线程池ThreadPoolExecutor与阻塞队列BlockingQueue应用
作者QQ:1095737364 QQ群:123300273 欢迎加入!
1.线程池介绍
public ThreadPoolExecutor(
int corePoolSize,
int maximumPoolSize,
long keepAliveTime,
TimeUnit unit,
BlockingQueue<Runnable> workQueue,
RejectedExecutionHandler handler
);
2.运行策略
3.测试示例
(1)LinkedBlockingQueue<Runnable>队列使用1:
package threadQueueTest;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.LinkedBlockingQueue;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
/**
* User: 杨永生
* Date: 15:47 2017/8/8
* Email: kevin@hiibook.com
*/
public class ThreadPoolTest implements Runnable {
public void run() {
synchronized(this) {
try{
System.out.println(Thread.currentThread().getName());
Thread.sleep(3000);
}catch (InterruptedException e){
e.printStackTrace();
}
}
} public static void main(String[] args) {
BlockingQueue<Runnable> queue = new LinkedBlockingQueue<Runnable>();
ThreadPoolExecutor executor = new ThreadPoolExecutor(2, 6, 1, TimeUnit.DAYS, queue);
for (int i = 0; i < 10; i++) {
executor.execute(new Thread(new ThreadPoolTest(),"TestThread".concat(""+i)));
int threadSize = queue.size();
System.out.println("线程队列大小为-->"+threadSize);
}
executor.shutdown();
}
}
(2)LinkedBlockingQueue<Runnable>队列使用2:
package threadQueueTest;
import java.util.concurrent.BlockingQueue;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.ThreadPoolExecutor;
import java.util.concurrent.TimeUnit;
/**
* User: 杨永生
* Date: 15:55 2017/8/8
* Email: kevin@hiibook.com
*/
public class ThreadPoolTest2 implements Runnable {
public void run() {
synchronized(this) {
try{
System.out.println("线程名称:"+Thread.currentThread().getName());
Thread.sleep(3000); //休眠是为了让该线程不至于执行完毕后从线程池里释放
}catch (InterruptedException e){
e.printStackTrace();
}
}
} public static void main(String[] args) throws InterruptedException {
BlockingQueue<Runnable> queue = new ArrayBlockingQueue<Runnable>(4); //固定为4的线程队列
ThreadPoolExecutor executor = new ThreadPoolExecutor(2, 6, 1, TimeUnit.DAYS, queue);
for (int i = 0; i < 10; i++) {
executor.execute(new Thread(new ThreadPoolTest2(), "TestThread".concat(""+i)));
int threadSize = queue.size();
System.out.println("线程队列大小为-->"+threadSize);
}
executor.shutdown();
}
}
(3)LinkedBlockingQueue<Runnable>队列使用3(测试异常):
public static void main(String[] args) throws InterruptedException {
BlockingQueue<Runnable> queue = new ArrayBlockingQueue<Runnable>(4); //固定为4的线程队列
ThreadPoolExecutor executor = new ThreadPoolExecutor(2, 6, 1, TimeUnit.DAYS, queue);
for (int i = 0; i < 11; i++) {
executor.execute(new Thread(new ThreadPoolTest2(), "TestThread".concat(""+i)));
int threadSize = queue.size();
System.out.println("线程队列大小为-->"+threadSize);
}
executor.shutdown();
}
(4)LinkedBlockingQueue<Runnable>队列使用3(测试add(E e)异常):
public static void main(String[] args) throws InterruptedException {
BlockingQueue<Runnable> queue = new ArrayBlockingQueue<Runnable>(4); //固定为4的线程队列
ThreadPoolExecutor executor = new ThreadPoolExecutor(2, 6, 1, TimeUnit.DAYS, queue);
for (int i = 0; i < 10; i++) {
executor.execute(new Thread(new ThreadPoolTest4(), "TestThread".concat(""+i)));
int threadSize = queue.size();
System.out.println("线程队列大小为-->"+threadSize);
if (threadSize==4){
queue.add(new Runnable() { //队列已满,抛异常
@Override
public void run(){
System.out.println("我是新线程,看看能不能搭个车加进去!"); }
});
}
}
executor.shutdown();
}
(5)LinkedBlockingQueue<Runnable>队列使用3(测试 offer(E e)异常):
public static void main(String[] args) throws InterruptedException {
BlockingQueue<Runnable> queue = new ArrayBlockingQueue<Runnable>(4); //固定为4的线程队列
ThreadPoolExecutor executor = new ThreadPoolExecutor(2, 6, 1, TimeUnit.DAYS, queue);
for (int i = 0; i < 10; i++) {
executor.execute(new Thread(new ThreadPoolTest5(), "TestThread".concat(""+i)));
int threadSize = queue.size();
System.out.println("线程队列大小为-->"+threadSize);
if (threadSize==4){
final boolean flag = queue.offer(new Runnable() {
@Override
public void run(){
System.out.println("我是新线程,看看能不能搭个车加进去!");
}
});
System.out.println("添加新线程标志为-->"+flag);
}
}
executor.shutdown();
}
(6)LinkedBlockingQueue<Runnable>队列使用3(测试put(E e)异常):
public static void main(String[] args) throws InterruptedException {
BlockingQueue<Runnable> queue = new ArrayBlockingQueue<Runnable>(4); //固定为4的线程队列
ThreadPoolExecutor executor = new ThreadPoolExecutor(2, 6, 1, TimeUnit.DAYS, queue);
for (int i = 0; i < 10; i++) {
executor.execute(new Thread(new ThreadPoolTest6(), "TestThread".concat(""+i)));
int threadSize = queue.size();
System.out.println("线程队列大小为-->"+threadSize);
if (threadSize==4){
queue.put(new Runnable() {
@Override
public void run(){
System.out.println("我是新线程,看看能不能搭个车加进去!");
}
});
}
}
executor.shutdown();
}
4.总结:
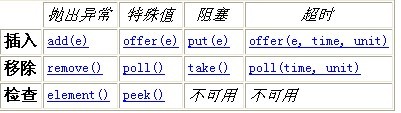
版权声明: 本文有 ```...裥簞點 发表于 bloghome博客
转载声明: 可自由转载、引用,但需要属名作者且注明文章出处。
线程池ThreadPoolExecutor与阻塞队列BlockingQueue应用的更多相关文章
- 转:JAVA线程池ThreadPoolExecutor与阻塞队列BlockingQueue
从Java5开始,Java提供了自己的线程池.每次只执行指定数量的线程,java.util.concurrent.ThreadPoolExecutor 就是这样的线程池.以下是我的学习过程. 首先是构 ...
- spring线程池ThreadPoolTaskExecutor与阻塞队列BlockingQueue
一: ThreadPoolTaskExecutor是一个spring的线程池技术,查看代码可以看到这样一个字段: private ThreadPoolExecutor threadPoolExecut ...
- java多线程:线程池原理、阻塞队列
一.线程池定义和使用 jdk 1.5 之后就引入了线程池. 1.1 定义 从上面的空间切换看得出来,线程是稀缺资源,它的创建与销毁是一个相对偏重且耗资源的操作,而Java线程依赖于内核线程,创建线程需 ...
- java线程(7)——阻塞队列BlockingQueue
回顾: 阻塞队列,英文名叫BlockingQueue.首先他是一种队列,联系之前Java基础--集合中介绍的Queue与Collection,我们就很容易开始今天的阻塞队列的学习了.来看一下他们的接口 ...
- 多线程学习笔记八之线程池ThreadPoolExecutor实现分析
目录 简介 继承结构 实现分析 ThreadPoolExecutor类属性 线程池状态 构造方法 execute(Runnable command) addWorker(Runnable firstT ...
- 21.线程池ThreadPoolExecutor实现原理
1. 为什么要使用线程池 在实际使用中,线程是很占用系统资源的,如果对线程管理不善很容易导致系统问题.因此,在大多数并发框架中都会使用线程池来管理线程,使用线程池管理线程主要有如下好处: 降低资源消耗 ...
- 常用阻塞队列 BlockingQueue 有哪些?
为什么要使用阻塞队列 之前,介绍了一下 ThreadPoolExecutor 的各参数的含义(并发编程之线程池ThreadPoolExecutor),其中有一个 BlockingQueue,它是一个阻 ...
- [转] 引用 Java自带的线程池ThreadPoolExecutor详细介绍说明和实例应用
PS: Spring ThreadPoolTaskExecutor vs Java Executorservice cachedthreadpool 引用 [轰隆隆] 的 Java自带的线程池Thre ...
- java线程API学习 线程池ThreadPoolExecutor(转)
线程池ThreadPoolExecutor继承自ExecutorService.是jdk1.5加入的新特性,将提交执行的任务在内部线程池中的可用线程中执行. 构造函数 ThreadPoolExecut ...
随机推荐
- 【Android Developers Training】 86. 基于连接类型修改您的下载模式
注:本文翻译自Google官方的Android Developers Training文档,译者技术一般,由于喜爱安卓而产生了翻译的念头,纯属个人兴趣爱好. 原文链接:http://developer ...
- android怎么输出信息到logcat
- java后端程序员1年工作经验总结
java后端1年经验和技术总结(1) 1.引言 毕业已经一年有余,这一年里特别感谢技术管理人员的器重,以及同事的帮忙,学到了不少东西.这一年里走过一些弯路,也碰到一些难题,也受到过做为一名开发却经常为 ...
- POJ 2502 Subway-经过预处理的最短路
Description You have just moved from a quiet Waterloo neighbourhood to a big, noisy city. Instead of ...
- voa 2015 / 4 / 15
illustrated - v. to explain or decorate a story, book, etc., with pictures pediatrician – n. a docto ...
- 参数错误。 (异常来自 HRESULT:0x80070057 (E_INVALIDARG))
异常来自 HRESULT:0x80070057 (E_INVALIDARG)未能加载程序集.......几次删除引用然后重新引用程序集还是报错 奔溃中....网上搜索还真有解决办法:解决方法 是 删除 ...
- css 定位属性position的使用方法实例-----一个层叠窗口
运行结果: <!DOCTYPE html> <html> <head> <title>重叠样式窗口</title> <style ty ...
- JS - dateFormat
// date必填, pattern默认'yyyy-MM-dd HH:mm:ss'function dateFormat (date, pattern) { var week = {'0':'日', ...
- Nancy基于JwtBearer认证的使用与实现
前言 最近在看JSON Web Token(Jwt)相关的东西,但是发现在Nancy中直接使用Jwt的组件比较缺乏,所以就在空闲时间写了一个. 这个组件是开源的,不过目前只支持.NET Core,后续 ...
- Spring定时器实现(二)
Spring结合quarzt可以实现更复杂的定时器,现做简单介绍相关配置: <?xml version="1.0" encoding="UTF-8"?&g ...