【leetcode】208. Implement Trie (Prefix Tree 字典树)
- Trie() Initializes the trie object.
- void insert(String word) Inserts the string word into the trie.
- boolean search(String word) Returns true if the string word is in the trie (i.e., was inserted before), and false otherwise.
- boolean startsWith(String prefix) Returns true if there is a previously inserted string word that has the prefix prefix, andfalse otherwis
struct TreeNode {
VALUETYPE value; //结点值
TreeNode* children[NUM]; //指向孩子结点 //指针数组
};
/**
* Definition for a binary tree node.
struct TreeNode {
int val;
TreeNode *left;
TreeNode *right;
TreeNode(int x) : val(x), left(NULL), right(NULL) {}
* };
*/
struct Trie {
bool isEnd; //到当前节点是否结束
Trie* next[26]; //指向孩子结点 //指针数组
};
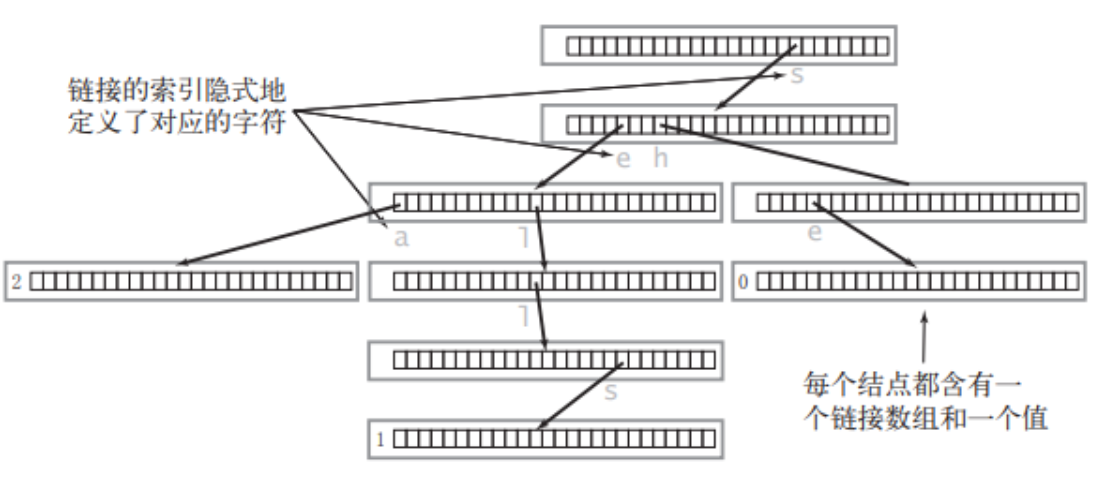
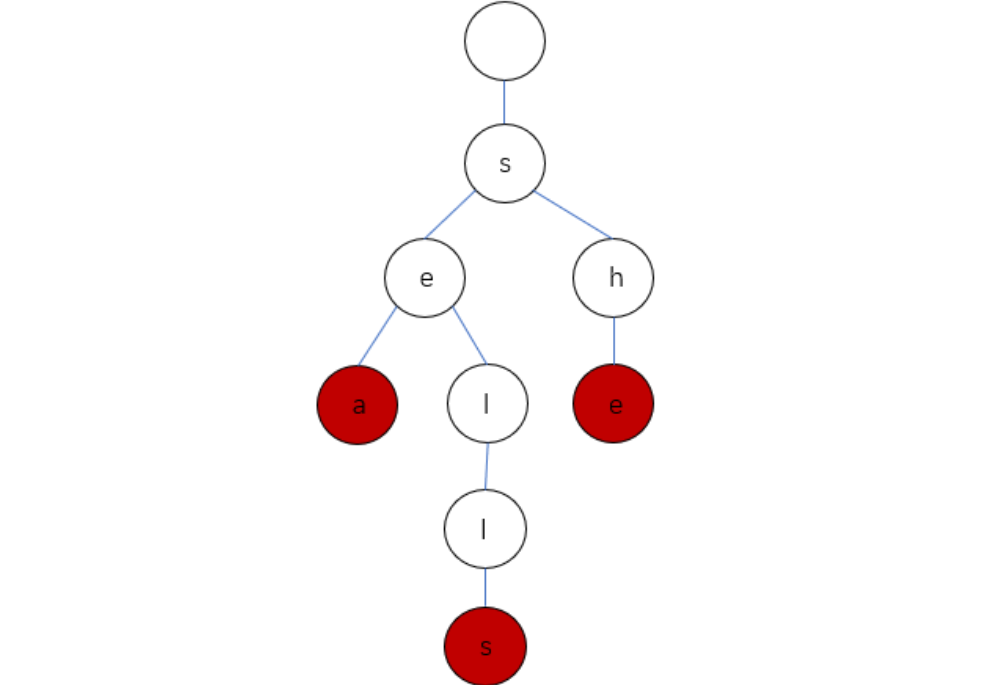
按照上述字典树的介绍,先构建字典树,每一级用一个长度为26的指针数组来存储当前的字符以及下级的位置。
class Trie {
private:
bool isEnd;
Trie *next[26];
public:
Trie() {
isEnd=false;
memset(next,0,sizeof(next));//初始化多叉树的索引 } void insert(string word) {
Trie *node=this;
for(auto ww:word){
if(node->next[ww-'a']==NULL){
node->next[ww-'a']=new Trie();
}
node=node->next[ww-'a'];
}
node->isEnd=true; } bool search(string word) {
Trie *node=this;
for(auto ww:word){
if(node->next[ww-'a']==NULL) return false;
node=node->next[ww-'a'];
}
return node->isEnd; } bool startsWith(string prefix) {
Trie *node=this;
for(auto ww:prefix){
if(node->next[ww-'a']==NULL) return false;
node=node->next[ww-'a'];
}
return true; }
}; /**
* Your Trie object will be instantiated and called as such:
* Trie* obj = new Trie();
* obj->insert(word);
* bool param_2 = obj->search(word);
* bool param_3 = obj->startsWith(prefix);
*/
【leetcode】208. Implement Trie (Prefix Tree 字典树)的更多相关文章
- LeetCode 208 Implement Trie (Prefix Tree) 字典树(前缀树)
Implement a trie with insert, search, and startsWith methods.Note:You may assume that all inputs are ...
- 208 Implement Trie (Prefix Tree) 字典树(前缀树)
实现一个 Trie (前缀树),包含 insert, search, 和 startsWith 这三个方法.注意:你可以假设所有的输入都是小写字母 a-z.详见:https://leetcode.co ...
- 字典树(查找树) leetcode 208. Implement Trie (Prefix Tree) 、211. Add and Search Word - Data structure design
字典树(查找树) 26个分支作用:检测字符串是否在这个字典里面插入.查找 字典树与哈希表的对比:时间复杂度:以字符来看:O(N).O(N) 以字符串来看:O(1).O(1)空间复杂度:字典树远远小于哈 ...
- [LeetCode] 208. Implement Trie (Prefix Tree) ☆☆☆
Implement a trie with insert, search, and startsWith methods. Note:You may assume that all inputs ar ...
- [LeetCode] 208. Implement Trie (Prefix Tree) 实现字典树(前缀树)
Implement a trie with insert, search, and startsWith methods. Example: Trie trie = new Trie(); trie. ...
- Java for LeetCode 208 Implement Trie (Prefix Tree)
Implement a trie with insert, search, and startsWith methods. Note: You may assume that all inputs a ...
- leetcode@ [208] Implement Trie (Prefix Tree)
Trie 树模板 https://leetcode.com/problems/implement-trie-prefix-tree/ class TrieNode { public: char var ...
- 208. Implement Trie (Prefix Tree) -- 键树
Implement a trie with insert, search, and startsWith methods. Note:You may assume that all inputs ar ...
- 【LeetCode】208. Implement Trie (Prefix Tree)
Implement Trie (Prefix Tree) Implement a trie with insert, search, and startsWith methods. Note:You ...
随机推荐
- properties 文件解析
1.提供properties文件 jdbc.driver=com.mysql.jdbc.Driver jdbc.url=jdbc:mysql://localhost:3306/future?useUn ...
- CSS 海盗船加载特效
CSS 海盗船加载特效 <!DOCTYPE html> <html lang="en"> <head> <meta charset=
- Go语言核心36讲(Go语言实战与应用二)--学习笔记
24 | 测试的基本规则和流程(下) Go 语言是一门很重视程序测试的编程语言,所以在上一篇中,我与你再三强调了程序测试的重要性,同时,也介绍了关于go test命令的基本规则和主要流程的内容.今天我 ...
- 关于 RocketMQ 事务消息的正确打开方式 → 你学废了吗
开心一刻 昨晚和一哥们一起吃夜宵,点了几瓶啤酒 不一会天空下起了小雨,哥们突然道:糟了 我:怎么了 哥们:外面下雨了,我老婆还在等着我去接她 他给了自己一巴掌,说道:真他妈不是个东西 我心想:哥们真是 ...
- linux 系统ssh超时设置
1.修改client端的etc/ssh/ssh_config添加以下:(在没有权限改server配置的情形下) ServerAliveInterval 60 #client每隔60秒发送一次请求给se ...
- CTF入门学习2->Web基础了解
Web安全基础 00 Web介绍 00-00 Web本意是网,这里多指万维网(World Wide Web),是由许多互相连接的超文本系统组成的,通过互联网访问. Web是非常广泛的互联网应用,每天都 ...
- [atAGC004F]Namori
考虑树的情况,将其以任意一点为根建树 对于每一个节点,考虑其要与父亲操作几次才能使子树内均为黑色,这可以用形如$(0/1,x)$的二元组来描述,其中0/1即表示其要求操作时父亲是白色/黑色且要操作$x ...
- [luogu5665]划分
暴力dp,用f[i][j]表示前i个数,最后一个区间是(j,i]的最小答案,转移方程用可以用前缀和来优化,复杂度为$o(n^3)$(然后可以各种优化到$o(n^2)$,但这不需要)输出f[i][j], ...
- mybatis新增账号并且返回主键id
<!--新增账号和权限的关联关系--><insert id="save" useGeneratedKeys="true" keyPropert ...
- 直接插入100w数据报错
### Cause: com.mysql.cj.jdbc.exceptions.PacketTooBigException: Packet for query is too large (77,600 ...