poj 1872 A Dicey Problem WA的代码,望各位指教!!!
Time Limit: 1000MS | Memory Limit: 65536K | |
Total Submissions: 832 | Accepted: 278 |
Description
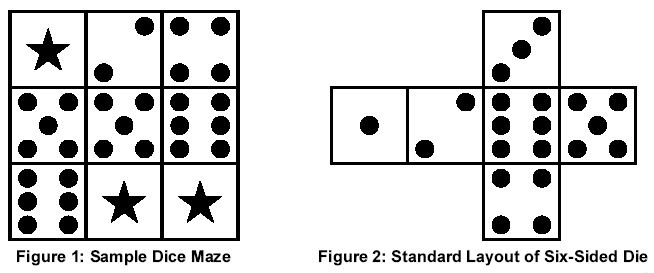
To move through the maze you must tip the die over on an edge to land on an adjacent square, effecting horizontal or vertical movement from one square to another. However, you can only move onto a square that contains the same number as the number displayed on the top of the die before the move, or onto a "wild" square which contains a star. Movement onto a wild square is always allowed regardless of the number currently displayed on the top of the die. The goal of the maze is to move the die off the starting square and to then find a way back to that same square.
For example, at the beginning of the maze there are two possible moves. Since the 5 is on top of the die, it is possible to move down one square, and since the square to the left of the starting position is wild it is also possible to move left. If the first move chosen is to move down, this brings the 6 to the top of the die and moves are now possible both to the right and down. If the first move chosen is instead to the left, this brings the 3 to the top of the die and no further moves are possible.
If we consider maze locations as ordered pairs of row and column numbers (row, column) with row indexes starting at 1 for the top row and increasing toward the bottom, and column indexes starting at 1 for the left column and increasing to the right, the solution to this simple example maze can be specified as: (1,2), (2,2), (2,3), (3,3), (3,2), (3,1), (2,1), (1,1), (1,2). A bit more challenging example maze is shown in Figure 3.
The goal of this problem is to write a program to solve dice mazes. The input file will contain several mazes for which the program should search for solutions. Each maze will have either a unique solution or no solution at all. That is, each maze in the input may or may not have a solution, but those with a solution are guaranteed to have only one unique solution. For each input maze, either a solution or a message indicating no solution is possible will be sent to the output.
Input
Output
Sample Input
DICEMAZE1
3 3 1 2 5 1
-1 2 4
5 5 6
6 -1 -1
DICEMAZE2
4 7 2 6 3 6
6 4 6 0 2 6 4
1 2 -1 5 3 6 1
5 3 4 5 6 4 2
4 1 2 0 3 -1 6
DICEMAZE3
3 3 1 1 2 4
2 2 3
4 5 6
-1 -1 -1
END
Sample Output
DICEMAZE1
(1,2),(2,2),(2,3),(3,3),(3,2),(3,1),(2,1),(1,1),(1,2)
DICEMAZE2
(2,6),(2,5),(2,4),(2,3),(2,2),(3,2),(4,2),(4,1),(3,1),
(2,1),(2,2),(2,3),(2,4),(2,5),(1,5),(1,6),(1,7),(2,7),
(3,7),(4,7),(4,6),(3,6),(2,6)
DICEMAZE3
No Solution Possible
Source
#include<stdio.h>
#include<string.h>
#include<ctype.h> int r,c,map[15][15],vis[15][15][7][7],die[][6]={{7,4,2,5,3,7},{3,7,6,1,7,4},{5,1,7,7,6,2},{2,6,7,7,1,5},{4,7,1,6,7,3},{7,3,5,2,4,7}};//die[i][j]表示i+1为top j+1为face时骰子左边的点数
int sr,sc;//face[7]={0,6,5,4,3,2,1},
int dx[4]={0,0,1,-1},dy[4]={1,-1,0,0},w,deep;
char name[25]; struct point
{
int i,j,fi,fj;
}s[3000]; int bound(int y,int x)
{
if(x>=1&&x<=c&&y>=1&&y<=r)
return 1;
else
return 0;
} int dfs(struct point a,int facenum,int topnum)//r是行c是列
{
int i,j;
if(a.i==sr&&a.j==sc&&deep>0)
return 1;
deep++;
for(i=0;i<4;i++)
{
if(bound(a.i+dy[i],a.j+dx[i])&&(map[a.i+dy[i]][ a.j+dx[i]]==-1||map[a.i+dy[i]][a.j+dx[i]]==topnum))
{
if(i<2)
{
if(i==0)
{
if(!vis[a.i+1][a.j][topnum][7-facenum])//表示向下滚(i增大)
{
vis[a.i+1][a.j][topnum][7-facenum]=1;
s[w].i=a.i+1;
s[w].j=a.j;//s[w].fi=a.i;s[w].fj=a.j;
w++;
if(dfs(s[w-1],topnum,7-facenum))
{
// printf("(%d,%d)",a.i,a.j);
return 1;
}
else w--;
}
}
else
{
if(!vis[a.i-1][a.j][7-topnum][facenum])//向上滚(也就是i减小的方向)
{
vis[a.i-1][a.j][7-topnum][facenum]=1;
s[w].i=a.i-1;
s[w].j=a.j;//s[w].fi=a.i;s[w].fj=a.j;
w++;
if(dfs(s[w-1],7-topnum,facenum))
{
// printf("(%d,%d)",a.i,a.j);
return 1;
}
else w--;
}
}
}
else
{
if(i==2)
{
if(!vis[a.i][a.j+1][facenum][die[topnum-1][facenum-1]])//向右滚
{
vis[a.i][a.j+1][facenum][die[topnum-1][facenum-1]]=1;
s[w].i=a.i;
s[w].j=a.j+1;//s[w].fi=a.i;s[w].fj=a.j;
w++;
if(dfs(s[w-1],facenum,die[topnum-1][facenum-1]))
{
// printf("(%d,%d)",a.i,a.j);
return 1;
}
else w--;
}
}
else
{
if(!vis[a.i][a.j-1][facenum][7-die[topnum-1][facenum-1]])//向左滚
{
vis[a.i][a.j-1][facenum][7-die[topnum-1][facenum-1]]=1;
s[w].i=a.i;
s[w].j=a.j-1;//s[w].fi=a.i;s[w].fj=a.j;
w++;
if(dfs(s[w-1],facenum,7-die[topnum-1][facenum-1]))
{
// printf("(%d,%d)",a.i,a.j);
return 1;
}
else w--;
}
}
}
}
}
return 0;
} int scan()//优化输入的
{
char c;
int ret;
int sig=0;
while(isspace(c=getchar()))
;
if(c=='-')
{
sig=1;
c=getchar();
}
ret=c-'0';
while((c=getchar())>='0'&& c<='9')
ret=ret*10+c-'0';
return sig?-ret:ret;
}
int main()
{
int i,j,k,ft,ff;
while(1)
{
gets(name);
if(strcmp(name,"END")==0)
break;
//scanf("%d%d%d%d%d%d",&r,&c,&sr,&sc,&ft,&ff);
r=scan();
c=scan();
sr=scan();
sc=scan();
ft=scan();
ff=scan();
for(i=1;i<=r;i++)
for(j=1;j<=c;j++)
{
//scanf("%d",&map[i][j]);
map[i][j]=scan();
}
//getchar();
memset(s,0,sizeof(s));
memset(vis,0,sizeof(vis));
s[0].i=sr;
s[0].j=sc;
w=1;
puts(name);
deep=0;
if(dfs(s[0],ff,ft))
{
printf(" ");
for(i=0;i<w-1;i++)
{
printf("(%d,%d),",s[i].i,s[i].j);
if((i+1)%9==0)
{
printf("\n ");
}
}
printf("(%d,%d)\n",s[i].i,s[i].j);
}
else printf(" No Solution Possible\n");
}
return 0;
}
poj 1872 A Dicey Problem WA的代码,望各位指教!!!的更多相关文章
- POJ 2826 An Easy Problem? 判断线段相交
POJ 2826 An Easy Problem?! -- 思路来自kuangbin博客 下面三种情况比较特殊,特别是第三种 G++怎么交都是WA,同样的代码C++A了 #include <io ...
- UVA 810 - A Dicey Problem(BFS)
UVA 810 - A Dicey Problem 题目链接 题意:一个骰子,给你顶面和前面.在一个起点,每次能移动到周围4格,为-1,或顶面和该位置数字一样,那么问题来了,骰子能不能走一圈回到原地, ...
- UVA-810 A Dicey Problem (BFS)
题目大意:滚骰子游戏,骰子的上面的点数跟方格中的数相同时或格子中的数是-1时能把格子滚过去,找一条从起点滚到起点的路径. 题目大意:简单BFS,状态转移时细心一些即可. 代码如下; # include ...
- POJ.3468 A Simple Problem with Integers(线段树 区间更新 区间查询)
POJ.3468 A Simple Problem with Integers(线段树 区间更新 区间查询) 题意分析 注意一下懒惰标记,数据部分和更新时的数字都要是long long ,别的没什么大 ...
- POJ 3468 A Simple Problem with Integers 【线段树,区间更新】
题意:你有N个整数,A1,A2,-,一个.你须要处理两种类型的操作.一种类型的操作是加入了一些给定的数字,每一个数字在一个给定的时间间隔. 还有一种是在给定的时间间隔要求数量的总和. 难点:主要是la ...
- POJ 3468.A Simple Problem with Integers-线段树(成段增减、区间查询求和)
POJ 3468.A Simple Problem with Integers 这个题就是成段的增减以及区间查询求和操作. 代码: #include<iostream> #include& ...
- poj 3468 A Simple Problem with Integers 【线段树-成段更新】
题目:id=3468" target="_blank">poj 3468 A Simple Problem with Integers 题意:给出n个数.两种操作 ...
- POJ 1152 An Easy Problem! (取模运算性质)
题目链接:POJ 1152 An Easy Problem! 题意:求一个N进制的数R.保证R能被(N-1)整除时最小的N. 第一反应是暴力.N的大小0到62.发现当中将N进制话成10进制时,数据会溢 ...
- poj1872A Dicey Problem
Home Problems Status Contest 284:28:39 307:00:00 Overview Problem Status Rank A B C D E F G H ...
随机推荐
- STM32启动模式
STM32三种启动模式对应的存储介质均是芯片内置的,它们是: 1)用户闪存 = 芯片内置的Flash.2)SRAM = 芯片内置的RAM区,就是内存啦.3)系统存储器 = 芯片内部一块特定的区域,芯片 ...
- BZOJ 2005: [Noi2010]能量采集( 数论 + 容斥原理 )
一个点(x, y)的能量损失为 (gcd(x, y) - 1) * 2 + 1 = gcd(x, y) * 2 - 1. 设g(i)为 gcd(x, y) = i ( 1 <= x <= ...
- VS2010对C++11的支持列表(感觉大部分都不支持)
c++11,就是之前的c++0x,已经成为了最新的c++标准.像咱这样天天用c++的,就赶紧follow一下.学习成果,放在这里,不说分享,至少自己增强下记忆. 首先,给出一些有用的链接. http: ...
- 远程调用内核接口(remote call kernel)
-------------------------------------------------------------------------------- 标题: 远程调用内核接口(remote ...
- Android Studio经常使用操作技巧(不断更新)
这段时间一直在用Android Studio做一些Demo的开发.一開始从Eclipse中转向这个开发工具,各种不适应,希望此博文能够一直更新.还有网友能够分享出自己方便更好更快开发的一些技巧. 首先 ...
- 用js模拟struts2的多action调用
近期修了几个struts2.1升级到2.3后动态方法调用失效的bug,深有感悟, 原始方法能够參考我之前的博文:struts2.1升级到2.3后动态调用方法问题 可是我那种原始方法有一个局限,就是在s ...
- 学习算法-基数排序(radix sort)卡片分类(card sort) C++数组实现
基数排序称为卡片分类,这是一个比较早的时间越多,排名方法. 现代计算机出现之前,它已被用于排序老式打孔卡. 说下基数排序的思想.前面我有写一个桶式排序,基数排序的思想是桶式排序的推广. 桶式排序:ht ...
- HDU4850 构造一个长度n串,它需要随机长度4子是不相同
n<=50W.(使用26快报) 构造函数:26一个.截至构建26^4不同的字符串,最长的长度26^4+3.如此之大的输出"impossble",被判重量的四维阵列. 在正向结 ...
- 可执行程序的入口点在那里?(强化概念:程序真正的入口是mainCRTstartup)
今天终于有时间来研究一下一个很大很大的工程编译成一个exe和若干dll后,程序是如果执行它的第一条指令的?操作系统以什么规则来找到应该执行的第一条指令(或说如何找到第一个入口函数的)? 我们以前写wi ...
- HDU3709:Balanced Number(数位DP+记忆化DFS)
Problem Description A balanced number is a non-negative integer that can be balanced if a pivot is p ...