leetcode 542. 01 Matrix 、663. Walls and Gates(lintcode) 、773. Sliding Puzzle 、803. Shortest Distance from All Buildings
542. 01 Matrix
https://www.cnblogs.com/grandyang/p/6602288.html
将所有的1置为INT_MAX,然后用所有的0去更新原本位置为1的值。
最短距离肯定使用bfs。
每次更新了值的地方还要再加入队列中 。
class Solution {
public:
vector<vector<int>> updateMatrix(vector<vector<int>>& matrix) {
int m = matrix.size(),n = matrix[].size();
queue<pair<int,int>> q;
for(int i = ;i < m;i++){
for(int j = ;j < n;j++){
if(matrix[i][j] == )
q.push(make_pair(i,j));
else
matrix[i][j] = INT_MAX;
}
}
while(!q.empty()){
auto coordinate = q.front();
q.pop();
int x = coordinate.first,y = coordinate.second;
for(auto dir : dirs){
int new_x = x + dir[];
int new_y = y + dir[];
if(new_x < || new_x >= matrix.size() || new_y < || new_y >= matrix[].size() || matrix[new_x][new_y] <= matrix[x][y] + )
continue;
matrix[new_x][new_y] = matrix[x][y] + ;
q.push(make_pair(new_x,new_y));
}
}
return matrix;
}
private:
vector<vector<int>> dirs{{-,},{,},{,-},{,}};
};
663. Walls and Gates
https://www.cnblogs.com/grandyang/p/5285868.html
这个题跟542. 01 Matrix很像,主要利用bfs。先把所有的0位置放入队列中,然后通过0更新周围的位置达到更新所有的位置。
class Solution {
public:
/**
* @param rooms: m x n 2D grid
* @return: nothing
*/
void wallsAndGates(vector<vector<int>> &rooms) {
// write your code here
queue<pair<int,int>> q;
for(int i = ;i < rooms.size();i++){
for(int j = ;j < rooms[].size();j++){
if(rooms[i][j] == )
q.push(make_pair(i,j));
}
}
while(!q.empty()){
int x = q.front().first;
int y = q.front().second;
q.pop();
for(auto dir : dirs){
int x_new = x + dir[];
int y_new = y + dir[];
if(x_new < || x_new >= rooms.size() || y_new < || y_new >= rooms[].size() || rooms[x_new][y_new] < rooms[x][y] + )
continue;
rooms[x_new][y_new] = rooms[x][y] + ;
q.push(make_pair(x_new,y_new));
}
}
return;
}
private:
vector<vector<int>> dirs{{-,},{,},{,-},{,}};
};
773. Sliding Puzzle
https://www.cnblogs.com/grandyang/p/8955735.html
queue里面存储的是每次变换后的board的样子和当前新的board中0所在的坐标。
将队列一次清空完了之后才能更新res,因为这就是一次变换。
用set相当于决定什么时候停止循环
class Solution {
public:
int slidingPuzzle(vector<vector<int>>& board) {
int res = ,m = board.size(),n = board[].size();
set<vector<vector<int>>> visited;
vector<vector<int>> correct{{,,},{,,}};
queue<pair<vector<vector<int>>,vector<int>>> q;
for(int i = ;i < m;i++){
for(int j = ;j < n;j++){
if(board[i][j] == ){
vector<int> tmp;
tmp.push_back(i);
tmp.push_back(j);
q.push(make_pair(board,tmp));
}
}
}
while(!q.empty()){
for(int i = q.size();i > ;i--){
int x = q.front().second[];
int y = q.front().second[];
vector<vector<int>> board_new = q.front().first;
if(board_new == correct)
return res;
visited.insert(board_new);
q.pop();
for(auto dir : dirs){
int new_x = x + dir[];
int new_y = y + dir[];
if(new_x < || new_x >= m || new_y < || new_y >= n)
continue;
vector<vector<int>> cand = board_new;
swap(cand[x][y],cand[new_x][new_y]);
if(visited.find(cand) != visited.end())
continue;
vector<int> tmp;
tmp.push_back(new_x);
tmp.push_back(new_y);
q.push(make_pair(cand,tmp));
}
}
res++;
}
return -;
}
private:
vector<vector<int>> dirs{{-,},{,},{,-},{,}};
};
注意犯的错误:
for(int i = q.size();i > 0;i--)不能写成for(int i = 0;i < q.size();i++),因为q的size在循环一次后,大小是缩小的,这样不能保证正确的次数。
803. Shortest Distance from All Buildings
https://www.cnblogs.com/grandyang/p/5297683.html
到所有建筑物的距离和最小,其实也就等于到每个建筑物最小距离的和。
以每个建筑物做bfs求最小的距离,然后用一个dis的vector保存,每当增加一个建筑物,在相应位置叠加当前建筑物的最小距离即可。
有可能有些点无法访问到某一些建筑物,所以通过一个count矩阵存储每个位置能访问到的建筑物的个数,最后再判断能否达到。
class Solution {
public:
/**
* @param grid: the 2D grid
* @return: the shortest distance
*/
int shortestDistance(vector<vector<int>> &grid) {
// write your code here
int m = grid.size(),n = grid[].size();
int res = INT_MAX,building = ;
vector<vector<int>> dist(m,vector<int>(n,));
vector<vector<int>> count = dist;
for(int i = ;i < m;i++){
for(int j = ;j < n;j++){
if(grid[i][j] == ){
building++;
queue<pair<int,int>> q;
vector<vector<bool>> visited(m,vector<bool>(n,false));
q.push(make_pair(i,j));
int pace = ;
while(!q.empty()){
pace++;
for(int i = q.size();i > ;i--){
int x = q.front().first;
int y = q.front().second;
q.pop();
for(auto dir : dirs){
int new_x = x + dir[];
int new_y = y + dir[];
if(new_x < || new_x >= m || new_y < || new_y >= n || visited[new_x][new_y]== true || grid[new_x][new_y] != )
continue;
dist[new_x][new_y] += pace;
count[new_x][new_y] += ;
visited[new_x][new_y] = true;
q.push(make_pair(new_x,new_y));
}
}
}
}
}
}
for(int i = ;i < m;i++){
for(int j = ;j < n;j++){
if(grid[i][j] == && count[i][j] == building)
res = min(res,dist[i][j]);
}
}
return res == INT_MAX ? - : res;
}
private:
vector<vector<int>> dirs{{-,},{,},{,-},{,}};
};
另一种写法:
class Solution {
public:
/**
* @param grid: the 2D grid
* @return: the shortest distance
*/
int shortestDistance(vector<vector<int> > &grid) {
// write your code here
int m = grid.size(),n = grid[].size();
vector<vector<int> > path(m,vector<int>(n,));
vector<vector<int> > count(m,vector<int>(n,));
int building = ;
for(int i = ;i < m;i++){
for(int j = ;j < n;j++){
if(grid[i][j] == ){
building++;
vector<vector<bool> > visited(m,vector<bool>(n,false));
search(grid,i,j,path,count,visited);
}
}
}
int res = INT_MAX;
for(int i = ;i < m;i++){
for(int j = ;j < n;j++){
if(grid[i][j] == && count[i][j] == building)
res = min(res,path[i][j]);
}
}
return res == INT_MAX ? - : res;
}
void search(vector<vector<int> > grid,int x,int y,vector<vector<int> >& path,vector<vector<int> >& count,vector<vector<bool> > visited){
queue<pair<int,int> > q;
q.push(make_pair(x,y));
int pace = ;
while(!q.empty()){
pace++;
for(int i = q.size();i > ;i--){
int x0 = q.front().first;
int y0 = q.front().second;
q.pop();
//visited[x0][y0] = true;
for(int j = ;j < dirs.size();j++){
int new_x = x0 + dirs[j][];
int new_y = y0 + dirs[j][];
if(new_x < || new_x >= path.size() || new_y < || new_y >= path[].size() || visited[new_x][new_y] || grid[new_x][new_y] != )
continue;
path[new_x][new_y] += pace;
count[new_x][new_y]++;
visited[new_x][new_y] = true;
q.push(make_pair(new_x,new_y));
}
}
}
}
private:
vector<vector<int> > dirs{{-,},{,},{,-},{,}};
}; //visited[new_x][new_y] = true;只能放在新生成的坐标的位置
leetcode 542. 01 Matrix 、663. Walls and Gates(lintcode) 、773. Sliding Puzzle 、803. Shortest Distance from All Buildings的更多相关文章
- [leetcode] 542. 01 Matrix (Medium)
给予一个矩阵,矩阵有1有0,计算每一个1到0需要走几步,只能走上下左右. 解法一: 利用dp,从左上角遍历一遍,再从右下角遍历一遍,dp存储当前位置到0的最短距离. 十分粗心的搞错了col和row,改 ...
- LeetCode 542. 01 Matrix
输入:只包含0,1的矩阵 输出:元素1到达最近0的距离 算法思想:广度优先搜索. 元素为0为可达区域,元素为1为不可达区域,我们的目标是为了从可达区域不断地扩展至不可达区域,在扩展的过程中,也就计算出 ...
- 【LeetCode】01 Matrix 解题报告
[LeetCode]01 Matrix 解题报告 标签(空格分隔): LeetCode 题目地址:https://leetcode.com/problems/01-matrix/#/descripti ...
- Java实现 LeetCode 542 01 矩阵(暴力大法,正反便利)
542. 01 矩阵 给定一个由 0 和 1 组成的矩阵,找出每个元素到最近的 0 的距离. 两个相邻元素间的距离为 1 . 示例 1: 输入: 0 0 0 0 1 0 0 0 0 输出: 0 0 0 ...
- [Leetcode Week10]01 Matrix
01 Matrix 题解 原创文章,拒绝转载 题目来源:https://leetcode.com/problems/01-matrix/description/ Description Given a ...
- [LeetCode] Shortest Distance from All Buildings 建筑物的最短距离
You want to build a house on an empty land which reaches all buildings in the shortest amount of dis ...
- [LeetCode] 317. Shortest Distance from All Buildings 建筑物的最短距离
You want to build a house on an empty land which reaches all buildings in the shortest amount of dis ...
- Leetcode 542.01矩阵
01矩阵 给定一个由 0 和 1 组成的矩阵,找出每个元素到最近的 0 的距离. 两个相邻元素间的距离为 1 . 示例 1: 输入: 0 0 0 0 1 0 0 0 0 输出: 0 0 0 0 1 0 ...
- 542 01 Matrix 01 矩阵
给定一个由 0 和 1 组成的矩阵,找出每个元素到最近的 0 的距离.两个相邻元素间的距离为 1 .示例 1:输入:0 0 00 1 00 0 0输出:0 0 00 1 00 0 0 示例 2:输入: ...
随机推荐
- Immediate Decodability UVA-644(qsort排序 + 模拟)
#include<iostream> #include<cstdio> #include<cstring> #include<algorithm> us ...
- Java精通并发-透过openjdk源码分析wait与notify方法的本地实现
上一次https://www.cnblogs.com/webor2006/p/11442551.html中通过openjdk从c++的底层来审视了ObjectMonitor的底层实现,这次继续来探究底 ...
- Dubbo源码分析(5):ExtensionLoader
背景 Dubbo所有的模块加载是基于SPI机制的.在接口名的上一行加个@SPI注解表明要此模块要通过ExtensionLoader加载.基于SPI机制的扩展性比较好,在不修改原有代码,可以实现新模块的 ...
- python - django (实现电子邮箱的账户注册和验证码功能)
使用 Django 来做一个电子邮箱注册 并 发送验证码的功能 (此处以 163 邮箱为例) 一. 登陆 163 邮箱账号, 然后进行下列操作 二. settings 配置文件 # 发送邮箱验证码 ...
- Zookeeper选举(fastleaderelection算法)
1.选举相关概念: 选票:(myid,zxid,当前节点选取轮次,被推举服务器选举轮次,状态(looking)). 选举发生情况:启动时选举,运行时选举. 外部投票:其他服务器发送来的投票. 内部投票 ...
- 当服务全部宕机时候的处理方法,md
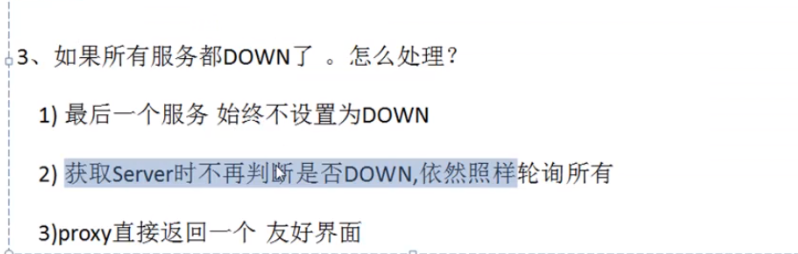 来自为知笔记(Wiz)
- Bootstrap是什么意思?
Bootstrap是一组用于网站和网络应用程序开发的开源前端(所谓“前端”,指的是展现给最终用户的界面.与之对应的“后端”是在服务器上面运行的代码)框架,包括HTML.CSS及JavaScript的框 ...
- mongodb 副本集的主的选举
primary的选举依赖于各个实例的优先权重,默认权重都是1 复本集的主挑选权重最高的,权重一样的无法控制谁为主 设置各个实例的优先权重,挑选自己想要的实例为主,只有primary可以更改权重配置 c ...
- SaltStack 在 Windows 上的操作基础
SaltStack 在 windows上的操作基础 1.删除文件: salt '172.16.3.11' file.remove 'D:\downup\111.msu' 2.删除文件夹 salt '1 ...
- PHP 源码安装常用配置参数和说明
常用的配置参数1. --prefix=/usr/local/php指定 php 安装目录install architecture-independent files in PREFIX 默认/usr/ ...