rocketmq刷盘过程
public enum FlushDiskType {
// 同步刷盘
SYNC_FLUSH,
// 异步刷盘
ASYNC_FLUSH
}
线程服务 | 场景 | 写消息性能 |
CommitRealTimeService | 异步刷盘 && 开启内存字节缓冲区 | 第一 |
FlushRealTimeService | 异步刷盘 | 第二 |
GroupCommitService | 同步刷盘 | 第三 |
其中CommitRealTimeService是老一些版本中没有的,它为开启内存字节缓存的刷盘服务。
介绍各个线程工作之前,先需要重点了解一下waitForRunning方法,因为在三个刷盘服务线程中都频繁使用该方法:
protected void waitForRunning(long interval) {
if (hasNotified.compareAndSet(true, false)) {
this.onWaitEnd();
return;
}
//entry to wait
waitPoint.reset(); try {
waitPoint.await(interval, TimeUnit.MILLISECONDS);
} catch (InterruptedException e) {
log.error("Interrupted", e);
} finally {
hasNotified.set(false);
this.onWaitEnd();
}
}
shutdown()
stop()
wakeup()
这里我们关心的是wakeup()方法,调用wakeup方法的几处如下
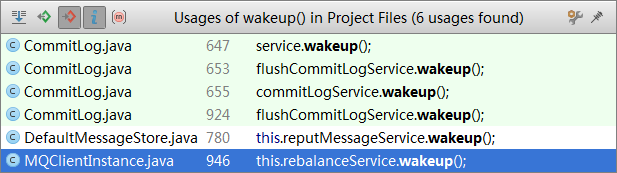
public void run() {
CommitLog.log.info(this.getServiceName() + " service started"); while (!this.isStopped()) {
try {
this.waitForRunning(10);
this.doCommit();
} catch (Exception e) {
CommitLog.log.warn(this.getServiceName() + " service has exception. ", e);
}
} // Under normal circumstances shutdown, wait for the arrival of the
// request, and then flush
try {
Thread.sleep(10);
} catch (InterruptedException e) {
CommitLog.log.warn("GroupCommitService Exception, ", e);
} synchronized (this) {
this.swapRequests();
} this.doCommit(); CommitLog.log.info(this.getServiceName() + " service end");
}
if (FlushDiskType.SYNC_FLUSH == this.defaultMessageStore.getMessageStoreConfig().getFlushDiskType()) {
final GroupCommitService service = (GroupCommitService) this.flushCommitLogService;
if (messageExt.isWaitStoreMsgOK()) {
GroupCommitRequest request = new GroupCommitRequest(result.getWroteOffset() + result.getWroteBytes());
service.putRequest(request);
boolean flushOK = request.waitForFlush(this.defaultMessageStore.getMessageStoreConfig().getSyncFlushTimeout());
if (!flushOK) {
log.error("do groupcommit, wait for flush failed, topic: " + messageExt.getTopic() + " tags: " + messageExt.getTags()
+ " client address: " + messageExt.getBornHostString());
putMessageResult.setPutMessageStatus(PutMessageStatus.FLUSH_DISK_TIMEOUT);
}
} else {
service.wakeup();
}
}
这条消息是否已经刷盘成功进行汇报的逻辑 -- waitForFlush方法:
public static class GroupCommitRequest {
private final long nextOffset;
private final CountDownLatch countDownLatch = new CountDownLatch(1);
private volatile boolean flushOK = false; public GroupCommitRequest(long nextOffset) {
this.nextOffset = nextOffset;
} public long getNextOffset() {
return nextOffset;
} public void wakeupCustomer(final boolean flushOK) {
this.flushOK = flushOK;
this.countDownLatch.countDown();
} public boolean waitForFlush(long timeout) {
try {
// 阻塞当前工作线程,等待时间5s后或者countDownLatch记数为0时,停止阻塞,执行下一条语句
this.countDownLatch.await(timeout, TimeUnit.MILLISECONDS);
return this.flushOK;
} catch (InterruptedException e) {
log.error("Interrupted", e);
return false;
}
}
}
private void swapRequests() {
List<GroupCommitRequest> tmp = this.requestsWrite;
this.requestsWrite = this.requestsRead;
this.requestsRead = tmp;
}
private void doCommit() {
//
synchronized (this.requestsRead) {
if (!this.requestsRead.isEmpty()) {
for (GroupCommitRequest req : this.requestsRead) {
// There may be a message in the next file, so a maximum of
// two times the flush
boolean flushOK = false;
for (int i = 0; i < 2 && !flushOK; i++) {
flushOK = CommitLog.this.mappedFileQueue.getFlushedWhere() >= req.getNextOffset(); if (!flushOK) {
CommitLog.this.mappedFileQueue.flush(0);
}
} req.wakeupCustomer(flushOK);
} long storeTimestamp = CommitLog.this.mappedFileQueue.getStoreTimestamp();
if (storeTimestamp > 0) {
CommitLog.this.defaultMessageStore.getStoreCheckpoint().setPhysicMsgTimestamp(storeTimestamp);
} this.requestsRead.clear();
} else {
// Because of individual messages is set to not sync flush, it
// will come to this process
CommitLog.this.mappedFileQueue.flush(0);
}
}
}
public boolean flush(final int flushLeastPages) {
boolean result = true;
MappedFile mappedFile = this.findMappedFileByOffset(this.flushedWhere, false);
if (mappedFile != null) {
long tmpTimeStamp = mappedFile.getStoreTimestamp();
int offset = mappedFile.flush(flushLeastPages);
long where = mappedFile.getFileFromOffset() + offset;
result = where == this.flushedWhere;
this.flushedWhere = where;
if (0 == flushLeastPages) {
this.storeTimestamp = tmpTimeStamp;
}
} return result;
}
commit
public boolean commit(final int commitLeastPages) {
boolean result = true;
MappedFile mappedFile = this.findMappedFileByOffset(this.committedWhere, false);
if (mappedFile != null) {
int offset = mappedFile.commit(commitLeastPages);
long where = mappedFile.getFileFromOffset() + offset;
result = where == this.committedWhere;
this.committedWhere = where;
} return result;
}
rocketmq刷盘过程的更多相关文章
- RocketMQ消息丢失解决方案:同步刷盘+手动提交
前言 之前我们一起了解了使用RocketMQ事务消息解决生产者发送消息时消息丢失的问题,但使用了事务消息后消息就一定不会丢失了吗,肯定是不能保证的. 因为虽然我们解决了生产者发送消息时候的消息丢失问题 ...
- RocketMQ中Broker的刷盘源码分析
上一篇博客的最后简单提了下CommitLog的刷盘 [RocketMQ中Broker的消息存储源码分析] (这篇博客和上一篇有很大的联系) Broker的CommitLog刷盘会启动一个线程,不停地 ...
- 【RocketMQ】消息的刷盘机制
刷盘策略 CommitLog的asyncPutMessage方法中可以看到在写入消息之后,调用了submitFlushRequest方法执行刷盘策略: public class CommitLog { ...
- HTC A510C电信手机刷机过程
HTC A510C电信手机刷机过程记录 Writed by Peter Hu(2014.6.7) ON WIN7_64 刷机需要的步骤: 1) 将S-ON加密保护式去掉,改成S-OFF模式,这样才能 ...
- mq刷盘方式
Broker 在收到Producer发送过来的消息后,会存入CommitLog对应的内存映射区中,见CommitLog类的putMessage方法.该方法执行OK后,会判断存储配置中刷盘模式:同步or ...
- Rocket重试机制,消息模式,刷盘方式
一.Consumer 批量消费(推模式) 可以通过 consumer.setConsumeMessageBatchMaxSize(10);//每次拉取10条 这里需要分为2种情况 Consumer端先 ...
- MySQL InnoDB 日志管理机制中的MTR和日志刷盘
1.MTR(mini-transaction) 在MySQL的 InnoDB日志管理机制中,有一个很重要的概念就是MTR.MTR是InnoDB存储擎中一个很重要的用来保证物理写的完整性和持久性的机制. ...
- 【软件安装与环境配置】TX2刷机过程
前言 使用TX2板子之前需要进行刷机,一般都是按照官网教程的步骤刷机,无奈买不起的宝宝只有TX2核心板,其他外设自己搭建,所以只能重新制作镜像,使用该镜像进行刷机. 系统需求 1.Host Platf ...
- 从CM刷机过程和原理分析Android系统结构
前面101篇文章都是分析Android系统源代码,似乎不够接地气. 假设能让Android系统源代码在真实设备上跑跑看效果,那该多好.这不就是传说中的刷ROM吗?刷ROM这个话题是老罗曾经一直避免谈的 ...
随机推荐
- mock的使名用一(生成随机数据)
Mock.Random 是一个工具类,用于生成各种随机数据. Mock.Random 的方法在数据模板中称为『占位符』,书写格式为 @占位符(参数 [, 参数]) . var Random = Moc ...
- struts 2整合spring要注意的问题(二)
在 struts2_spring_plugin.xml配置文件里有一个strus.objectFactory.spring.autoWire 属性 默认值为name 也就是说你不想装载.它都会找个 ...
- What is DB time in AWR?
AWR中有 DB time这个术语,那么什么是DB time呢? Oracle10gR2 官方文档 给出了详细解释(Oracle10gPerformance Tuning Guide 5.1.1.2 ...
- WaitHandle学习笔记
信号量与互斥体 互斥体(Mutex)是操作系统中一种独占访问共享资源的机制.它像一把所锁,哪个线程获取到互斥体的控制权,则可以访问共享的资源,或者执行处于受保护的代码.而其他的线程如果也想获取控制权, ...
- 【转载】细粒度图像识别Object-Part Attention Driven Discriminative Localization for Fine-grained Image Classification
细粒度图像识别Object-Part Attention Driven Discriminative Localization for Fine-grained Image Classificatio ...
- RK3288 HDMI增加特殊分辨率
转载请注明出处:https://www.cnblogs.com/lialong1st/p/9174475.html CPU:RK3288 系统:Android 5.1 本帖以 HDMI 800x600 ...
- 排序 第K大等问题总结
在公司面试时,当场写排序比较多,虽然都是老掉牙的问题,还是要好好准备下 快速排序,以第一个元素为关键词比较,每次比较结束,关键词都会去到最终位置上 //7 3 2 9 8 3 4 6 //7 3 2 ...
- 【转】用Jmeter制造测试数据
在平时的测试过程中,肯定会有碰到需要一批大量的数据的情况,如果这些数据本身没有太多的要求,或者说需求比较简单,可以通过简单的参数化实现的,推荐用Jmeter来造数据. 限制: Jmeter只能支持ja ...
- PTA 1004 Counting Leaves (30)(30 分)(dfs或者bfs)
1004 Counting Leaves (30)(30 分) A family hierarchy is usually presented by a pedigree tree. Your job ...
- [转][c#]注册表经验集
在 win7 64位的系统中,为了将程序做成绿化版(单EXE文件),一些设置准备放到 regedit(注册表)中. 测试时发现网上的 demo 可以读,但写的值调试不报错,相应位置却不存在,困扰了许久 ...