POJ 3150 Cellular Automaton(矩阵高速幂)
题目大意:给定n(1<=n<=500)个数字和一个数字m,这n个数字组成一个环(a0,a1.....an-1)。假设对ai进行一次d-step操作,那么ai的值变为与ai的距离小于d的全部数字之和模m。求对此环进行K次d-step(K<=10000000)后这个环的数字会变为多少。
看了一篇博客:http://www.cppblog.com/varg-vikernes/archive/2011/02/08/139804.html说的非常清楚。
拿例子来说:
a矩阵:
a = 1 2 2 1 2
构造出来一个矩阵:
b =
1 1 0 0 1
1 1 1 0 0
0 1 1 1 0
0 0 1 1 1
1 0 0 1 1
(a4+a0+a1) (a0+a1+a2) (a1+a2+a3) (a2+a3+a4) (a3+a4+a0)得到的矩阵e = 5 5 5 5 4
所以题意就是:a*(b^k) = e。求出e就是通过解b^k。由于直接求b^k会超时,所以要二分求幂。
这个题的矩阵另一个性质:
利用矩阵A。B具有A[i][j]=A[i-1][j-1],B[i][j]=B[i-1][j-1](i-1<0则表示i-1+n,j-1<0则表示j-1+n)
我们能够得出矩阵C=a*b也具有这个性质
C[i][j]=sum(A[i][t]*B[t][j])=sum(A[i-1][t-1],B[t-1][j-1])=sum(A[i-1][t],B[t][j-1])=C[i-1][j-1]
“”“
所以能够求出C[0][0],以后的递推得到。
Time Limit: 12000MS | Memory Limit: 65536K | |
Total Submissions: 3057 | Accepted: 1232 | |
Case Time Limit: 2000MS |
Description
A cellular automaton is a collection of cells on a grid of specified shape that evolves through a number of discrete time steps according to a set of rules that describe the new state of a cell based on the states of neighboring cells. The order
of the cellular automaton is the number of cells it contains. Cells of the automaton of order n are numbered from 1 to n.
The order of the cell is the number of different values it may contain. Usually, values of a cell of order m are considered to be integer numbers from 0 to m − 1.
One of the most fundamental properties of a cellular automaton is the type of grid on which it is computed. In this problem we examine the special kind of cellular automaton — circular cellular automaton of order n with cells of order m.
We will denote such kind of cellular automaton as n,m-automaton.
A distance between cells i and j in n,m-automaton is defined as min(|i − j|, n − |i − j|). A d-environment of a cell is the set of cells at a distance not greater than d.
On each d-step values of all cells are simultaneously replaced by new values. The new value of cell i after d-step is computed as a sum of values of cells belonging to the d-enviroment of the cell i modulo m.
The following picture shows 1-step of the 5,3-automaton.
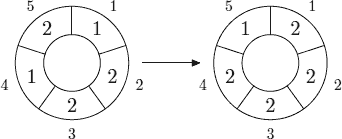
The problem is to calculate the state of the n,m-automaton after k d-steps.
Input
The first line of the input file contains four integer numbers n, m, d, and k (1 ≤ n ≤ 500, 1 ≤ m ≤ 1 000 000, 0 ≤ d < n⁄2 , 1 ≤ k ≤ 10 000 000). The second
line contains n integer numbers from 0 to m − 1 — initial values of the automaton’s cells.
Output
Output the values of the n,m-automaton’s cells after k d-steps.
Sample Input
sample input #1
5 3 1 1
1 2 2 1 2 sample input #2
5 3 1 10
1 2 2 1 2
Sample Output
sample output #1
2 2 2 2 1 sample output #2
2 0 0 2 2
#include <algorithm>
#include <iostream>
#include <stdlib.h>
#include <string.h>
#include <iomanip>
#include <stdio.h>
#include <string>
#include <queue>
#include <cmath>
#include <stack>
#include <map>
#include <set>
#define eps 1e-10
#define LL __int64
///#define LL long long
///#define INF 0x7ffffff
#define INF 0x3f3f3f3f
#define PI 3.1415926535898
#define zero(x) ((fabs(x)<eps)? 0:x) const int maxn = 550; using namespace std; LL e[maxn][maxn];
LL c[maxn][maxn];
LL xmul[maxn][maxn];
LL num[maxn];
LL ans[maxn];
int n, m, d; void Mul(LL a[][maxn], LL b[][maxn])
{
memset(c, 0, sizeof(c));
for(int i = 0; i < n; i++)
{
if(!a[0][i])
continue;
for(int j = 0; j < n; j++)
{
c[0][j] += a[0][i]*b[i][j];
c[0][j] %= m;
}
}
for(int i = 1; i < n; i++)
for(int j = 0; j < n; j++) c[i][j] = c[i-1][(j-1+n)%n];
for(int i = 0; i < n; i++)
for(int j = 0; j < n; j++) b[i][j] = c[i][j];
} void expo(LL a[][maxn], int k)
{
if(k == 1)
{
for(int i = 0; i < n; i++)
for(int j = 0; j < n; j++) e[i][j] = a[i][j];
return;
}
memset(e, 0, sizeof(e));
for(int i = 0; i < n; i++) e[i][i] = 1;
while(k)
{
if(k&1) Mul(a, e);
Mul(a, a);
k /= 2;
}
}
int main()
{
int k;
while(cin >>n>>m>>d>>k)
{
for(int i = 0; i < n; i++) cin >>num[i];
memset(xmul, 0, sizeof(xmul));
xmul[0][0] = 1;
for(int i = 1; i <= d; i++) xmul[0][i] = xmul[0][n-i] = 1;
for(int i = 1; i < n; i++)
for(int j = 0; j < n; j++) xmul[i][j] = xmul[i-1][(j-1+n)%n];
expo(xmul, k);
memset(ans, 0, sizeof(ans));
for(int i = 0; i < n; i++)
{
for(int j = 0; j < n; j++)
{
ans[i] += e[i][j]*num[j];
ans[i] %= m;
}
}
for(int i = 0; i < n-1; i++) cout<<ans[i]<<" ";
cout<<ans[n-1]<<endl;
}
return 0;
}
POJ 3150 Cellular Automaton(矩阵高速幂)的更多相关文章
- [POJ 3150] Cellular Automaton (矩阵高速幂 + 矩阵乘法优化)
Cellular Automaton Time Limit: 12000MS Memory Limit: 65536K Total Submissions: 3048 Accepted: 12 ...
- POJ 3150 Cellular Automaton --矩阵快速幂及优化
题意:给一个环,环上有n块,每块有个值,每一次操作是对每个点,他的值变为原来与他距离不超过d的位置的和,问k(10^7)次操作后每块的值. 解法:一看就要化为矩阵来做,矩阵很好建立,大白书P157页有 ...
- POJ 3150 Cellular Automaton(矩阵快速幂)
Cellular Automaton Time Limit: 12000MS Memory Limit: 65536K Total Submissions: 3504 Accepted: 1421 C ...
- POJ - 3150 :Cellular Automaton(特殊的矩阵,降维优化)
A cellular automaton is a collection of cells on a grid of specified shape that evolves through a nu ...
- POJ 3150 Cellular Automaton(矩阵乘法+二分)
题目链接 题意 : 给出n个数形成环形,一次转化就是将每一个数前后的d个数字的和对m取余,然后作为这个数,问进行k次转化后,数组变成什么. 思路 :下述来自here 首先来看一下Sample里的第一组 ...
- poj 3150 Cellular Automaton
首先来看一下Sample里的第一组数据.1 2 2 1 2经过一次变换之后就成了5 5 5 5 4它的原理就是a0 a1 a2 a3 a4->(a4+a0+a1) (a0+a1+a2) (a1+ ...
- poj 2778 AC自己主动机 + 矩阵高速幂
// poj 2778 AC自己主动机 + 矩阵高速幂 // // 题目链接: // // http://poj.org/problem?id=2778 // // 解题思路: // // 建立AC自 ...
- poj 3233(矩阵高速幂)
题目链接:http://poj.org/problem?id=3233. 题意:给出一个公式求这个式子模m的解: 分析:本题就是给的矩阵,所以非常显然是矩阵高速幂,但有一点.本题k的值非常大.所以要用 ...
- POJ 3613 Cow Relays (floyd + 矩阵高速幂)
题目大意: 求刚好经过K条路的最短路 我们知道假设一个矩阵A[i][j] 表示表示 i-j 是否可达 那么 A*A=B B[i][j] 就表示 i-j 刚好走过两条路的方法数 那么同理 我们把 ...
随机推荐
- 详解JavaScript中的原型和继承-转自颜海镜大大
本文将会介绍面向对象,继承,原型等相关知识,涉及的知识点如下: 面向对象与继承 CEOC OLOO 臃肿的对象 原型与原型链 修改原型的方式 面向对象与继承 最近学习了下python,还写了篇博文&l ...
- mongodb报错:connection refused because too many open connections: 819
问题: 发现mongodb无法连接,查看mongodb日志,出现大量的如下报错: [initandlisten] connection refused because too many open co ...
- 登录生成令牌token存于redis
package com.medic.rest.province.base.home; import java.util.HashMap;import java.util.List;import jav ...
- SASS 使用(vs code)
二.在vs code中编译sass 1.在拓展商店里搜索“easy sass”,并安装,安装成功后点重新加载. 2.接下来进行配置: 在 vs code 菜单栏依次点击“文件 首选项 设置”,打开 s ...
- POJ-1113 Wall 计算几何 求凸包
题目链接:https://cn.vjudge.net/problem/POJ-1113 题意 给一些点,求一个能够包围所有点且每个点到边界的距离不下于L的周长最小图形的周长 思路 求得凸包的周长,再加 ...
- 栈(stack)--c实现(使用双链表)
是不是直接贴代码不太好,我竟然不知道说什么. 写这个考虑的问题,或者是纠结的问题是这个头指针怎么处理,也就是栈的顶部,最后采用的是初始化第一个栈空间浪费掉,栈顶是有元素的.好像应该去学习下画图,没图不 ...
- poj1284 && caioj 1159 欧拉函数1:原根
这道题不知道这个定理很难做出来. 除非暴力找规律. 我原本找的时候出了问题 暴力找出的从13及以上的答案就有问题了 因为13的12次方会溢出 那么该怎么做? 快速幂派上用场. 把前几个素数的答案找出来 ...
- 【Divide by Zero 2017 and Codeforces Round #399 (Div. 1 + Div. 2, combined) B】 Code For 1
[链接] 我是链接,点我呀:) [题意] 在这里输入题意 [题解] 把序列生成的过程看成一颗树 会发现最后形成的是一颗二叉树. 每个二叉树上的节点就对应了序列中的一个数字. 如果我们把每个节点都往下投 ...
- Camera Calibration 相机标定:原理简介(五)
5 基于2D标定物的标定方法 基于2D标定物的标定方法,原理与基于3D标定物相同,只是通过相机对一个平面进行成像,就可得到相机的标定参数,由于标定物为平面,本身所具有的约束条机,相对后者标定更为简单. ...
- uva live 2326 - Moving Tables
把房间号映射在一条坐标上,然后排序,最后找从左到右找一次可行的计划,最后找从左到右找一次可行的计划,最后找从左到右找一次可行的计划,最后找从左到右找一次可行的计划, ............ 次数*1 ...