poj2632 Crashing Robots
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 9859 | Accepted: 4209 |
Description
A robot crashes with a wall if it attempts to move outside the area of the warehouse, and two robots crash with each other if they ever try to occupy the same spot.
Input
The second line contains two integers, 1 <= N, M <= 100, denoting the numbers of robots and instructions respectively.
Then follow N lines with two integers, 1 <= Xi <= A, 1 <= Yi <= B and one letter (N, S, E or W), giving the starting position and direction of each robot, in order from 1 through N. No two robots start at the same position.
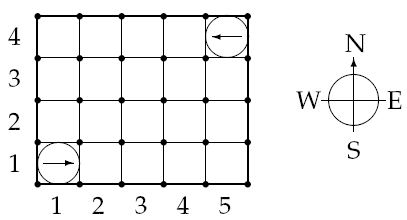
Figure 1: The starting positions of the robots in the sample warehouse
Finally there are M lines, giving the instructions in sequential order.
An instruction has the following format:
< robot #> < action> < repeat>
Where is one of
- L: turn left 90 degrees,
- R: turn right 90 degrees, or
- F: move forward one meter,
and 1 <= < repeat> <= 100 is the number of times the robot should perform this single move.
Output
- Robot i crashes into the wall, if robot i crashes into a wall. (A robot crashes into a wall if Xi = 0, Xi = A + 1, Yi = 0 or Yi = B + 1.)
- Robot i crashes into robot j, if robots i and j crash, and i is the moving robot.
- OK, if no crashing occurs.
Only the first crash is to be reported.
Sample Input
- 4
- 5 4
- 2 2
- 1 1 E
- 5 4 W
- 1 F 7
- 2 F 7
- 5 4
- 2 4
- 1 1 E
- 5 4 W
- 1 F 3
- 2 F 1
- 1 L 1
- 1 F 3
- 5 4
- 2 2
- 1 1 E
- 5 4 W
- 1 L 96
- 1 F 2
- 5 4
- 2 3
- 1 1 E
- 5 4 W
- 1 F 4
- 1 L 1
- 1 F 20
Sample Output
- Robot 1 crashes into the wall
- Robot 1 crashes into robot 2
- OK
- Robot 1 crashes into robot 2
Source
题目大意如下:给定几个机器人在一个房间里的坐标和面对的方向(东南西北),再给定几个移动(转几次方向或沿原方向前进其次),判断这些机器人是否会撞到房间墙壁或撞到互相或平安无事。
题解:模拟即可。把方向数组设成按顺时针或逆时针的顺序可缩减代码量。转方向时,注意mod4来减少要转圈数。
15775948 | ksq2013 | 2632 | Accepted | 732K | 0MS | G++ | 1985B | 2016-07-21 18:05:33 |
代码稍长:
- #include<cstdio>
- #include<cstring>
- #include<iostream>
- using namespace std;//横坐标x代表WE方向,纵坐标y代表NS方向;
- //const int mx[]={0,+1,-1,0,0};mov[1]->E,沿x轴向东/右移动+1;mov[2]->W,向西/左移动-1;mov[3]->N,沿x轴不移动;mov[4]->S,沿x轴不移动.
- //const int my[]={0,0,0,+1,-1};mov[1]->E,沿y轴不移动;mov[2]->W,沿y轴不移动;mov[3]->N,沿y轴向北/上移动+1;mov[4]->S,沿y轴向南/下移动-1.
- //顺序:东南西北;从0开始;
- const int mx[]={+1,0,-1,0};
- const int my[]={0,-1,0,+1};
- int n,m,p,q,mp[101][101];
- struct robot{
- int x,y,z;
- }rt[101];
- struct req{
- int k,rep;
- char pos;
- }rq[101];
- void Init()
- {
- scanf("%d%d%d%d",&n,&m,&p,&q);
- for(int i=1;i<=p;i++){
- char z;
- scanf("%d%d",&rt[i].x,&rt[i].y);
- getchar();
- z=getchar();
- mp[rt[i].x][rt[i].y]=i;
- switch(z){
- case 'E':rt[i].z=0;break;
- case 'S':rt[i].z=1;break;
- case 'W':rt[i].z=2;break;
- case 'N':rt[i].z=3;break;
- }
- }
- for(int i=1;i<=q;i++){
- scanf("%d",&rq[i].k);
- getchar();
- rq[i].pos=getchar();
- scanf("%d",&rq[i].rep);
- }
- }
- bool judge(int k)
- {
- if(rt[k].x<1||rt[k].x>n||rt[k].y<1||rt[k].y>m){
- printf("Robot %d crashes into the wall\n",k);
- return false;
- }
- if(mp[rt[k].x][rt[k].y]){
- printf("Robot %d crashes into robot %d\n",k,mp[rt[k].x][rt[k].y]);
- return false;
- }
- mp[rt[k].x][rt[k].y]=k;//修改移动后位置数据;
- return true;
- }
- bool solve()
- {
- for(int i=1;i<=q;i++){
- int k=rq[i].k;
- switch(rq[i].pos){
- case 'F':
- for(int j=1;j<=rq[i].rep;j++){
- mp[rt[k].x][rt[k].y]=0;//清空原位置数据;
- rt[k].x+=mx[rt[k].z];
- rt[k].y+=my[rt[k].z];
- if(!judge(k))return false;
- }
- break;
- case 'L':rt[k].z=(rt[k].z-rq[i].rep%4+4)%4;break;
- case 'R':rt[k].z=(rt[k].z+rq[i].rep%4)%4;break;
- }
- }
- return true;
- }
- int main()
- {
- int T;
- scanf("%d",&T);
- while(T--){
- memset(mp,0,sizeof(mp));
- Init();
- if(solve())puts("OK");
- }
- return 0;
- }
poj2632 Crashing Robots的更多相关文章
- POJ2632——Crashing Robots
Crashing Robots DescriptionIn a modernized warehouse, robots are used to fetch the goods. Careful pl ...
- POJ2632 Crashing Robots 解题报告
Description In a modernized warehouse, robots are used to fetch the goods. Careful planning is neede ...
- POJ2632 Crashing Robots(模拟)
题目链接. 分析: 虽说是简单的模拟,却调试了很长时间. 调试这么长时间总结来的经验: 1.坐标系要和题目建的一样,要不就会有各种麻烦. 2.在向前移动过程中碰到其他的机器人也不行,这个题目说啦:a ...
- POJ-2632 Crashing Robots模拟
题目链接: https://vjudge.net/problem/POJ-2632 题目大意: 在一个a×b的仓库里有n个机器人,编号为1到n.现在给出每一个机器人的坐标和它所面朝的方向,以及m条指令 ...
- Crashing Robots(imitate)
Crashing Robots Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 8124 Accepted: 3528 D ...
- 模拟 POJ 2632 Crashing Robots
题目地址:http://poj.org/problem?id=2632 /* 题意:几个机器人按照指示,逐个朝某个(指定)方向的直走,如果走过的路上有机器人则输出谁撞到:如果走出界了,输出谁出界 如果 ...
- Crashing Robots 分类: POJ 2015-06-29 11:44 10人阅读 评论(0) 收藏
Crashing Robots Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 8340 Accepted: 3607 D ...
- poj 2632 Crashing Robots
点击打开链接 Crashing Robots Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 6655 Accepted: ...
- Poj OpenJudge 百练 2632 Crashing Robots
1.Link: http://poj.org/problem?id=2632 http://bailian.openjudge.cn/practice/2632/ 2.Content: Crashin ...
随机推荐
- 请各位帮帮忙:Android LBS应用——CityExplorer (v1.0) 调研
Hello哇各位亲!! 请各位帮帮忙:Android LBS应用——CityExplorer(V1.0)调研 嗯,这个事情是这样的,要填一个调查问卷,但是问卷中部分问题是关于这个叫做CityExplo ...
- Android 网络图片查看器
今天来实现一下android下的一款简单的网络图片查看器 界面如下: 代码如下: <LinearLayout xmlns:android="http://schemas.android ...
- iOS 获取设备版本型号
#import "sys/utsname.h" /** * 设备版本 * * @return e.g. iPhone 5S */+ (NSString*)deviceVersi ...
- 【读书笔记】iOS网络-同步请求,队列式异步请求,异步请求的区别
一,同步请求的最佳实践. 1,只在后台过程中使用同步请求,除非确定访问的是本地文件资源,否则请不要在主线程上使用. 2,只有在知道返回的数据不会超出应用的内存时才使用同步请求.记住,整个响应体都会位于 ...
- CocoaPods的安装和使用那些事(Xcode 7.2,iOS 9.2,Swift)
Using The CocoaPods to Manage The Third Party Open-source Libaries 介绍 CocoaPods是用来管理你的Xcode项目的依赖库的.使 ...
- My97DatePicker时间控件使用
刚刚工作中遇到一个修改时间空间的bug,顺带学习了My97DatePicker时间空间 网上查到的资料已经很详细: http://www.360doc.com/content/14/0606/11/1 ...
- Ember.js 应用入口
大凡研究一套系统,调试一段代码,最先需要做的就是找到入口, 话说师傅领进门,修行在个人.找到入口,找到门,路就可以自己一步一步的往下走. Ember强大不? 强大! 好不? 看看流行度就知道了, 远比 ...
- Let's Encrypt 正式出發(免费HTTPS证书即将到来)
转自:https://blog.gslin.org/archives/2015/10/20/6073/lets-encrypt-%E6%AD%A3%E5%BC%8F%E5%87%BA%E7%99%BC ...
- 关于oracle数据库报12505错误的问题!
问题阐述: 导致oracle报12505错误的原因比较多,但是最可能一种原因就是客户端的监听出了问题. 解决办法: 在oracle安装目录下找到listener.ora 和 tnsnames.ora, ...
- Toritoisegit记住用户名密码
TortoiseGit每次连接git都得输入密码了,如果我们用到的比较频繁这样是很麻烦的,那么下面我们来看一篇关于window设置TortoiseGit连接git不用每次输入用户名和密码的配置,具体的 ...