POJ:2049Finding Nemo(bfs+优先队列)
http://poj.org/problem?id=2049
Description
After checking the map, Marlin found that the sea is like a labyrinth with walls and doors. All the walls are parallel to the X-axis or to the Y-axis. The thickness of the walls are assumed to be zero.
All the doors are opened on the walls and have a length of 1. Marlin cannot go through a wall unless there is a door on the wall. Because going through a door is dangerous (there may be some virulent medusas near the doors), Marlin wants to go through as few doors as he could to find Nemo.
Figure-1 shows an example of the labyrinth and the path Marlin went through to find Nemo.
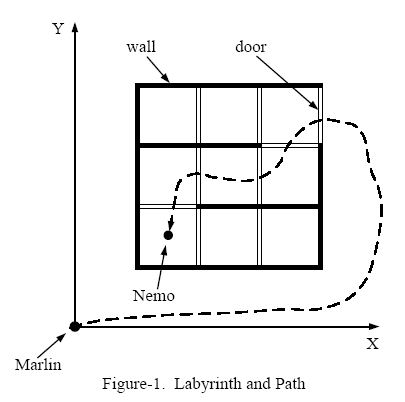
We assume Marlin's initial position is at (0, 0). Given the position of Nemo and the configuration of walls and doors, please write a program to calculate the minimum number of doors Marlin has to go through in order to reach Nemo.
Input
Then follow M lines, each containing four integers that describe a wall in the following format:
x y d t
(x, y) indicates the lower-left point of the wall, d is the direction of the wall -- 0 means it's parallel to the X-axis and 1 means that it's parallel to the Y-axis, and t gives the length of the wall.
The coordinates of two ends of any wall will be in the range of [1,199].
Then there are N lines that give the description of the doors:
x y d
x, y, d have the same meaning as the walls. As the doors have fixed length of 1, t is omitted.
The last line of each case contains two positive float numbers:
f1 f2
(f1, f2) gives the position of Nemo. And it will not lie within any wall or door.
A test case of M = -1 and N = -1 indicates the end of input, and should not be processed.
Output
Sample Input
8 9
1 1 1 3
2 1 1 3
3 1 1 3
4 1 1 3
1 1 0 3
1 2 0 3
1 3 0 3
1 4 0 3
2 1 1
2 2 1
2 3 1
3 1 1
3 2 1
3 3 1
1 2 0
3 3 0
4 3 1
1.5 1.5
4 0
1 1 0 1
1 1 1 1
2 1 1 1
1 2 0 1
1.5 1.7
-1 -1
Sample Output
5
-1 题目大意:有一个迷宫,在迷宫中有墙与门
有m道墙,每一道墙表示为(x,y,d,t)
x,y表示墙的起始坐标
d为0即向右t个单位,都是墙
d为1即向上t个单位,都是墙
有n道门,每一道门表示为(x,y,d)
x,y表示门的起始坐标
d为0即向右一个单位表示门
d为1即向上一个单位表示门
再给出你起点的位置(f1,f2),并保证这个点的位置不会再墙或者门中,为起点到(0,0)最少要穿过多少条门。
题目解析:
这个题忒坑,让它坑死了,首先这个地图可能完全由空格组成,所以要判断n==0&&m==0的状况,其次那个死孩子可能在地图外面,所以要判断孩子的位置。
解决方案:
如果坐标的位置不乘2的话,在给墙赋完值后,在给窗户赋值就把墙的值给覆盖了,所以坐标乘2进行处理。
刚开始对于孩子的位置坐标怎么处理没想明白,之后懂了,(int)x*2+1,(int)y*2+1是奇数,
而坐标乘2之后都是偶数,又因为孩子不在墙上与窗户上,所以(int)x*2+1,(int)y*2+1还是在原本孩子
呆的范围内。因为两墙之内最短的距离是2。
#include <iostream>
#include <stdlib.h>
#include <string.h>
#include <stdio.h>
#include <queue>
using namespace std;
int map[][],v[][];
int n,m,maxx,maxy;
float tx,ty;
struct node
{
int ans;
int x,y;
friend bool operator<(struct node a,struct node b)//按ans从小到大排序
{
return a.ans>b.ans;
}
};
struct node t,f;
int jx[]= {,-,,};
int jy[]= {,,,-};
void bfs(int ww,int ee)
{
priority_queue<node>q;
memset(v,,sizeof(v));
t.x=ww;
t.y=ee;
t.ans=;
q.push(t);
v[ww][ee]=;
while(!q.empty())
{
t=q.top();
q.pop();
if(t.x==&&t.y==)
{
printf("%d\n",t.ans);
return ;
}
for(int i=; i<; i++)
{
f.x=t.x+jx[i];
f.y=t.y+jy[i];
if(f.x>=&&f.x<=maxx&&f.y>=&&f.y<=maxy&&v[f.x][f.y]==&&map[f.x][f.y]!=)
{
if(map[f.x][f.y]==)
{
f.ans=t.ans;
}
else if(map[f.x][f.y]==)
{
f.ans=t.ans+;
}
q.push(f);
v[f.x][f.y]=;
}
}
}
printf("-1\n");
}
int main()
{
int xx,yy,zz,ww;
while(scanf("%d%d",&m,&n)!=EOF)
{
if(n==-&&m==-) break;
maxx=-;
maxy=-;
memset(map,,sizeof(map));
for(int i=; i<=m; i++)
{
scanf("%d%d%d%d",&xx,&yy,&zz,&ww);
if(zz==)
{
for(int j=xx*; j<=(xx+ww)*; j++)
{
map[j][yy*]=;//墙是2
}
if(maxx<(xx+ww)*)
maxx=(xx+ww)*;
if(maxy<yy*)
maxy=yy*;
}
else if(zz==)
{
for(int j=yy*; j<=(yy+ww)*; j++)
{
map[xx*][j]=;
}
if(maxy<(yy+ww)*)
maxy=(yy+ww)*;
if(maxx<xx*)
maxx=xx*;
}
}
for(int i=; i<=n; i++)
{
scanf("%d%d%d",&xx,&yy,&zz);
if(zz==)
{
map[xx*+][yy*]=;//路是1
}
else if(zz==)
{
map[xx*][yy*+]=;
}
}
scanf("%f%f",&tx,&ty);
if(!(tx>=&&tx<=&&ty>=&&ty<=))//他在迷宫之外
{
printf("0\n");
}
else if(!n&&!m)
printf("0\n");
else
{
int ww=(int)tx*+;
int ee=(int)ty*+;
maxx+=;
maxy+=;
bfs(ww,ee);
} }
return ;
}
POJ:2049Finding Nemo(bfs+优先队列)的更多相关文章
- POJ 1724 ROADS(BFS+优先队列)
题目链接 题意 : 求从1城市到n城市的最短路.但是每条路有两个属性,一个是路长,一个是花费.要求在花费为K内,找到最短路. 思路 :这个题好像有很多种做法,我用了BFS+优先队列.崔老师真是千年不变 ...
- Meteor Shower POJ - 3669 (bfs+优先队列)
Meteor Shower Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 26455 Accepted: 6856 De ...
- POJ - 2312 Battle City BFS+优先队列
Battle City Many of us had played the game "Battle city" in our childhood, and some people ...
- poj 3253 Fence Repair 优先队列
poj 3253 Fence Repair 优先队列 Description Farmer John wants to repair a small length of the fence aroun ...
- hdu 1242 找到朋友最短的时间 (BFS+优先队列)
找到朋友的最短时间 Sample Input7 8#.#####. //#不能走 a起点 x守卫 r朋友#.a#..r. //r可能不止一个#..#x.....#..#.##...##...#.... ...
- HDU 1428 漫步校园 (BFS+优先队列+记忆化搜索)
题目地址:HDU 1428 先用BFS+优先队列求出全部点到机房的最短距离.然后用记忆化搜索去搜. 代码例如以下: #include <iostream> #include <str ...
- hdu1839(二分+优先队列,bfs+优先队列与spfa的区别)
题意:有n个点,标号为点1到点n,每条路有两个属性,一个是经过经过这条路要的时间,一个是这条可以承受的容量.现在给出n个点,m条边,时间t:需要求在时间t的范围内,从点1到点n可以承受的最大容量... ...
- BFS+优先队列+状态压缩DP+TSP
http://acm.hdu.edu.cn/showproblem.php?pid=4568 Hunter Time Limit: 2000/1000 MS (Java/Others) Memo ...
- HDU 1242 -Rescue (双向BFS)&&( BFS+优先队列)
题目链接:Rescue 进度落下的太多了,哎╮(╯▽╰)╭,渣渣我总是埋怨进度比别人慢...为什么不试着改变一下捏.... 開始以为是水题,想敲一下练手的,后来发现并非一个简单的搜索题,BFS做肯定出 ...
随机推荐
- 【转载】Eclipse智能提示及快捷键
1.java智能提示 (1). 打开Eclipse,选择打开" Window - Preferences". (2). 在目录树上选择"Java-Editor-Conte ...
- C数组&结构体&联合体快速初始化
背景 C89标准规定初始化语句的元素以固定顺序出现,该顺序即待初始化数组或结构体元素的定义顺序. C99标准新增指定初始化(Designated Initializer),即可按照任意顺序对数组某些元 ...
- Geeks面试题:Min Cost Path
Min Cost Path Given a cost matrix cost[][] and a position (m, n) in cost[][], write a function tha ...
- 【大数据系列】HDFS安全模式
一.什么是安全模式 安全模式时HDFS所处的一种特殊状态,在这种状态下,文件系统只接受读数据请求,而不接受删除.修改等变更请求.在NameNode主节点启动时,HDFS首先进入安全模式,DataNod ...
- Linux(Ubuntu) 下如何解压 .tar.gz 文件
在终端输入以下命令即可解压: tar -zxvf YOUR_FILE_NAME.tar.gz 如果出现“权限不够”的错误提示,在命令前加上 sudo ,即 sudo tar -zxvf YOUR_FI ...
- jQuery事件处理(一)
1.jQuery事件绑定的用法: $( "elem" ).on( events, [selector], [data], handler ); events:事件名称,可以是自定义 ...
- Delete触发器
- Elasticsearch学习之查询去重
1. 实现查询去重.分页,例如:实现依据qid去重,createTime排序,命令行为: GET /nb_luban_answer/_search { "query": { &qu ...
- sencha touch 自定义cardpanel控件 模仿改进NavigationView 灵活添加按钮组,导航栏,自由隐藏返回按钮(废弃 仅参考)
最新版本我将会放在:http://www.cnblogs.com/mlzs/p/3382229.html这里的示例里面,这里不会再做更新 代码: /* *模仿且改进NavigationView *返回 ...
- jenkins部署war包到远程服务器的tomcat
一.目的 jenkins上将war包,部署到远程服务器的tomcat上. 这边tomcat在windows 主机A上,版本apache-tomcat-8.5.23. jenkins在主机B上,cent ...