HDU 3221 Brute-force Algorithm
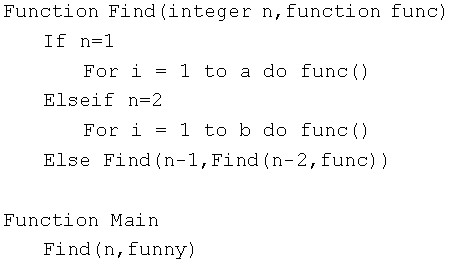

#include<cstdio>
#include<cstring>
using namespace std;
typedef long long ll;
const int maxn=1e6+;
int euler[maxn]; ll a,b,m,n; int phi()
{
for(int i=;i<maxn;i++) euler[i]=i;
for(int i=;i<maxn;i++)
{
if(euler[i]==i)
{
for(int j=i;j<maxn;j+=i)
{
euler[j]=euler[j]/i*(i-);
}
}
}
} struct Matrix
{
ll a[][];
Matrix(){memset(a,,sizeof(a));}
Matrix operator* (const Matrix &p)
{
Matrix res;
for(int i=;i<;i++)
{
for(int j=;j<;j++)
{
for(int k=;k<;k++)
{
res.a[i][j]+=a[i][k]*p.a[k][j];
if(res.a[i][j]>euler[m])//特别要注意这个条件
{
res.a[i][j]=res.a[i][j]%euler[m]+euler[m];
}
}
}
}
return res;
}
}ans,base; Matrix quick_pow(Matrix base,ll n)
{
Matrix res;
for(int i=;i<;i++)
{
res.a[i][i]=;
}
while(n)
{
if(n&) res=res*base;
base=base*base;
n>>=;
}
return res;
} ll pow(ll a,ll n)
{
ll ans=;
while(n)
{
if(n&) ans=ans*a%m;
a=a*a%m;
n>>=;
}
return ans;
} void Matrix_init()
{
ans.a[][]=;
ans.a[][]=;
ans.a[][]=;
ans.a[][]=;
base.a[][]=;
base.a[][]=;
base.a[][]=;
base.a[][]=;
} int main()
{
int t,cas=;
ll ansa,ansb,faca,facb;
phi();
scanf("%d",&t);
while(t--)
{
cas++;
scanf("%lld%lld%lld%lld",&a,&b,&m,&n);
printf("Case #%d: ",cas);
if(n==) printf("%lld\n",a%m);
else if(n==) printf("%lld\n",b%m);
else if(n==) printf("%lld\n",a*b%m);
else if(m==) printf("0\n");
else
{
Matrix_init();
ans=ans*quick_pow(base,n-);
faca=ans.a[][];
facb=ans.a[][];
ansa=pow(a,faca)%m;
ansb=pow(b,facb)%m;
printf("%lld\n",ansa*ansb%m);
}
}
return ;
}
HDU 3221 Brute-force Algorithm的更多相关文章
- HDU 6215 Brute Force Sorting(模拟链表 思维)
Brute Force Sorting Time Limit: 1000/1000 MS (Java/Others) Memory Limit: 65535/32768 K (Java/Othe ...
- HDU 6215 Brute Force Sorting(链表)
http://acm.hdu.edu.cn/showproblem.php?pid=6215 题意:给出一个序列,对于每个数,它必须大于等于它前一个数,小于等于后一个数,如果不满足,就删去.然后继续去 ...
- hdu 6215 -- Brute Force Sorting(双向链表+队列)
题目链接 Problem Description Beerus needs to sort an array of N integers. Algorithms are not Beerus's st ...
- hdu 6215 Brute Force Sorting(模拟)
题目链接:http://acm.hdu.edu.cn/showproblem.php?pid=6215 题解:类似双链表的模拟. #include <iostream> #include ...
- HDU 6215 Brute Force Sorting 模拟双端链表
一层一层删 链表模拟 最开始写的是一个一个删的 WA #include <bits/stdc++.h> #define PI acos(-1.0) #define mem(a,b) mem ...
- HDU - 6215 2017 ACM/ICPC Asia Regional Qingdao Online J - Brute Force Sorting
Brute Force Sorting Time Limit: 1 Sec Memory Limit: 128 MB 题目连接 http://acm.hdu.edu.cn/showproblem.p ...
- HDU 4971 A simple brute force problem.
A simple brute force problem. Time Limit: 1000ms Memory Limit: 65536KB This problem will be judged o ...
- SRM 582 Div II Level Three: ColorTheCells, Brute Force 算法
题目来源:http://community.topcoder.com/stat?c=problem_statement&pm=12581 Burte Force 算法,求解了所有了情况,注意 ...
- Test SRM Level Three: LargestCircle, Brute Force
题目来源:http://community.topcoder.com/stat?c=problem_statement&pm=3005&rd=5858 思路: 如果直接用Brute F ...
- hdu6215 Brute Force Sorting
地址:http://acm.split.hdu.edu.cn/showproblem.php?pid=6215 题目: Brute Force Sorting Time Limit: 1000/100 ...
随机推荐
- github for window的代理设置方法
修改 .gitconfig 文件,主要是针对http 和 https进行修改,设置代理 [user] name = name email = mail@.com [http] proxy = 配置文件 ...
- "mkimage" command not found - U-Boot images will not be built
编译内核的时候出现错误:"mkimage" command not found - U-Boot images will not be built 参考链接 http://blog ...
- Python开发程序:选课系统-改良版
程序名称: 选课系统 角色:学校.学员.课程.讲师要求:1. 创建北京.上海 2 所学校2. 创建linux , python , go 3个课程 , linux\py 在北京开, go 在上海开3. ...
- MyBatis操作指南-与Spring集成(基于注解)
- 《奥威Power-BI案例应用:带着漫画看报告》腾讯课程开课啦
元旦小假期过去了,不管是每天只给自己两次下床机会的你,还是唱K看电影逛街样样都嗨的你,是时候重振旗鼓,重新上路了!毕竟为了不给国家的平均工资水平拖后腿,还是要努力工作的.话说2016年已经过去了,什么 ...
- R12.2 URL Validation failed. The error could have been caused through the use of the browser's navigation buttons
EBS升级到R12.2.4后,进入系统操作老是报以下错误: 通过谷歌发现有人遇到相同的问题,并提供了解决方案. 原文地址:http://onlineappsdbaoracle.blogspot.com ...
- 开发Windows Phone应用程序之后的感觉
刚刚历时一个多月完成了酒美网(我之前的公司)Windows Phone版客户端,发现自己的自学能力还可以,但是还是有好多东西摸不清,到今天我才刚刚对MVVM入门,更对MVVMLight这个框架有进一步 ...
- Linux启用和配置Java
Firefox 在安装 Java 平台时,Java 插件文件将作为该安装的一部分包含在内.要在 Firefox 中使用 Java,您需要从该发行版中的插件文件手动创建符号链接指向 Firefox 预期 ...
- 夺命雷公狗----Git---1---安装步骤
除了上面的路径修改一下,别的都用默认的问题即可解决.....
- 【Lua学习笔记之:Lua环境搭建 Windows 不用 visual studio】
Lua 环境搭建 Windows 不用 visual studio 系统环境:Win7 64bit 联系方式:yexiaopeng1992@126.com 前言: 最近需要学习Unity3d游戏中的热 ...