Record:Handle onClick for our custom LinearLayout for Gallery-like HorizontalScrollView
Handle onClick for our custom LinearLayout for Gallery-like HorizontalScrollView
In this post, we will implement OnClickListener for the ImageView(s) to handle user click.
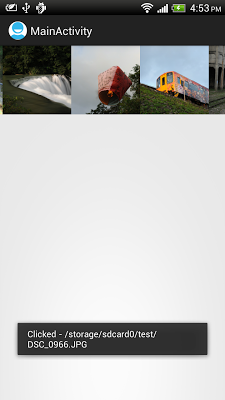
Modify MyHorizontalLayout.java from the exercise "Implement custom LinearLayout for Gallery-like HorizontalScrollView" to call setOnClickListener() when create ImageView, in getImageView() method. For "Implement Gallery-like HorizontalScrollView" without custom LinearLayout, you can do the same in insertPhoto() method.
package com.example.androidhorizontalscrollviewgallery;
import java.util.ArrayList;
import android.content.Context;
import android.graphics.Bitmap;
import android.graphics.BitmapFactory;
import android.util.AttributeSet;
import android.view.View;
import android.widget.ImageView;
import android.widget.LinearLayout;
import android.widget.Toast;
public class MyHorizontalLayout extends LinearLayout {
Context myContext;
ArrayList<String> itemList = new ArrayList<String>();
public MyHorizontalLayout(Context context) {
super(context);
myContext = context;
}
public MyHorizontalLayout(Context context, AttributeSet attrs) {
super(context, attrs);
myContext = context;
}
public MyHorizontalLayout(Context context, AttributeSet attrs,
int defStyle) {
super(context, attrs, defStyle);
myContext = context;
}
void add(String path){
int newIdx = itemList.size();
itemList.add(path);
addView(getImageView(newIdx));
}
ImageView getImageView(final int i){
Bitmap bm = null;
if (i < itemList.size()){
bm = decodeSampledBitmapFromUri(itemList.get(i), 220, 220);
}
ImageView imageView = new ImageView(myContext);
imageView.setLayoutParams(new LayoutParams(220, 220));
imageView.setScaleType(ImageView.ScaleType.CENTER_CROP);
imageView.setImageBitmap(bm);
imageView.setOnClickListener(new OnClickListener(){
@Override
public void onClick(View v) {
Toast.makeText(myContext,
"Clicked - " + itemList.get(i),
Toast.LENGTH_LONG).show();
}});
return imageView;
}
public Bitmap decodeSampledBitmapFromUri(String path, int reqWidth, int reqHeight) {
Bitmap bm = null;
// First decode with inJustDecodeBounds=true to check dimensions
final BitmapFactory.Options options = new BitmapFactory.Options();
options.inJustDecodeBounds = true;
BitmapFactory.decodeFile(path, options);
// Calculate inSampleSize
options.inSampleSize = calculateInSampleSize(options, reqWidth, reqHeight);
// Decode bitmap with inSampleSize set
options.inJustDecodeBounds = false;
bm = BitmapFactory.decodeFile(path, options);
return bm;
}
public int calculateInSampleSize(
BitmapFactory.Options options, int reqWidth, int reqHeight) {
// Raw height and width of image
final int height = options.outHeight;
final int width = options.outWidth;
int inSampleSize = 1;
if (height > reqHeight || width > reqWidth) {
if (width > height) {
inSampleSize = Math.round((float)height / (float)reqHeight);
} else {
inSampleSize = Math.round((float)width / (float)reqWidth);
}
}
return inSampleSize;
}
}
Record:Handle onClick for our custom LinearLayout for Gallery-like HorizontalScrollView的更多相关文章
- Android零基础入门第25节:最简单最常用的LinearLayout线性布局
原文:Android零基础入门第25节:最简单最常用的LinearLayout线性布局 良好的布局设计对于UI界面至关重要,在前面也简单介绍过,目前Android中的布局主要有6种,创建的布局文件默认 ...
- WordPress主题制作教程10:添加文章类型插件Custom Post Type UI
下载 Custom Post Type UI>> 用Custom Post Type UI添加自定义文章类型对于新手来说最简单不过了,下载安装后,在插件栏启用一下,就可以开始添加文章类型了 ...
- Record:逻辑分区下新建简单卷后其他卷被删除
上图是恢复后的磁盘情况,恢复前的情况没有截图. 事情是这样:扩展分区中原本有4个逻辑分区.想将其中一个分区(MySpace,第一个分区)压缩出部分空间新建一个分区.经过 压缩卷->新建简单卷 后 ...
- Akka(23): Stream:自定义流构件功能-Custom defined stream processing stages
从总体上看:akka-stream是由数据源头Source,流通节点Flow和数据流终点Sink三个框架性的流构件(stream components)组成的.这其中:Source和Sink是stre ...
- python爬虫(六)_urllib2:handle处理器和自定义opener
本文将介绍handler处理器和自定义opener,更多内容请参考:python学习指南 opener和handleer 我们之前一直使用的是urllib2.urlopen(url)这种形式来打开网页 ...
- Nodejs事件引擎libuv源码剖析之:句柄(handle)结构的设计剖析
声明:本文为原创博文,转载请注明出处. 句柄(handle)代表一种对持有资源的索引,句柄的叫法在window上较多,在unix/linux等系统上大多称之为描述符,为了抽象不同平台的差异,libuv ...
- 原生js:click和onclick本质的区别(转https://www.cnblogs.com/web1/p/6555662.html)
原生javascript的click在w3c里边的阐述是DOM button对象,也是html DOM click() 方法,可模拟在按钮上的一次鼠标单击. button 对象代表 HTML 文档中的 ...
- python+selenium自动化软件测试(第2章):WebDriver API
2.1 操作元素基本方法 前言前面已经把环境搭建好了,从这篇开始,正式学习selenium的webdriver框架.我们平常说的 selenium自动化,其实它并不是类似于QTP之类的有GUI界面的可 ...
- 【Bugly 技术干货】Android开发必备知识:为什么说Kotlin值得一试
1.Hello, Kotlin Bugly 技术干货系列内容主要涉及移动开发方向,是由 Bugly邀请腾讯内部各位技术大咖,通过日常工作经验的总结以及感悟撰写而成,内容均属原创,转载请标明出处. 1. ...
随机推荐
- jquery.validate:
jqueryValidation: jquery-UI 小组组长;https://jqueryvalidation.org/;从页面性能的角度来说: 最好是把js的引入放在结束的body标签上面;基本 ...
- CentOS_7.2服务器前期
一.禁用SELinux:# 永久禁用,需要重启生效: sed -i 's/SELINUX=enforcing/SELINUX=disabled/g' /etc/sysconfig/selinux se ...
- 查看cpu
使用系统命令top即可看到如下类似信息: Cpu(s): 0.0%us, 0.5%sy, 0.0%ni, 99.5%id, 0.0%wa, 0.0%hi, 0.0%si, 0.0%st ...
- sfliter__except_handler4
sfliter源码在vs08中编译 出现 错误error LNK2019: unresolved external symbol __except_handler4 referenced in fun ...
- Android中的布局动画
简介 布局动画是给布局的动画,会影响到布局中子对象 使用方法 给布局添加动画效果: 先找到要设置的layout的id,然后创建布局动画,创建一个LayoutAnimationController,并把 ...
- Android中的多线程编程
问题 Android的UI也是线程不安全的,如果要更新应用程序里的UI元素,必须在主线程中进行,否则就会抛异常.比如用一个Button的onClick函数去更新界面上的元素,就会得到一个CalledF ...
- 解决远程连接mysql错误1130代码的方法
命令行登陆 mysql -uroot -p 输入密码后登陆 use mysql; select host,user from user ; grant allon *.*to root i ...
- Delphi 有关Dbgrideh控件:变色处理
procedure OnDrawColumnCell( Sender: TObject; const Rect: TRect; DataCol: Integer; Column: TColumnEh; ...
- Permutations II 再分析
记得第一遍做这题的时候其实是没什么思路的,但是第二次的时候,我已经有"结果空间树"的概念了.这时候再看https://oj.leetcode.com/problems/permut ...
- 提高开发效率的十五个Visual Studio 2010使用技巧
相信做开发的没有不重视效率的.开发C#,VB的都知道,我们很依赖VS,或者说,我们很感谢VS.能够对一个IDE产生依赖,说明这个IDE确实有它的独特之处.无容置疑,VS是一个非常强大的IDE,它支持多 ...