poj3126Prime Path (BFS+素数筛)
素数筛:需要一个数组进行标记
最小的素数2,所有是2的倍数的数都是合数,对合数进行标记,然后找大于2的第一个非标记的数(肯定是素数),将其倍数进行标记,如此反复,若是找n以内的所有素数,只需要对[2,n^0.5]进行循环即可,因为n以内的所有数如果不是[2,n^0.5]的倍数,则一定是素数。复杂度:O(nloglogn);
for(int i=2;i*i<=n;i++){
if(a[i]!=-1)
for(int k=i*i;k<=n;k+=i)
a[i]=-1;
}
poj3126题目链接:http://poj.org/problem?id=3126
素数筛所占用的空间较大,毕竟是需要数组进行标记的,如果n太大就不可以做出(内存超出)。
若是让求[a,b]内的素数,a<b<=1e12,b-a<=1e6; 这个如果直接用素数筛是内存超出,可以先用一个数组存储[0,b^0.5]的素数,再用这些素数筛选[a,b],注意令一个数组表示[a,b]的素数,不能让下标直接表示这个数字(>=a&&<=b),而是开一个数组,c[i]表示a+i是否是素数。
Description
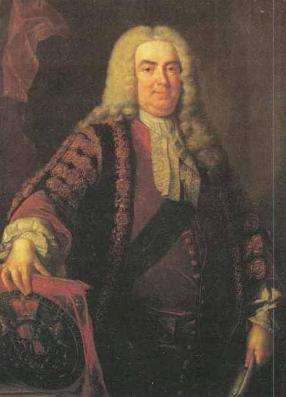
— It is a matter of security to change such things every now and then, to keep the enemy in the dark.
— But look, I have chosen my number 1033 for good reasons. I am the Prime minister, you know!
— I know, so therefore your new number 8179 is also a prime. You will just have to paste four new digits over the four old ones on your office door.
— No, it’s not that simple. Suppose that I change the first digit to an 8, then the number will read 8033 which is not a prime!
— I see, being the prime minister you cannot stand having a non-prime number on your door even for a few seconds.
— Correct! So I must invent a scheme for going from 1033 to 8179 by a path of prime numbers where only one digit is changed from one prime to the next prime.
Now, the minister of finance, who had been eavesdropping, intervened.
— No unnecessary expenditure, please! I happen to know that the price of a digit is one pound.
— Hmm, in that case I need a computer program to minimize the cost. You don't know some very cheap software gurus, do you?
— In fact, I do. You see, there is this programming contest going on... Help the prime minister to find the cheapest prime path between any two given four-digit primes! The first digit must be nonzero, of course. Here is a solution in the case above.
1033
1733
3733
3739
3779
8779
8179
The cost of this solution is 6 pounds. Note that the digit 1 which got pasted over in step 2 can not be reused in the last step – a new 1 must be purchased.
Input
Output
Sample Input
3
1033 8179
1373 8017
1033 1033
Sample Output
6
7
0 现附上AC代码:
#include<iostream>
#include<cstdio>
#include<queue>
#include<cmath>
using namespace std;
const int maxn=1e4+5;
int main(){
int T,num[4],temp;cin>>T;
int plu[maxn];
while(T--){
for(int i=0;i<=maxn;i++)
plu[i]=0;
for(int i=2;i*i<=maxn;i++){
for(int j=i*i;j<=maxn;j+=i){
plu[j]=-1;
}
}
int x,y;scanf("%d%d",&x,&y);
queue<int >q;
q.push(x);
plu[x]=1;//注意这一步的重要性,没有这一步的话,可能会导致 plu[temp]=plu[t]+1;当plu[t]==0时就执行了,
while(!q.empty()&&plu[y]==0){
int m=q.front(),t=q.front(); q.pop();
for(int i=3;i>=0;i--)
num[i]=m%10,m/=10;
for(int j=0;j<4;j++){
int mm=num[j],i;
if(j==0) i=1;
else i=0;
for(;i<=9;i++){
num[j]=i;
temp=num[0]*1000+num[1]*100+num[2]*10+num[3];
if(plu[temp]==0) {
plu[temp]=plu[t]+1;
q.push(temp);
}
}
num[j]=mm;
}
}
// printf("%d %d %d %d %d %d %d\n",plu[1033],plu[1733],plu[3733],plu[3739],plu[3779],plu[8779],plu[8179]);
if(plu[y]==-1) printf("Impossible\n");
else printf("%d\n",plu[y]-1);
}
return 0;
}
这道题是要求最小步骤到达目标,就是一个变形的最短路问题。对于最短路问题,肯定是首选BFS,而我们首先对x进行,个位十位百位千位的遍历,若新生成的数是素数(且之前没有访问过,毕竟是求最短路,若之前已经到过,则再到肯定不是最短,便剪枝),则将其放进队列。最后得出plu[y]基本就可以知道答案了。
回过头来一想,发现的确是很简单,但之前却wrong answer了很多次,自己都对自己无语了。
poj3126Prime Path (BFS+素数筛)的更多相关文章
- POJ 3126 Prime Path (BFS + 素数筛)
链接 : Here! 思路 : 素数表 + BFS, 对于每个数字来说, 有四个替换位置, 每个替换位置有10种方案(对于最高位只有9种), 因此直接用 BFS 搜索目标状态即可. 搜索的空间也不大. ...
- POJ——3126Prime Path(双向BFS+素数筛打表)
Prime Path Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 16272 Accepted: 9195 Descr ...
- POJ3126 Prime Path (bfs+素数判断)
POJ3126 Prime Path 一开始想通过终点值双向查找,从最高位开始依次递减或递增,每次找到最接近终点值的素数,后来发现这样找,即使找到,也可能不是最短路径, 而且代码实现起来特别麻烦,后来 ...
- poj 3126 Prime Path( bfs + 素数)
题目:http://poj.org/problem?id=3126 题意:给定两个四位数,求从前一个数变到后一个数最少需要几步,改变的原则是每次只能改变某一位上的一个数,而且每次改变得到的必须是一个素 ...
- POJ3126Prime Path(BFS)
#include"cstdio" #include"queue" #include"cstring" using namespace std ...
- POJ 3126 Prime Path (bfs+欧拉线性素数筛)
Description The ministers of the cabinet were quite upset by the message from the Chief of Security ...
- Prime Path素数筛与BFS动态规划
埃拉托斯特尼筛法(sieve of Eratosthenes ) 是古希腊数学家埃拉托斯特尼发明的计算素数的方法.对于求解不大于n的所有素数,我们先找出sqrt(n)内的所有素数p1到pk,其中k = ...
- poj3126--Prime Path(广搜)
Prime Path Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 11751 Accepted: 6673 Descr ...
- POJ 3126 Prime Path(素数路径)
POJ 3126 Prime Path(素数路径) Time Limit: 1000MS Memory Limit: 65536K Description - 题目描述 The minister ...
随机推荐
- [集合Set]HashSet、LinkedHashSet TreeSet
Set Set是不包含重复元素的集合.更正式地,集合不包含一对元素e1和e2,使得e1.equals(e2),并且最多一个空元素. 无索引,不可以重复,无序(存取不一致) Set接口除了继承自Coll ...
- 初学css list-style属性
网上很多css布局中会看到这样的一句:list-style:none: 那么list-style到底什么意思?中文即:列表样式:无: 其实它是一个简写属性,包含了所有列表属性,具体包含list-sty ...
- Codeforces - 1199C - MP3 - 尺取
https://codeforc.es/contest/1199/problem/C 擦,最后移位运算符溢出了,真的蠢. 肯定是选中间的连续的某段是最优的,维护这个段的长度和其中的元素种类就可以了.小 ...
- 实现类似add(1)(2)(3)结果为6的效果
前两天看到一个问题说怎样实现add方法实现add(1)(2)(3)结果为6,于是开始引发了我的思考. 1.想要实现add()()这样调用方式,add()方法的返回值务必是一个函数 function a ...
- ZeroAccess分析
来源:http://bbs.pediy.com/showthread.php?t=141124&highlight=ZeroAccess 总序这分成四个部分的系列文章,是一个完全的一步一步来分 ...
- Ionic创建混合App(一)
前言 最近公司要开始做App项目,最终选定了ionic开发方案,在这里我将学习的过程记录在这里,一方面避免自己忘记,另一方面方便大家交流学习.这里我们采用的是 Ionic2 + Angular2 : ...
- redis-Nosql
Nosql: CAP:C(Consistency):强一致性.A(Availability):可用性.P(Partitio Tolerance):分区容错性 CAP 理论的核心是: 一个分布式系统,不 ...
- vue中的scope
在vue文件中的style标签上,有一个特殊的属性:scoped. 当一个style标签拥有scoped属性时,它的CSS样式就只能作用于当前的组件,也就是说,该样式只能适用于当前组件元素. 通过该属 ...
- linux强制用户下线命令
linux强制用户下线命令 前提:必须是root权限操作:(1)使用who查看目前有哪些用户登录了服务器,见下图(2)使用pkill -kill -t pts/1命令踢出第一个用户.命令解释:pt ...
- 学Python的第四天
第四天啦,今天依旧代码少的啃树皮.... 但是作业留的有了幼儿园的水准!!!让小编我看到了希望.... #!/usr/bin/env python # -*- coding:utf8 -*- impo ...