poj 2299(离散化+树状数组)
Time Limit: 7000MS | Memory Limit: 65536K | |
Total Submissions: 53777 | Accepted: 19766 |
Description
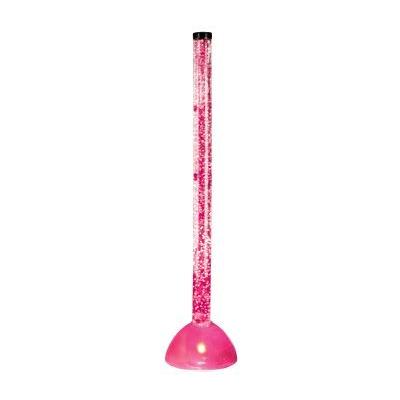
9 1 0 5 4 ,
Ultra-QuickSort produces the output
0 1 4 5 9 .
Your task is to determine how many swap operations Ultra-QuickSort needs to perform in order to sort a given input sequence.
Input
input contains several test cases. Every test case begins with a line
that contains a single integer n < 500,000 -- the length of the input
sequence. Each of the the following n lines contains a single integer 0
≤ a[i] ≤ 999,999,999, the i-th input sequence element. Input is
terminated by a sequence of length n = 0. This sequence must not be
processed.
Output
every input sequence, your program prints a single line containing an
integer number op, the minimum number of swap operations necessary to
sort the given input sequence.
Sample Input
5
9
1
0
5
4
3
1
2
3
0
Sample Output
6
0
Source
题意:求解一个串的逆序数的个数是多少??
题解:离散化数组变成下标,然后每次将离散化的下标放进树状数组,放进去之后统计小于他的数的个数是多少。用 i - getsum(a[i])即为大于它的数的个数,其中 i 为当前已经插入的数的个数。
#include <iostream>
#include <stdio.h>
#include <string.h>
#include <stack>
#include <vector>
#include <algorithm>
using namespace std;
const int N = ; struct Node{
int v,id;
}node[N];
int a[N],c[N],n;
int lowbit(int x){
return x&(-x);
}
void update(int idx,int v){
for(int i=idx;i<=N;i+=lowbit(i)){
c[i]+=v;
}
}
int getsum(int idx){
int sum = ;
for(int i=idx;i>=;i-=lowbit(i)){
sum+=c[i];
}
return sum;
}
int cmp(Node a,Node b){
return a.v<b.v;
}
int main()
{
while(scanf("%d",&n)!=EOF,n){
memset(c,,sizeof(c));
for(int i=;i<=n;i++){
scanf("%d",&node[i].v);
node[i].id = i;
}
sort(node+,node+n+,cmp);
for(int i=;i<=n;i++){
a[node[i].id] = i;
}
long long cnt = ;
for(int i=;i<=n;i++){
update(a[i],);
cnt=cnt+ i - getsum(a[i]);
}
printf("%lld\n",cnt);
}
return ;
}
poj 2299(离散化+树状数组)的更多相关文章
- POJ 2299 【树状数组 离散化】
题目链接:POJ 2299 Ultra-QuickSort Description In this problem, you have to analyze a particular sorting ...
- POJ 2299 Ultra-QuickSort (树状数组 && 离散化&&逆序)
题意 : 给出一个数n(n<500,000), 再给出n个数的序列 a1.a2.....an每一个ai的范围是 0~999,999,999 要求出当通过相邻两项交换的方法进行升序排序时需要交换 ...
- POJ 2299 Ultra-QuickSort (树状数组 && 离散化)
题意 : 给出一个数n(n<500,000), 再给出n个数的序列 a1.a2.....an每一个ai的范围是 0~999,999,999 要求出当通过相邻两项交换的方法进行升序排序时需要交换 ...
- POJ 2299 Ultra-QuickSort (树状数组+离散化 求逆序数)
In this problem, you have to analyze a particular sorting algorithm. The algorithm processes a seque ...
- CodeForces 540E - Infinite Inversions(离散化+树状数组)
花了近5个小时,改的乱七八糟,终于A了. 一个无限数列,1,2,3,4,...,n....,给n个数对<i,j>把数列的i,j两个元素做交换.求交换后数列的逆序对数. 很容易想到离散化+树 ...
- Ultra-QuickSort(归并排序+离散化树状数组)
Ultra-QuickSort Time Limit: 7000MS Memory Limit: 65536K Total Submissions: 50517 Accepted: 18534 ...
- HDU 5862 Counting Intersections(离散化+树状数组)
HDU 5862 Counting Intersections(离散化+树状数组) 题目链接http://acm.split.hdu.edu.cn/showproblem.php?pid=5862 D ...
- BZOJ_4627_[BeiJing2016]回转寿司_离散化+树状数组
BZOJ_4627_[BeiJing2016]回转寿司_离散化+树状数组 Description 酷爱日料的小Z经常光顾学校东门外的回转寿司店.在这里,一盘盘寿司通过传送带依次呈现在小Z眼前.不同的寿 ...
- poj-----Ultra-QuickSort(离散化+树状数组)
Ultra-QuickSort Time Limit: 7000MS Memory Limit: 65536K Total Submissions: 38258 Accepted: 13784 ...
随机推荐
- JZOJ 5777. 【NOIP2008模拟】小x玩游戏
5777. [NOIP2008模拟]小x玩游戏 (File IO): input:game.in output:game.out Time Limits: 1000 ms Memory Limits ...
- pandas知识点(汇总和计算描述统计)
调用DataFrame的sum方法会返还一个含有列的Series: In [5]: df = DataFrame([[1.4,np.nan],[7.1,-4.5],[np.nan,np.nan],[0 ...
- (新手)使用pandas操作EXCEL
import pandas as pdimport numpy as npfrom pandas import DataFrame,Series#path = r'C:\Users\tsl\Deskt ...
- POJ 3414 BFS 输出过程
Pots Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 17456 Accepted: 7407 Special J ...
- js:随记
typeof:没有大写,因为typeof是运算符 *1:是转数字 +string:是转数字,在Date对象上是getTime ""+:是转字符串 "":bool ...
- 4 Template层- HTML转义
1.HTML转义 Django对字符串进行自动HTML转义,如在模板中输出如下值: 视图代码: def index(request): return render(request, 'temtest/ ...
- day23 Model 操作,Form 验证以及序列化操作
Model 操作 1创建数据库表 定制表名: 普通索引: 创建两个普通索引,这样就会生成两个索引文件 联合索引: 为了只生成一个索引文件,才 ...
- Python+Selenium基础篇之5-第一个完整的自动化测试脚本
前面文章,我们介绍了如何采用XPath表达式去定位网页元素,在掌握了如何抓取或者如何书写精确的XPath表达式后,我们可以开始写自己的第一个真正意义上的webui 自动化测试脚本,就相当于,你在学习P ...
- python 学习分享-面向对象2
面向对象进阶 静态方法 一种普通函数,就位于类定义的命名空间中,它不会对任何实例类型进行操作.使用装饰器@staticmethod定义静态方法.类对象和实例都可以调用静态方法: class Foo: ...
- vlc无法播放.flv视频文件
解决方法:https://videoconverter.wondershare.com/vlc/flv-not-displaying-video-vlc-media-player.html. 在pre ...