UVA-1533 Moving Pegs (路径寻找问题)
Description
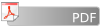
Venture MFG Company, Inc. has made a game board. This game board has 15 holes and these holes are filled with pegs except one hole. A peg can jump over one or more consecutive peg s to the nearest empty hole along the straight line. As a peg jump over the pegs you remove them from the board. In the following figure, the peg at the hole number 12 or the peg at the hole number 14 can jump to the empty hole number 5. If the peg at the hole number 12 is moved then the peg at the hole number 8 is removed. Instead, if the peg at the hole number 14 is moved then the peg at the hole number 9 is removed.
Write a program which find a shortest sequence of moving pegs to leave the last peg in the hole that was initially empty. If such a sequence does not exist the program should write a message ``IMPOSSIBLE".
Input
The input consists of T test cases. The number of test cases (T) is given in the first line of the input file. Each test case is a single integer which means an empty hole number.
Output
For each test case, the first line of the output file contains an integer which is the number of jumps in a shortest sequence of moving pegs. In the second line of the output file, print a sequence of peg movements. A peg movement consists of a pair o f integers separated by a space. The first integer of the pair denotes the hole number of the peg that is moving, and the second integer denotes a destination (empty) hole number.
If there are multiple solutions, output the lexicographically smallest one.
Sample Input
1
5
Sample Output
10
12 5 3 8 15 12 6 13 7 9 1 7 10 8 7 9 11 14 14 5 题目大意:在如图中的棋盘(固定5行)上,每个棋子的走法类似于象棋中“炮”的走法,只能隔着棋子沿直线走,每走一步造成的效果是该棋子落到第一个空白处,并且沿途经过的棋子全部消失。求使最后一个棋子恰好落在第n个点上的最短、字典序最小的路径。
题目分析:这道题说白了有15个位置,每个位置上可能有棋子也可能没有棋子,棋子的状况总共有2^15种。起点是(2^15)-1,终点是1<<(n-1),BFS即可,状态转移也不难,但比较复杂。 代码如下:
# include<iostream>
# include<cstdio>
# include<queue>
# include<map>
# include<cmath>
# include<string>
# include<cstring>
# include<algorithm> using namespace std; const int tot=(1<<15)-1; struct node
{
int s,t;
string step;
node(int _s,int _t,string _step):s(_s),t(_t),step(_step){}
bool operator < (const node &a) const {
if(t==a.t)
return step>a.step;
return t>a.t;
}
}; int mark[1<<15];
map<int,char>mp;
int d[6][2]={{-1,-1},{-1,0},{0,-1},{0,1},{1,0},{1,1}}; int get_pos(int x,int y)
{
return x*(x-1)/2+y;
} void get_XY(int n,int &x,int &y)
{
x=1;
for(int i=1;i<=5&&n-i>0;++i)
++x,n-=i;
y=n;
} bool ok(int x,int y)
{
if(x>=1&&x<=5&&y>=1&&y<=x)
return true;
return false;
} void print(string p)
{
for(int i=0;i<p.size();++i)
printf("%d%c",p[i]-'A'+1,(i==p.size()-1)?'\n':' ');
} void bfs(int goal)
{
priority_queue<node>q;
memset(mark,0,sizeof(mark));
mark[tot^goal]=1;
q.push(node(tot^goal,0,""));
while(!q.empty())
{
node u=q.top();
q.pop();
//cout<<u.t<<' '<<u.s<<' '<<u.step<<endl;
if(u.s==goal){
printf("%d\n",u.t);
print(u.step);
return ;
}
int x,y;
for(int i=1;i<=15;++i){
if(u.s&(1<<(i-1))){
get_XY(i,x,y);
for(int j=0;j<6;++j){
int nx=x+d[j][0],ny=y+d[j][1];
if(!ok(nx,ny))
continue;
int pos=get_pos(nx,ny);
if(!(u.s&(1<<(pos-1))))
continue;
int s=u.s^(1<<(i-1));
while(u.s&(1<<(pos-1)))
{
s^=(1<<(pos-1));
nx=nx+d[j][0],ny=ny+d[j][1];
if(!ok(nx,ny))
break;
pos=get_pos(nx,ny);
}
s^=(1<<(pos-1));
string step=u.step+mp[i];
step+=mp[get_pos(nx,ny)];
if(ok(nx,ny)&&!mark[s]){
mark[s]=1;
q.push(node(s,u.t+1,step));
}
}
}
}
}
printf("IMPOSSIBLE\n");
} int main()
{
for(int i=1;i<=15;++i)
mp[i]=i+'A'-1;
int T,n;
scanf("%d",&T);
while(T--)
{
scanf("%d",&n);
bfs(1<<(n-1));
}
return 0;
}
UVA-1533 Moving Pegs (路径寻找问题)的更多相关文章
- 八数码问题+路径寻找问题+bfs(隐式图的判重操作)
Δ路径寻找问题可以归结为隐式图的遍历,它的任务是找到一条凑够初始状态到终止问题的最优路径, 而不是像回溯法那样找到一个符合某些要求的解. 八数码问题就是路径查找问题背景下的经典训练题目. 程序框架 p ...
- 基于JavaFX图形界面演示的迷宫创建与路径寻找
事情的起因是收到了一位网友的请求,他的java课设需要设计实现迷宫相关的程序--如标题概括. 我这边不方便透露相关信息,就只把任务要求写出来. 演示视频指路: 视频过审后就更新链接 完整代码链接: 网 ...
- 【路径寻找问题】UVa 10603 - Fill
如家大神书上的例题.第一次接触也是按代码敲得.敲的过程感觉很直观.但自己写估计会写的乱七八糟.以后不能砍得难就不愿意做这种题.否则只能做一些水题了.(PS:48) 紫书 #include<ios ...
- Uva 10054 欧拉回路 打印路径
看是否有欧拉回路 有的话打印路径 欧拉回路存在的条件: 如果是有向图的话 1.底图必须是连通图 2.最多有两个点的入度不等于出度 且一个点的入度=出度+1 一个点的入度=出度-1 如果是无向图的话 1 ...
- Python解释器路径寻找规则
Python编辑器路径寻址总结 Python编程优化 这场表演邀请了三位角色:run.sh.main.py.path.sh,拍摄场地选在了 Windows -> Git Bash 群演1号 ru ...
- Fill-倒水问题(Uva-10603-隐式图路径寻找问题)
原题:https://uva.onlinejudge.org/external/106/10603.pdf 有三个没有刻度的杯子,它们的容量分别是a, b, c, 最初只有c中的杯子装满水,其他的被子 ...
- What Goes Up UVA - 481 LIS+打印路径 【模板】
打印严格上升子序列: #include<iostream> #include<cstdio> #include<algorithm> #include<cst ...
- 【DP】UVA 624 CD 记录路径
开一个数组p 若dp[i-1][j]<dp[i-1][j-a[i]]+a[i]时就记录下p[j]=a[i];表示此时放进一个轨道 递归输出p #include <stdio.h> # ...
- UVA 356 - Square Pegs And Round Holes
题目:在一个2n*2n的网格中间画一个直径为2n-1的圆,问圆内部的格子以及和圆相交的格子个数. 思路:只要考虑1 / 4圆的点就行,用点到原点距离与半径比较,当格子左下方和右上方都在格子里时,格子在 ...
随机推荐
- (二) MySQL常用命令及语法规范
- Python入门之字典的操作详解
这篇文章主要介绍了Python 字典(Dictionary)的详细操作方法,需要的朋友可以参考下: Python字典是另一种可变容器模型,且可存储任意类型对象,如字符串.数字.元组等其他容器模型. 一 ...
- Redis 如何保持和MySQL数据一致【二】
需求起因 在高并发的业务场景下,数据库大多数情况都是用户并发访问最薄弱的环节.所以,就需要使用redis做一个缓冲操作,让请求先访问到redis,而不是直接访问MySQL等数据库. 这个业务场景,主要 ...
- 01: RestfulAPI与HTTP
1.1 RestfulAPI与HTTP简介 1.什么是RestfulAPI 1.REST直接翻译:表现层状态转移,实质就是一种面向资源编程的方法 2.REST描述的是在网络中client和server ...
- 在ubuntu英文系统下使用中文输入法
How to install and use Chinese Input Method in the English Locale in Ubuntu ?(1) Check if there exis ...
- 20145105 《Java程序设计》实验二总结
实验二 Java面向对象程序设计 一. 实验内容: 初步掌握单元测试和TDD 理解并掌握面向对象三要素:封装.多态.建模 初步掌握UML 熟悉S.O.L.I.D原则 了解设计模式 二. 实验步骤 (一 ...
- 20165310_java_blog_week1.md
2165310 <Java程序设计>第1周学习总结 教材学习内容总结 了解Java基本配置环境 学习Java命名.编译.运行规则 拓展学习包的编译.运行 熟悉Git的运用方式 教材学习中的 ...
- 【前端】纯html+css+javascript实现楼层跳跃式的页面布局
实现效果演示: 实现代码及注释: <!DOCTYPE html> <html> <head> <title>楼层跳跃式的页面布局</title&g ...
- [BZOJ2963][JLOI2011]飞行路线 分层图+spfa
Description Alice和Bob现在要乘飞机旅行,他们选择了一家相对便宜的航空公司.该航空公司一共在n个城市设有业务,设这些城市分别标记为0到n-1,一共有m种航线,每种航线连接两个城市,并 ...
- Mac OSX 安装qemu
参考: Installing QEMU on OS X Homebrew Mac OSX 安装qemu 1.Install Homebrew: /usr/bin/ruby -e "$(cur ...