POJ 2446 最小点覆盖
Time Limit: 2000MS | Memory Limit: 65536K | |
Total Submissions: 14787 | Accepted: 4607 |
Description
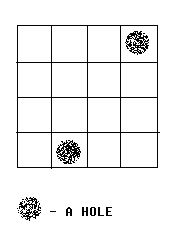
We call a grid, which doesn’t contain a hole, a normal grid. Bob has to follow the rules below:
1. Any normal grid should be covered with exactly one card.
2. One card should cover exactly 2 normal adjacent grids.
Some examples are given in the figures below:
A VALID solution.
An invalid solution, because the hole of red color is covered with a card.
An invalid solution, because there exists a grid, which is not covered.
Your task is to help Bob to decide whether or not the chessboard can be covered according to the rules above.
Input
Output
Sample Input
- 4 3 2
- 2 1
- 3 3
Sample Output
- YES
Hint
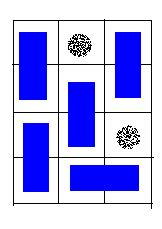
A possible solution for the sample input.
Source
- #include <cstdio>
- #include <cstring>
- #include <algorithm>
- #include <iostream>
- #include <vector>
- #include <queue>
- #include <cmath>
- #include <set>
- using namespace std;
- #define N 35
- int max(int x,int y){return x>y?x:y;}
- int min(int x,int y){return x<y?x:y;}
- int abs(int x,int y){return x<?-x:x;}
- int n, m;
- vector<int>ve[N*N];
- int from[N*N];
- bool visited[N*N];
- int march(int u){
- int i, v;
- for(i=;i<ve[u].size();i++){
- v=ve[u][i];
- if(!visited[v]){
- visited[v]=true;
- if(from[v]==-||march(from[v])){
- from[v]=u;
- return ;
- }
- }
- }
- return ;
- }
- int map[N][N];
- main()
- {
- int i, j, k;
- int x, y;
- while(scanf("%d %d %d",&n,&m,&k)==){
- memset(map,-,sizeof(map));
- int maxh=;
- for(i=;i<n;i++){
- for(j=;j<m;j++){
- if(j==){
- if(i==) map[i][j]=;
- else {
- if(m&) map[i][j]=map[i-][m-]+;
- else map[i][j]=map[i-][m-]+;
- }
- }
- else map[i][j]=map[i][j-]+;
- maxh=max(maxh,map[i][j]);
- // printf("%d ",map[i][j]);
- }
- //cout<<endl;
- }
- for(i=;i<k;i++){
- scanf("%d %d",&x,&y);
- map[y-][x-]=-;
- }
- int nn=;
- for(i=;i<n;i++){
- for(j=;j<m;j++){
- if(map[i][j]==-) nn++;
- }
- }
- for(i=;i<=maxh;i++) ve[i].clear();
- for(i=;i<n;i++){
- for(j=;j<m;j++){
- if(map[i][j]!=-&&(map[i][j]&)){
- if(i>&&map[i-][j]!=-) ve[map[i][j]].push_back(map[i-][j]);
- if(i<n-&&map[i+][j]!=-) ve[map[i][j]].push_back(map[i+][j]);
- if(j>&&map[i][j-]!=-) ve[map[i][j]].push_back(map[i][j-]);
- if(j<m-&&map[i][j+]!=-) ve[map[i][j]].push_back(map[i][j+]);
- }
- }
- }
- int num=;
- memset(from,-,sizeof(from));
- for(i=;i<=maxh;i++){
- memset(visited,false,sizeof(visited));
- if((i&)&&march(i)) num++;
- }
- if(num*==n*m-nn) printf("YES\n");
- else printf("NO\n");
- }
- }
POJ 2446 最小点覆盖的更多相关文章
- POJ 2226 最小点覆盖(经典建图)
Muddy Fields Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 8881 Accepted: 3300 Desc ...
- poj 3041 最小点覆盖=最大匹配
#include<stdio.h> #include<string.h> #define N 510 int map[N][N],n,mark[N],link[N]; in ...
- Poj(1325),最小点覆盖
题目链接:http://poj.org/problem?id=1325 Machine Schedule Time Limit: 1000MS Memory Limit: 10000K Total ...
- poj 1325 Machine Schedule 最小点覆盖
题目链接:http://poj.org/problem?id=1325 As we all know, machine scheduling is a very classical problem i ...
- POJ 2226 Muddy Fields (最小点覆盖集,对比POJ 3041)
题意 给出的是N*M的矩阵,同样是有障碍的格子,要求每次只能消除一行或一列中连续的格子,最少消除多少次可以全部清除. 思路 相当于POJ 3041升级版,不同之处在于这次不能一列一行全部消掉,那些非障 ...
- poj 3041 Asteroids(最小点覆盖)
http://poj.org/problem?id=3041 Asteroids Time Limit: 1000MS Memory Limit: 65536K Total Submissions ...
- POJ 3041 Asteroids (二分图最小点覆盖)
题目链接:http://poj.org/problem?id=3041 在一个n*n的地图中,有m和障碍物,你每一次可以消除一行或者一列的障碍物,问你最少消除几次可以将障碍物全部清除. 用二分图将行( ...
- [POJ] 2226 Muddy Fields(二分图最小点覆盖)
题目地址:http://poj.org/problem?id=2226 二分图的题目关键在于建图.因为“*”的地方只有两种木板覆盖方式:水平或竖直,所以运用这种方式进行二分.首先按行排列,算出每个&q ...
- 二分图 最小点覆盖 poj 3041
题目链接:Asteroids - POJ 3041 - Virtual Judge https://vjudge.net/problem/POJ-3041 第一行输入一个n和一个m表示在n*n的网格 ...
随机推荐
- jquery validate 自定义验证方法
query validate有很多验证规则,但是更多的时候,需要根据特定的情况进行自定义验证规则. 这里就来聊一聊jquery validate的自定义验证. jquery validate有一个方法 ...
- paper 106:图像增强方面的介绍
图像增强是从像素到像素的操作,是以预定的方式改变图像的灰度直方图.有时又称为对比度增强,灰度变换.点运算不可能改变图像内的空间关系,输出像素的灰度值由输入像素的值决定.其作用: 对比度增强:扩展感兴趣 ...
- 【002: NetBeans 的 代码补全】
在 代码完成中,输入如下内容 q;w;e;r;t;y;u;i;o;p;a;s;d;f;g;h;j;k;z;x;c;v;b;n;m;Q;W;E;R;T;Y;U;I;O;P;A;S;D;F;G;H;J;K ...
- Excel应该这么玩——1、命名单元格:干掉常数
命名单元格:通过名称来引用单元格中的值,常用于引用固定不变的值. 单元格是Excel中存储数据的最小单位,在公式中通过A1.B2之类的名称来引用其中的值.A1只是单元格的坐标,就好像人的身份证号.生活 ...
- innodb必收藏
http://www.xaprb.com/blog/2015/08/08/innodb-book-outline/
- C语言笔试常考知识点
1. const 关键字 a) const int a; b) int const a; c) const int *a; d) int * const a; e) int const * ...
- Postgresql 帐号密码修改方法
1.Linux环境下 #su postgres -bash-3.2$psql -U postgres postgres=#alter user postgres with password 'new ...
- HTML5新增元素、标签总结
总是遇到h5新标签的笔试题目,就查阅了资料来总结一下: 1.form相关: (1)form属性:在HTML5中表单元素可放在表单之外,通过给该元素添加form属性来指向目标表单(form属性值设为目标 ...
- WebRequest使用
// 待请求的地址 string url = "http://www.cnblogs.com"; // 创建 WebRequest 对象,WebRequest 是抽象类,定义了请求 ...
- easyui datagrid 行右键生成 动态获取(toolbar) 按钮
var createGridRowContextMenu = function(e, rowIndex, rowData) { e.preventDefault(); var grid = $(thi ...