HDU 4288-Coder(模拟)
Coder
Time Limit: 20000/10000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 3327 Accepted Submission(s): 1316
an algorithm that will replace many other employees. An added benefit to the employer is that the coder will also become redundant once their work is done. 1
You are now the signle coder, and have been assigned a new task writing code, since your boss would like to replace many other employees (and you when you become redundant once your task is complete).
Your code should be able to complete a task to replace these employees who do nothing all day but eating: make the digest sum.
By saying “digest sum” we study some properties of data. For the sake of simplicity, our data is a set of integers. Your code should give response to following operations:
1. add x – add the element x to the set;
2. del x – remove the element x from the set;
3. sum – find the digest sum of the set. The digest sum should be understood by
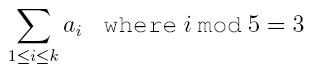
where the set S is written as {a1, a2, ... , ak} satisfying a1 < a2 < a3 < ... < ak
Can you complete this task (and be then fired)?
------------------------------------------------------------------------------
1 See http://uncyclopedia.wikia.com/wiki/Algorithm
In each test case, the first line contains one integer N ( 1 <= N <= 105 ), the number of operations to process.
Then following is n lines, each one containing one of three operations: “add x” or “del x” or “sum”.
You may assume that 1 <= x <= 109.
Please see the sample for detailed format.
For any “add x” it is guaranteed that x is not currently in the set just before this operation.
For any “del x” it is guaranteed that x must currently be in the set just before this operation.
Please process until EOF (End Of File).
9
add 1
add 2
add 3
add 4
add 5
sum
add 6
del 3
sum
6
add 1
add 3
add 5
add 7
add 9
sum
3
4
题意:给一个集合(严格升序。无反复) add x是将x增加集合。del x是将x从集合中删除,sum是求下标%5==3的元素的和。用数组模拟集合,插入和删除的时候要保证数组有序。然后求和暴力解决 6000+MS..#include <cstdio>
#include <iostream>
#include <algorithm>
#include <cstring>
#include <cctype>
#include <cmath>
#include <cstdlib>
#include <vector>
#include <queue>
#include <set>
#include <map>
#include <list>
#define ll long long
using namespace std;
const int INF=1<<27;
const int maxn=100010;
int a[maxn];
int Binary_search(int low,int high,int x)
{
int mid;
while(low<=high)
{
mid=(low+high)/2;
if(a[mid]==x)
return mid;
else if(a[mid]>x)
high=mid-1;
else if(a[mid]<x)
low=mid+1;
}
return -1;
}
int main()
{
int n,x,i;char op[5];
while(scanf("%d",&n)!=EOF)
{
int len=0;
while(n--)
{
scanf("%s",op);
if(op[0]=='a')
{
scanf("%d",&x);
for(i=len++;i>0;i--)
if(a[i-1]>x)
a[i]=a[i-1];
else
break;
a[i]=x;
}
else if(op[0]=='d')
{
scanf("%d",&x);
int indx=Binary_search(0,len-1,x);
while(indx<len-1)
{
a[indx]=a[indx+1];
indx++;
}
len--;
}
else
{
__int64 sum=0;
for(i=2;i<len;i+=5)
sum+=a[i];
printf("%I64d\n",sum);
}
}
}
return 0;
}
HDU 4288-Coder(模拟)的更多相关文章
- HDU 4288 Coder (线段树)
Coder 题目:http://acm.hdu.edu.cn/showproblem.php?pid=4288 题意:有三种类型的操作,(1)."add x",表示往集合里加入�数 ...
- hdu 4288 Coder
线段树好题,和 15 年的广东省省赛 C 题有相似之处,一开始我的思路有偏差,看了别人的博客后感觉处处技巧都是精华,主要是区间合并的技巧一时很难想到,先附上代码: #include<cstdio ...
- HDU 4288 Coder 【线段树+离线处理+离散化】
题意略. 离线处理,离散化.然后就是简单的线段树了.需要根据mod 5的值来维护.具体看代码了. /* 线段树+离散化+离线处理 */ #include <cstdio> #include ...
- HDU 4288 Coder(线段树)
题意: 给定三种操作 1. add x 向序列中添加x,添加之后序列还保持有序 2. del x 删除序列中值为x的元素 3. sum 求下边模5等于3的元素和 思路: 直接暴力也可以过,就是看暴 ...
- HDU 4288 Coder ( 离散化 + 离线 + 线段树 )
这题跟ZOJ 3606的解题思路很相似. 题意:有3中操作:1.向集合中增加一个数x(1≤x≤1e9):2.从集合中删去一个数x(保证这个数存在):3.查询集合中所有位置满足i%5==3的数a[i]的 ...
- hdu 4288 Coder(单点操作,查询)
题意: 三种操作: 1. add x – add the element x to the set;2. del x – remove the element x from the set;3. su ...
- hdu 4288 Coder (线段树+离线)
题意: 刚开始有一个空集合.有三种操作: 1.往集合中加入一个集合中不存在的数 x 2.从集合中删除一个已经存在的数 x 3.计算集合的digest sum并输出. digest sum求 ...
- hdu 4288 离线线段树+间隔求和
Coder Time Limit: 20000/10000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others) Total Su ...
- 线段树(单点更新) HDOJ 4288 Coder
题目传送门 #include <cstdio> #include <cstring> #define lson l, m, rt << 1 #define rson ...
- HDU 4121 Xiangqi 模拟题
Xiangqi Time Limit: 20 Sec Memory Limit: 256 MB 题目连接 http://acm.hdu.edu.cn/showproblem.php?pid=4121 ...
随机推荐
- SpringBoot2.0 监听器ApplicationListener的使用-监听ApplicationReadyEvent事件
参考:http://www.shareniu.com/article/73.htm 一.需求是想将我的写一个方法能在项目启动后就运行,之前使用了redis的消息监听器,感觉可以照着监听器这个思路做,于 ...
- 【 【henuacm2016级暑期训练】动态规划专题 K】 Really Big Numbers
[链接] 我是链接,点我呀:) [题意] 在这里输入题意 [题解] 会发现如果x是reallynumber那么x+1也会是reallynumber.... (个位数+1,各位数的和+1了但是整个数也+ ...
- spark源代码action系列-foreach与foreachPartition
RDD.foreachPartition/foreach的操作 在这个action的操作中: 这两个action主要用于对每一个partition中的iterator时行迭代的处理.通过用户传入的fu ...
- HDU Train Problem I (STL_栈)
Problem Description As the new term comes, the Ignatius Train Station is very busy nowadays. A lot o ...
- [cocos2dx笔记013]一个使用CCRenderTexture创建动态纹理显示数字的类
用CCLabelTTF显示的数字不好看.于是就想到用图片来代理.眼下网上的实现都是把每一个数字做一个CCSprite组合的方式. 可是我想.动态生成纹理的方式.没有就仅仅好自己手动写一个. 头文件 # ...
- atitit。流程图的设计与制作 attilax 总结
atitit.流程图的设计与制作 attilax 总结 1. 流程图的规范1 2. 画图语言2 2.1. atitit.CSDN-markdown编辑器2 2.2. js-sequence-diagr ...
- Cacti使用安装具体解释
Cacti是一套基于PHP,MySQL,SNMP及RRDTool开发的网络流量监測图形分析工具.Cacti是通过 snmpget来获取数据.使用 RRDtool绘绘图形,而且你全然能够不须要了解RRD ...
- Android开发之AudioManager(音频管理器)具体解释
AudioManager简单介绍: AudioManager类提供了訪问音量和振铃器mode控制. 使用Context.getSystemService(Context.AUDIO_SERVICE)来 ...
- ubuntu 下的文件校验(md5、sha256)
在本地使用 md5sum/sha256sum 生成某待测文件的 hash 值,以跟标准文件的 hash 值做对比验证,确定经网络传输过程得到的文件是否真实无损.一般而言,hash 值如果一致,大概率上 ...
- DB-MySQL:MySQL 运算符
ylbtech-DB-MySQL:MySQL 运算符 MySQL 运算符 本章节我们主要介绍 MySQL 的运算符及运算符的优先级. MySQL 主要有以下几种运算符: 算术运算符 比较运算符 逻辑运 ...