[ACM_数据结构] POJ2352 [树状数组稍微变形]
Description
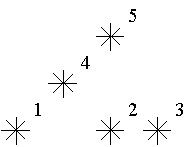
You are to write a program that will count the amounts of the stars of each level on a given map.
Input
Output
Sample Input
5
1 1
5 1
7 1
3 3
5 5
Sample Output
1
2
1
1
0
#include<iostream>
#include<cstdio>
#include<memory.h>
using namespace std;
#define maxn 32100//是叶节点能达到的最大值多一点
int C[maxn];//从1开始编号
//--------------------------
int lowbit(int x){
return x&-x;
}
int sum(int x){
int ret=;
while(x>){
ret+=C[x];
x-=lowbit(x);
}
return ret;
}
void add(int x,int d){
while(x<=maxn){
C[x]+=d;
x+=lowbit(x);
}
}
//--------------------------
/*
给定n个点,问这一点左下角的区域的点的个数,不包括这一点
因为输入是按照y从小到达,然后是按x从小到达,且x不同
因此先出现的肯定比后出现的y小即在后出现的点之下,
因此只要把x作为树状数组下标就可以啦。然后用一个out[num]维护
个数为num的点的数量
*/
int out[maxn];
int main(){
int n;
while(scanf("%d",&n)!=EOF){
int x,y;
int i,j;
memset(out,,sizeof(out));
memset(C,,sizeof(C));
for(i=;i<=n;i++){
scanf("%d%d",&x,&y);
out[sum(x+)]++;
add(x+,);
}
for(i=;i<n;i++){
printf("%d\n",out[i]);
}
}
return ;
}
[ACM_数据结构] POJ2352 [树状数组稍微变形]的更多相关文章
- Codeforces Round #248 (Div. 2) B称号 【数据结构:树状数组】
主题链接:http://codeforces.com/contest/433/problem/B 题目大意:给n(1 ≤ n ≤ 105)个数据(1 ≤ vi ≤ 109),当中有m(1 ≤ m ≤ ...
- poj2352树状数组
Astronomers often examine star maps where stars are represented by points on a plane and each star h ...
- poj2352树状数组解决偏序问题
树状数组解决这种偏序问题是很厉害的! /* 输入按照y递增,对于第i颗星星,它的level就是之前出现过的星星中,横坐标小于i的总数 */ #include<iostream> #incl ...
- 数据结构(树状数组):HEOI2012 采花
[题目描述] 萧薰儿是古国的公主,平时的一大爱好是采花. 今天天气晴朗,阳光明媚,公主清晨便去了皇宫中新建的花园采花.花园足够大,容纳了n朵花,花有c种颜色(用整数1-c表示),且花是排成一排的,以便 ...
- [poj 1533]最长上升子序列nlogn树状数组
题目链接:http://poj.org/problem?id=2533 其实这个题的数据范围n^2都可以过,只是为了练习一下nlogn的写法. 最长上升子序列的nlogn写法有两种,一种是变形的dp, ...
- 【poj 3167】Cow Patterns(字符串--KMP匹配+数据结构--树状数组)
题意:给2个数字序列 a 和 b ,问按从小到达排序后,a中的哪些子串与b的名次匹配. a 的长度 N≤100,000,b的长度 M≤25,000,数字的大小 K≤25. 解法:[思考]1.X 暴力. ...
- cdoj841-休生伤杜景死惊开 (逆序数变形)【线段树 树状数组】
http://acm.uestc.edu.cn/#/problem/show/841 休生伤杜景死惊开 Time Limit: 3000/1000MS (Java/Others) Memory ...
- 牛客练习赛22-E.简单数据结构1(扩展欧拉定理降幂 +树状数组)
链接:E.简单数据结构1 题意: 给一个长为n的序列,m次操作,每次操作: 1.区间加 2.对于区间,查询 ,一直到- 请注意每次的模数不同. 题解:扩展欧拉定理降幂 对一个数p取log(p)次的 ...
- 「BZOJ1537」Aut – The Bus(变形Dp+线段树/树状数组 最优值维护)
网格图给予我的第一反应就是一个状态 f[i][j] 表示走到第 (i,j) 这个位置的最大价值. 由于只能往下或往右走转移就变得显然了: f[i][j]=max{f[i-1][j], f[i][j-1 ...
随机推荐
- 把Excel导入SQL server时出现错误
在把Excel导入SQL server时出现“未在本地计算机上注册 Microsoft.ACE.OLEDB.12.0 ”该 错误信息:未在本地计算机上注册“microsoft.ACE.oledb.12 ...
- monkey初接触
第一次听说monkey,根本不知道是什么东西,脑海里就一个印象,很厉害的自动化测试工具,可是体验了一下,似乎不是那么回事... 一.Monkey 是什么? monkey就是SDK中附带的一个工具. 二 ...
- Intersection(Check)
Intersection http://poj.org/problem?id=1410 Time Limit: 1000MS Memory Limit: 10000K Total Submissi ...
- DOS中命令的格式
---------------siwuxie095 一.DOS中,命令使用格式的一般形式 用中文表达的形式为: [路径] 关键字 [盘符] [路径] 文件名 [扩展名] (参数) [参数 ...
- 获取客户端真实IP地址
Java-Web获取客户端真实IP: 发生的场景:服务器端接收客户端请求的时候,一般需要进行签名验证,客户端IP限定等情况,在进行客户端IP限定的时候,需要首先获取该真实的IP. 一般分为两种情况: ...
- Maven 学习笔记(一) 基础环境搭建
在Java的世界里,项目的管理与构建,有两大常用工具,一个是Maven,另一个是Gradle,当然,还有一个正在淡出的Ant.Maven 和 Gradle 都是非常出色的工具,排除个人喜好,用哪个工具 ...
- laravel加载视图
1.控制器 2.路由 3.视图
- 如何使用tapd?
tapd 可以编写测试用例 测试计划等 敏捷开发常用的工具.稍后会更新..
- fastjson解析List对象
List<String[]> body = JSON.parseObject(msg.getBody().toString(), new TypeToken<List<Stri ...
- Linux下JDK应该安装在哪个位置
在百度知道上看到的回答觉得不错:https://zhidao.baidu.com/question/1692690545668784588.html 如果你认为jdk是系统提供给你可选的程序,放在op ...