10Flutter页面布局 Padding Row Column Expanded组件详解:
Padding组件:
main.dart
- import 'package:flutter/material.dart';
- import 'res/listData.dart';
- /*
- flutter页面布局Padding Row Column Expanded组件详情:
- */
- void main() {
- runApp(MyApp());
- }
- class MyApp extends StatelessWidget {
- @override
- Widget build(BuildContext context) {
- // TODO: implement build
- return MaterialApp(
- home: Scaffold(
- appBar: AppBar(
- title: Text('Padding Row Column Expanded'),
- ),
- body: HomeContent(),
- ),
- );
- }
- }
- class HomeContent extends StatelessWidget {
- @override
- Widget build(BuildContext context) {
- return Padding(
- padding: EdgeInsets.fromLTRB(, , , ),
- child: GridView.count(
- crossAxisCount: ,
- childAspectRatio: 1.7,
- children: <Widget>[
- Padding(
- padding: EdgeInsets.fromLTRB(, , , ),
- child: Image.network("https://www.itying.com/images/flutter/1.png",
- fit: BoxFit.cover),
- ),
- Padding(
- padding: EdgeInsets.fromLTRB(, , , ),
- child: Image.network("https://www.itying.com/images/flutter/1.png",
- fit: BoxFit.cover),
- ),
- Padding(
- padding: EdgeInsets.fromLTRB(, , , ),
- child: Image.network("https://www.itying.com/images/flutter/1.png",
- fit: BoxFit.cover),
- ),
- Padding(
- padding: EdgeInsets.fromLTRB(, , , ),
- child: Image.network("https://www.itying.com/images/flutter/1.png",
- fit: BoxFit.cover),
- ),
- Padding(
- padding: EdgeInsets.fromLTRB(, , , ),
- child: Image.network("https://www.itying.com/images/flutter/1.png",
- fit: BoxFit.cover),
- )
- ],
- ),
- );
- }
- }
Row水平布局组件:
- import 'package:flutter/material.dart';
- import 'res/listData.dart';
- /*
- flutter页面布局Padding Row Column Expanded组件详情:
- Row水平布局组件:
- mainAxisAlignment 主轴的排序方式
- crossAxisAlignment 次轴的排序方式
- children 组件子元素:
- */
- void main() {
- runApp(MyApp());
- }
- class MyApp extends StatelessWidget {
- @override
- Widget build(BuildContext context) {
- // TODO: implement build
- return MaterialApp(
- home: Scaffold(
- appBar: AppBar(
- title: Text('Padding Row Column Expanded'),
- ),
- body: HomeContent(),
- ),
- );
- }
- }
- class HomeContent extends StatelessWidget {
- @override
- Widget build(BuildContext context) {
- return Container(
- height: 800.0,
- width: 400.0,
- color: Colors.pink,
- child: Row(
- mainAxisAlignment: MainAxisAlignment.spaceEvenly,
- crossAxisAlignment: CrossAxisAlignment.start,
- children: <Widget>[
- IconContainer(Icons.home, color: Colors.blue),
- IconContainer(Icons.search, color: Colors.orange),
- IconContainer(Icons.select_all, color: Colors.red)
- ],
- ),
- );
- }
- }
- class IconContainer extends StatelessWidget {
- double size = 32.0;
- Color color = Colors.red;
- IconData icon;
- IconContainer(this.icon, {this.color, this.size}) {}
- @override
- Widget build(BuildContext context) {
- // TODO: implement build
- return Container(
- height: 100.0,
- width: 100.0,
- color: this.color,
- child: Center(
- child: Icon(this.icon, size: this.size, color: Colors.white),
- ),
- );
- }
- }
Column 垂直布局:
- import 'package:flutter/material.dart';
- import 'res/listData.dart';
- /*
- flutter页面布局Padding Row Column Expanded组件详情:
- Column水平布局组件:
- mainAxisAlignment 主轴的排序方式
- crossAxisAlignment 次轴的排序方式
- children 组件子元素:
- */
- void main() {
- runApp(MyApp());
- }
- class MyApp extends StatelessWidget {
- @override
- Widget build(BuildContext context) {
- // TODO: implement build
- return MaterialApp(
- home: Scaffold(
- appBar: AppBar(
- title: Text('Padding Row Column Expanded'),
- ),
- body: HomeContent(),
- ),
- );
- }
- }
- class HomeContent extends StatelessWidget {
- @override
- Widget build(BuildContext context) {
- return Container(
- height: 800.0,
- width: 400.0,
- color: Colors.pink,
- child: Column(
- mainAxisAlignment: MainAxisAlignment.spaceEvenly,
- crossAxisAlignment: CrossAxisAlignment.start,
- children: <Widget>[
- IconContainer(Icons.home, color: Colors.blue),
- IconContainer(Icons.search, color: Colors.orange),
- IconContainer(Icons.select_all, color: Colors.red)
- ],
- ),
- );
- }
- }
- class IconContainer extends StatelessWidget {
- double size = 32.0;
- Color color = Colors.red;
- IconData icon;
- IconContainer(this.icon, {this.color, this.size}) {}
- @override
- Widget build(BuildContext context) {
- // TODO: implement build
- return Container(
- height: 100.0,
- width: 100.0,
- color: this.color,
- child: Center(
- child: Icon(this.icon, size: this.size, color: Colors.white),
- ),
- );
- }
- }
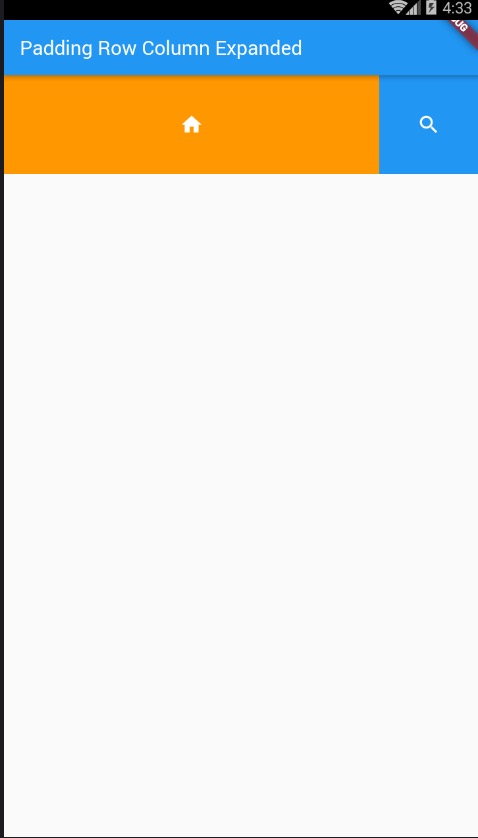
- import 'package:flutter/material.dart';
- import 'res/listData.dart';
- /*
- Flutter Expanded类似Web中的Flex布局:
- flex:元素占整个父Row/Column的比例:
- child 子元素
- */
- void main() {
- runApp(MyApp());
- }
- class MyApp extends StatelessWidget {
- @override
- Widget build(BuildContext context) {
- // TODO: implement build
- return MaterialApp(
- home: Scaffold(
- appBar: AppBar(
- title: Text('Padding Row Column Expanded'),
- ),
- body: HomeContent(),
- ),
- );
- }
- }
- class HomeContent extends StatelessWidget {
- @override
- Widget build(BuildContext context) {
- return Row(
- children: <Widget>[
- // Expanded(
- // flex: 1,
- // child: IconContainer(Icons.search,color:Colors.blue),
- // ),
- // Expanded(
- // flex: 2,
- // child: IconContainer(Icons.home,color:Colors.orange),
- // ),
- // Expanded(
- // flex: 1,
- // child: IconContainer(Icons.select_all,color:Colors.red),
- // )
- Expanded(
- flex: ,
- child: IconContainer(Icons.home,color:Colors.orange),
- ),
- IconContainer(Icons.search,color:Colors.blue)
- ],
- );
- }
- }
- class IconContainer extends StatelessWidget {
- double size = 32.0;
- Color color = Colors.red;
- IconData icon;
- IconContainer(this.icon, {this.color, this.size}) {}
- @override
- Widget build(BuildContext context) {
- // TODO: implement build
- return Container(
- height: 100.0,
- width: 100.0,
- color: this.color,
- child: Center(
- child: Icon(this.icon, size: this.size, color: Colors.white),
- ),
- );
- }
- }
10Flutter页面布局 Padding Row Column Expanded组件详解:的更多相关文章
- 页面布局 Paddiing Row Column Expanded 组件详解
一.Paddiing 组件 padding EdgeInsetss 设置填充的值 child 组件 return Padding( padding: EdgeIn ...
- flutter 页面布局 Paddiing Row Column Expanded 组件
Flutter Paddiing 组件 在 html 中常见的布局标签都有 padding 属性,但是 Flutter 中很多 Widget 是没有 padding 属 性.这个时候我们可以用 Pad ...
- Tomcat负载均衡、调优核心应用进阶学习笔记(一):tomcat文件目录、页面、架构组件详解、tomcat运行方式、组件介绍、tomcat管理
文章目录 tomcat文件目录 bin conf lib logs temp webapps work 页面 架构组件详解 tomcat运行方式 组件介绍 tomcat管理 tomcat文件目录 ➜ ...
- Echars 6大公共组件详解
Echars 六大组件详解 : title tooltip toolbox legend dataZoom visualMap 一.title标题详解 myTitleStyle = { color ...
- Angular6 学习笔记——组件详解之组件通讯
angular6.x系列的学习笔记记录,仍在不断完善中,学习地址: https://www.angular.cn/guide/template-syntax http://www.ngfans.net ...
- Angular6 学习笔记——组件详解之模板语法
angular6.x系列的学习笔记记录,仍在不断完善中,学习地址: https://www.angular.cn/guide/template-syntax http://www.ngfans.net ...
- Tomcat系列之服务器的安装与配置以及各组件详解
Tomcat系列之服务器的安装与配置以及各组件详解 大纲 一.前言 二.安装与配置Tomcat 三.Tomcat 目录的结构 四.Tomcat 配置文件 注,本文的测试的操作系统为CentOS 6.4 ...
- Android中Intent组件详解
Intent是不同组件之间相互通讯的纽带,封装了不同组件之间通讯的条件.Intent本身是定义为一个类别(Class),一个Intent对象表达一个目的(Goal)或期望(Expectation),叙 ...
- Android笔记——四大组件详解与总结
android四大组件分别为activity.service.content provider.broadcast receiver. ------------------------------- ...
随机推荐
- vi/vim常用按键
最近这段时间坚持了vim的使用,我在我的IDEA里面加了一个插件,可以支持vim. 然后不管是IDEA还是Vim都有自己的按键,而且都很好用,所以我就总结下在IDEA下的vim使用命令 当然,都是原生 ...
- 7.MapReduce操作Hbase
7 HBase的MapReduce HBase中Table和Region的关系,有些类似HDFS中File和Block的关系.由于HBase提供了配套的与MapReduce进行交互的API如 Ta ...
- select函数的详细使用(C语言)
Select在Socket编程中还是比较重要的,可是对于初学Socket的人来说都不太爱用Select写程序,他们只是习惯写诸如connect.accept.recv或recvfrom这样的阻塞程序( ...
- 一个异常研究InvalidApartmentStateChange
微软资料:invalidApartmentStateChange MDA 地址:https://docs.microsoft.com/zh-cn/dotnet/framework/debug-trac ...
- ASP.NET Core 2.0身份和角色管理入门
见 https://blog.csdn.net/mzl87/article/details/84892916 https://www.codeproject.com/Articles/1235077 ...
- ubunu安装qq、微信等、
参考: https://www.lulinux.com/archives/1319 安装下面下载deepin-wine-for-ubuntu,然后进去安装 https://github.com/wsz ...
- npm设置成淘宝镜像
1.淘宝 npm 网址 https://npm.taobao.org/ 2.修改 2.1 通过命令配置 2.1.1. 命令 npm config set registry https://regist ...
- Entity Framework学习过程
///安装ef nuget中文控制台指令 PM> Install-Package EntityFramework.zh-Hans <!--配置数据库连接--> <connect ...
- Python 11--文件流
- js 获取窗口大小
//获得窗口大小 function findDimensions() //函数:获取尺寸 { var point = {}; ...