Chapter 6(树)
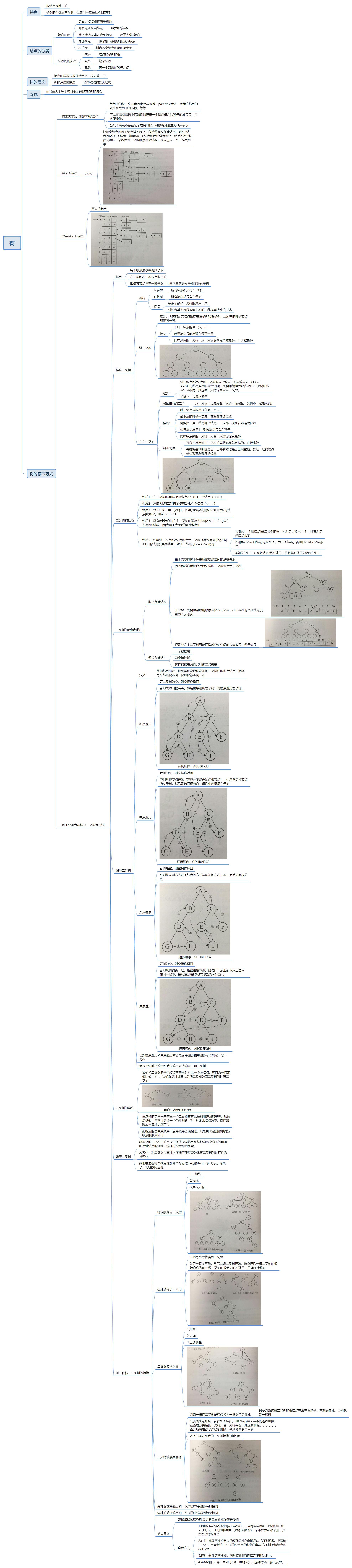
//****************双亲表示法************************
#define Max_TREE_SIZE 100
typedef int TElemType;
typedef struct PTNode //结点结构
{
TElemType data;
int parent;
}PTNode;
typedef struct
{
PTNode nodes[Max_TREE_SIZE];//结点数组
int r,n;
}Ptree;
//****************孩子表示法************************
typedef struct CTNode //孩子结点
{
int child;
struct CTNode *next;
}*ChildPtr;
typedef struct //表头结构
{
TElemType data;
ChildPtr firstChild;
}CTBox;
typedef struct
{
CTBox nodes[Max_TREE_SIZE]; //结点数组
int r,n;
}CTree;
//****************双亲孩子表示法************************
typedef struct CTNode
{
int child;
struct CTNode *next;
}*ChildPtr;
typedef struct
{
TElemType data;
int parent;
ChildPtr firstChild;
}CTBox;
typedef struct
{
CTBox nodes[Max_TREE_SIZE];
int r,n;
}CTree;
- //****************双亲表示法************************
- #define Max_TREE_SIZE 100
- typedef int TElemType;
- typedef struct PTNode //结点结构
- {
- TElemType data;
- int parent;
- }PTNode;
- typedef struct
- {
- PTNode nodes[Max_TREE_SIZE];//结点数组
- int r,n;
- }Ptree;
- //****************孩子表示法************************
- typedef struct CTNode //孩子结点
- {
- int child;
- struct CTNode *next;
- }*ChildPtr;
- typedef struct //表头结构
- {
- TElemType data;
- ChildPtr firstChild;
- }CTBox;
- typedef struct
- {
- CTBox nodes[Max_TREE_SIZE]; //结点数组
- int r,n;
- }CTree;
- //****************双亲孩子表示法************************
- typedef struct CTNode
- {
- int child;
- struct CTNode *next;
- }*ChildPtr;
- typedef struct
- {
- TElemType data;
- int parent;
- ChildPtr firstChild;
- }CTBox;
- typedef struct
- {
- CTBox nodes[Max_TREE_SIZE];
- int r,n;
- }CTree;
//**************************BiTree.h*******************************
#ifndef BITREE_H
#define BITREE_H
#include <stdio.h>
#include <stdlib.h>
typedef int datatype;
typedef struct Tree
{
datatype data;
struct Tree *left;
struct Tree *right;
}Node,*BiTree;
//以输入扩展前序递归的方式创建二叉树
void CreateBiTree(BiTree *T);
//后序遍历
void NextOrderTravel(BiTree T);
#endif //BITREE_H
//**************************BiTree.c*******************************
#include "BiTree.h"
//以输入扩展前序递归的方式创建二叉树
void CreateBiTree(BiTree *T)
{
char ch;
scanf("%c",&ch);
if('#' == ch)
{
*T = NULL;
}
else
{
*T = (BiTree)malloc(sizeof(Node));
(*T)->data = ch;
CreateBiTree(&(*T)->left);
CreateBiTree(&(*T)->right);
}
}
void NextOrderTravel(BiTree T)
{
if(NULL == T)return;
NextOrderTravel(T->left);
NextOrderTravel(T->right);
printf("%c ",T->data);
}
//**************************BiTreeTest.c*******************************
#include <stdio.h>
#include "BiTree.h"
char *str;
void CreateBiTreeStr(BiTree *T)
{
char ch = *str++;
if('#' == ch)
{
*T = NULL;
}
else
{
*T = (BiTree)malloc(sizeof(Node));
(*T)->data = ch;
CreateBiTreeStr(&(*T)->left);
CreateBiTreeStr(&(*T)->right);
}
}
//将这个序列以字符串的形式输入函数生成二叉树
void Create(BiTree *T,char *str1)
{
str = str1;
CreateBiTreeStr(T);
}
int main()
{
BiTree T = NULL;
CreateBiTree(&T);
NextOrderTravel(T);
printf("\n-------\n");
char *str1 = "AB#D##C##";
Create(&T,str1);
NextOrderTravel(T);
}
- //**************************BiTree.h*******************************
- #ifndef BITREE_H
- #define BITREE_H
- #include <stdio.h>
- #include <stdlib.h>
- typedef int datatype;
- typedef struct Tree
- {
- datatype data;
- struct Tree *left;
- struct Tree *right;
- }Node,*BiTree;
- //以输入扩展前序递归的方式创建二叉树
- void CreateBiTree(BiTree *T);
- //后序遍历
- void NextOrderTravel(BiTree T);
- #endif //BITREE_H
- //**************************BiTree.c*******************************
- #include "BiTree.h"
- //以输入扩展前序递归的方式创建二叉树
- void CreateBiTree(BiTree *T)
- {
- char ch;
- scanf("%c",&ch);
- if('#' == ch)
- {
- *T = NULL;
- }
- else
- {
- *T = (BiTree)malloc(sizeof(Node));
- (*T)->data = ch;
- CreateBiTree(&(*T)->left);
- CreateBiTree(&(*T)->right);
- }
- }
- void NextOrderTravel(BiTree T)
- {
- if(NULL == T)return;
- NextOrderTravel(T->left);
- NextOrderTravel(T->right);
- printf("%c ",T->data);
- }
- //**************************BiTreeTest.c*******************************
- #include <stdio.h>
- #include "BiTree.h"
- char *str;
- void CreateBiTreeStr(BiTree *T)
- {
- char ch = *str++;
- if('#' == ch)
- {
- *T = NULL;
- }
- else
- {
- *T = (BiTree)malloc(sizeof(Node));
- (*T)->data = ch;
- CreateBiTreeStr(&(*T)->left);
- CreateBiTreeStr(&(*T)->right);
- }
- }
- //将这个序列以字符串的形式输入函数生成二叉树
- void Create(BiTree *T,char *str1)
- {
- str = str1;
- CreateBiTreeStr(T);
- }
- int main()
- {
- BiTree T = NULL;
- CreateBiTree(&T);
- NextOrderTravel(T);
- printf("\n-------\n");
- char *str1 = "AB#D##C##";
- Create(&T,str1);
- NextOrderTravel(T);
- }
#include <stdio.h>
#include <stdlib.h>
typedef char ElemType;
// 线索存储标志位
// Link(0):表示指向左右孩子的指针
// Thread(1):表示指向前驱后继的线索
typedef enum {Link, Thread} PointerTag;
typedef struct BiThrNode
{
char data;
struct BiThrNode *lchild, *rchild;
PointerTag ltag;
PointerTag rtag;
} BiThrNode, *BiThrTree;
// 全局变量,始终指向刚刚访问过的结点
BiThrTree pre;
// 创建一棵二叉树,约定用户遵照前序遍历的方式输入数据
void CreateBiThrTree( BiThrTree *T )
{
char c;
scanf("%c", &c);
if( '#' == c )
{
*T = NULL;
}
else
{
*T = (BiThrNode *)malloc(sizeof(BiThrNode));
(*T)->data = c;
(*T)->ltag = Link;
(*T)->rtag = Link;
CreateBiThrTree(&(*T)->lchild);
CreateBiThrTree(&(*T)->rchild);
}
}
// 中序遍历线索化
void InThreading(BiThrTree T)
{
if( T )
{
InThreading( T->lchild ); // 递归左孩子线索化
if( !T->lchild ) // 如果该结点没有左孩子,设置ltag为Thread,并把lchild指向刚刚访问的结点。
{
T->ltag = Thread;
T->lchild = pre;
}
if( !pre->rchild )
{
pre->rtag = Thread;
pre->rchild = T;
}
pre = T;
InThreading( T->rchild ); // 递归右孩子线索化
}
}
void InOrderThreading( BiThrTree *p, BiThrTree T )
{
*p = (BiThrTree)malloc(sizeof(BiThrNode));
(*p)->ltag = Link;
(*p)->rtag = Thread;
(*p)->rchild = *p;
if( !T )
{
(*p)->lchild = *p;
}
else
{
(*p)->lchild = T;
pre = *p;
InThreading(T);
pre->rchild = *p;
pre->rtag = Thread;
(*p)->rchild = pre;
}
}
void visit( char c )
{
printf("%c", c);
}
// 中序遍历二叉树,非递归
void InOrderTraverse( BiThrTree T )
{
BiThrTree p;
p = T->lchild;
while( p != T )
{
while( p->ltag == Link )
{
p = p->lchild;
}
visit(p->data);
while( p->rtag == Thread && p->rchild != T )
{
p = p->rchild;
visit(p->data);
}
p = p->rchild;
}
}
int main()
{
BiThrTree P, T = NULL;
CreateBiThrTree( &T );
InOrderThreading( &P, T );
printf("中序遍历输出结果为: ");
InOrderTraverse( P );
printf("\n");
return 0;
}
- #include <stdio.h>
- #include <stdlib.h>
- typedef char ElemType;
- // 线索存储标志位
- // Link(0):表示指向左右孩子的指针
- // Thread(1):表示指向前驱后继的线索
- typedef enum {Link, Thread} PointerTag;
- typedef struct BiThrNode
- {
- char data;
- struct BiThrNode *lchild, *rchild;
- PointerTag ltag;
- PointerTag rtag;
- } BiThrNode, *BiThrTree;
- // 全局变量,始终指向刚刚访问过的结点
- BiThrTree pre;
- // 创建一棵二叉树,约定用户遵照前序遍历的方式输入数据
- void CreateBiThrTree( BiThrTree *T )
- {
- char c;
- scanf("%c", &c);
- if( '#' == c )
- {
- *T = NULL;
- }
- else
- {
- *T = (BiThrNode *)malloc(sizeof(BiThrNode));
- (*T)->data = c;
- (*T)->ltag = Link;
- (*T)->rtag = Link;
- CreateBiThrTree(&(*T)->lchild);
- CreateBiThrTree(&(*T)->rchild);
- }
- }
- // 中序遍历线索化
- void InThreading(BiThrTree T)
- {
- if( T )
- {
- InThreading( T->lchild ); // 递归左孩子线索化
- if( !T->lchild ) // 如果该结点没有左孩子,设置ltag为Thread,并把lchild指向刚刚访问的结点。
- {
- T->ltag = Thread;
- T->lchild = pre;
- }
- if( !pre->rchild )
- {
- pre->rtag = Thread;
- pre->rchild = T;
- }
- pre = T;
- InThreading( T->rchild ); // 递归右孩子线索化
- }
- }
- void InOrderThreading( BiThrTree *p, BiThrTree T )
- {
- *p = (BiThrTree)malloc(sizeof(BiThrNode));
- (*p)->ltag = Link;
- (*p)->rtag = Thread;
- (*p)->rchild = *p;
- if( !T )
- {
- (*p)->lchild = *p;
- }
- else
- {
- (*p)->lchild = T;
- pre = *p;
- InThreading(T);
- pre->rchild = *p;
- pre->rtag = Thread;
- (*p)->rchild = pre;
- }
- }
- void visit( char c )
- {
- printf("%c", c);
- }
- // 中序遍历二叉树,非递归
- void InOrderTraverse( BiThrTree T )
- {
- BiThrTree p;
- p = T->lchild;
- while( p != T )
- {
- while( p->ltag == Link )
- {
- p = p->lchild;
- }
- visit(p->data);
- while( p->rtag == Thread && p->rchild != T )
- {
- p = p->rchild;
- visit(p->data);
- }
- p = p->rchild;
- }
- }
- int main()
- {
- BiThrTree P, T = NULL;
- CreateBiThrTree( &T );
- InOrderThreading( &P, T );
- printf("中序遍历输出结果为: ");
- InOrderTraverse( P );
- printf("\n");
- return 0;
- }
#include<stdio.h>
#include<stdlib.h>
typedef int ElemType;
struct BTreeNode
{
ElemType data;
struct BTreeNode* left;
struct BTreeNode* right;
};
//1、输出二叉树,可在前序遍历的基础上修改。采用广义表格式,元素类型为int
void PrintBTree_int(struct BTreeNode* BT)
{
if (BT != NULL)
{
printf("%d", BT->data); //输出根结点的值
if (BT->left != NULL || BT->right != NULL)
{
printf("(");
PrintBTree_int(BT->left); //输出左子树
if (BT->right != NULL)
printf(",");
PrintBTree_int(BT->right); //输出右子树
printf(")");
}
}
}
//2、根据数组 a 中 n 个权值建立一棵哈夫曼树,返回树根指针
struct BTreeNode* CreateHuffman(ElemType a[], int n)
{
int i, j;
struct BTreeNode **b, *q;
b = malloc(n*sizeof(struct BTreeNode));
for (i = 0; i < n; i++) //初始化b指针数组,使每个指针元素指向a数组中对应的元素结点
{
b[i] = malloc(sizeof(struct BTreeNode));
b[i]->data = a[i];
b[i]->left = b[i]->right = NULL;
}
for (i = 1; i < n; i++)//进行 n-1 次循环建立哈夫曼树
{
//k1表示森林中具有最小权值的树根结点的下标,k2为次最小的下标
int k1 = -1, k2;
for (j = 0; j < n; j++)//让k1初始指向森林中第一棵树,k2指向第二棵
{
if (b[j] != NULL && k1 == -1)
{
k1 = j;
continue;
}
if (b[j] != NULL)
{
k2 = j;
break;
}
}
for (j = k2; j < n; j++)//从当前森林中求出最小权值树和次最小
{
if (b[j] != NULL)
{
if (b[j]->data < b[k1]->data)
{
k2 = k1;
k1 = j;
}
else if (b[j]->data < b[k2]->data)
k2 = j;
}
}
//由最小权值树和次最小权值树建立一棵新树,q指向树根结点
q = malloc(sizeof(struct BTreeNode));
q->data = b[k1]->data + b[k2]->data;
q->left = b[k1];
q->right = b[k2];
b[k1] = q;//将指向新树的指针赋给b指针数组中k1位置
b[k2] = NULL;//k2位置为空
}
free(b); //删除动态建立的数组b
return q; //返回整个哈夫曼树的树根指针
}
//3、求哈夫曼树的带权路径长度
ElemType WeightPathLength(struct BTreeNode* FBT, int len)//len初始为0
{
if (FBT == NULL) //空树返回0
return 0;
else
{
if (FBT->left == NULL && FBT->right == NULL)//访问到叶子结点
return FBT->data * len;
else //访问到非叶子结点,进行递归调用,返回左右子树的带权路径长度之和,len递增
return WeightPathLength(FBT->left,len+1)+WeightPathLength(FBT->right,len+1);
}
}
//4、哈夫曼编码(可以根据哈夫曼树带权路径长度的算法基础上进行修改)
void HuffManCoding(struct BTreeNode* FBT, int len)//len初始值为0
{
static int a[10];//定义静态数组a,保存每个叶子的编码,数组长度至少是树深度减一
if (FBT != NULL)//访问到叶子结点时输出其保存在数组a中的0和1序列编码
{
if (FBT->left == NULL && FBT->right == NULL)
{
int i;
printf("结点权值为%d的编码:", FBT->data);
for (i = 0; i < len; i++)
printf("%d", a[i]);
printf("\n");
}
else//访问到非叶子结点时分别向左右子树递归调用,并把分支上的0、1编码保存到数组a
{ //的对应元素中,向下深入一层时len值增1
a[len] = 0;
HuffManCoding(FBT->left, len + 1);
a[len] = 1;
HuffManCoding(FBT->right, len + 1);
}
}
}
//主函数
void main()
{
int n, i;
ElemType* a;
struct BTreeNode* fbt;
printf("从键盘输入待构造的哈夫曼树中带权叶子结点数n:");
while(1)
{
scanf("%d", &n);
if (n > 1)
break;
else
printf("重输n值:");
}
a = malloc(n*sizeof(ElemType));
printf("从键盘输入%d个整数作为权值:", n);
for (i = 0; i < n; i++)
scanf(" %d", &a[i]);
fbt = CreateHuffman(a, n);
printf("广义表形式的哈夫曼树:");
PrintBTree_int(fbt);
printf("\n");
printf("哈夫曼树的带权路径长度:");
printf("%d\n", WeightPathLength(fbt, 0));
printf("树中每个叶子结点的哈夫曼编码:\n");
HuffManCoding(fbt, 0);
}
- #include<stdio.h>
- #include<stdlib.h>
- typedef int ElemType;
- struct BTreeNode
- {
- ElemType data;
- struct BTreeNode* left;
- struct BTreeNode* right;
- };
- //1、输出二叉树,可在前序遍历的基础上修改。采用广义表格式,元素类型为int
- void PrintBTree_int(struct BTreeNode* BT)
- {
- if (BT != NULL)
- {
- printf("%d", BT->data); //输出根结点的值
- if (BT->left != NULL || BT->right != NULL)
- {
- printf("(");
- PrintBTree_int(BT->left); //输出左子树
- if (BT->right != NULL)
- printf(",");
- PrintBTree_int(BT->right); //输出右子树
- printf(")");
- }
- }
- }
- //2、根据数组 a 中 n 个权值建立一棵哈夫曼树,返回树根指针
- struct BTreeNode* CreateHuffman(ElemType a[], int n)
- {
- int i, j;
- struct BTreeNode **b, *q;
- b = malloc(n*sizeof(struct BTreeNode));
- for (i = 0; i < n; i++) //初始化b指针数组,使每个指针元素指向a数组中对应的元素结点
- {
- b[i] = malloc(sizeof(struct BTreeNode));
- b[i]->data = a[i];
- b[i]->left = b[i]->right = NULL;
- }
- for (i = 1; i < n; i++)//进行 n-1 次循环建立哈夫曼树
- {
- //k1表示森林中具有最小权值的树根结点的下标,k2为次最小的下标
- int k1 = -1, k2;
- for (j = 0; j < n; j++)//让k1初始指向森林中第一棵树,k2指向第二棵
- {
- if (b[j] != NULL && k1 == -1)
- {
- k1 = j;
- continue;
- }
- if (b[j] != NULL)
- {
- k2 = j;
- break;
- }
- }
- for (j = k2; j < n; j++)//从当前森林中求出最小权值树和次最小
- {
- if (b[j] != NULL)
- {
- if (b[j]->data < b[k1]->data)
- {
- k2 = k1;
- k1 = j;
- }
- else if (b[j]->data < b[k2]->data)
- k2 = j;
- }
- }
- //由最小权值树和次最小权值树建立一棵新树,q指向树根结点
- q = malloc(sizeof(struct BTreeNode));
- q->data = b[k1]->data + b[k2]->data;
- q->left = b[k1];
- q->right = b[k2];
- b[k1] = q;//将指向新树的指针赋给b指针数组中k1位置
- b[k2] = NULL;//k2位置为空
- }
- free(b); //删除动态建立的数组b
- return q; //返回整个哈夫曼树的树根指针
- }
- //3、求哈夫曼树的带权路径长度
- ElemType WeightPathLength(struct BTreeNode* FBT, int len)//len初始为0
- {
- if (FBT == NULL) //空树返回0
- return 0;
- else
- {
- if (FBT->left == NULL && FBT->right == NULL)//访问到叶子结点
- return FBT->data * len;
- else //访问到非叶子结点,进行递归调用,返回左右子树的带权路径长度之和,len递增
- return WeightPathLength(FBT->left,len+1)+WeightPathLength(FBT->right,len+1);
- }
- }
- //4、哈夫曼编码(可以根据哈夫曼树带权路径长度的算法基础上进行修改)
- void HuffManCoding(struct BTreeNode* FBT, int len)//len初始值为0
- {
- static int a[10];//定义静态数组a,保存每个叶子的编码,数组长度至少是树深度减一
- if (FBT != NULL)//访问到叶子结点时输出其保存在数组a中的0和1序列编码
- {
- if (FBT->left == NULL && FBT->right == NULL)
- {
- int i;
- printf("结点权值为%d的编码:", FBT->data);
- for (i = 0; i < len; i++)
- printf("%d", a[i]);
- printf("\n");
- }
- else//访问到非叶子结点时分别向左右子树递归调用,并把分支上的0、1编码保存到数组a
- { //的对应元素中,向下深入一层时len值增1
- a[len] = 0;
- HuffManCoding(FBT->left, len + 1);
- a[len] = 1;
- HuffManCoding(FBT->right, len + 1);
- }
- }
- }
- //主函数
- void main()
- {
- int n, i;
- ElemType* a;
- struct BTreeNode* fbt;
- printf("从键盘输入待构造的哈夫曼树中带权叶子结点数n:");
- while(1)
- {
- scanf("%d", &n);
- if (n > 1)
- break;
- else
- printf("重输n值:");
- }
- a = malloc(n*sizeof(ElemType));
- printf("从键盘输入%d个整数作为权值:", n);
- for (i = 0; i < n; i++)
- scanf(" %d", &a[i]);
- fbt = CreateHuffman(a, n);
- printf("广义表形式的哈夫曼树:");
- PrintBTree_int(fbt);
- printf("\n");
- printf("哈夫曼树的带权路径长度:");
- printf("%d\n", WeightPathLength(fbt, 0));
- printf("树中每个叶子结点的哈夫曼编码:\n");
- HuffManCoding(fbt, 0);
- }
附件列表
Chapter 6(树)的更多相关文章
- Chapter 3 树与二叉树
Chapter 3 树与二叉树 1- 二叉树 主要性质: 1 叶子结点数 = 度为2的结点数 + 1 2 二叉树第i层上最多有 (i≥1)个结点 3 深度为k的二叉树最多有 个结点 ...
- Render树、RenderObject与RenderLayer
Chapter: 呈现树的构建 1. 呈现树与CSS盒子模型千丝万缕的关系 2. 呈现树与DOM树的关系 3. 浏览器构建呈现树的流程 4. Firefox的规则树和样式上下文树 5. 规则树是如何解 ...
- 《Linux内核设计与实现》Chapter 3 读书笔记
<Linux内核设计与实现>Chapter 3 读书笔记 进程管理是所有操作系统的心脏所在. 一.进程 1.进程就是处于执行期的程序以及它所包含的资源的总称. 2.线程是在进程中活动的对象 ...
- Chapter 13. Miscellaneous PerlTk Methods PerlTk 方法杂项:
Chapter 13. Miscellaneous PerlTk Methods PerlTk 方法杂项: 到目前为止,这本书的大部分章节 集中在特定的几个部件, 这个章节覆盖了方法和子程序 可以被任 ...
- 《算法导论》 — Chapter 7 高速排序
序 高速排序(QuickSort)也是一种排序算法,对包括n个数组的输入数组.最坏情况执行时间为O(n^2). 尽管这个最坏情况执行时间比較差.可是高速排序一般是用于排序的最佳有用选择.这是由于其平均 ...
- Java编程思想总结笔记The first chapter
总觉得书中太啰嗦,看完总结后方便日后回忆,本想偷懒网上找别人的总结,无奈找不到好的,只好自食其力,尽量总结得最好. 第一章 对象导论 看到对象导论觉得这本书 目录: 1.1 抽象过程1.2 每个对象 ...
- 机器学习(Machine Learning)&深度学习(Deep Learning)资料(Chapter 2)
##机器学习(Machine Learning)&深度学习(Deep Learning)资料(Chapter 2)---#####注:机器学习资料[篇目一](https://github.co ...
- Formal Grammars of English -10 chapter(Speech and Language Processing)
determiner 限定词 DET propernoun 专有名词 NP (or noun phrase) mass noun 不可数名词 Det Nouns 限定词名词 relative pro ...
- 梦殇 chapter three
chapter three 悲伤有N个层面.对于生命是孤独的底色,对于时间是流动的伤感,对于浪漫是起伏的变奏,对于善和怜悯是终生的慨叹…… 出去和舍友买完东西,刚回到宿舍,舍友就说,刚才有人给你打电话 ...
随机推荐
- js中 null, undefined, 0,空字符串,false,不全等比较
null == undefined // true null == '' // false null == 0 // false null == false // false undefined = ...
- python的多路复用实现聊天群
在我的<python高级编程和异步io编程>中我讲解了socket编程,这里贴一段用socket实现聊天室的功能的源码,因为最近工作比较忙,后期我会将这里的代码细节分析出来,目前先把代码贴 ...
- Notes of Daily Scrum Meeting(11.3)
Notes of Daily Scrum Meeting(11.3) 2014年11月3日 星期一 20:00—20:30 团队成员 今日团队任务 当日工作分配额 完成情况 陈少杰 阅读理解代码中 ...
- Daily Scrumming 2015.10.21(Day 2)
今明两天任务表 Member Today’s Task Tomorrow’s Task 江昊 配置ruby与rails环境 配置mysql与数据库用户管理 配置apache2环境 学习rails Ac ...
- 2018软工实践—Alpha冲刺(10)
队名 火箭少男100 组长博客 林燊大哥 作业博客 Alpha 冲鸭鸭鸭鸭鸭鸭鸭鸭鸭鸭! 成员冲刺阶段情况 林燊(组长) 过去两天完成了哪些任务 协调各成员之间的工作 测试整体软件 展示GitHub当 ...
- 23_IO_第23天(字节流、字符流)_讲义
今日内容介绍 1.字节流 2.字符流 01输入和输出 * A:输入和输出 * a: 参照物 * 到底是输入还是输出,都是以Java程序为参照 * b: Output * 把内存中的数据存储到持久化设备 ...
- SQL Server 无法连接到本地服务器
未找到或无法访问服务器.请验证实例名称是否正确并且 SQL Server 已配置为允许远程连接: 解决办法: 在服务中启动SQL Server (MSSQLSERVER)这个服务.
- 2D变换
<!DOCTYPE html> <html> <head> <meta charset="UTF-8"> <title> ...
- ORACLE:一列的多行数据拼成字符串
查询表中的一个字段,返回了多行,就把这么多行的数据都拼成一个字符串. 例: id name 1 aa 2 bb 3 cc 要的结果是&quo ...
- 【Web Shell】- 技术剖析中国菜刀 - Part II
在第一部分,简单描述了中国菜刀的基本功能.本文我将剖析中国菜刀的平台多功能性.传输机制.交互模式和检测.我希望通过我的讲解,您能够根据您的环境检测出并清除它. 平台 那么中国菜刀可以在哪些平台上运行? ...