poj1872A Dicey Problem
Home | Problems | Status | Contest |
A
B
C
D
E
F
G
H
I
J
K
L
M
N
O
Time Limit:1000MS Memory Limit:65536KB 64bit IO Format:%I64d & %I64u
Status
Description
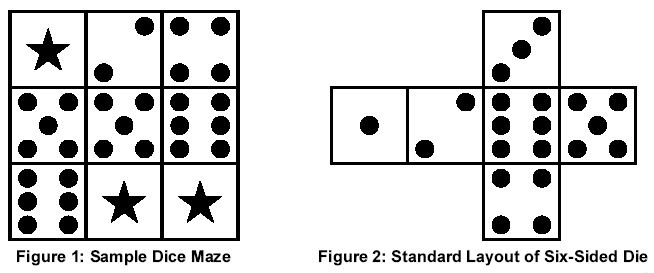
To move through the maze you must tip the die over on an edge to land on an adjacent square, effecting horizontal or vertical movement from one square to another. However, you can only move onto a square that contains the same number as the number displayed on the top of the die before the move, or onto a "wild" square which contains a star. Movement onto a wild square is always allowed regardless of the number currently displayed on the top of the die. The goal of the maze is to move the die off the starting square and to then find a way back to that same square.
For example, at the beginning of the maze there are two possible moves. Since the 5 is on top of the die, it is possible to move down one square, and since the square to the left of the starting position is wild it is also possible to move left. If the first move chosen is to move down, this brings the 6 to the top of the die and moves are now possible both to the right and down. If the first move chosen is instead to the left, this brings the 3 to the top of the die and no further moves are possible.
If we consider maze locations as ordered pairs of row and column numbers (row, column) with row indexes starting at 1 for the top row and increasing toward the bottom, and column indexes starting at 1 for the left column and increasing to the right, the solution to this simple example maze can be specified as: (1,2), (2,2), (2,3), (3,3), (3,2), (3,1), (2,1), (1,1), (1,2). A bit more challenging example maze is shown in Figure 3.
The goal of this problem is to write a program to solve dice mazes. The input file will contain several mazes for which the program should search for solutions. Each maze will have either a unique solution or no solution at all. That is, each maze in the input may or may not have a solution, but those with a solution are guaranteed to have only one unique solution. For each input maze, either a solution or a message indicating no solution is possible will be sent to the output.
Input
Output
Sample Input
DICEMAZE1
3 3 1 2 5 1
-1 2 4
5 5 6
6 -1 -1
DICEMAZE2
4 7 2 6 3 6
6 4 6 0 2 6 4
1 2 -1 5 3 6 1
5 3 4 5 6 4 2
4 1 2 0 3 -1 6
DICEMAZE3
3 3 1 1 2 4
2 2 3
4 5 6
-1 -1 -1
END
Sample Output
DICEMAZE1
(1,2),(2,2),(2,3),(3,3),(3,2),(3,1),(2,1),(1,1),(1,2)
DICEMAZE2
(2,6),(2,5),(2,4),(2,3),(2,2),(3,2),(4,2),(4,1),(3,1),
(2,1),(2,2),(2,3),(2,4),(2,5),(1,5),(1,6),(1,7),(2,7),
(3,7),(4,7),(4,6),(3,6),(2,6)
DICEMAZE3
No Solution Possible
用了个bfs就过了,水题,在最后格式输出的时候错了几次,做题的时候,不防做个小正方形,这样省去了大量的想象,速度也快的多!
#include <iostream>
#include <stdio.h>
#include<string.h>
#include<string>
#include <queue>
using namespace std;
int row,column,up,down,n,m,left,right,front,back,startx,starty;
struct mazntree{int x[7];string re;int row,colomn;};
mazntree start,p,temp;
int visit[7][7][50][50];
int map[100][100];
int dir[4][2]={{0,-1},{1,0},{0,1},{-1,0}};
int init()
{
scanf("%d%d%d%d%d%d",&n,&m,&startx,&starty,&up,&front);
if(up==1)
{
if(front==2)
{
start.x[1]=1,start.x[2]=6,start.x[3]=4,start.x[4]=3,start.x[5]=2,start.x[6]=5;
}
else if(front==3)
{
start.x[1]=1,start.x[2]=6,start.x[3]=2,start.x[4]=5,start.x[5]=3,start.x[6]=4;
}
else if(front==4)
{
start.x[1]=1,start.x[2]=6,start.x[3]=5,start.x[4]=2,start.x[5]=4,start.x[6]=3;
}
else if( front==5)
{
start.x[1]=1,start.x[2]=6,start.x[3]=3,start.x[4]=4,start.x[5]=5,start.x[6]=2;
}
}
else if(up==2)
{
if(front==1)
{
start.x[1]=2,start.x[2]=5,start.x[3]=3,start.x[4]=4,start.x[5]=1,start.x[6]=6;
}
else if(front==3)
{
start.x[1]=2,start.x[2]=5,start.x[3]=6,start.x[4]=1,start.x[5]=3,start.x[6]=4;
}
else if(front==4)
{
start.x[1]=2,start.x[2]=5,start.x[3]=1,start.x[4]=6,start.x[5]=4,start.x[6]=3;
}
else if( front==6)
{
start.x[1]=2,start.x[2]=5,start.x[3]=4,start.x[4]=3,start.x[5]=6,start.x[6]=1;
}
}
else if(up==3)
{
if(front==1)
{
start.x[1]=3,start.x[2]=4,start.x[3]=5,start.x[4]=2,start.x[5]=1,start.x[6]=6;
}
else if(front==2)
{
start.x[1]=3,start.x[2]=4,start.x[3]=1,start.x[4]=6,start.x[5]=2,start.x[6]=5;
}
else if(front==5)
{
start.x[1]=3,start.x[2]=4,start.x[3]=6,start.x[4]=1,start.x[5]=5,start.x[6]=2;
}
else if( front==6)
{
start.x[1]=3,start.x[2]=4,start.x[3]=2,start.x[4]=5,start.x[5]=6,start.x[6]=1;
}
}
else if(up==4)
{
if(front==1)
{
start.x[1]=4,start.x[2]=3,start.x[3]=2,start.x[4]=5,start.x[5]=1,start.x[6]=6;
}
else if(front==2)
{
start.x[1]=4,start.x[2]=3,start.x[3]=6,start.x[4]=1,start.x[5]=2,start.x[6]=5;
}
else if(front==5)
{
start.x[1]=4,start.x[2]=3,start.x[3]=1,start.x[4]=6,start.x[5]=5,start.x[6]=2;
}
else if( front==6)
{
start.x[1]=4,start.x[2]=3,start.x[3]=5,start.x[4]=2,start.x[5]=6,start.x[6]=1;
}
}
else if(up==5)
{
if(front==1)
{
start.x[1]=5,start.x[2]=2,start.x[3]=4,start.x[4]=3,start.x[5]=1,start.x[6]=6;
}
else if(front==3)
{
start.x[1]=5,start.x[2]=2,start.x[3]=1,start.x[4]=6,start.x[5]=3,start.x[6]=4;
}
else if(front==4)
{
start.x[1]=5,start.x[2]=2,start.x[3]=6,start.x[4]=1,start.x[5]=4,start.x[6]=3;
}
else if( front==6)
{
start.x[1]=5,start.x[2]=2,start.x[3]=3,start.x[4]=4,start.x[5]=6,start.x[6]=1;
}
}
else if(up==6)
{
if(front==2)
{
start.x[1]=6,start.x[2]=1,start.x[3]=3,start.x[4]=4,start.x[5]=2,start.x[6]=5;
}
else if(front==3)
{
start.x[1]=6,start.x[2]=1,start.x[3]=5,start.x[4]=2,start.x[5]=3,start.x[6]=4;
}
else if(front==4)
{
start.x[1]=6,start.x[2]=1,start.x[3]=2,start.x[4]=5,start.x[5]=4,start.x[6]=3;
}
else if( front==5)
{
start.x[1]=6,start.x[2]=1,start.x[3]=4,start.x[4]=3,start.x[5]=5,start.x[6]=2;
}
}
int i,j;
for(i=1;i<=n;i++)
{
for(j=1;j<=m;j++)
{
scanf("%d",&map[i][j]);
}
}
return 1;
}
bool changecan(int i)//判定能否走
{
int x[7];
for( int j=1;j<7;j++)
{
x[j]=p.x[j];
}
p.row=p.row+dir[i][0];
p.colomn=p.colomn+dir[i][1];
if((map[p.row][p.colomn]!=0)&&p.row>=1&&p.row<=n&&p.colomn>=1&&p.colomn<=m&&((p.x[1]==map[p.row][p.colomn])||(map[p.row][p.colomn]==-1)))//不越界
{ if( i==0)//左移
{
p.x[3]=x[1];
p.x[4]=x[2];
p.x[2]=x[3];
p.x[1]=x[4];
p.re=temp.re+'0';
}
else if(i==1)//下称
{
p.x[5]=x[1];
p.x[6]=x[2];
p.x[2]=x[5];
p.x[1]=x[6];
p.re=temp.re+'1'; }
else if ( i==2)//右移
{
p.x[4]=x[1];
p.x[3]=x[2];
p.x[1]=x[3];
p.x[2]=x[4];
p.re=temp.re+'2'; }
else if (i==3)//上移
{
p.x[6]=x[1];
p.x[5]=x[2];
p.x[1]=x[5];
p.x[2]=x[6];
p.re=temp.re+'3';
}
if(visit[p.x[1]][p.x[5]][p.row][p.colomn]==0)//没有访问过-1能走,0不能走
{
visit[p.x[1]][p.x[5]][p.row][p.colomn]=1;//标记为已访问
return true;
}
else
return false;
}
else
{
return false;
}
return false;
}
int bfs()
{
queue<mazntree> q;
int i,xx,yy;
memset(visit ,0,sizeof(visit));
while(!q.empty())//先清空
{
q.pop();
}
start.re="";
start.colomn=starty;
start.row=startx;
q.push(start); while(!q.empty())
{
temp=q.front();
q.pop();
for( i=0;i<4;i++)
{
p=temp;
if(changecan(i))
{ q.push(p);
if(visit[p.x[1]][p.x[5]][p.row][p.colomn]&&(p.row==startx)&&(p.colomn==starty))//找到
{ int count=0;
xx=startx,yy=starty;
count=1;
printf(" (%d,%d),",startx,starty); for( i=0;i<p.re.length()-1;i++,count++)
{
xx=xx+dir[p.re[i]-'0'][0];
yy=yy+dir[p.re[i]-'0'][1];
if(count==0) printf(" "); printf("(%d,%d),",xx,yy); if(count==8)
{
printf("\n");
count=-1;
}
}
xx=xx+dir[p.re[i]-'0'][0];
yy=yy+dir[p.re[i]-'0'][1];
printf("(%d,%d)\n",xx,yy); return 1;
}
}
}
}
printf(" No Solution Possible\n");
return -1;
}
int main()
{
char str[200];
while(1)
{ gets(str);
if(str[0]=='E'&&str[1]=='N'&&str[2]=='D')
break;
init();
printf("%s\n",str);
gets(str);//把最后的回车吸收
bfs();
}
return 0;
}
FAQ | About Virtual Judge | Forum | Discuss | Open Source Project
All Copyright Reserved ©2010-2012
HUST ACM/ICPC TEAM
Anything about the OJ, please ask in the
forum, or contact author:
Isun
Server Time:
poj1872A Dicey Problem的更多相关文章
- poj 1872 A Dicey Problem WA的代码,望各位指教!!!
A Dicey Problem Time Limit: 1000MS Memory Limit: 65536K Total Submissions: 832 Accepted: 278 Des ...
- UVA 810 - A Dicey Problem(BFS)
UVA 810 - A Dicey Problem 题目链接 题意:一个骰子,给你顶面和前面.在一个起点,每次能移动到周围4格,为-1,或顶面和该位置数字一样,那么问题来了,骰子能不能走一圈回到原地, ...
- UVA-810 A Dicey Problem (BFS)
题目大意:滚骰子游戏,骰子的上面的点数跟方格中的数相同时或格子中的数是-1时能把格子滚过去,找一条从起点滚到起点的路径. 题目大意:简单BFS,状态转移时细心一些即可. 代码如下; # include ...
- A Dicey Problem 骰子难题(Uva 810)
题目描述:https://uva.onlinejudge.org/external/8/810.pdf 把一个骰子放在一个M x N的地图上,让他按照规定滚动,求滚回原点的最短路径. 思路: 记忆化 ...
- Uva - 810 - A Dicey Problem
根据状态进行bfs,手动打表维护骰子滚动. AC代码: #include <iostream> #include <cstdio> #include <cstdlib&g ...
- BFS广搜题目(转载)
BFS广搜题目有时间一个个做下来 2009-12-29 15:09 1574人阅读 评论(1) 收藏 举报 图形graphc优化存储游戏 有时间要去做做这些题目,所以从他人空间copy过来了,谢谢那位 ...
- 1199 Problem B: 大小关系
求有限集传递闭包的 Floyd Warshall 算法(矩阵实现) 其实就三重循环.zzuoj 1199 题 链接 http://acm.zzu.edu.cn:8000/problem.php?id= ...
- No-args constructor for class X does not exist. Register an InstanceCreator with Gson for this type to fix this problem.
Gson解析JSON字符串时出现了下面的错误: No-args constructor for class X does not exist. Register an InstanceCreator ...
- C - NP-Hard Problem(二分图判定-染色法)
C - NP-Hard Problem Crawling in process... Crawling failed Time Limit:2000MS Memory Limit:262144 ...
随机推荐
- 多线程学习之五超时模式Timer
timed[超时模式]案例:一个线程提供下载数据,另一个线程执行下载,如果有5秒钟以上,提供下载的线程没有提供数据,下载线程因超时异常,停止下载线程运行. 超时异常类 /** * */ package ...
- javascript7
语句:条件,循环,跳转, 表达式语句,复合语句和空语句,声明语句,var,function,条件语句,switch,循环,标签语句,break语句,continue语句,return语句,throw语 ...
- each与list的用法
each与list的用法(PHP学习) 1.each的用法 先看API array each ( array &$array ) api里是这么描述的:each — 返回数组中当前的键/值对并 ...
- 使用oracle的exp命令时,提示出--hash: exp: command not found
使用oracle的exp命令时,提示出--hash: exp: command not found 原因:当你在终端使用exp的命名时,当前的账户,并不是oracle认可的账户. 在安装oracle时 ...
- 关于Java String对象创建的几点疑问
我们通过JDK源码会知道String实质是字符数组,而且是不可被继承(final)和具有不可变性(immutable).可以如果想要了解String的创建我们需要先了解下JVM的内存结构. 1.JVM ...
- ReSharper 8.1支持Visual Studio 2013的特色——超强滚动条
自ReSharper 8.1发布以来,便支持Visual Studio 2013.其中peek功能是它的亮点,滚动条则是它的特色. 接下来小编将展示ReSharper在Visual Studio 20 ...
- html postMessage 创建聊天应用
应用说明: 这个例子演示如何在门户页面以iframe方式嵌入第三方插件,示例中使用了一个来域名下的应用部件,门户页面通过postMessage来通信.iframe中的聊天部件通过父页面标题内容的闪烁来 ...
- ssis的script task作业失败(调用外部dll)
原文 ssis的script task作业失败 我的ssis作业包里用了一个script task,会查询一个http的页面接口,获取json数据后解析然后做后续处理,其中解析json引用了本地目录下 ...
- WITH (NOLOCK)浅析
SQL Server 中WITH (NOLOCK)浅析 2014-08-30 11:58 by 潇湘隐者, 503 阅读, 2 评论, 收藏, 编辑 概念介绍 开发人员喜欢在SQL脚本中使用WITH( ...
- validate大表单验证
Vaidate 插件 在前端开发中, 我们会遇到大表单的验证和组合成JSON, 这是一项巨大的任务, 如果都通过 手动编写低级代码来实现 50+ input类型的验证和复杂JSON的组装, 这无疑是异 ...