2019 GDUT Rating Contest I : Problem A. The Bucket List
题面:
A. The Bucket List
FJ has a storage room containing buckets that are sequentially numbered with labels 1, 2, 3, and so on. In his current milking strategy, whenever some cow (say, cow i) starts milking (at time si), FJ runs to the storage room and collects the bi buckets with the smallest available labels and allocates these for milking cow i.
题目描述:
题目分析:

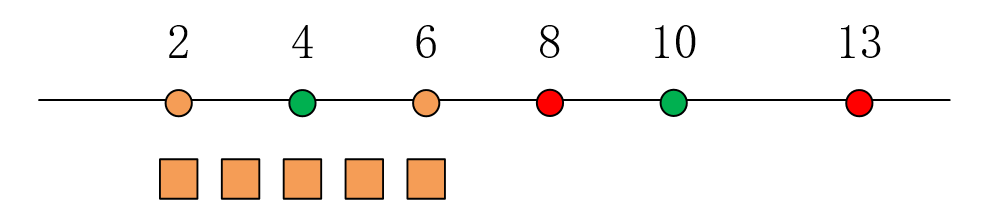
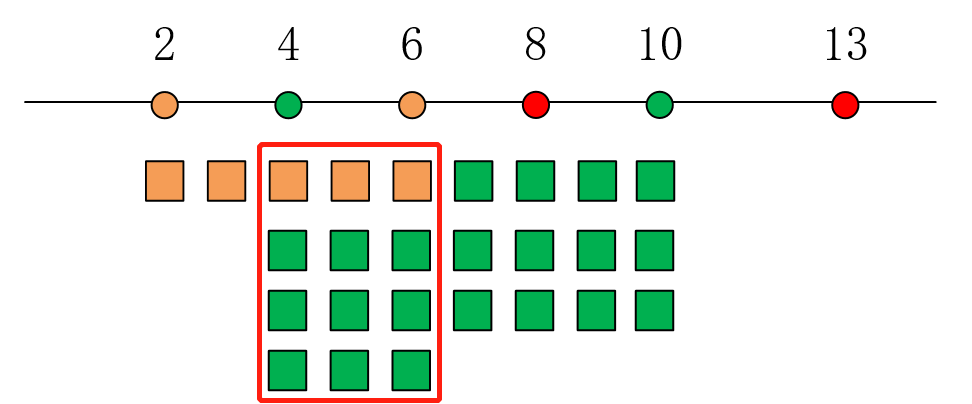
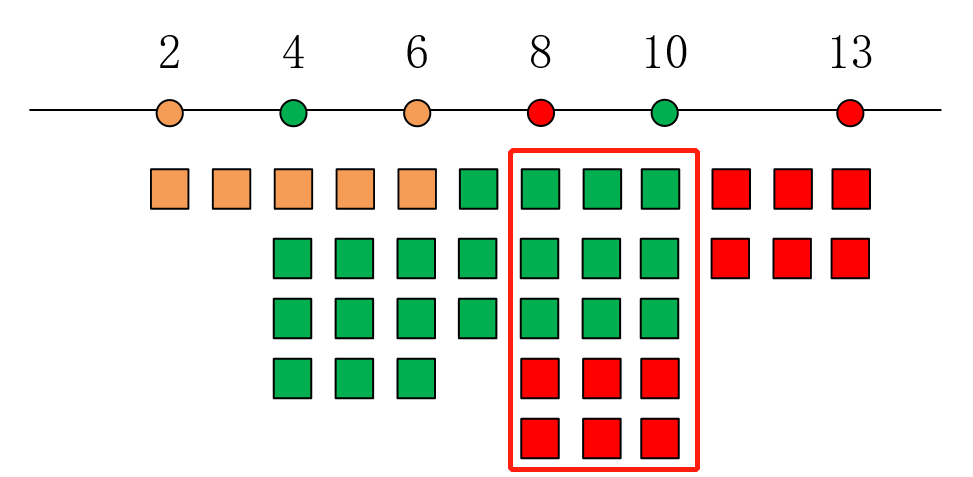
- 1 #include <cstdio>
- 2 #include <cstring>
- 3 #include <iostream>
- 4 using namespace std;
- 5 int n, s[105], b[105], t[105];
- 6 int bk[1005]; //桶
- 7
- 8 int main(){
- 9 memset(bk, 0, sizeof(bk));
- 10 cin >> n;
- 11 for(int i = 0; i < n; i++){
- 12 cin >> s[i] >> t[i] >> b[i];
- 13 }
- 14
- 15 for(int i = 0; i < n; i++){
- 16 for(int k = s[i]; k <= t[i]; k++){
- 17 bk[k] += b[i];
- 18 }
- 19 }
- 20
- 21 int maxn = 0;
- 22 for(int i = 0; i <= 1000; i++){
- 23 if(bk[i] > maxn){
- 24 maxn = bk[i];
- 25 }
- 26 }
- 27
- 28 cout << maxn << endl;
- 29 return 0;
- 30 }
2019 GDUT Rating Contest I : Problem A. The Bucket List的更多相关文章
- 2019 GDUT Rating Contest II : Problem F. Teleportation
题面: Problem F. Teleportation Input file: standard input Output file: standard output Time limit: 15 se ...
- 2019 GDUT Rating Contest III : Problem D. Lemonade Line
题面: D. Lemonade Line Input file: standard input Output file: standard output Time limit: 1 second Memo ...
- 2019 GDUT Rating Contest I : Problem H. Mixing Milk
题面: H. Mixing Milk Input file: standard input Output file: standard output Time limit: 1 second Memory ...
- 2019 GDUT Rating Contest I : Problem G. Back and Forth
题面: G. Back and Forth Input file: standard input Output file: standard output Time limit: 1 second Mem ...
- 2019 GDUT Rating Contest III : Problem E. Family Tree
题面: E. Family Tree Input file: standard input Output file: standard output Time limit: 1 second Memory ...
- 2019 GDUT Rating Contest III : Problem C. Team Tic Tac Toe
题面: C. Team Tic Tac Toe Input file: standard input Output file: standard output Time limit: 1 second M ...
- 2019 GDUT Rating Contest III : Problem A. Out of Sorts
题面: 传送门 A. Out of Sorts Input file: standard input Output file: standard output Time limit: 1 second M ...
- 2019 GDUT Rating Contest II : Problem G. Snow Boots
题面: G. Snow Boots Input file: standard input Output file: standard output Time limit: 1 second Memory ...
- 2019 GDUT Rating Contest II : Problem C. Rest Stops
题面: C. Rest Stops Input file: standard input Output file: standard output Time limit: 1 second Memory ...
随机推荐
- zzuli-2259 matrix
题目描述 在麦克雷的面前有N个数,以及一个R*C的矩阵.现在他的任务是从N个数中取出 R*C 个,并填入这个矩阵中.矩阵每一行的法值为本行最大值与最小值的差,而整个矩阵的法值为每一行的法值的最大值.现 ...
- 关于优先队列的总结II
优先队列这个数据结构还是很有用的,可以帮我们解决很多棘手的排序的问题,所以再来细细看一下, priority_queue<Type, Container, Functional> Type ...
- springboot(六)Email demo
项目中经常使用邮件发送提醒功能,比如说更新安全机制,发送邮件通知用户等 一.简单邮件发送 导入依赖: <dependency> <groupId>org.springframe ...
- 大数据开发-linux后台运行,关闭,查看后台任务
在日常开发过程中,除了例行调度的任务和直接在开发环境下比如Scripts,开发,很多情况下是shell下直接搞起(小公司一般是这样),看一下常见的linux后台运行和关闭的命令,这里做一个总结,主要包 ...
- funny 生成器
funny 生成器 https://www.zhihu.com/question/380741546/answer/1190570384 举牌小人生成器 https://small-upup.upup ...
- element-ui的树型结构图,带有复选框的,没有子项的,横排展示
// 修改树形图样式,如果不含有下箭头的块,要变成行内样式 treeChildInline(){ let hasCaretRight = $("#permission_panel" ...
- BGV再度爆发,流通市值破500万美金!
BGV似乎以超乎寻常的姿态,开启了爆发的模式.这两天,BGV一路上涨,日内最高涨至548.78美金,24小时成交额达到了98.07万美金,24小时成交量达到1844.93枚BGV,流通市值更是突破了5 ...
- rabbitMQ高可用方案
普通模式 默认的集群模式,以两个节点(rabbit01.rabbit02)为例来进行说明.对于Queue来说,消息实体只存在于其中一个节点rabbit01(或者rabbit02),rabbit01和r ...
- JDBC概念理解
##JDBC: 概念:Java DataBase Connectivity Java 数据库连接 Java语言操作数据库 JDBC本质:其实是官方(sun公司)定义的一套操作所有关系型数据库的规则 ...
- WPF 解决内置谷歌浏览器(Cef.ChromiumWebBrowser)在触摸屏无法进行滚动的问题
1.问题描述: 最近在WPF的项目中,需要在控件中嵌套可以浏览特定网页的内容,所以使用了 Cef.ChromiumWebBrowser来解决问题.在执行项目的过程中,主要碰到的问题有: 1.1 当把项 ...