Spring MVC Hello World Example(转)
In Spring MVC web application, it consist of 3 standard MVC (Model, Views, Controllers) components :
- Models – Domain objects that are processed by service layer (business logic) or persistent layer (database operation).
- Views – Usually JSP page written with Java Standard Tag Library (JSTL).
- Controllers – Interact with service layer for business processing and return a Model.
See following figures 1.1, 1.2 to demonstrate how Spring MVC web application handle a web request.
Figure 1.1 – Image copied from Spring MVC reference with slightly modification.
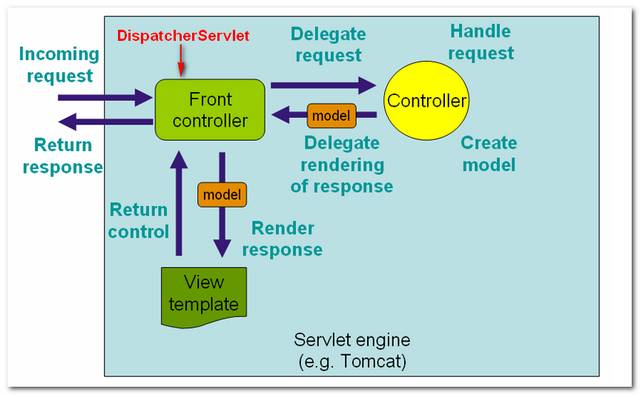
Figure 1.2 – P.S Image copied from book : Spring Recipes
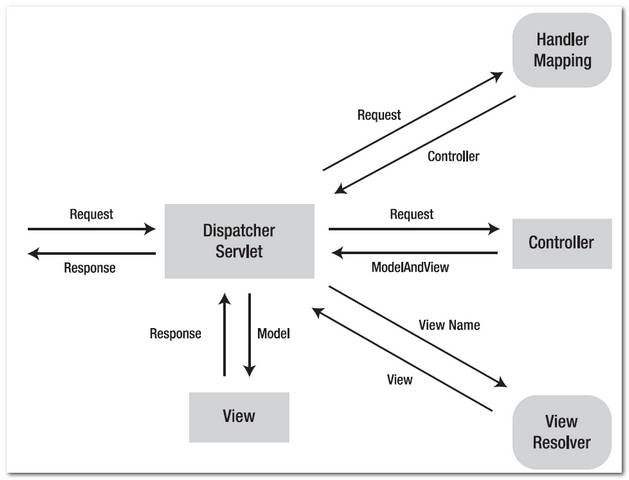
Spring MVC Tutorial
In this tutorial, you will create a simple Spring MVC hello world web application in order to understand the basic concepts and configurations of this framework.
Technologies used in this tutorial.
- Spring 2.5.6
- JDK 1.6
- Eclipse 3.6
- Maven 3
1. Directory Structure
Final directory structure of this tutorial.

2. Dependency library
Spring MVC required two core dependency libraries, spring-version.jar and spring-mvc-version.jar. If you are using JSP page with jstl, include the jstl.jar and standard.jar as well.
File : pom.xml
<!-- Spring framework -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring</artifactId>
<version>2.5.6</version>
</dependency>
<!-- Spring MVC framework -->
<dependency>
<groupId>org.springframework</groupId>
<artifactId>spring-webmvc</artifactId>
<version>2.5.6</version>
</dependency>
<!-- JSTL -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>jstl</artifactId>
<version>1.1.2</version>
</dependency>
<dependency>
<groupId>taglibs</groupId>
<artifactId>standard</artifactId>
<version>1.1.2</version>
</dependency>
<!-- for compile only, your container should have this -->
<dependency>
<groupId>javax.servlet</groupId>
<artifactId>servlet-api</artifactId>
<version>2.5</version>
<scope>provided</scope>
</dependency>
3. Spring Controller
Spring comes with many Controllers, normally, you just need to extend the AbstractController
, if you do not have other special requirement, and override the handleRequestInternal()
method and return a ModelAndView object.
File : HelloWorldController.java
package com.mkyong.common.controller;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import org.springframework.web.servlet.ModelAndView;
import org.springframework.web.servlet.mvc.AbstractController;
public class HelloWorldController extends AbstractController{
@Override
protected ModelAndView handleRequestInternal(HttpServletRequest request,
HttpServletResponse response) throws Exception {
ModelAndView model = new ModelAndView("HelloWorldPage");
model.addObject("msg", "hello world");
return model;
}
}
- ModelAndView(“HelloWorldPage”) – The “HelloWorldPage” will pass to Spring’s viewResolver later, to indentify which view should return back to the user. (see step 6)
- model.addObject(“msg”, “hello world”) – Add a “hello world” string into a model named “msg”, later you can use JSP EL ${msg} to display the “hello world” string.
4. View (JSP page)
In this case, “view” is a jSP page, you can display the value “hello world” that is store in the model “msg” via expression language (EL) ${msg}.
File : HelloWorldPage.jsp
<%@ taglib prefix="c" uri="http://java.sun.com/jsp/jstl/core"%>
<html>
<body>
<h1>Spring MVC Hello World Example</h1>
<h2>${msg}</h2>
</body>
</html>
5. Spring Configuration
In web.xml
, declared a DispatcherServlet servlet, named “mvc-dispatcher“, and act as the front-controller to handle all the entire web request which end with “htm” extension.
File : web.xml
<web-app id="WebApp_ID" version="2.4"
xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee
http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd">
<display-name>Spring Web MVC Application</display-name>
<servlet>
<servlet-name>mvc-dispatcher</servlet-name>
<servlet-class>
org.springframework.web.servlet.DispatcherServlet
</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>mvc-dispatcher</servlet-name>
<url-pattern>*.htm</url-pattern>
</servlet-mapping>
</web-app>
Alternatively, you can explicitly specify the Spring configuration file in the “contextConfigLocation” servlet parameter, to ask Spring to load your configurations besides the default “mvc-dispatcher-servlet.xml“.
File : web.xml
<web-app id="WebApp_ID" version="2.4"
xmlns="http://java.sun.com/xml/ns/j2ee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/j2ee
http://java.sun.com/xml/ns/j2ee/web-app_2_4.xsd">
<display-name>Spring Web MVC Application</display-name>
<servlet>
<servlet-name>mvc-dispatcher</servlet-name>
<servlet-class>
org.springframework.web.servlet.DispatcherServlet
</servlet-class>
<load-on-startup>1</load-on-startup>
</servlet>
<servlet-mapping>
<servlet-name>mvc-dispatcher</servlet-name>
<url-pattern>*.htm</url-pattern>
</servlet-mapping>
<context-param>
<param-name>contextConfigLocation</param-name>
<param-value>/WEB-INF/SpringMVCBeans.xml</param-value>
</context-param>
<listener>
<listener-class>
org.springframework.web.context.ContextLoaderListener
</listener-class>
</listener>
</web-app>
6. Spring Beans Configuration
Declared the Spring Controller and viewResolver.
File : mvc-dispatcher-servlet.xml
<beans xmlns="http://www.springframework.org/schema/beans"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://www.springframework.org/schema/beans
http://www.springframework.org/schema/beans/spring-beans-2.5.xsd">
<bean name="/welcome.htm"
class="com.mkyong.common.controller.HelloWorldController" />
<bean id="viewResolver"
class="org.springframework.web.servlet.view.InternalResourceViewResolver" >
<property name="prefix">
<value>/WEB-INF/pages/</value>
</property>
<property name="suffix">
<value>.jsp</value>
</property>
</bean>
</beans>
- Controller – Declared a bean name “/welcome.htm” and map it to HelloWorldController class. It means, if an URL with “/welcome.htm” pattern is requested, it will send to the HelloWorldController controller to handle the request.
- viewResolver – Define how Spring will looking for the view template. In this case, the controller “HelloWorldController” will return a ModelAndView object named “HelloWorldPage”, and the viewResolver will find the file with following mechanism : “prefix + ModelAndView name + suffix“, which is “/WEB-INF/pages/HelloWorldPage.jsp“.
7. Demo
Run it and access via URL : http://localhost:8080/SpringMVC/welcome.htm , the “SpringMVC” is your project context name.

How it works?
- http://localhost:8080/SpringMVC/welcome.htm is requested.
- URL is end with “.htm” extension, so it will redirect to “DispatcherServlet” and send request to the default BeanNameUrlHandlerMapping.
- BeanNameUrlHandlerMapping return HelloWorldController to the DispatcherServlet.
- DispatcherServlet forward request to the HelloWorldController.
- HelloWorldController process it and return a ModelAndView object named “HelloWorldPage”.
- DispatcherServlet received the ModelAndView and call the viewResolver to process it.
- viewResolver return the “/WEB-INF/pages/HelloWorldPage.jsp” back to the DispatcherServlet.
- DispatcherServlet return the “HelloWorldPage.jsp” back to user.
Download Source Code
Spring MVC Hello World Example(转)的更多相关文章
- 如何用Java类配置Spring MVC(不通过web.xml和XML方式)
DispatcherServlet是Spring MVC的核心,按照传统方式, 需要把它配置到web.xml中. 我个人比较不喜欢XML配置方式, XML看起来太累, 冗长繁琐. 还好借助于Servl ...
- Spring MVC重定向和转发以及异常处理
SpringMVC核心技术---转发和重定向 当处理器对请求处理完毕后,向其他资源进行跳转时,有两种跳转方式:请求转发与重定向.而根据要跳转的资源类型,又可分为两类:跳转到页面与跳转到其他处理器.对于 ...
- Spring MVC入门
1.什么是SpringMvc Spring MVC属于SpringFrameWork的后续产品,已经融合在Spring Web Flow里面.Spring 框架提供了构建 Web 应用程序的全功能 M ...
- Spring7:基于注解的Spring MVC(下篇)
Model 上一篇文章<Spring6:基于注解的Spring MVC(上篇)>,讲了Spring MVC环境搭建.@RequestMapping以及参数绑定,这是Spring MVC中最 ...
- Spring6:基于注解的Spring MVC(上篇)
什么是Spring MVC Spring MVC框架是一个MVC框架,通过实现Model-View-Controller模式来很好地将数据.业务与展现进行分离.从这样一个角度来说,Spring MVC ...
- 高性能的关键:Spring MVC的异步模式
我承认有些标题党了,不过话说这样其实也没错,关于“异步”处理的文章已经不少,代码例子也能找到很多,但我还是打算发表这篇我写了好长一段时间,却一直没发表的文章,以一个更简单的视角,把异步模式讲清楚. 什 ...
- Java Spring mvc 操作 Redis 及 Redis 集群
本文原创,转载请注明:http://www.cnblogs.com/fengzheng/p/5941953.html 关于 Redis 集群搭建可以参考我的另一篇文章 Redis集群搭建与简单使用 R ...
- 深入分析Spring 与 Spring MVC容器
1 Spring MVC WEB配置 Spring Framework本身没有Web功能,Spring MVC使用WebApplicationContext类扩展ApplicationContext, ...
- spring mvc DispatcherServlet详解之前传---FrameworkServlet
做项目时碰到Controller不能使用aop进行拦截,从网上搜索得知:使用spring mvc 启动了两个context:applicationContext 和WebapplicationCont ...
- 我是如何进行Spring MVC文档翻译项目的环境搭建、项目管理及自动化构建工作的
感兴趣的同学可以关注这个翻译项目 . 我的博客原文 和 我的Github 前段时间翻译的Spring MVC官方文档完成了第一稿,相关的文章和仓库可以点击以下链接.这篇文章,主要是总结一下这个翻译项目 ...
随机推荐
- Buenos Aires和Rio de Janeiro怎么发音?
Buenos Aires和Rio de Janeiro怎么发音?_百度知道 Buenos Aires和Rio de Janeiro怎么发音? 2009-09-25 08:58 zd029 ...
- 刘德华夏日Fiesta演唱会上那个表演探戈舞的演员是谁啊?_百度知道
刘德华夏日Fiesta演唱会上那个表演探戈舞的演员是谁啊?_百度知道 刘德华夏日Fiesta演唱会上那个表演探戈舞的演员是谁啊? 2008-05-28 00:04 topofhill | ...
- javascript 偏移量
通过四个属性可以获得元素的偏移量: 1.offsetHeight: 元素在垂直方向上占用的空间的大小,(像素).包括元素的高度,(可见的)水平滚动条的高度,上边框高度和下边框高度. 2.offsetW ...
- JAVA学习笔记 -- 数据结构
一.数据结构的接口 在Java中全部类的鼻祖是Object类,可是全部有关数据结构处理的鼻祖就是Collection和Iterator接口,也就是集合与遍历. 1.Collection接口 Colle ...
- Hadoop 2.x从零基础到挑战百万年薪第一季
鉴于目前大数据Hadoop 2.x被企业广泛使用,在实际的企业项目中需要更加深入的灵活运用,并且Hadoop 2.x是大数据平台处理 的框架的基石,尤其在海量数据的存储HDFS.分布式资源管理和任务调 ...
- 解决cocoapods在64位iOS7系统以下的警告问题
今天碰到一个非常奇怪的问题.XCODE提示这种警告 Pods was rejected as an implicit dependency for 'libPods.a' because its ar ...
- HDU 4669 Mutiples on a circle (DP , 统计)
转载请注明出处,谢谢http://blog.csdn.net/ACM_cxlove?viewmode=contents by---cxlove 题意:给出一个环,每个点是一个数字,取一个子串,使 ...
- Swift - 跑酷游戏开发(SpriteKit游戏开发)
一,下面演示了如何开发一个跑酷游戏,实现的功能如下: 1,平台工厂会不断地生成平台,并且向左移动.当平台移出游戏场景时就可将其移除. 2,生成的平台宽度随机,高度随机.同时短平台踩踏的时候会下落. 3 ...
- 获取字符宽度:并非自适应。coretext去计算
获取字符宽度:并非自适应.coretext去计算 UniChar ch = [ns_str characterAtIndex:0]; CGGlyph glyph = 0; CTFontGetGlyph ...
- 九道大型软件公司.net面试题!一定得看(附答案)
1:a=10,b=15,在不用第三方变量的前提下,把a,b的值互换 2:已知数组int[] max={6,5,2,9,7,4,0};用快速排序算法按降序对其进行排列,并返回数组 3:请简述面向 ...