Spring boot Sample 006之spring-boot-custom-servlet
一、环境
1.1、Idea 2020.1
1.2、JDK 1.8
二、步骤
2.1、点击File -> New Project -> Spring Initializer,点击next
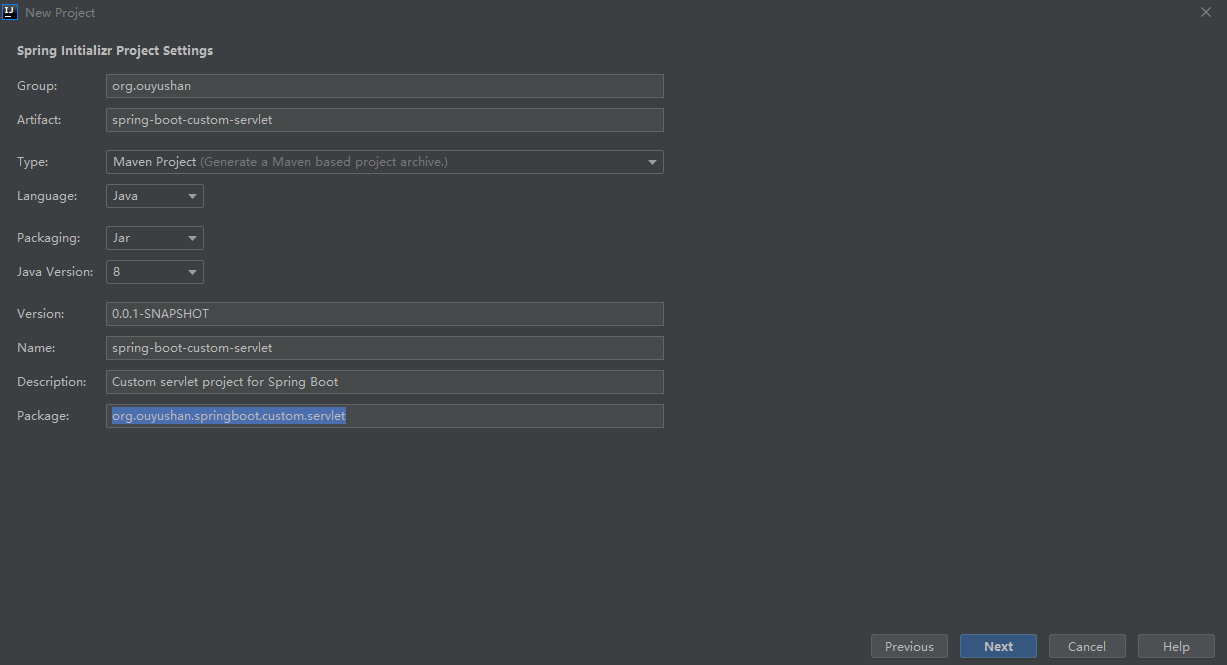
2.3、选择Web依赖,选中Spring Web。可以选择Spring Boot版本,本次默认为2.2.6,点击Next
2.4、项目结构
三、自定义实现
3.1、通过bean实现
package org.ouyushan.springboot.custom.servlet.config.listener; import javax.servlet.ServletContextEvent;
import javax.servlet.ServletContextListener; /**
* @Description: 自定义监听器
* @Author: ouyushan
* @Email: ouyushan@hotmail.com
* @Date: 2020/4/28 10:45
*/
public class CustomListener implements ServletContextListener { @Override
public void contextInitialized(ServletContextEvent sce) {
System.out.println("========contextInitialized========");
} @Override
public void contextDestroyed(ServletContextEvent sce) {
System.out.println("========contextDestroyed========");
}
}
package org.ouyushan.springboot.custom.servlet.config.filter; import javax.servlet.*;
import java.io.IOException; /**
* @Description: 自定义fileter
* @Author: ouyushan
* @Email: ouyushan@hotmail.com
* @Date: 2020/4/28 10:38
*/
public class CustomFilter implements Filter {
@Override
public void init(FilterConfig filterConfig) throws ServletException {
System.out.println("========init filter========");
} @Override
public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException {
System.out.println("========do filter========");
filterChain.doFilter(servletRequest, servletResponse);
} @Override
public void destroy() {
System.out.println("========destroy filter========");
}
}
package org.ouyushan.springboot.custom.servlet.config.servlet; import javax.servlet.ServletException;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException; /**
* @Description: 自定义servlet
* @Author: ouyushan
* @Email: ouyushan@hotmail.com
* @Date: 2020/4/28 10:25
*/
public class CustomServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("========servlet get method is called========");
resp.getWriter().write("hello world by get");
} @Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws ServletException, IOException {
System.out.println("========servlet post method is called========");
resp.getWriter().write("hello world by post");
}
}
package org.ouyushan.springboot.custom.servlet.controller; import org.springframework.web.bind.annotation.RequestMapping;
import org.springframework.web.bind.annotation.RestController; /**
* @Description:
* @Author: ouyushan
* @Email: ouyushan@hotmail.com
* @Date: 2020/4/28 10:55
*/
@RestController
@RequestMapping("/api")
public class ServletController { @RequestMapping("/servlet")
public String servlet() {
return "custom servlet";
} @RequestMapping("/filter")
public String filter() {
return "custom filter";
}
}
package org.ouyushan.springboot.custom.servlet; import org.ouyushan.springboot.custom.servlet.config.filter.CustomFilter;
import org.ouyushan.springboot.custom.servlet.config.listener.CustomListener;
import org.ouyushan.springboot.custom.servlet.config.servlet.CustomServlet;
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.boot.web.servlet.FilterRegistrationBean;
import org.springframework.boot.web.servlet.ServletListenerRegistrationBean;
import org.springframework.boot.web.servlet.ServletRegistrationBean;
import org.springframework.context.annotation.Bean; @SpringBootApplication
public class SpringBootCustomServletApplication { @Bean
public ServletRegistrationBean servletRegistrationBean() {
// 只有路径是以/custom/servlet开始的才会触发
return new ServletRegistrationBean(new CustomServlet(), "/custom/servlet");
} @Bean
public FilterRegistrationBean filterRegistrationBean() {
//第二个参数为需要拦截的路径,不传则拦截所有
return new FilterRegistrationBean(new CustomFilter(), servletRegistrationBean());
} @Bean
public ServletListenerRegistrationBean<CustomListener> servletListenerRegistrationBean() {
return new ServletListenerRegistrationBean<CustomListener>(new CustomListener());
} public static void main(String[] args) {
SpringApplication.run(SpringBootCustomServletApplication.class, args);
} }
========contextInitialized========
========init filter========
custom servlet
========do filter========
========servlet get method is called========
========destroy filter========
========contextDestroyed========
3.2、通过实现ServletContextInitializer实现
// 方式二 通过实现ServletContextInitializer
@SpringBootApplication
public class SpringBootCustomServletApplication implements ServletContextInitializer { @Override
public void onStartup(ServletContext servletContext) {
// 创建Servlet,并映射访问路径为/custom/servlet
servletContext.addServlet("customServlet", new CustomServlet()).addMapping("/custom/servlet"); // 创建Filter,拦截的Servlet
servletContext.addFilter("customFilter", new CustomFilter())
.addMappingForServletNames(EnumSet.of(DispatcherType.REQUEST), true, "customServlet"); // 设置自定义filter
servletContext.addListener(new CustomListener());
} public static void main(String[] args) {
SpringApplication.run(SpringBootCustomServletApplication.class, args);
}
}
========contextInitialized========
========init filter========
custom servlet
========do filter========
========servlet get method is called========
========destroy filter========
========contextDestroyed========
3.3、通过@ServletComponentScan结合注解实现
@ServletComponentScan
@SpringBootApplication
public class SpringBootCustomServletApplication {
public static void main(String[] args) {
SpringApplication.run(SpringBootCustomServletApplication.class, args);
}
}
package org.ouyushan.springboot.custom.servlet.config.servlet; import javax.servlet.ServletException;
import javax.servlet.annotation.WebServlet;
import javax.servlet.http.HttpServlet;
import javax.servlet.http.HttpServletRequest;
import javax.servlet.http.HttpServletResponse;
import java.io.IOException; /**
* @Description: 自定义servlet
* @Author: ouyushan
* @Email: ouyushan@hotmail.com
* @Date: 2020/4/28 10:25
*/ @WebServlet(name = "customServlet", urlPatterns = "/custom/servlet")
public class CustomServlet extends HttpServlet {
@Override
protected void doGet(HttpServletRequest req, HttpServletResponse resp) throws IOException {
System.out.println("========servlet get method is called========");
resp.getWriter().write("hello world by get");
} @Override
protected void doPost(HttpServletRequest req, HttpServletResponse resp) throws IOException {
System.out.println("========servlet post method is called========");
resp.getWriter().write("hello world by post");
}
} package org.ouyushan.springboot.custom.servlet.config.filter; import javax.servlet.*;
import javax.servlet.annotation.WebFilter;
import java.io.IOException; /**
* @Description: 自定义fileter
* @Author: ouyushan
* @Email: ouyushan@hotmail.com
* @Date: 2020/4/28 10:38
*/ @WebFilter(filterName = "customFilter", urlPatterns = "/*")
public class CustomFilter implements Filter {
@Override
public void init(FilterConfig filterConfig) {
System.out.println("========init filter========");
} @Override
public void doFilter(ServletRequest servletRequest, ServletResponse servletResponse, FilterChain filterChain) throws IOException, ServletException {
System.out.println("========do filter========");
filterChain.doFilter(servletRequest, servletResponse);
} @Override
public void destroy() {
System.out.println("========destroy filter========");
}
} package org.ouyushan.springboot.custom.servlet.config.listener; import javax.servlet.ServletContextEvent;
import javax.servlet.ServletContextListener;
import javax.servlet.annotation.WebListener; /**
* @Description: 自定义监听器
* @Author: ouyushan
* @Email: ouyushan@hotmail.com
* @Date: 2020/4/28 10:45
*/
@WebListener
public class CustomListener implements ServletContextListener { @Override
public void contextInitialized(ServletContextEvent sce) {
System.out.println("========contextInitialized========");
} @Override
public void contextDestroyed(ServletContextEvent sce) {
System.out.println("========contextDestroyed========");
}
}
========contextInitialized========
========init filter========
custom servlet
========do filter========
========servlet get method is called========
========destroy filter========
========contextDestroyed========
Spring boot Sample 006之spring-boot-custom-servlet的更多相关文章
- Spring boot Sample 012之spring-boot-web-upload
一.环境 1.1.Idea 2020.1 1.2.JDK 1.8 二.目的 spring boot 整合web实现文件上传下载 三.步骤 3.1.点击File -> New Project -& ...
- Spring boot Sample 0010之spring-boot-web-freemarker
一.环境 1.1.Idea 2020.1 1.2.JDK 1.8 二.目的 spring boot 整合freemarker模板开发web项目 三.步骤 3.1.点击File -> New Pr ...
- Spring boot Sample 009之spring-boot-web-thymeleaf
一.环境 1.1.Idea 2020.1 1.2.JDK 1.8 二.目的 spring boot 整合thymeleaf模板开发web项目 三.步骤 3.1.点击File -> New Pro ...
- Spring boot Sample 005之spring-boot-profile
一.环境 1.1.Idea 2020.1 1.2.JDK 1.8 二.目的 通过yaml文件配置spring boot 属性文件 三.步骤 3.1.点击File -> New Project - ...
- Spring Boot (五)Spring Data JPA 操作 MySQL 8
一.Spring Data JPA 介绍 JPA(Java Persistence API)Java持久化API,是 Java 持久化的标准规范,Hibernate是持久化规范的技术实现,而Sprin ...
- 一起学JAVA之《spring boot》03 - 开始spring boot基本配置及项目结构(转)
<div class="markdown_views"> <h3 id="一导航"><a name="t0"& ...
- Spring 5.x 、Spring Boot 2.x 、Spring Cloud 与常用技术栈整合
项目 GitHub 地址:https://github.com/heibaiying/spring-samples-for-all 版本说明: Spring: 5.1.3.RELEASE Spring ...
- spring cloud教程之使用spring boot创建一个应用
<7天学会spring cloud>第一天,熟悉spring boot,并使用spring boot创建一个应用. Spring Boot是Spring团队推出的新框架,它所使用的核心技术 ...
- Spring Boot——2分钟构建spring web mvc REST风格HelloWorld
之前有一篇<5分钟构建spring web mvc REST风格HelloWorld>介绍了普通方式开发spring web mvc web service.接下来看看使用spring b ...
随机推荐
- C:简单实现BaseCode64编码
What is Base64? 前言 目前来看遇到过Base 16.Base 32.Base 64的编解码,这种编码格式是二进制和文本编码转化,是对称并且可逆的转化.Base 64总共有64个ASCI ...
- 最简单的手机预览WEB移动端网页的方法
网上看了很多关于该问题的解决办法,各种各样的都有,个人也测试了一些, 最后总结出一个最简单且实用的方法. 1.安装nodejs node官网下载对应版本的nodejs,安装好了之后,在node.js执 ...
- 使用IR2104S搭建的H桥-机器人队比赛经典版(原作者答疑)
原理图地址:http://bbs.ednchina.com/BLOG_ARTICLE_3020313.HTM?click_from=8800020962,4950449047,2014-05-01,E ...
- Java语言简介、基础组成、封装、继承、多态、抽象类、内部类、接口
目录 Java简介 Java语言基础组成 面向对象 对象 封装 构造函数 this关键字 static(静态关键字) 主函数 静态什么时候用呢? 面向对象(数组工具对象建立) 设计模式 继承 成员变量 ...
- Java网络小结
1,定位 IP对机器的定位 端口对软件的定位(65535) URL对软件上每一份资源的定位 2,TCP和UDP TCP 安全,性能低 ①ServerSocket②Socket UDP不安全,性能高 ① ...
- [hdu5226]组合数求和取模(Lucas定理)
题意:给一个矩阵a,a[i][j] = C[i][j](i>=j) or 0(i < j),求(x1,y1),(x2,y2)这个子矩阵里面的所有数的和. 思路:首先问题可以转化为求(0,0 ...
- VMware Centos7 桥接 DHCP无法获得IP
问题描述 VMware Centos7 桥接模式下,虚拟机无法获得IP,无法联网 解决方案 网络设置为DHCP自动获取IP 查看主机(不是虚拟机)的相关服务是否打开,主要是VMware DHCP 这个 ...
- linux-设置代理和取消代理
设置代理: export http_proxy="http://proxy-XXXXX" export https_proxy="https://proxy-XXXXX: ...
- Spark aggregateByKey函数
aggregateByKey与aggregate类似,都是进行两次聚合,不同的是后者只对分区有效,前者对分区中key进一步细分 def aggregateByKey[U: ClassTag](zero ...
- git pull 部分文件无法获取
今天发现git pull origin master 的时候部分文件无法获取,然后学到了一个新方法: git fetch git checkout origin/master -- path/to/f ...