Balancing Act(poj1655)
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 12703 | Accepted: 5403 |
Description
For example, consider the tree:
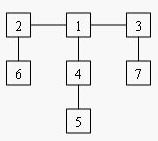
Deleting node 4 yields two trees whose member nodes are {5} and {1,2,3,6,7}. The larger of these two trees has five nodes, thus the balance of node 4 is five. Deleting node 1 yields a forest of three trees of equal size: {2,6}, {3,7}, and {4,5}. Each of these trees has two nodes, so the balance of node 1 is two.
For each input tree, calculate the node that has the minimum balance. If multiple nodes have equal balance, output the one with the lowest number.
Input
Output
Sample Input
1
7
2 6
1 2
1 4
4 5
3 7
3 1
Sample Output
1 2
题意:给你n个点,n-1条边形成一颗棵树,然后让你找树的重心;
思路:树形dp;
先dfs求出每个点所形成的子树的权值,然后再dfs求权值中的最大值更新dp。因为当前点的各个子树的权值都知道,那么只要求出当前节点父亲节点的权值,nod-sum[n];
复杂度O(n);
1 #include<stdio.h>
2 #include<math.h>
3 #include<queue>
4 #include<algorithm>
5 #include<string.h>
6 #include<iostream>
7 #include<stack>
8 #include<vector>
9 using namespace std;
10 typedef long long LL;
11 vector<int>vec[20005];
12 int dp[20005];
13 bool flag[20005];
14 int sum[20005];
15 void dfs(int n);
16 void dfs2(int n);
17 int nod;
18 int main(void)
19 {
20 int t;
21 scanf("%d",&t);
22 while(t--)
23 {
24 int n;
25 scanf("%d",&nod);
26 n = nod;
27 for(int i = 0;i < 20005;i++)
28 vec[i].clear();
29 for(int i = 0; i < n-1; i++)
30 {
31 int a,b;
32 scanf("%d %d",&a,&b);
33 vec[a].push_back(b);
34 vec[b].push_back(a);
35 }
36 memset(flag,0,sizeof(flag));
37 memset(dp,0,sizeof(dp));
38 memset(sum,0,sizeof(sum));
39 dfs(1);
40 memset(flag,0,sizeof(flag));
41 dfs2(1);
42 int id = 0;
43 int maxx = 1e9;
44 for(int i = 1; i <= n; i++)
45 {
46 if(maxx > dp[i])
47 maxx = dp[i],id = i;
48 }
49 printf("%d %d\n",id,maxx);
50 }
51 return 0;
52 }
53 void dfs(int n)
54 {
55 int i,j;
56 flag[n] = true;
57 for(i = 0; i < vec[n].size(); i++)
58 {
59 int id = vec[n][i];
60 if(!flag[id])
61 {
62 dfs(id);
63 sum[n]+=sum[id];
64 }
65 }
66 sum[n]++;
67 }
68 void dfs2(int n)
69 {
70 flag[n] = true;
71 int i,j;
72 for(i = 0; i < vec[n].size(); i++)
73 {
74 int id = vec[n][i];
75 if(!flag[id])
76 {
77 dp[n] = max(dp[n],sum[id]);
78 dfs2(id);
79 }
80 }
81 dp[n] = max(dp[n],nod-sum[n]);
82 }
Balancing Act(poj1655)的更多相关文章
- 『Balancing Act 树的重心』
树的重心 我们先来认识一下树的重心. 树的重心也叫树的质心.找到一个点,其所有的子树中最大的子树节点数最少,那么这个点就是这棵树的重心,删去重心后,生成的多棵树尽可能平衡. 根据树的重心的定义,我们可 ...
- poj1655 Balancing Act 找树的重心
http://poj.org/problem? id=1655 Balancing Act Time Limit: 1000MS Memory Limit: 65536K Total Submis ...
- POJ1655 Balancing Act(树的重心)
题目链接 Balancing Act 就是求一棵树的重心,然后统计答案. #include <bits/stdc++.h> using namespace std; #define REP ...
- poj-1655 Balancing Act(树的重心+树形dp)
题目链接: Balancing Act Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 11845 Accepted: 4 ...
- poj1655 Balancing Act (dp? dfs?)
Balancing Act Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 14247 Accepted: 6026 De ...
- POJ 1655 Balancing Act 树的重心
Balancing Act Description Consider a tree T with N (1 <= N <= 20,000) nodes numbered 1...N. ...
- POJ 1655 Balancing Act【树的重心】
Balancing Act Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 14251 Accepted: 6027 De ...
- POJ 1655.Balancing Act 树形dp 树的重心
Balancing Act Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 14550 Accepted: 6173 De ...
- POJ.1655 Balancing Act POJ.3107 Godfather(树的重心)
关于树的重心:百度百科 有关博客:http://blog.csdn.net/acdreamers/article/details/16905653 1.Balancing Act To POJ.165 ...
随机推荐
- 汇编LED实验
汇编语言点亮LED 拿到一款全新的芯片,第一个要做的事情的就是驱动其 GPIO,控制其 GPIO 输出高低电平. GPIO口是IO口的一个功能之一. 一.接下来的步骤离不开芯片手册: 1.使能所有时钟 ...
- Prometheus概述
Prometheus是什么 首先, Prometheus 是一款时序(time series) 数据库, 但他的功能却并非支部与 TSDB , 而是一款设计用于进行目标 (Target) 监控的关键组 ...
- IPFS是什么?IPFS原理、IPFS存储
以下内容调研截止到2021/11/5日 IPFS简介 IPFS是一种内容可寻址.点对点.分布式文件系统.IPFS采用内容-地址寻址技术,即通过文件内容进行检索而不是通过文件的网络地址.简单来说,就是对 ...
- 巩固javaweb的第三十一天
巩固内容 变量的作用范围 如果要访问的信息在 pageScope.requestScope.sessionScope 和 applicationScope 中存储, 则使用表达式语言访问的时候可以直接 ...
- [源码解析] PyTorch分布式优化器(1)----基石篇
[源码解析] PyTorch分布式优化器(1)----基石篇 目录 [源码解析] PyTorch分布式优化器(1)----基石篇 0x00 摘要 0x01 从问题出发 1.1 示例 1.2 问题点 0 ...
- springcloud - alibaba - 3 - 整合config - 更新完毕
0.补充 1.需求 如果我有这么一个请求:我想要gitee中的配置改了之后,我程序yml中的配置也可以跟着相应产生变化,利用原生的方式怎么做?一般做法如下: 而有了SpringCloud-alibab ...
- 日常Java 2021/11/15
Applet类 每一个Applet都是java.applet Applet类的子类,基础的Applet类提供了供衍生类调用的方法,以此来得到浏览器上下文的信息和服务.这些方法做了如下事情: 得到App ...
- 27.0 linux VM虚拟机IP问题
我的虚拟机是每次换一个不同的网络,b不同的ip,使用桥接模式就无法连接,就需要重新还原默认设置才行: 第一步:点击虚拟机中的编辑-->虚拟网络编辑器 第二步:点击更改设置以管理员权限进入 第三步 ...
- 源码分析-NameServer
架构设计 消息中间件的设计思路一般是基于主题订阅发布的机制,消息生产者(Producer)发送某一个主题到消息服务器,消息服务器负责将消息持久化存储,消息消费者(Consumer)订阅该兴趣的主题,消 ...
- CRLF漏洞浅析
部分情况下,由于与客户端存在交互,会形成下面的情况 也就是重定向且Location字段可控 如果这个时候,可以向Location字段传点qqgg的东西 形成固定会话 但服务端应该不会存储,因为后端貌似 ...