digital clock based C
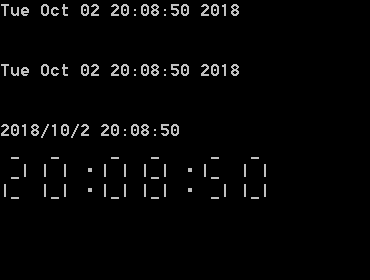
/**********************************
* Name : timeDisplay.cpp
* Purpose: Display digital clock according to current system time.
* Author : feicaixian
***********************************/ #include <stdio.h>
#include <time.h>
#include <stdlib.h>
void segDisp(int n);
void numToArr(int n, int *a); /***********************
* 7段码笔画编号,编号可以随意,不同编号方案对应不同7段码
* _0
* 5|_6| 1
* 4|_3| 2
*
***********************/
//将0~9的7段码存入数组,0表示不显示笔画,1表示显示笔画
const int segments[][] = {
{, , , , , , },//
{, , , , , , },//
{, , , , , , },//
{, , , , , , },//
{, , , , , , },//
{, , , , , , },//
{, , , , , , },//
{, , , , , , },//
{, , , , , , },//
{, , , , , , } //
}; int main()
{
/*
Use time() to get system time.The function prototype is:
#include <time.h>
time_t time(time_t *); function type is time_t, function name is time, parameter is pointer, whose type is time_t.
time(time_t *timer) returns
the number of seconds
between January 1, 1970 and the current system time
to timer, which can be print as long int.
*/
time_t t;//define a time_t type variable time(&t);//Pass time_t type pointer to time() printf("The number of seconds: %d\n",t);//print the seconds from January 1, 1970 to now. /*Use functions followed to convert seconds to a structure:
struct tm *gmtime(const time_t *timer); //convert seconds to Greenwich Mean Time(GMT)
struct tm *localtime(const time_t * timer); //convert seconds to localtime struct tm * means that the type of return value is a struct tm type pointer. struct tm
{
int tm_sec;//seconds 0-61
int tm_min;//minutes 1-59
int tm_hour;//hours 0-23
int tm_mday;//day of the month 1-31
int tm_mon;//months since jan 0-11, so add 1 to get the real months.
int tm_year;//years from 1900, add 1900 to get the real years.
int tm_wday;//days since Sunday, 0-6
int tm_yday;//days since Jan 1, 0-365
int tm_isdst;//Daylight Saving time indicator
}; */
struct tm *p = localtime(&t);//不一定要把当前系统时间传给指针,你也可以自定义各成员的值。
int oldSeconds; while() {
system("cls");//clear screen. /*
方法一:
char *asctime(const struct tm *timeptr) 返回一个表示当地时间的字符串,它代表了timeptr的日期和时间。
*/
printf("%s\n\n",asctime(p));//输出结果像这样:Sat Mar 25 06:10:10 1989 /*
方法二:
char *ctime(const time_t *timer) 返回一个表示当地时间的字符串,当地时间是基于参数 timer。
*/
printf("%s\n\n",ctime(&t));//输出结果和asctime一样 /*
方法三:
也可以自己输出日期和时间,自定义格式
*/
int a[];
int timeToInt = p->tm_hour * + p->tm_min * + p->tm_sec;
printf("%d/%d/%d ",+p->tm_year,p->tm_mon + , p->tm_mday);//date
numToArr(timeToInt, a);
printf("%d%d:%d%d:%d%d\n",a[],a[],a[],a[],a[],a[]); //使用7段码显示时间 segDisp(timeToInt); //不断获取当前时间,直到秒钟刷新,跳出循环,更新显示
oldSeconds = p->tm_sec;
do {
time(&t);
p = localtime(&t);
}while (p->tm_sec == oldSeconds);
}
return ;
} void numToArr(int n, int *a)
{
int k = ; //数组a[]的下标 do {
a[k++] = n % ;
n /= ;
}while (n != );
} void segDisp(int n)
{
int i; //打印7段码时形参n的各位的序号
int k = ; //数组a[]的下标
int a[]; //存储形参的各个位的数字
const int num = ;//时间显示的所需数字个数 //将形参n的各位存入数组a[]
numToArr(n, a); //-------------------------------------
//--- 按行显示数字的7段码,共3行。
//--- 第一行显示编号0,第二行显示编号5 6 1,第三行显示编号4 3 2.
//------------------------------------- //显示编号0
for (i = num-; i >= ; i--) {
if (i == | i == ){
//在第二个数字和第四个数字后面要显示冒号,如12:34:43。由于使用中文下的·号,采用全角模式,占两个字符宽度,
//所以额外增加两个空格。
if (segments[a[i]][])
printf(" _ ");
else
printf(" ");
}
else {
if (segments[a[i]][])
printf(" _ ");
//附加两个空格,显示下一个数字的笔画。
//第一个空格是因为本数字右边还有一个笔画,第二个空格是因为两个数字之间应有间隔。
else
printf(" ");//用空格代替下划线
}
}
printf("\n");//在下一行打印 //显示编号5 6 1
for (i = num-; i >= ; i--) {
if (segments[a[i]][])
printf("|");
else
printf(" ");
if (segments[a[i]][])
printf("_");
else
printf(" ");
if (segments[a[i]][])
printf("| ");
else
printf(" ");
if (i == | i == )
printf("·");//显示冒号
}
printf("\n");//在下一行打印 //显示编号4 3 2
for (i = num-; i >= ; i--) {
if (segments[a[i]][])
printf("|");
else
printf(" ");
if (segments[a[i]][])
printf("_");
else
printf(" ");
if (segments[a[i]][])
printf("| ");
else
printf(" ");
if (i == | i == )
printf("·");
}
}
digital clock based C的更多相关文章
- UVALive - 6269 Digital Clock 模拟
UVALive - 6269 Digital Clock 题意:时钟坏了,给你一段连续的时间,问你现在可能的时间是多少. 思路:直接模拟,他妈的居然这场就跪在了这题,卧槽,他妈的就在111行,居然多打 ...
- UVALive 6269 Digital Clock --枚举,模拟
题意:说不清楚,自己看吧,太恶心. 这题真是SB了,当时看了一下以为乱搞就好了,于是开始动手拍,结果拍了好几个小时都没拍出来,而且越想越想不通,直接把自己绕进去了,结果gg了. 总结:甭管什么题,想清 ...
- UVa 579 Clock Hands
水题.. 求任意时刻时针和分针的夹角,其结果在0°到180°之间. 这里又一次用到了sscanf()函数,确实很方便. 思路:我们分别求出时针和分针转过的角度,然后大的减小的,如果结果ans大于180 ...
- 40个新鲜的 jQuery 插件,使您的网站用户友好
作为最流行的 JavaScript 开发框架,jQuery 在现在的 Web 开发项目中扮演着重要角色,它简化了 HTML 文档遍历,事件处理,动画以及 Ajax 交互,这篇文章特别收集了40个新鲜的 ...
- Design Patterns Example Code (in C++)
Overview Design patterns are ways to reuse design solutions that other software developers have crea ...
- angularJS 杂
慎用ng-repeat 中的 $index http://web.jobbole.com/82470/ 服务provider,公共代码的抽象 (语法糖)分为: constant常量:constant初 ...
- Qt 之 数字钟
本例用来展示 QTimer 的使用,如何定时的更新一个窗口部件. 1 QLCDNumber 类 QLCDNumber 是一种可将数字显示为类似 LCD 形式的窗口部件,它同 QLabel 一样,都继 ...
- RAC的QA
RAC: Frequently Asked Questions [ID 220970.1] 修改时间 13-JAN-2011 类型 FAQ 状态 PUBLISHED Appli ...
- FPGA相关术语(一)
参考资料: 1. 数字时钟管理单元DCM 2. RS-232 知识点: ● Xilinx) Digital Clock Manager(DCM) primitive用于实现延迟锁相环(delay lo ...
随机推荐
- Unity中AndroidManifest增加权限,打开应用时不弹出权限申请
一 屏蔽第一次打开apk时权限弹窗: 在Activity下添加<meta-data android:name="unityplayer.SkipPermissionsDialog&qu ...
- HLAPI
和SPS硬件交互的API
- angular6 表单验证
这里使用的是模型驱动的表单 1.app.module.ts import { ReactiveFormsModule } from '@angular/forms'; @NgModule({ ... ...
- Jenkins连接Git仓库时候报错Permission denied, please try again.
一.连接GIT仓库报错 Failed to connect to repository : Command : stdout: stderr: Permission denied, please tr ...
- 【洛谷P5331】 [SNOI2019]通信
洛谷 题意: \(n\)个哨站排成一列,第\(i\)个哨站的频段为\(a_i\). 现在每个哨站可以选择: 直接连接到中心,代价为\(w\): 连接到前面某个哨站\(j(j<i)\),代价为\( ...
- 【OI备忘录】dalao博文收藏夹
[dalao学习笔记总览] [数学] 数论分块:数论分块 矩阵树定理Matrix_Tree:矩阵树Matrix-Tree定理与行列式 杨氏矩阵:杨氏矩阵和钩子公式 Hall定理:Hall定理学习小记 ...
- 201871010136 -赵艳强《面向对象程序设计(java)》第十六周学习总结
201871010136-赵艳强<面向对象程序设计(java)>第十六周学习总结 项目 内容 这个作业属于哪个课程 <任课教师博客主页链接>https://www.cnbl ...
- 算法竞赛入门经典 LA 4329(树状数组)
题意: 一排有着不同能力值的人比赛,规定裁判的序号只能在两人之间,而且技能值也只能在两人之间 问题: <算法竞赛入门经典-训练指南>的分析: 上代码: #include<iostre ...
- 错误: 找不到或无法加载主类 com.leyou.LeyouItemApplication Process finished with exit code 1
在IDEA的使用过程中,经常断掉服务或者重启服务,最近断掉服务重启时突然遇到了一个启动报错: 错误:找不到或无法加载主类 猜测:1,未能成功编译: 尝试:菜单--->Build--->Re ...
- 使用构造函数 Boolean 创造的对象不是布尔值,而是对象,typeof new Boolean(1) == 'object'
注意,使用构造函数 Boolean 创造的对象不是布尔值: 事实上 new Boolean() 返回的是一个 Boolean 对象: typeof new Boolean(1) == 'object' ...