springboot2.x实现oauth2授权码登陆
一 进行授权页
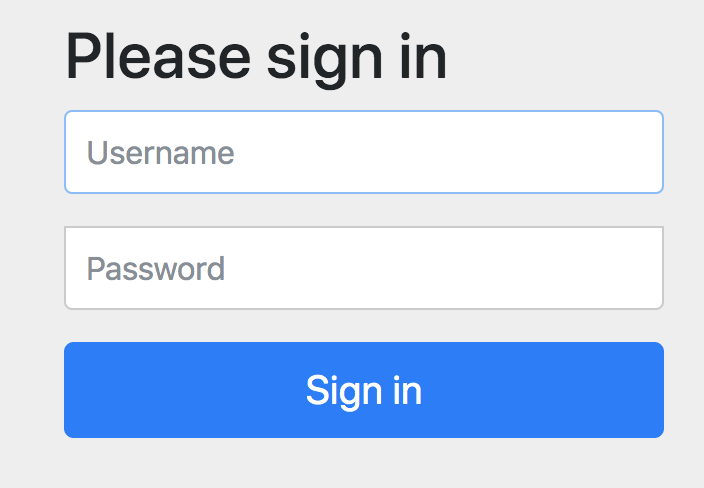
三 授权资源类型
@Override
public void configure(ClientDetailsServiceConfigurer clients) throws Exception {
clients.inMemory()
.withClient(ClientID)
.secret(passwordEncoder.encode(ClientSecret))
.authorizedGrantTypes("authorization_code", "refresh_token",
"password", "implicit")
.scopes("read","write","del","userinfo")
.redirectUris(RedirectURLs);
}
四 接到code
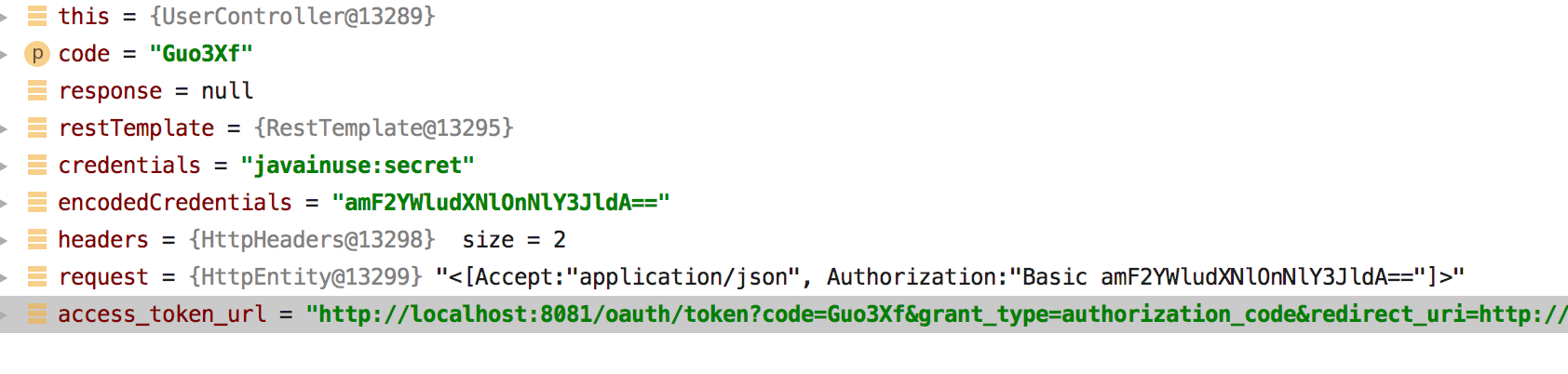
五 获取access_token
@Override
public void configure(AuthorizationServerSecurityConfigurer oauthServer) throws Exception {
oauthServer.tokenKeyAccess("isAuthenticated()")
.checkTokenAccess("permitAll()")
.allowFormAuthenticationForClients();//支持把secret和clientid写在url上,否则需要在头上
}
Authorization Ccode------RFRLFY
access_token_url http://localhost:8081/oauth/token?client_id=android1&code=RFRLFY&grant_type=authorization_code&redirect_uri=http://localhost:8081/callback&client_secret=android1
Access Token Response ---------{"access_token":"faadf3bf-6488-4036-bc3b-21b0a979602c","token_type":"bearer","refresh_token":"1b01f133-c5ab-419f-8125-088c85916ecb","expires_in":,"scope":"read"}
回调页面代码,主要实现了对code的获取,对access_token的组织,然后请求时把access_token带上,这个方法一般会做成公用的过滤器
@Controller
public class UserController {
@RequestMapping(value = "/callback", method = RequestMethod.GET)
public ResponseEntity<String> callback(@RequestParam("code") String code) throws JsonProcessingException, IOException {
ResponseEntity<String> response = null;
System.out.println("Authorization Ccode------" + code);
RestTemplate restTemplate = new RestTemplate();
String access_token_url = "http://localhost:8081/oauth/token";
access_token_url += "?client_id=android1&code=" + code;
access_token_url += "&grant_type=authorization_code";
access_token_url += "&redirect_uri=http://localhost:8081/callback";
access_token_url += "&client_secret=android1";
System.out.println("access_token_url " + access_token_url);
response = restTemplate.exchange(access_token_url, HttpMethod.POST, null, String.class);
ObjectMapper mapper = new ObjectMapper();
JsonNode node = mapper.readTree(response.getBody());
String token = node.path("access_token").asText(); System.out.println("access_token" +access_token);
String url = "http://localhost:8081/index"; HttpHeaders headers1 = new HttpHeaders(); headers1.add("Authorization", "Bearer " + token); HttpEntity<String> entity = new HttpEntity<>(headers1); ResponseEntity<String> result = restTemplate.exchange(url, HttpMethod.GET, entity, String.class); return result; }
六 拿着access_token去请求具体的资源
@Configuration
@EnableResourceServer
@Order(6)
public class ResourceServerConfig extends ResourceServerConfigurerAdapter {
@Override
public void configure(HttpSecurity http) throws Exception {
http.csrf().disable()//禁用了 csrf 功能
.authorizeRequests()//限定签名成功的请求
.antMatchers("/index").access("#oauth2.hasScope('del')") //授权码scopes里需要选中del才可以访问
.antMatchers("/user").authenticated()//签名成功后可访问,不受role限制
.anyRequest().permitAll()//其他没有限定的请求,允许访问
.and().anonymous()//对于没有配置权限的其他请求允许匿名访问
.and().formLogin()//使用 spring security 默认登录页面
.and().httpBasic();//启用http 基础验证 }
}
八 需要注意的地方
如果你对用户进行了角色和权限的配置,对于某些保护接口需要有指定权限才能访问的话,需要重getAuthorities方法,否则,你的权限将会失效!
@Entity
@Data
public class User extends BaseEntity implements UserDetails {
@Id
@GeneratedValue
private Long id;
private String username;
private String password;
private String firstName;
private String lastName;
private String email;
private String imageUrl; @JsonIgnore
@ManyToMany(targetEntity = Role.class, fetch = FetchType.EAGER)
@BatchSize(size = 20)
private Set<Role> roles = new HashSet<>(); @Transient
private Set<GrantedAuthority> authorities = new HashSet<>(); /**
* 注意,这块需要加@Override重写,否则权限无效.
*
* @return
*/
@Override
public Set<GrantedAuthority> getAuthorities() {
Set<GrantedAuthority> authorities = new HashSet<>();
for (Role role : this.roles) {
for (Authority authority : role.getAuthorities()) {
authorities.add(new SimpleGrantedAuthority(authority.getValue()));
}
}
return authorities;
} @Override
public boolean isAccountNonExpired() {
return true;
} @Override
public boolean isAccountNonLocked() {
return true;
} @Override
public boolean isCredentialsNonExpired() {
return true;
} @Override
public boolean isEnabled() {
return true;
}
}
感谢阅读!
springboot2.x实现oauth2授权码登陆的更多相关文章
- 13.详解oauth2授权码流程
13.详解oauth2授权码流程 把登陆系统单独独立出来,可以给自己写的微服务用,也可以给第三方的系统调用我们的服务 显式的和隐式的,两种方式,
- 阶段5 3.微服务项目【学成在线】_day16 Spring Security Oauth2_06-SpringSecurityOauth2研究-Oauth2授权码模式-申请令牌
3.3 Oauth2授权码模式 3.3.1 Oauth2授权模式 Oauth2有以下授权模式: 授权码模式(Authorization Code) 隐式授权模式(Implicit) 密码模式(Reso ...
- Spring Security OAuth2 授权码模式
背景: 由于业务实现中涉及到接入第三方系统(app接入有赞商城等),所以涉及到第三方系统需要获取用户信息(用户手机号.姓名等),为了保证用户信息的安全和接入方式的统一, 采用Oauth2四种模式之一 ...
- 微信授权就是这个原理,Spring Cloud OAuth2 授权码模式
上一篇文章Spring Cloud OAuth2 实现单点登录介绍了使用 password 模式进行身份认证和单点登录.本篇介绍 Spring Cloud OAuth2 的另外一种授权模式-授权码模式 ...
- 阶段5 3.微服务项目【学成在线】_day16 Spring Security Oauth2_07-SpringSecurityOauth2研究-Oauth2授权码模式-资源服务授权测试
下面要完成 5.6两个步骤 3.3.4 资源服务授权 3.3.4.1 资源服务授权流程 资源服务拥有要访问的受保护资源,客户端携带令牌访问资源服务,如果令牌合法则可成功访问资源服务中的资 源,如下图 ...
- asp.net利用QQ邮箱发送邮件,关键在于开启pop并设置授权码为发送密码
public static bool SendEmail(string mailTo, string mailSubject, string mailContent) { ...
- oAuth2授权协议 & 微信授权登陆和绑定 & 多环境共用一个微信开发平台回调设置
OAuth2(open Auth)开放授权协议 授权码模式流程: 1.浏览器(客户端)点击一个比如使用微信登陆按钮 2.会跳到认证服务器页面,让用户选择是否授权 3.如果用户点击授权,那么会跳转到开始 ...
- Spring Cloud2.0之Oauth2环境搭建(授权码模式和密码授权模式)
oauth2 server 微服务授权中心, github源码 https://github.com/spring-cloud/spring-cloud-security 对微服务接口做一些权 ...
- Oauth2.0认证---授权码模式
目录: 1.功能描述 2.客户端的授权模式 3.授权模式认证流程 4.代码实现 1.功能描述 OAuth在"客户端"与"服务提供商"之间,设置了一个授权层(au ...
随机推荐
- Python面试的一些心得,与Python练习题分享【华为云技术分享】
版权声明:本文为CSDN博主「华为云」的原创文章,遵循CC 4.0 BY-SA版权协议,转载请附上原文出处链接及本声明.原文链接:https://blog.csdn.net/devcloud/arti ...
- 挑战10个最难的Java面试题(附答案)【上】【华为云技术分享】
版权声明:本文为博主原创文章,遵循 CC 4.0 BY-SA 版权协议,转载请附上原文出处链接和本声明.本文链接:https://blog.csdn.net/devcloud/article/deta ...
- 华为担纲建设基础软硬件国家新一代AI开放创新平台
[摘要] 全栈全场景AI能力爆发! [上海,2019年8月29日] 凭借领先的全栈全场景AI能力华为入选国家新一代人工智能开放创新平台 8月29日,科技部在2019世界人工智能大会宣布,将依托华为建设 ...
- vue axios 总结篇
1.npm --save 和 --save-dev 有什么区别 发布到线上的叫生产环境~,在本地开发的时候叫开发环境,--save就是会打包到线上去并且在线上环境能用到的,比如你npm install ...
- syslog日志
Syslog协议 系统日志(Syslog)协议是在一个IP网络中转发系统日志信息的标准,它是在美国加州大学伯克利软件分布研究中心(BSD)的TCP/IP系统实施中开发的,目前已成为工业标准协议,可用它 ...
- 使用SQL计算宝宝每次吃奶的时间间隔
需求:媳妇儿最近担心宝宝的吃奶时间不够规律,网上说是正常平均3小时喂奶一次,让我记录下每次的吃奶时间,分析下实际是否偏差很大,好在下次去医院复查时反馈给医生. 此外,还要注意有时候哭闹要吃奶,而实际只 ...
- Nginx(二)--nginx的核心功能
反向代理 nginx反向代理的指令不需要新增额外的模块,默认自带proxy_pass指令,只需要修改配置文件就可以实现反向代理. proxy_pass 既可以是ip地址,也可以是域名,同时还可以指定端 ...
- 1.使用大clob入库出错问题
在代码中调用pstm.setString(str) str>4000,这种大字符串插入时出现字符过长插入报错问题. 解决问题:用声明变量方式: <insert id ="inse ...
- 【spring boot】配置信息
======================================================================== 1.feign 超时配置 2.上传文件大小控制 3.J ...
- Linux之CentOS设置别名与屏蔽别名
一.环境 CentOS6.8 二.设置别名 ◆别名功能:让grep符合的关键字高亮 1.临时生效 [root@localhost ~]#alias grep="grep --color=au ...