[iOS微博项目 - 4.0] - 自定义微博cell
- 创建自定义cell的雏形
- cell包含:内容、工具条
- 内容包含:原创内容、转发内容

- 使用分层控件,逐层实现
- 分离model和view
- model:数据模型、frame模型
- view:就是控件本身
- frame模型:包含数据模型和子控件frame
- 根据数据模型来决定子控件是否显示(例如转发内容)

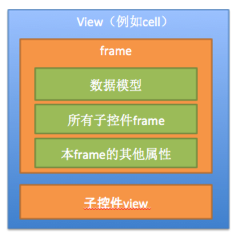

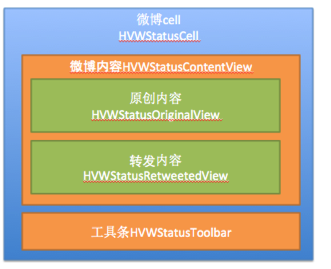
//
// HVWStatusCell.m
// HVWWeibo
//
// Created by hellovoidworld on 15/2/12.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import "HVWStatusCell.h"
#import "HVWStatusContentView.h"
#import "HVWStatusToolbar.h" @interface HVWStatusCell() /** 微博内容控件 */
@property(nonatomic, weak) HVWStatusContentView *statusContentView; /** 微博工具条控件 */
@property(nonatomic, weak) HVWStatusToolbar *toolbar; @end @implementation HVWStatusCell - (instancetype)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString *)reuseIdentifier {
self = [super initWithStyle:style reuseIdentifier:reuseIdentifier]; if (self) { // 初始化子控件开始
// 初始化微博内容控件
[self setupStatusContentView]; // 初始化工具条控件 */
[self setupToolbar];
} return self;
} /** 初始化微博内容控件 */
- (void) setupStatusContentView {
HVWStatusContentView *statusContentView = [[HVWStatusContentView alloc] init];
self.statusContentView = statusContentView;
[self.contentView addSubview:statusContentView];
} /** 初始化工具条控件 */
- (void) setupToolbar {
HVWStatusToolbar *toolbar = [[HVWStatusToolbar alloc] init];
self.toolbar = toolbar;
[self.contentView addSubview:toolbar];
} @end
//
// HVWStatusContentView.m
// HVWWeibo
//
// Created by hellovoidworld on 15/2/12.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import "HVWStatusContentView.h"
#import "HVWStatusOriginalView.h"
#import "HVWStatusRetweetedView.h" @interface HVWStatusContentView() /** 原创内容 */
@property(nonatomic, weak) HVWStatusOriginalView *originalView; /** 转发内容 */
@property(nonatomic, weak) HVWStatusRetweetedView *retweetedView; @end @implementation HVWStatusContentView - (instancetype)initWithFrame:(CGRect)frame {
self = [super initWithFrame:frame]; if (self) { // 初始化子控件开始
// 初始化原创内容控件
[self setupOriginalView]; // 初始化转发内容控件
[self setupRetweetedView];
} return self;
} /** 初始化原创内容控件 */
- (void) setupOriginalView { } /** 初始化转发内容控件 */
- (void) setupRetweetedView { } @end
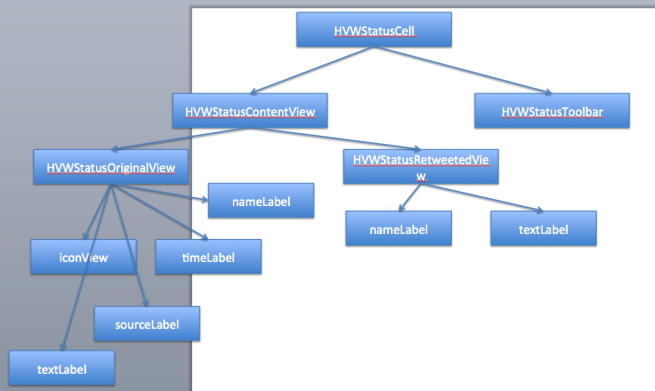
//
// HVWStatusCell.h
// HVWWeibo
//
// Created by hellovoidworld on 15/2/12.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import <UIKit/UIKit.h> @interface HVWStatusCell : UITableViewCell + (instancetype) cellWithTableView:(UITableView *)tableView; @end
//
// HVWStatusCell.m
// HVWWeibo
//
// Created by hellovoidworld on 15/2/12.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import "HVWStatusCell.h"
#import "HVWStatusContentView.h"
#import "HVWStatusToolbar.h" @interface HVWStatusCell() /** 微博内容控件 */
@property(nonatomic, weak) HVWStatusContentView *statusContentView; /** 微博工具条控件 */
@property(nonatomic, weak) HVWStatusToolbar *toolbar; @end @implementation HVWStatusCell /** 创建 */
+ (instancetype) cellWithTableView:(UITableView *)tableView {
static NSString *ID = @"HVWStatusCell";
HVWStatusCell *cell = [tableView dequeueReusableCellWithIdentifier:ID]; if (nil == cell) {
cell = [[self alloc] initWithStyle:UITableViewCellStyleSubtitle reuseIdentifier:ID];
} return cell;
} /** 初始化 */
- (instancetype)initWithStyle:(UITableViewCellStyle)style reuseIdentifier:(NSString *)reuseIdentifier {
self = [super initWithStyle:style reuseIdentifier:reuseIdentifier]; if (self) { // 初始化子控件开始
// 初始化微博内容控件
[self setupStatusContentView]; // 初始化工具条控件 */
[self setupToolbar];
} return self;
} /** 初始化微博内容控件 */
- (void) setupStatusContentView {
HVWStatusContentView *statusContentView = [[HVWStatusContentView alloc] init];
self.statusContentView = statusContentView;
[self.contentView addSubview:statusContentView];
} /** 初始化工具条控件 */
- (void) setupToolbar {
HVWStatusToolbar *toolbar = [[HVWStatusToolbar alloc] init];
self.toolbar = toolbar;
[self.contentView addSubview:toolbar];
} @end
//
// HVWStatusContentView.h
// HVWWeibo
//
// Created by hellovoidworld on 15/2/12.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import <UIKit/UIKit.h> @interface HVWStatusContentView : UIView @end
//
// HVWStatusContentView.m
// HVWWeibo
//
// Created by hellovoidworld on 15/2/12.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import "HVWStatusContentView.h"
#import "HVWStatusOriginalView.h"
#import "HVWStatusRetweetedView.h" @interface HVWStatusContentView() /** 原创内容 */
@property(nonatomic, weak) HVWStatusOriginalView *originalView; /** 转发内容 */
@property(nonatomic, weak) HVWStatusRetweetedView *retweetedView; @end @implementation HVWStatusContentView - (instancetype)initWithFrame:(CGRect)frame {
self = [super initWithFrame:frame]; if (self) { // 初始化子控件开始
// 初始化原创内容控件
[self setupOriginalView]; // 初始化转发内容控件
[self setupRetweetedView];
} return self;
} /** 初始化原创内容控件 */
- (void) setupOriginalView { } /** 初始化转发内容控件 */
- (void) setupRetweetedView { } @end
//
// HVWStatusToolbar.h
// HVWWeibo
//
// Created by hellovoidworld on 15/2/12.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import <UIKit/UIKit.h> @interface HVWStatusToolbar : UIView @end
//
// HVWStatusToolbar.m
// HVWWeibo
//
// Created by hellovoidworld on 15/2/12.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import "HVWStatusToolbar.h" @implementation HVWStatusToolbar /** 代码自定义初始化方法 */
- (instancetype)initWithFrame:(CGRect)frame {
self = [super initWithFrame:frame]; if (self) {
self.backgroundColor = [UIColor greenColor];
} return self;
} @end
//
// HVWStatusOriginalView.h
// HVWWeibo
//
// Created by hellovoidworld on 15/2/12.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import <UIKit/UIKit.h> @interface HVWStatusOriginalView : UIView @end
//
// HVWStatusOriginalView.m
// HVWWeibo
//
// Created by hellovoidworld on 15/2/12.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import "HVWStatusOriginalView.h" @interface HVWStatusOriginalView() /** 昵称 */
@property(nonatomic, weak) UILabel *nameLabel; /** 头像 */
@property(nonatomic, weak) UIImageView *iconView; /** 微博发表时间 */
@property(nonatomic, weak) UILabel *timeLabel; /** 微博来源 */
@property(nonatomic, weak) UILabel *sourceLabel; /** 微博文本内容 */
@property(nonatomic, weak) UILabel *textLabel; @end @implementation HVWStatusOriginalView /** 代码初始化方法 */
- (instancetype)initWithFrame:(CGRect)frame {
self = [super initWithFrame:frame]; if (self) { // 初始化子控件开始
// 昵称
UILabel *nameLabel = [[UILabel alloc] init];
nameLabel.font = HVWStatusOriginalNameFont;
self.nameLabel = nameLabel;
[self addSubview:nameLabel]; // 头像
UIImageView *iconView = [[UIImageView alloc] init];
self.iconView = iconView;
[self addSubview:iconView]; // 发表时间
UILabel *timeLabel = [[UILabel alloc] init];
self.timeLabel = timeLabel;
[self addSubview:timeLabel]; // 来源
UILabel *sourceLabel = [[UILabel alloc] init];
self.sourceLabel = sourceLabel;
[self addSubview:sourceLabel]; // 正文
UILabel *textLabel = [[UILabel alloc] init];
self.textLabel = textLabel;
[self addSubview:textLabel];
} return self;
} @end
//
// HVWStatusRetweetedView.h
// HVWWeibo
//
// Created by hellovoidworld on 15/2/12.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import <UIKit/UIKit.h> @interface HVWStatusRetweetedView : UIView @end
//
// HVWStatusRetweetedView.m
// HVWWeibo
//
// Created by hellovoidworld on 15/2/12.
// Copyright (c) 2015年 hellovoidworld. All rights reserved.
// #import "HVWStatusRetweetedView.h" @interface HVWStatusRetweetedView() /** 昵称 */
@property(nonatomic, weak) UILabel *nameLabel; /** 微博文本内容 */
@property(nonatomic, weak) UILabel *textLabel; @end @implementation HVWStatusRetweetedView /** 代码初始化方法 */
- (instancetype)initWithFrame:(CGRect)frame {
self = [super initWithFrame:frame]; if (self) { // 初始化子控件开始
// 昵称
UILabel *nameLabel = [[UILabel alloc] init];
nameLabel.font = HVWStatusOriginalNameFont;
self.nameLabel = nameLabel;
[self addSubview:nameLabel]; // 正文
UILabel *textLabel = [[UILabel alloc] init];
self.textLabel = textLabel;
[self addSubview:textLabel];
} return self;
} @end
[iOS微博项目 - 4.0] - 自定义微博cell的更多相关文章
- [iOS微博项目 - 3.0] - 手动刷新微博
github: https://github.com/hellovoidworld/HVWWeibo A.下拉刷新微博 1.需求 在“首页”界面,下拉到一定距离的时候刷新微博数据 刷新数据的时候使 ...
- [iOS微博项目 - 3.2] - 发送微博
github: https://github.com/hellovoidworld/HVWWeibo A.使用微博API发送微博 1.需求 学习发送微博API 发送文字微博 发送带有图片的微博 ...
- [iOS微博项目 - 2.0] - OAuth授权3步
A.概念 OAUTH协议为用户资源的授权提供了一个安全的.开放而又简易的标准.与以往的授权方式不同之处是OAUTH的授权不会使第三方触及到用户的帐号信息(如用户名与密码),即第三方无需使用用 ...
- [iOS微博项目 - 1.6] - 自定义TabBar
A.自定义TabBar 1.需求 控制TabBar内的item的文本颜色(普通状态.被选中状态要和图标一致).背景(普通状态.被选中状态均为透明) 重新设置TabBar内的item位置,为下一步在Ta ...
- [iOS微博项目 - 1.0] - 搭建基本框架
A.搭建基本环境 github: https://github.com/hellovoidworld/HVWWeibo 项目结构: 1.使用代码构建UI,不使用storyboard ...
- AJ学IOS 之微博项目实战(12)发送微博自定义工具条代理实现点击事件
AJ分享,必须精品 一:效果 二:封装好的工具条 NYComposeToolbar.h 带代理方法 #import <UIKit/UIKit.h> typedef enum { NYCom ...
- AJ学IOS 之微博项目实战(11)发送微博自定义TextView实现带占位文字
AJ分享,必须精品 一:效果 二:代码: 由于系统自带的UITextField:和UITextView:不能满足我们的需求,所以我们需要自己设计一个. UITextField: 1.文字永远是一行,不 ...
- [iOS微博项目 - 3.1] - 发微博界面
github: https://github.com/hellovoidworld/HVWWeibo A.发微博界面:自定义UITextView 1.需求 用UITextView做一个编写微博的输 ...
- [iOS微博项目 - 2.6] - 获取微博数据
github: https://github.com/hellovoidworld/HVWWeibo A.新浪获取微博API 1.读取微博API 2.“statuses/home_time ...
随机推荐
- Activity生命运行中的几个方法
给大家看看一个周期图
- CA证书的信认
为什么不同的品牌证书,价钱不一样: 衡量证书一般是看通用型,就是大家认不认你,或者说,你买的证书的根证书有没有被设备内置,内置了,你就是可信的, 没内置,就没法用.
- struts2防止表单重复提交的解决方案
一.造成重复提交主要的两个原因: 在平时的开发过程中,经常可以遇到表单重复提交的问题,如做一个注册页面,如果表单重复提交,那么一个用户就会注册多次,重复提交主要由于两种原因. 1. 一是,服务器 ...
- ognl概念和原理详解
一.问题的提出 在mvc中,数据是在各个层次之间进行流转是一个不争的事实.而这种流转,也就会面临一些困境,这些困境,是由于数据在不同世界中的表现形式不同而造成的: 1. 数据在页面上是一个扁平的, ...
- Nginx设置expires设定页面缓存时间 不缓存或一直使用缓存
配置expires expires起到控制页面缓存的作用,合理的配置expires可以减少很多服务器的请求 要配置expires,可以在http段中或者server段中或者location段中加入 l ...
- dp之多重背包poj1276
题意:有现今cash,和n种钱币,每种钱币有ni个,价值为di,求各种钱币组成的不超过cash的最大钱数....... 思路:二进制拆分转化为01背包,或者转化为完全背包都是可以的. 反思:这个题目我 ...
- Java 之泛型通配符 ? extends T 与 ? super T 解惑
简述 大家在平时的工作学习中, 肯定会见过不少如下的语句: List<? super T> List<? extends T> 我们都知道, 上面的代码时关于 Java 泛型的 ...
- arm + fpga 核心板
- 树莓派+android things+实时音视频传输demo之遥控小车
做了个测试小车,上面安装了摄像头,通过外网进行视频传输: https://www.bilibili.com/video/av23817880/
- 线段树 + 字符串Hash - Codeforces 580E Kefa and Watch
Kefa and Watch Problem's Link Mean: 给你一个长度为n的字符串s,有两种操作: 1 L R C : 把s[l,r]全部变为c; 2 L R d : 询问s[l,r]是 ...