AtCoder Beginner Contest 086 D - Checker
Time limit : 2sec / Memory limit : 256MB
Score : 500 points
Problem Statement
AtCoDeer is thinking of painting an infinite two-dimensional grid in a checked pattern of side K. Here, a checked pattern of side K is a pattern where each square is painted black or white so that each connected component of each color is a K × K square. Below is an example of a checked pattern of side 3:
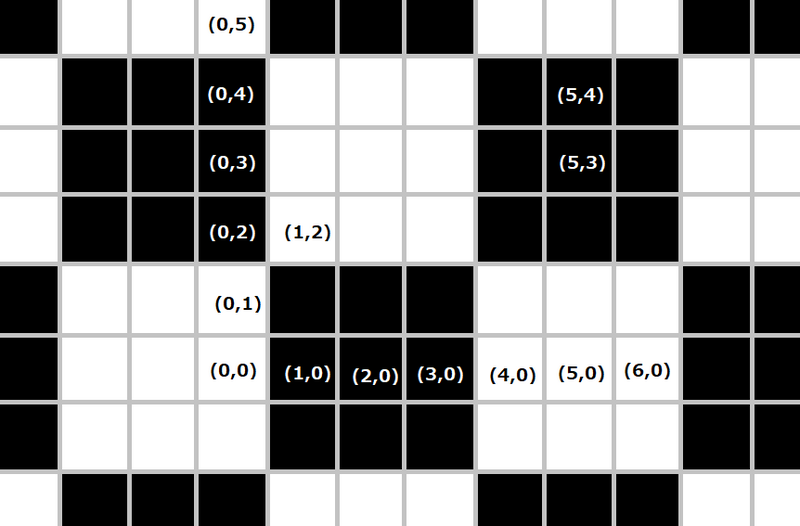
AtCoDeer has N desires. The i-th desire is represented by xi, yi and ci. If ci is B
, it means that he wants to paint the square (xi,yi) black; if ci is W
, he wants to paint the square (xi,yi) white. At most how many desires can he satisfy at the same time?
Constraints
- 1 ≤ N ≤ 105
- 1 ≤ K ≤ 1000
- 0 ≤ xi ≤ 109
- 0 ≤ yi ≤ 109
- If i ≠ j, then (xi,yi) ≠ (xj,yj).
- ci is
B
orW
. - N, K, xi and yi are integers.
Input
Input is given from Standard Input in the following format:
N K
x1 y1 c1
x2 y2 c2
:
xN yN cN
Output
Print the maximum number of desires that can be satisfied at the same time.
Sample Input 1
4 3
0 1 W
1 2 W
5 3 B
5 4 B
Sample Output 1
4
He can satisfy all his desires by painting as shown in the example above.
Sample Input 2
2 1000
0 0 B
0 1 W
Sample Output 2
2
Sample Input 3
6 2
1 2 B
2 1 W
2 2 B
1 0 B
0 6 W
4 5 W
Sample Output 3
4 这道题数据挺大的,首先是移动到2k*2k的框框里,不影响坐标(也就是横纵坐标都移动2k的整数倍,如果只移动k的整数倍不能保证颜色不变)。
最初先默认数黑色或者白色都可以,这里先默认数黑色,白色的通过变色移动(横或纵坐标+k)映射到黑色的位置,然后整个图里只剩下黑色的。
最后求出结果,只需要取sum 和 n - sum中大的那个就行了,因为默认是黑色,黑白可以互换。
然后是移动k宫格在不同位置,一共有k*k种情况,直接在2k*2k的框框里操作,需要计算二维前缀和,之前是一个一个点去判断超时了,想想也不能一味的暴力啊,肯定得用点妙招,然后移动x轴,计算一维前缀和时间勉强没超。
k宫格是从左下角开始移动就是从(0,0)开始,需要记录的是k宫格里黑色的个数,以及斜对角的矩形里黑色的个数,因为斜对角的颜色相同,横竖相邻的区域颜色相异。
计算二维前缀和需要用离散学的容斥定理,最后求单独区域需要前缀和相减,也要用到容斥定理。
代码:
#include <iostream>
#include <algorithm>
#include <cstdio>
#include <cstdlib>
#include <cstring>
#include <cmath>
#include <iomanip> using namespace std; int v[][];
int n,k;
int num(int x1,int y1,int x2,int y2){
return v[x2][y2] - v[x2][y1] - v[x1][y2] + v[x1][y1];///前缀和 减去左边 下边和左下边的k宫格前缀和 容斥定理:左边和下边都包含了左下边 需要加上一个左下边
}
int main()
{
int x,y,ans = 0,sum;
char ch;
scanf("%d%d",&n,&k);
for(int i = ;i <= n;i ++){
scanf("%d%d %c",&x,&y,&ch);
x %= * k;///把所有点都移动到 2k * 2k 的区域
y %= * k;
x += k * (ch == 'W');///x可以变成y W 也可以变成 B 这里白色都变成黑色的 如果转换后的黑色是满足的那么原来的白色也一定是满足的
v[x % ( * k) + ][y % ( * k) + ] ++;///求前缀和要求从(1,1)开始
}
for(int i = ;i <= * k;i ++)///求前缀和
for(int j = ;j <= * k;j ++)
v[i][j] += v[i - ][j] + v[i][j - ] - v[i - ][j - ];///加上左边下边和左下角的和 容斥定理:左边和下边的都包含了左下边的 要减去一个左下边的for(int i = ;i <= k;i++)///k * k个格子依次做为起点构成新的k宫格 也就是移动k宫格 看看有几个黑色点包含在内 (默认黑色 可互换)
for(int j = ;j <= k;j++)
{
///斜对角的k宫格是相同颜色 2k * 2k区域最多有五个这样的区域
sum = num(,,i,j) + num(i,j,k + i,k + j) + num(k + i,k + j, * k, * k) + num(k + i,, * k,j) + num(,k + j,i, * k);
ans = max(ans,max(sum,n - sum));///黑白色可以互换
}
printf("%d\n",ans);
}
AtCoder Beginner Contest 086 D - Checker的更多相关文章
- AtCoder Beginner Contest 086 (ABCD)
A - Product 题目链接:https://abc086.contest.atcoder.jp/tasks/abc086_a Time limit : 2sec / Memory limit : ...
- AtCoder Beginner Contest 100 2018/06/16
A - Happy Birthday! Time limit : 2sec / Memory limit : 1000MB Score: 100 points Problem Statement E8 ...
- AtCoder Beginner Contest 052
没看到Beginner,然后就做啊做,发现A,B太简单了...然后想想做完算了..没想到C卡了一下,然后还是做出来了.D的话瞎想了一下,然后感觉也没问题.假装all kill.2333 AtCoder ...
- AtCoder Beginner Contest 053 ABCD题
A - ABC/ARC Time limit : 2sec / Memory limit : 256MB Score : 100 points Problem Statement Smeke has ...
- AtCoder Beginner Contest 136
AtCoder Beginner Contest 136 题目链接 A - +-x 直接取\(max\)即可. Code #include <bits/stdc++.h> using na ...
- AtCoder Beginner Contest 137 F
AtCoder Beginner Contest 137 F 数论鬼题(虽然不算特别数论) 希望你在浏览这篇题解前已经知道了费马小定理 利用用费马小定理构造函数\(g(x)=(x-i)^{P-1}\) ...
- AtCoder Beginner Contest 076
A - Rating Goal Time limit : 2sec / Memory limit : 256MB Score : 100 points Problem Statement Takaha ...
- AtCoder Beginner Contest 079 D - Wall【Warshall Floyd algorithm】
AtCoder Beginner Contest 079 D - Wall Warshall Floyd 最短路....先枚举 k #include<iostream> #include& ...
- AtCoder Beginner Contest 064 D - Insertion
AtCoder Beginner Contest 064 D - Insertion Problem Statement You are given a string S of length N co ...
随机推荐
- imageView 的contentMode问题
UIViewContentModeScaleToFill : 图片拉伸至填充整个UIImageView(图片可能会变形) UIViewContentModeScaleAspectFit : 按照原来的 ...
- yii的安装
1.安装composer windows系统直接下载Composer-Setup.exe 运行安装. 2.安装Composer asset plugin composer安装完成后,在一个可通过web ...
- 关于*** WARNING L15: MULTIPLE CALL TO SEGMENT
编写51程序的时候,有时候会在主函数和中断函数里面调用同一个函数,如果正的出现这种情况,编译器会提出 这种警告: *** WARNING L15: MULTIPLE CALL TO SEGMENT(重 ...
- markdown公式编辑参考
原文作者,https://www.cnblogs.com/q735613050/p/7253073.html
- Supervisor安装与配置(Linux/Unix进程管理工具)
原文链接:http://blog.csdn.net/xyang81/article/details/51555473 Supervisor(http://supervisord.org/)是用Pyth ...
- POJ-1414 Life Line (暴力搜索)
Life Line Time Limit: 1000MS Memory Limit: 10000K Total Submissions: 855 Accepted: 623 Description L ...
- FZU2110 Star【计算几何】
Overpower often go to the playground with classmates. They play and chat on the playground. One day, ...
- opengl学习笔记(三):经过纹理贴图的棋盘
opengl纹理贴图的步骤: 1:创建纹理对象,并为它指定一个纹理 2:确定纹理如何应用到每个像素上 3:启用纹理贴图功能 4:绘制场景,提供纹理坐标和几何图形坐标 注意:纹理坐标必须在RGBA模式下 ...
- Lost connection to MySQL server during query ([Errno 104] Connection reset by peer)
Can't connect to MySQL server Lost connection to MySQL server during query · Issue #269 · PyMySQL/Py ...
- mybatis-3 cache 源码赏析
总结: 从缓存策略源码,可以分析java相关类库 mybatis-3/src/main/java/org/apache/ibatis/cache/decorators/SoftCache.java p ...