Part 9 Sorting data in AngularJS
To sort the data in Angular
1. Use orderBy filter
{{ orderBy_expression | orderBy : expression : reverse}}
Example : ng-repeat="employee in employees |
orderBy:'salary':false"
2. To sort in ascending order, set reverse to false
3. To sort in descending order, set reverse to true
4. You can also use + and - to sort in ascending and descending order respectively
Example : ng-repeat="employee in employees |
orderBy:'+salary'"
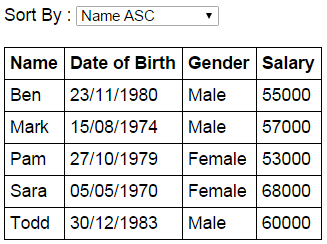
The dropdownlist shows the columns and the direction we want to sort
When a dropdownlist item is selected, the table data should be sorted by the selected column
Script.js :
The controller function builds the model. Also sortColumn property is
added to the $scope object. Notice sortColumn property is initialized to
name. This ensures that the data is sorted by name column in ascending
order, when the form first loads
var app = angular
.module("myModule", [])
.controller("myController", function ($scope) { var employees = [
{
name: "Ben", dateOfBirth: new Date("November 23, 1980"),
gender: "Male", salary: 55000
},
{
name: "Sara", dateOfBirth: new Date("May 05, 1970"),
gender: "Female", salary: 68000
},
{
name: "Mark", dateOfBirth: new Date("August 15, 1974"),
gender: "Male", salary: 57000
},
{
name: "Pam", dateOfBirth: new Date("October 27, 1979"),
gender: "Female", salary: 53000
},
{
name: "Todd", dateOfBirth: new Date("December 30, 1983"),
gender: "Male", salary: 60000
}
]; $scope.employees = employees;
$scope.sortColumn = "name"; });
HtmlPage1.html :
The select element, has the list of columns by which the data should be
sorted. + and - symbols control the sort direction. When the form
initially loads notice that the data is sorted by name column in
ascending order, and name option is automatically selected in the select
element. Notice the orderBy filter is using the sortColumn property
that is attached to the $scope object. When the selection in the select
element changes, the sortColumn property of the $scope object will be
updated automatically with the selected value, and in turn the updated
value is used by the orderBy filter to sort the data.
<!DOCTYPE html>
<html xmlns="http://www.w3.org/1999/xhtml">
<head>
<title></title>
<script src="Scripts/angular.min.js"></script>
<script src="Scripts/Script.js"></script>
<link href="Styles.css" rel="stylesheet" />
</head>
<body ng-app="myModule">
<div ng-controller="myController">
Sort By :
<select ng-model="sortColumn">
<option value="name">Name ASC</option>
<option value="+dateOfBirth">Date of Birth ASC</option>
<option value="+gender">Gender ASC</option>
<option value="-salary">Salary DESC</option>
</select>
<br /><br />
<table>
<thead>
<tr>
<th>Name</th>
<th>Date of Birth</th>
<th>Gender</th>
<th>Salary</th>
</tr>
</thead>
<tbody>
<tr ng-repeat="employee in employees | orderBy:sortColumn">
<td>
{{ employee.name }}
</td>
<td>
{{ employee.dateOfBirth | date:"dd/MM/yyyy" }}
</td>
<td>
{{ employee.gender }}
</td>
<td>
{{ employee.salary }}
</td>
</tr>
</tbody>
</table>
</div>
</body> </html>
Styles.css : CSS styles to make the form look pretty.
body {
font-family: Arial;
} table {
border-collapse: collapse;
} td {
border: 1px solid black;
padding: 5px;
} th {
border: 1px solid black;
padding: 5px;
text-align: left; }
Part 9 Sorting data in AngularJS的更多相关文章
- SQL Fundamentals:Restricting and Sorting Data限制和排序数据(FROM-WHERE-SELECT-ORDER BY)
SQL Fundamentals || Oracle SQL语言 控制操作的显示列:基本的SELECT语句 控制行:限定查询和排序显示 分组统计查询 限定查询:WHERE字句 排序显示:ORDER B ...
- [译]AngularJS sercies - 获取后端数据
原文:ANGULARJS SERVICES – FETCHING SERVER DATA $http是AngularJS内置的服务,能帮助我们完成从服务端获数据.简单的用法就是在你需要数据的时候,发起 ...
- [Angularjs]asp.net mvc+angularjs+web api单页应用之CRUD操作
写在前面 前篇文章整理了angularjs学习目录,有园子里的朋友问我要这方面的demo,周末也没什么事,就在之前的单页应用的demo上面添加了增删改查的操作.代码比较简单,这里只列举比较重要的代码片 ...
- AngularJs 基础(60分钟入门)
AngularJS 是一个创建富客户端应用的JavaScript MVC框架.你仍然需要具有服务端后台,但大多数的用户交互逻辑将放到客户端上处理.它可以创建单页的应用程序,一个页面的应用仅仅需要HTM ...
- AngularJs 基础(60分钟入门) (转)
AngularJs是一个不错的用于开发SPA应用(单页Web应用)的框架.单页Web应用(single page web application,SPA),就是只有一张Web页面的应用.浏览器一开始会 ...
- AngularJS学习笔记3
6.AngularJS 控制器 AngularJS 控制器 控制 AngularJS 应用程序的数据. AngularJS 控制器是常规的 JavaScript 对象. ng-controller 指 ...
- Lerning Entity Framework 6 ------ Inserting, Querying, Updating, and Deleting Data
Creating Entities First of all, Let's create some entities to have a test. Create a project Add foll ...
- [译]AngularJS Services 获取后端数据
原文:ANGULARJS SERVICES – FETCHING SERVER DATA $http是AngularJS内置的服务,能帮助我们完成从服务端获数据.简单的用法就是在你需要数据的时候,发起 ...
- Getting Started with Django Rest Framework and AngularJS
转载自:http://blog.kevinastone.com/getting-started-with-django-rest-framework-and-angularjs.html A ReST ...
随机推荐
- Delphi Interfaces
http://www.delphibasics.co.uk/Article.asp?Name=Interface The reason for interfaces Classes that ex ...
- Bit Twiddling Hacks
http://graphics.stanford.edu/~seander/bithacks.html Bit Twiddling Hacks By Sean Eron Andersonseander ...
- js日期计算及快速获取周、月、季度起止日
var now = new Date(); //当前日期 var nowDayOfWeek = (now.getDay() == 0) ? 7 : now.getDay() - 1; //今天是本周的 ...
- 反射遍历List<>泛型类型
有这样一个需求:一个实体模型,有一个属性是一个实体列表List<OrderDetail>,我需要对实体列表遍历,对每一个实体的属性根据属性特性进行验证,如是否为必填等等,如下一个反射方法能 ...
- linux 下cocos2dx-3.3.1环境搭建
1.安装依赖 依赖包含: libx11-dev libxmu-dev libglu1-mesa-dev libgl2ps-dev libxi-dev g++ libzip-dev libpng12-d ...
- webView用法小结
1.加入权限:AndroidManifest.xml中必须使用许可"android.permission.INTERNET",否则会出Web page not available错 ...
- direction:rtl demo
<style> body {font:14px/18px Consolas;} div { width:100px;padding:10px; margin:10px 0px 30px 0 ...
- 云服务器 ECS Linux 误删除文件恢复方法介绍
云服务器 ECS Linux 下,rm -rf 意味着一旦删除的文件是无法挽回的.但如果在没有文件覆盖操作的前提下,可以先尝试相关方式进行文件恢复. 本文对此进行简要说明. https://help ...
- 关于linux内核模块Makefile的解析
转载:http://www.embeddedlinux.org.cn/html/yingjianqudong/201403/23-2820.html Linux内核是一种单体内核,但是通过动态加载模块 ...
- TFS 2010 使用手册(二)项目集合与项目
1.项目集合 1.1 项目集合创建 打开TFS管理控制台,点击“团队项目集合”. 图1点击“团队项目集合” 图2 点击“创建集合” 然后按照向导一步步完成项目集合的创建. 1.2 项目集合的删除 选中 ...