WPF之DataContext(转)
WPF之DataContext(转)
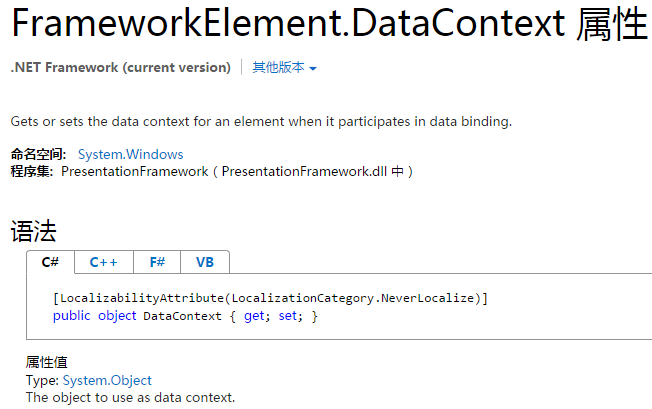
What is this “DataContext” you speak of?
I frequently see new WPF users confused about what the DataContext is, and how it is used. Hopefully, this can help clarify what the DataContext is, and how it is used.
What is the DataContext?
In WPF, there are two layers to an application: the UI layer and the Data layer.
The Data layer for an application starts out as null, and you can set it using the DataContext property. All UI objects will inherit their DataContext from their parent unless you specify otherwise.
When using the Model-View-ViewModel (MVVM) Design Pattern, the DataContext (Data Layer) is your application, while UI objects, like Buttons, Labels, DataGrids, and even Windows, are all just user-friendly items that allow a user to easily interact with the DataContext, which is your actual application and is typically comprised of ViewModels and Models.
How is it used
Whenever you do a basic binding in WPF, you are binding to the DataContext.
For example, when you write
< Label Name = "myLabel" Content = "{Binding Path=Name}" /> |
you are binding to myLabel.DataContext.Name, and not to myLabel.Name.
Other binding properties, such as ElementName or RelativeSource, can be used to tell the binding to lookup the property in something other than the current DataContext.
An Example
Lets start with a regular Window. Without setting the DataContext, the window still displays but there is no data behind it.
< Window x:Name = "MyWindow" ...> ... </ Window > |
Now suppose we set the DataContext to an object of type ClassA in the code-behind when this Window initializes:
public partial class MyWindow: Window { public MyWindow() { InitializeComponent(); this .DataContext = new ClassA(); } } |
Now the data layer behind that the Window is an object of type ClassA.
If ClassA has a property called Name, I could add a Label to the window and bind it to Name property of the DataContext, and whatever value is stored in ClassA.Name would get displayed.
< Window x:Name = "MyWindow" ...> < Label Content = "{Binding Name}" /> </ Window > |
Now, suppose ClassA has a property called ClassB, and both classes have a property called Name. Here is a block of XAML which illustrates how the DataContext works. It also includes an example of how a control would refer to a property not in its own DataContext.
<!-- DataContext set to ClassA in initialization code --> < Window x:Name = "MyWindow" > <!-- DataContext here is not specified, so it's inherited from its parent's DataContext, which is ClassA --> < StackPanel > <!-- DataContext inherited from parent, which is ClassA, so this will display ClassA.Name --> < Label Content = "{Binding Name}" /> <!-- DataContext is still ClassA, however we are setting it to ClassA.ClassB with a binding --> < StackPanel DataContext = "{Binding ClassB}" > <!-- DataContext inherited from parent, which is ClassB, so this will display ClassB.Name --> < Label Content = "{Binding Name}" /> <!-- DataContext is still ClassB, but we are binding to the Window's DataContext.Name, which is ClassA.Name --> < Label Content="{Binding ElementName = MyWindow , Path = DataContext .Name}" /> </ StackPanel > <!-- We've left the StackPanel with its DataContext bound to ClassB, so this Label's DataContext is ClassA (inherited from parent StackPanel), and we are binding to ClassA.ClassB.Name --> < Label Content = "{Binding ClassB.Name}" /> </ StackPanel > </ Window > |
As you can see, all the basic bindings look for their value in the data layer (DataContext) of the UI object
Summary
So to summarize, WPF applications have two layers: the UI layer and the Data layer. The data layer for an application starts out as null, and can be set using the DataContext property. UI objects without a DataContext set will inherit their data layer from their parent object. Bindings are used to look up values in the data layer, and display them in the UI layer.
When using the MVVM design pattern, the data layer is your application, while the UI layer just provides a user-friendly way to access the Data layer.
What is this “DataContext” you speak of?
I frequently see new WPF users confused about what the DataContext is, and how it is used. Hopefully, this can help clarify what the DataContext is, and how it is used.
What is the DataContext?
In WPF, there are two layers to an application: the UI layer and the Data layer.
The Data layer for an application starts out as null, and you can set it using the DataContext property. All UI objects will inherit their DataContext from their parent unless you specify otherwise.
When using the Model-View-ViewModel (MVVM) Design Pattern, the DataContext (Data Layer) is your application, while UI objects, like Buttons, Labels, DataGrids, and even Windows, are all just user-friendly items that allow a user to easily interact with the DataContext, which is your actual application and is typically comprised of ViewModels and Models.
How is it used
Whenever you do a basic binding in WPF, you are binding to the DataContext.
For example, when you write
< Label Name = "myLabel" Content = "{Binding Path=Name}" /> |
you are binding to myLabel.DataContext.Name, and not to myLabel.Name.
Other binding properties, such as ElementName or RelativeSource, can be used to tell the binding to lookup the property in something other than the current DataContext.
An Example
Lets start with a regular Window. Without setting the DataContext, the window still displays but there is no data behind it.
< Window x:Name = "MyWindow" ...> ... </ Window > |
Now suppose we set the DataContext to an object of type ClassA in the code-behind when this Window initializes:
public partial class MyWindow: Window { public MyWindow() { InitializeComponent(); this .DataContext = new ClassA(); } } |
Now the data layer behind that the Window is an object of type ClassA.
If ClassA has a property called Name, I could add a Label to the window and bind it to Name property of the DataContext, and whatever value is stored in ClassA.Name would get displayed.
< Window x:Name = "MyWindow" ...> < Label Content = "{Binding Name}" /> </ Window > |
Now, suppose ClassA has a property called ClassB, and both classes have a property called Name. Here is a block of XAML which illustrates how the DataContext works. It also includes an example of how a control would refer to a property not in its own DataContext.
<!-- DataContext set to ClassA in initialization code --> < Window x:Name = "MyWindow" > <!-- DataContext here is not specified, so it's inherited from its parent's DataContext, which is ClassA --> < StackPanel > <!-- DataContext inherited from parent, which is ClassA, so this will display ClassA.Name --> < Label Content = "{Binding Name}" /> <!-- DataContext is still ClassA, however we are setting it to ClassA.ClassB with a binding --> < StackPanel DataContext = "{Binding ClassB}" > <!-- DataContext inherited from parent, which is ClassB, so this will display ClassB.Name --> < Label Content = "{Binding Name}" /> <!-- DataContext is still ClassB, but we are binding to the Window's DataContext.Name, which is ClassA.Name --> < Label Content="{Binding ElementName = MyWindow , Path = DataContext .Name}" /> </ StackPanel > <!-- We've left the StackPanel with its DataContext bound to ClassB, so this Label's DataContext is ClassA (inherited from parent StackPanel), and we are binding to ClassA.ClassB.Name --> < Label Content = "{Binding ClassB.Name}" /> </ StackPanel > </ Window > |
As you can see, all the basic bindings look for their value in the data layer (DataContext) of the UI object
Summary
So to summarize, WPF applications have two layers: the UI layer and the Data layer. The data layer for an application starts out as null, and can be set using the DataContext property. UI objects without a DataContext set will inherit their data layer from their parent object. Bindings are used to look up values in the data layer, and display them in the UI layer.
When using the MVVM design pattern, the data layer is your application, while the UI layer just provides a user-friendly way to access the Data layer.
WPF之DataContext(转)的更多相关文章
- WPF之DataContext
1. 继承属性: DataContext is a property on FrameworkElement (base class for all WPF Controls) and is impl ...
- [WPF] How to bind to data when the datacontext is not inherited
原文:[WPF] How to bind to data when the datacontext is not inherited 原文地址:http://www.thomaslevesque.co ...
- WPF入门(1)——DataContext
在WPF中,应用程序有两层:UI层和Data层.这里新建一个项目说明哪些是UI层,哪些是数据层. UI层很明显,就是用户看到的界面.但是数据层并不是下图所示: 上图中是UI层view的后台代码.当然, ...
- 【我们一起写框架】MVVM的WPF框架(二)—绑定
MVVM的特点之一是实现数据同步,即,前台页面修改了数据,后台的数据会同步更新. 上一篇我们已经一起编写了框架的基础结构,并且实现了ViewModel反向控制Xaml窗体. 那么现在就要开始实现数据同 ...
- [No000012E]WPF(6/7):概念绑定
WPF 的体系结构,标记扩展,依赖属性,逻辑树/可视化树,布局,转换等.今天,我们将讨论 WPF 最重要的一部分——绑定.WPF 带来了优秀的数据绑定方式,可以让我们绑定数据对象,这样每次对象发生更改 ...
- 关于WPF中ItemsControl系列控件中Item不能继承父级的DataContext的解决办法
WPF中所有的集合类控件,子项都不能继承父级的DataContext,需要手动将绑定的数据源指向到父级控件才可以. <DataGridTemplateColumn Header="操作 ...
- WPF设置Window的数据上下文(DataContext)为自身
WPF设置Window的数据上下文(DataContext)为自身的XAML: DataContext="{Binding RelativeSource={RelativeSource Se ...
- WPF中 ItemsSource 和DataContext不同点
此段为原文翻译而来,原文地址 WPF 中 数据绑定 ItemSource和 DataContext的不同点: 1.DataContext 一般是一个非集合性质的对象,而ItemSource 更期望数据 ...
- WPF学习笔记——DataContext 与 ItemSource
作为一个WPF新手,在ListBox控件里,我分不清 DataContext 与 ItemSource的区别. 在实践中,似乎: <ListBox x:Name="Lst" ...
随机推荐
- PHPUnit简介及使用
一.PHPUnit是什么? 1.它是一款轻量级的PHP测试框架,地址:http://www.phpunit.cn 2.手册:http://www.phpunit.cn/manual/5.7/zh_cn ...
- 在C#中几种常见数组复制方法的效率对比
原文是在http://blog.csdn.net/jiangzhanchang/article/details/9998229 看到的,本文在原文基础上增加了新的方法,并对多种数据类型做了更全面的对比 ...
- Yii的HTML助手
Html 帮助类 基础 表单 样式表和脚本 超链接 图片 列表 任何一个 web 应用程序会生成很多 HTMl 超文本标记.如果超文本标记是静态的, 那么将 PHP 和 HTML 混合在一个文件里 这 ...
- HDU - 1847 巴什博弈
思路: 0 1 2 3 4 5 6 7 8 9 10 11 12 P N N P N N P N N P N N P 不难发现:当n为三的倍数时,KIKI ...
- 搭建多系统yum服务器
一.多系统服务器搭建 1.首先挂载光盘 2.安装vsftp 3.使用rpm -ql vsftpd查看vsftpd安装时都产生了哪些文件,找到以.server结尾的文件路径.此文件的文件名就是vsftp ...
- MS SQL 事务日志管理小结
本文是对SQL Server事务日志的总结,文章有一些内容和知识来源于官方文档或一些技术博客,本文对引用部分的出处都有标注. 事务日志介绍 在SQL Server中,事务日志是数据库的重要组件,如 ...
- 第3章 PCI总线的数据交换
PCI Agent设备之间,以及HOST处理器和PCI Agent设备之间可以使用存储器读写和I/O读写等总线事务进行数据传送.在大多数情况下,PCI桥不直接与PCI设备或者HOST主桥进行数据交换, ...
- spring schedule定时任务(二):配置文件的方式
接着上一篇,这里使用spring配置文件的方式生成spring定时任务. 1.相应的web.xml没有什么变化,因此便不再罗列.同样的,相应的java代码业务逻辑改动也不大,只是在原来的基础上去掉@C ...
- Android5.1系统WebView内存泄漏场景
问题现象 (该文章,引自零号路的私人博客,本人在浏览框架的开发过程中,用该方式,规避了内存泄露的问题.) 在Android5.1系统中,会发现App存在 WebView 泄漏情况,还比较严重.并且只是 ...
- dojo省份地市级联之省份封装类(一)
省份封装类 Province.java /** * 省份封装类 */ package com.you.model; import java.io.Serializable; /** * @author ...