Spring+SpringMVC整合----配置文件
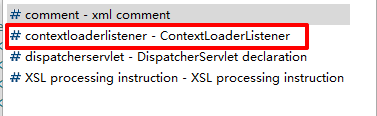
<!-- Spring -->
<context-param>
<param-name>contextConfigLocation</param-name>
<!-- 指定spring的配置文件的路径和名称 -->
<param-value>classpath:applicationContext.xml</param-value>
</context-param> <!-- Bootstraps the root web application context before servlet initialization -->
<listener>
<listener-class>org.springframework.web.context.ContextLoaderListener</listener-class>
</listener>

<!-- SpringMVC -->
<servlet>
<servlet-name>springDispatcherServlet</servlet-name>
<servlet-class>org.springframework.web.servlet.DispatcherServlet</servlet-class>
<init-param>
<param-name>contextConfigLocation</param-name>
<param-value>classpath:springmvc.xml</param-value>
</init-param>
<load-on-startup>1</load-on-startup>
</servlet> <!-- Map all requests to the DispatcherServlet for handling -->
<servlet-mapping>
<servlet-name>springDispatcherServlet</servlet-name>
<url-pattern>/</url-pattern>
</servlet-mapping>
3.在 web.xml 文件中设置处理POST请求乱码
<!-- 处理POST请求乱码 -->
<filter>
<filter-name>CharacterEncodingFilter</filter-name>
<filter-class>org.springframework.web.filter.CharacterEncodingFilter</filter-class>
<init-param>
<param-name>encoding</param-name>
<param-value>UTF-8</param-value>
</init-param>
</filter>
<filter-mapping>
<filter-name>CharacterEncodingFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
4.在 web.xml 文件中配置将POST请求转化为PUT、DELETE请求
<!-- 将POST请求转化为PUT、DELETE请求 -->
<filter>
<filter-name>HiddenHttpMethodFilter</filter-name>
<filter-class>org.springframework.web.filter.HiddenHttpMethodFilter</filter-class>
</filter>
<filter-mapping>
<filter-name>HiddenHttpMethodFilter</filter-name>
<url-pattern>/*</url-pattern>
</filter-mapping>
<context:component-scan base-package="com.neuedu" use-default-filters="false">
<context:include-filter type="annotation" expression="org.springframework.stereotype.Controller"/>
<context:include-filter type="annotation" expression="org.springframework.web.bind.annotation.ControllerAdvice"/>
</context:component-scan>
6.在 springmvc .xml 文件中配置视图解析器
<!-- 配置视图解析器 -->
<bean class="org.springframework.web.servlet.view.InternalResourceViewResolver">
<property name="prefix" value="WEB-INF/views/"></property>
<property name="suffix" value=".jsp"></property>
</bean>
<!-- 处理静态资源 -->
<mvc:default-servlet-handler/>
<mvc:annotation-driven/>
<context:component-scan base-package="com.neuedu">
<context:exclude-filter type="annotation" expression="org.springframework.stereotype.Controller"/>
<context:exclude-filter type="annotation" expression="org.springframework.web.bind.annotation.ControllerAdvice"/>
</context:component-scan>
9.在 bean.xml 文件中配置 jdbc.properties 及 C3P0 数据源
<!-- 加载外部属性文件 -->
<context:property-placeholder location="classpath:jdbc.properties"/>
<!-- 配置C3P0 数据源 -->
<bean id="comboPooledDataSource" class="com.mchange.v2.c3p0.ComboPooledDataSource">
<property name="user" value="${jdbc.username}"></property>
<property name="password" value="${jdbc.password}"></property>
<property name="driverClass" value="${jdbc.driver}"></property>
<property name="jdbcUrl" value="${jdbc.url}"></property>
</bean>
10.在 bean.xml 文件中配置 jdbcTemplate
<!-- 配置jdbcTemplate -->
<bean id="jdbcTemplate" class="org.springframework.jdbc.core.JdbcTemplate">
<property name="dataSource" ref="comboPooledDataSource"></property>
</bean>
11.在 bean.xml 文件中配置事务管理器及基于注解的事务
<!-- 配置事务管理器 -->
<bean id="dataSourceTransactionManager" class="org.springframework.jdbc.datasource.DataSourceTransactionManager">
<property name="dataSource" ref="comboPooledDataSource"></property>
</bean>
<!-- 开启基于注解的事务 -->
<tx:annotation-driven transaction-manager="dataSourceTransactionManager"/>
Spring+SpringMVC整合----配置文件的更多相关文章
- SpringMVC Spring MyBatis整合配置文件
1.spring管理SqlSessionFactory.mapper 1)在classpath下创建mybatis/sqlMapConfig.xml <?xml version="1. ...
- spring+springMVC 整合 MongoDB 实现注册登录
发现一入手 MongoDB,便无法脱离,简要说一下,MongoDB 是一个介于关系数据库和非关系数据库之间的产品,是非关系数据库当中功能最丰富,最像关系数据库的. 也是在 Nosql 中我最喜欢的一种 ...
- Spring+SpringMVC重复加载配置文件问题
sping+springmvc的框架中,IOC容器的加载过程 http://my.oschina.net/xianggao/blog/596476 基本上是先加载ContextLoaderListen ...
- 06_MyBatis,Spring,SpringMVC整合
项目结构 Spring的配置: beans.xml <?xml version="1.0" encoding="UTF-8"?> <be ...
- ssm整合(Spring+SpringMVC+Mybatis)
一.Spring Spring致力于提供一种方法管理你的业务对象.IOC容器,它可以装载bean(也就是我们java中的类,当然也包括service dao里面的),有了这个机制,我们就不用在每次使用 ...
- Spring Boot2 系列教程(二十四)Spring Boot 整合 Jpa
Spring Boot 中的数据持久化方案前面给大伙介绍了两种了,一个是 JdbcTemplate,还有一个 MyBatis,JdbcTemplate 配置简单,使用也简单,但是功能也非常有限,MyB ...
- spring+springMVC+mybatis框架整合——配置文件说明
如下图 web.xml配置说明: spring配置文件说明-1: spring配置文件说明-2: spring配置助记: 扫注(base) 读配(loc) 数据源(和comb(使用c3p0数据源)) ...
- Spring+SpringMVC+Mybaties整合之配置文件如何配置及内容解释--可直接拷贝使用--不定时更改之2017/4/27
以下配置可直接使用,只需更改包名. 关于内部标签的解释及用法,都以注解形式在代码内部说明.个人原创,转载需注明出处. 1,web.xml.添加jar包后首先需要配置WEB-INF下的web.xml文件 ...
- Eclipse Meaven Spring SpringMVC Mybaits整合
本示例是在:Ubuntu15上实现的:Windows上安装Maven将不太相同. Maven Install Run command sudo apt-get install maven, to in ...
随机推荐
- JZOJ 3875 星球联盟
[问题描述] 在遥远的 S 星系中一共有 N 个星球,编号为 1…N.其中的一些星球决定组成联盟, 以方便相互间的交流. 但是,组成联盟的首要条件就是交通条件.初始时,在这 N 个星球间有 M 条太空 ...
- 从零学习基于Python的RobotFramework自动化
从零学习基于Python的RobotFramework自动化 一. Python基础 1) 版本差异 版本 编码 语法 其他 2.X ASCII try: raise Type ...
- Java微服务(二):负载均衡、序列化、熔断
本文接着上一篇写的<Java微服务(二):服务消费者与提供者搭建>,上一篇文章主要讲述了消费者与服务者的搭建与简单的实现.其中重点需要注意配置文件中的几个坑. 本章节介绍一些零散的内容:服 ...
- Web安全 --Wfuzz 使用大全
前言: 做web渗透大多数时候bp来fuzz 偶尔会有觉得要求达不到的时候 wfuzz就很有用了这时候 用了很久了这点来整理一次 wfuzz 是一款Python开发的Web安全模糊测试工具. 下 ...
- socat的介绍与使用
Socat 是 Linux 下的一个多功能的网络工具,名字来由是 「Socket CAT」.其功能与有瑞士军刀之称的 Netcat 类似,可以看做是 Netcat 的加强版. Socat 的主要特点就 ...
- HTML DIV充满整个屏幕
<!DOCTYPE html> <html> <head> <title>A Little Game!</title> <meta c ...
- MySQL 数据库的设计规范
网址 :http://blog.csdn.net/yjjm1990/article/details/7525811 1.文档的建立日期.所属的单位.2.数据库的命名规范.视图.3.命名的规范:1)避免 ...
- [Abp vNext 源码分析] - 12. 后台作业与后台工作者
一.简要说明 文章信息: 基于的 ABP vNext 版本:1.0.0 创作日期:2019 年 10 月 24 日晚 更新日期:暂无 ABP vNext 提供了后台工作者和后台作业的支持,基本实现与原 ...
- 【Java必修课】各种集合类的合并(数组、List、Set、Map)
1 介绍 集合类可谓是学习必知.编程必用.面试必会的,而且集合的操作十分重要:本文主要讲解如何合并集合类,如合并两个数组,合并两个List等.通过例子讲解几种不同的方法,有JDK原生的方法,还有使用第 ...
- ios 11 系统CPU过高,xib中textfield使用导致出过高
ios11 发布之后,作为开发肯定是第一时间进行了升级测试,全新的系统不免会带来这样那样的问题.项目中使用xib的小伙伴们会发现,项目的cpu使用率非常高,尤其是初始化的时候,并没有线程的操作,CPU ...