题解报告:poj 1195 Mobile phones(二维BIT裸题)
Description
Write a program, which receives these reports and answers queries about the current total number of active mobile phones in any rectangle-shaped area.
Input
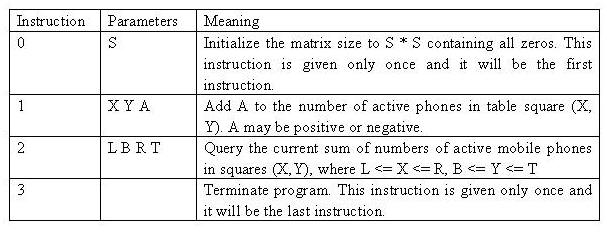
The values will always be in range, so there is no need to check them. In particular, if A is negative, it can be assumed that it will not reduce the square value below zero. The indexing starts at 0, e.g. for a table of size 4 * 4, we have 0 <= X <= 3 and 0 <= Y <= 3.
Table size: 1 * 1 <= S * S <= 1024 * 1024
Cell value V at any time: 0 <= V <= 32767
Update amount: -32768 <= A <= 32767
No of instructions in input: 3 <= U <= 60002
Maximum number of phones in the whole table: M= 2^30
Output
Sample Input
0 4
1 1 2 3
2 0 0 2 2
1 1 1 2
1 1 2 -1
2 1 1 2 3
3
Sample Output
3
4
解题思路:这是一道二维树状数组入门题---单点修改、单点(区间)查询,其思路和一维树状数组非常相似,多加了一个维度而已。下面我们来看看怎么实现这两个基本操作:
将一维数组A[]扩展到二维数组A[][],二维树状数组C[][]来维护矩阵前缀和。
设原始二维数组A[][]={a11,a12,a13,a14,a15,
a21,a22,a23,a24,a25,
a31,a32,a33,a34,a35,
a41,a42,a43,a44,a45,
a51,a52,a53,a54,a55};
那么二维树状数组表示如下:
C[1][]=a1,C[1][]=a1+a1,C[1][]=a1,C[1][]=a1+a1+a1+a1,C[1][]=a15
这是数组A[][]第一行的一维树状数组;
C[][1]=a1+a1,C[][]=a1+a+a+a,C[][]=a+a,C[][]=a+a+a+a+a+a+a+a,C[][]=a+a5
这是数组A[][]第一行和第二行相加后得到的树状数组;
C[3][]=a3,C[3][]=a3+a3,C[3][]=a3,C[3][]=a3+a3+a3+a3,C[3][]=a35
这是数组A[][]第三行的一维树状数组;
C[][]=a+a+a+a,C[][]=a+a+a+a+a+a+a+a,C[][]=a+a+a+a...
这是数组A[][]前4行相加后得到的树状数组;
C[5][]=a5,C[5][]=a5+a5,C[5][]=a5,C[5][]=a5+a5+a5+a5,C[5][]=a55
这是数组A[][]第5行的一维树状数组。
仔细观察以上式子可以发现,二维树状数组C[x][y]的值其实是分别在x、y上的一维树状数组向下、向右(x上+lowbit(x)跳跃(>n停止),y上+lowbit(y)跳跃(>n停止))进行求和,这就是矩阵中坐标点值的单点修改。对于区间查询,同样分别在x、y上的一维树状数组从下往上,从右往左进行累加(y上-lowbit(y)跳跃(<=0停止),x上-lowbit(x)跳跃(<=0停止)),这样就得到了(1,1)到(x,y)矩阵中所有元素的和。
回到本题,题意为给出一些命令进行一些操作:
0 S 初始化一个全0的S*S矩阵,这个命令只会在第一次给出一次;
1 X Y A 给坐标点(X,Y)的值加上A;
2 L B R T 询问(L,B)到(R,T)构成的矩阵中所有元素的总和;
3 结束对矩阵的操作,程序终止。
典型的二维BIT,套一下模板即可,但需要注意一点:给出命令中的坐标都是默认从下标0开始的,为避免陷入死循环和计算错误,在更新和询问操作上统一对每一个坐标点(横、纵坐标)加1。
怎么统计坐标点(L,B)到(R,T)矩阵内所有值呢?给出下面的矩阵:
1 2 3 4 5
1 0 0 0 0 0
2 0 0 0 0 0
3 0 0 0
4 0 0 0
5 0 0 0 0
从图上可得计算公式:[R,T]-[L-1,T]-[R,B-1]+[L-1,B-1](多减去了一个左上角的矩阵,还要把它加回来),这样就得到了点(L,B)到(R,T)矩阵中所有元素的和。
AC代码:
#include<cstdio>
#include<string.h>
const int maxn=;
int op,s,x,y,a,l,b,r,t,C[maxn][maxn];
void add(int x,int y,int val){//单点修改
for(int i=x;i<=s;i+=i&-i)
for(int j=y;j<=s;j+=j&-j)
C[i][j]+=val;
}
int query(int x,int y){//前缀和查询
int ans=;
for(int i=x;i>;i-=i&-i)
for(int j=y;j>;j-=j&-j)
ans+=C[i][j];
return ans;
}
int main(){
while(~scanf("%d%d",&op,&s)){
memset(C,,sizeof(C));
while(~scanf("%d",&op)&&op!=){
if(op==){
scanf("%d%d%d",&x,&y,&a);
x++,y++;add(x,y,a);//单点修改
}
else{
scanf("%d%d%d%d",&l,&b,&r,&t);l++,b++,r++,t++;
printf("%d\n",query(r,t)-query(l-,t)-query(r,b-)+query(l-,b-));//区间查询,求矩形中所有元素的和
}
}
}
return ;
}
题解报告:poj 1195 Mobile phones(二维BIT裸题)的更多相关文章
- poj 1195 Mobile phones(二维树状数组)
树状数组支持两种操作: Add(x, d)操作: 让a[x]增加d. Query(L,R): 计算 a[L]+a[L+1]……a[R]. 当要频繁的对数组元素进行修改,同时又要频繁的查询数组内任一 ...
- poj 1195:Mobile phones(二维树状数组,矩阵求和)
Mobile phones Time Limit: 5000MS Memory Limit: 65536K Total Submissions: 14489 Accepted: 6735 De ...
- poj 1195:Mobile phones(二维线段树,矩阵求和)
Mobile phones Time Limit: 5000MS Memory Limit: 65536K Total Submissions: 14391 Accepted: 6685 De ...
- (简单) POJ 1195 Mobile phones,二维树状数组。
Description Suppose that the fourth generation mobile phone base stations in the Tampere area operat ...
- POJ 1195 Mobile phones(二维树状数组)
Mobile phones Time Limit: 5000MS Mem ...
- POJ 1195 Mobile phones (二维树状数组)
Description Suppose that the fourth generation mobile phone base stations in the Tampere area operat ...
- POJ 1195:Mobile phones 二维树状数组
Mobile phones Time Limit: 5000MS Memory Limit: 65536K Total Submissions: 16893 Accepted: 7789 De ...
- POJ 1195 Mobile phones【二维树状数组】
<题目链接> 题目大意: 一个由数字构成的大矩阵,开始是全0,能进行两种操作1) 对矩阵里的某个数加上一个整数(可正可负)2) 查询某个子矩阵里所有数字的和要求对每次查询,输出结果 解题分 ...
- POJ 1195 Mobile phones【 二维树状数组 】
题意:基础的二维数组,注意 0 + lowbit(0)会陷入无限循环----- 之前做一道一维的一直tle,就是因为这个-------------------------- #include<i ...
随机推荐
- [转]JavaEE开发基础
JavaEE开发基础 1 JavaEE简介 Java平台有三个版本,分别是JavaSE(Java Platform, Standard Edition),JavaEE(Java Platform, E ...
- Linux 的 Socket IO 模型
前言 之前有看到用很幽默的方式讲解Windows的socket IO模型,借用这个故事,讲解下linux的socket IO模型: 老陈有一个在外地工作的女儿,不能经常回来,老陈和她通过信件联系. 他 ...
- SQL 用于各种数据库的数据类型
SQL 用于各种数据库的数据类型 Microsoft Access.MySQL 和 SQL Server 所使用的数据类型和范围. Microsoft Access 数据类型 数据类型 描述 存储 T ...
- 【转】Selenium2学习路线
课程大纲:第一部分:基础入门 第一课: SELENIUM2的原理介绍及环境搭建本节课主要讲解SELENIUM2的原理,让大家了解SELENIUM2的发展历程,同时解惑大家对自动化测试中产生的一些误区. ...
- C++标准I/O库:iostream, fstream, sstringstream
在写代码的过程中.我们最常做的事就是io操作,不管是对控制台,还是文件.但一段时间不写代码就忘了,这里理一下C++标准I/O库的详细类和操作. C++的标准I/O库包含我们常常使用的iostream, ...
- 如何更有效的消灭watchdogs挖矿病毒?华为云DCS Redis为您支招
漏洞概述 近日,互联网出现watchdogs挖矿病毒,攻击者可以利用Redis未授权访问漏洞入侵服务器,通过内外网扫描感染更多机器.被感染的主机出现 crontab 任务异常.系统文件被删除.CPU ...
- lmhostid获取hostid为空问题
lmhostid获取hostid为空问题 问题描写叙述 今天迁移曾经的一个装有flexlm的虚拟机,结果发如今迁移后启动时报错 ... Wrong hostid on SERVER line for ...
- Educational Codeforces Round 18 C. Divide by Three DP
C. Divide by Three A positive integer number n is written on a blackboard. It consists of not more ...
- caution
做好需求更改的准备,提高代码的扩展性和可维护性:预留出修改bug和需求的时间:对需求理解透彻再开始写代码:代码不要写死,防止需求变动.
- unbantu16.04安装jdk
1,解压缩jdk到指定目录 2,修改目录,方便使用 3,配置环境变量 sudo gedit /etc/environment 末尾加入以下配置(JAVA_HOME 后的路径就是jdk的文件位置) PA ...