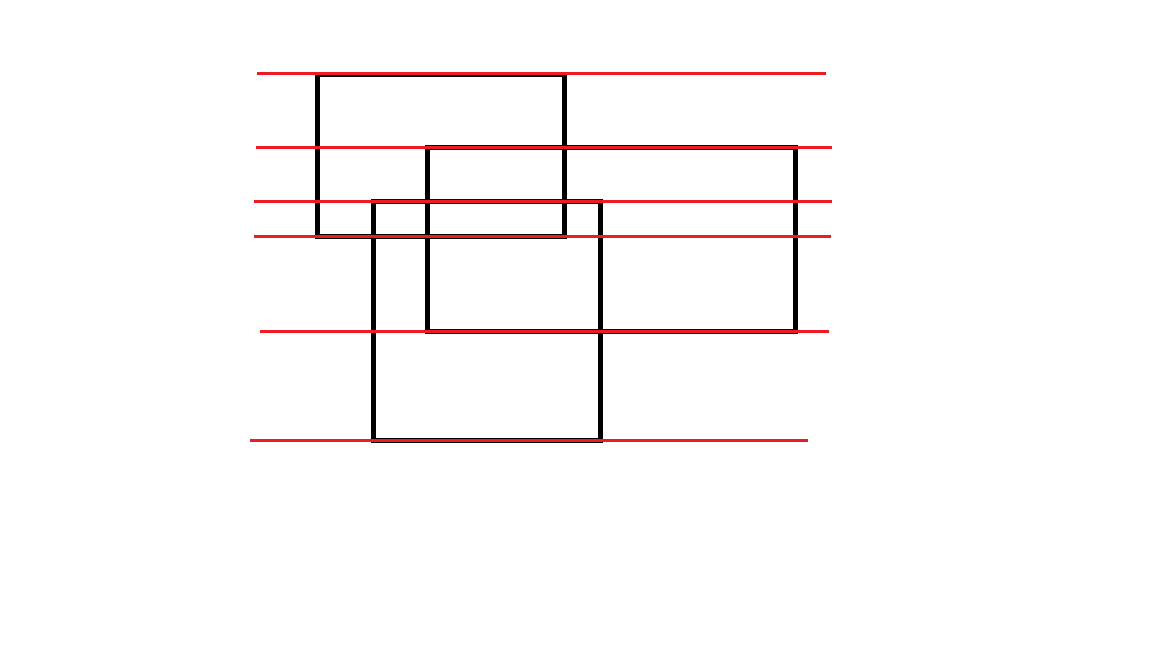
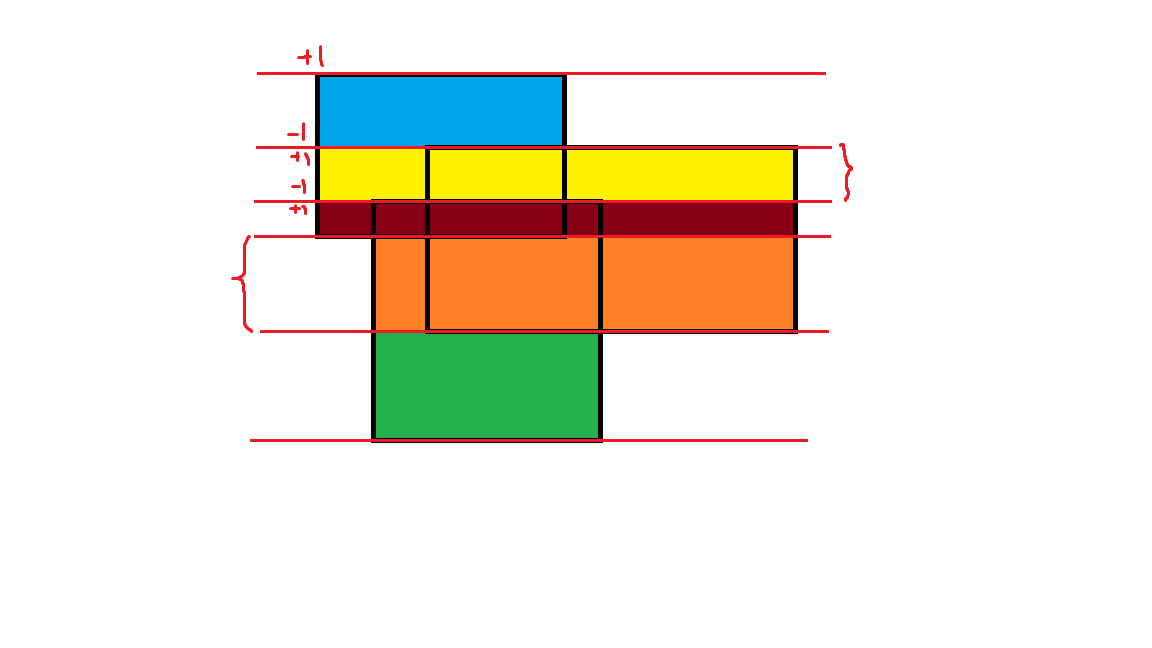
#include<iostream>
#include<cstring>
#include<cstdio>
#include<vector>
#include<queue>
#include<stack>
#include<algorithm>
using namespace std; inline int read(){
int x=0,f=1;char c=getchar();
for(;!isdigit(c);c=getchar()) if(c=='-') f=-1;
for(;isdigit(c);c=getchar()) x=x*10+c-'0';
return x*f;
}
const int MAXN=100001;
const int INF=999999;
int N,M;
double a[100001],b[100001];
int A[100001];
struct data2{
double sum,len;
int ct;
}tr[1000001];
int tmp,tmp2;
struct data{
double x,y1,y2;
bool end;
}poi[1000001];
int T; bool cmp(data a,data b){
return a.x<b.x;
}
void build(int l,int r,int rt){
tr[rt].ct=0;tr[rt].sum=0;
tr[rt].len=a[r]-a[l];
if(l+1>=r) return ;
int mid=(l+r)>>1;
build(l,mid,rt*2);
build(mid,r,rt*2+1);
return ;
}
void Update(int rt,int l,int r){
if(tr[rt].ct) tr[rt].sum=tr[rt].len;
else if(r-l>1) tr[rt].sum=tr[rt*2].sum+tr[rt*2+1].sum;
else tr[rt].sum=0;
return ;
}
void add(int l,int r,int rt,int L,int R){
if(L==l&&R==r){
tr[rt].ct++;
Update(rt,l,r);
return ;
}
int mid=(l+r)>>1;
if(L>=mid) add(mid,r,rt*2+1,L,R);
else if(R<=mid) add(l,mid,rt*2,L,R);
else{
add(mid,r,rt*2+1,mid,R);
add(l,mid,rt*2,L,mid);
}
Update(rt,l,r);
return;
}
void del(int l,int r,int rt,int L,int R){
if(L==l&&R==r){
tr[rt].ct--;
Update(rt,l,r);
return ;
}
int mid=(l+r)>>1;
if(L>=mid) del(mid,r,rt*2+1,L,R);
else if(R<=mid) del(l,mid,rt*2,L,R);
else{
del(mid,r,rt*2+1,mid,R);
del(l,mid,rt*2,L,mid);
}
Update(rt,l,r);
return;
} double ans;
int main(){
while(1){
N=read();
if(!N) break;
tmp2=tmp=0;double x1,x2,y1,y2;
for(int i=1;i<=N;i++){
cin>>x1>>y1>>x2>>y2;
poi[++tmp].x=x1;
poi[tmp].y1=y1;
poi[tmp].y2=y2;
poi[tmp].end=false;
poi[++tmp].x=x2;
poi[tmp].y1=y1;
poi[tmp].y2=y2;
poi[tmp].end=true;
}
for(int i=1;i<=tmp;i+=2){
a[tmp2]=b[tmp2]=poi[i].y1;tmp2++;
a[tmp2]=b[tmp2]=poi[i].y2;tmp2++;
}
sort(poi+1,poi+tmp+1,cmp);
sort(a,a+tmp2);
int tmp3=0,tmp4=0;
tmp3=unique(a,a+tmp2)-a;
ans=0;
build(0,tmp3-1,1);
double lasth;
for(int i=1;i<tmp;i++){
if(!poi[i].end) add(0,tmp3-1,1,lower_bound(a,a+tmp3,poi[i].y1)-a,lower_bound(a,a+tmp3,poi[i].y2)-a);
else del(0,tmp3-1,1,lower_bound(a,a+tmp3,poi[i].y1)-a,lower_bound(a,a+tmp3,poi[i].y2)-a);
ans+=(double)((poi[i+1].x-poi[i].x)*tr[1].sum);
}
printf("Test case #%d\nTotal explored area: %.2f\n\n",++T,ans);
}
}
【线段树】Atlantis的更多相关文章
- 线段树---Atlantis
题目网址:http://acm.hust.edu.cn/vjudge/contest/view.action?cid=110064#problem/A Description There are se ...
- hdu 1542 Atlantis(线段树,扫描线)
Atlantis Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others) Total S ...
- HDU 1542 Atlantis(线段树扫描线+离散化求面积的并)
Atlantis Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others) Total S ...
- POJ 1542 Atlantis(线段树 面积 并)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1542 参考网址:http://blog.csdn.net/sunmenggmail/article/d ...
- 【HDU 1542】Atlantis 矩形面积并(线段树,扫描法)
[题目] Atlantis Problem Description There are several ancient Greek texts that contain descriptions of ...
- 【POJ1151】Atlantis(线段树,扫描线)
[POJ1151]Atlantis(线段树,扫描线) 题面 Vjudge 题解 学一学扫描线 其实很简单啦 这道题目要求的就是若干矩形的面积和 把扫描线平行于某个轴扫过去(我选的平行\(y\)轴扫) ...
- hdu1542 Atlantis 线段树--扫描线求面积并
There are several ancient Greek texts that contain descriptions of the fabled island Atlantis. Some ...
- HDU 1542 Atlantis(线段树面积并)
描述 There are several ancient Greek texts that contain descriptions of the fabled island Atlantis. S ...
- hdu1542 Atlantis (线段树+扫描线+离散化)
Atlantis Time Limit: 2000/1000 MS (Java/Others) Memory Limit: 65536/32768 K (Java/Others) Total S ...
- HDU 1542 - Atlantis - [线段树+扫描线]
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=1542 Time Limit: 2000/1000 MS (Java/Others) Memory Li ...
随机推荐
- Http跨域时候预检没通过的几种原因
网上大多数涉及的原因(直接复制粘帖): CORS把HTTP请求分成两类,不同类别按不同的策略进行跨域资源共享协商. 1. 简单跨域请求. 当HTTP请求出现以下两种情况时,浏览器认为是简单跨域请求: ...
- 撩下Cookie和Session
Cookie Cookie其实还可以用在一些方便用户的场景下,设想你某次登陆过一个网站,下次登录的时候不想再次输入账号了,怎么办?这个信息可以写到Cookie里面,访问网站的时候,网站页面的脚本可以读 ...
- Yii2实现读写分离(MySQL主从数据库)
读写分离(Read/Write Splitting). 1.原理: 让主数据库(master)处理事务性增.改.删操作(INSERT.UPDATE.DELETE),而从数据库(slave)处理SELE ...
- 2017ACM暑期多校联合训练 - Team 1 1001 HDU 6033 Add More Zero (数学)
题目链接 Problem Description There is a youngster known for amateur propositions concerning several math ...
- ubuntu下调整cpu频率
环境:ubuntu15.10 查看内核支持的cpu策略 cat /sys/devices/system/cpu/cpu0/cpufreq/scaling_available_governors 比如我 ...
- 利用itext将html转为pdf
亲测代码没有问题,需要注意细节已经标注:需要jar包:iText-2.0.8.jar:core-renderer-R8.jar: core-renderer-R8.jar下载地址:http://cen ...
- mysql 之修改初始密码
转载自:https://www.cnblogs.com/ivictor/p/5142809.html 为了加强安全性,MySQL5.7为root用户随机生成了一个密码,在error log中,关于er ...
- linux命令行todo列表管理工具Taskwarrior介绍
Taskwarrior 是一款在命令行下使用的TODO列表管理工具,或者说任务管理工具,灵活,快速,高效. 安装 在ubuntu 14.04 中,可从官方仓库安装task软件包 sudo apt-ge ...
- Linux(Unix)密码策略问题导致root密码不能修改
Linux(Unix)密码策略问题导致root密码不能修改 发布时间: 2016-01-19 浏览次数: 1034 下载次数: 5 用户修改了密码配置文件,导致root账户修改密码时报如下错误: ...
- Python爬虫数据处理
一.首先理解下面几个函数 设置变量 length()函数 char_length() replace() 函数 max() 函数1.1.设置变量 set @变量名=值 set @address='中国 ...