Ducci Sequence
Description
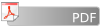
A Ducci sequence is a sequence of n-tuples of integers. Given an n-tuple of integers (a1, a2, ... , an), the next n-tuple in the sequence is formed by taking the absolute differences of neighboring integers:
Ducci sequences either reach a tuple of zeros or fall into a periodic loop. For example, the 4-tuple sequence starting with 8,11,2,7 takes 5 steps to reach the zeros tuple:
The 5-tuple sequence starting with 4,2,0,2,0 enters a loop after 2 steps:
Given an n-tuple of integers, write a program to decide if the sequence is reaching to a zeros tuple or a periodic loop.
Input
Your program is to read the input from standard input. The input consists of T test cases. The number of test cases T is given in the first line of the input. Each test case starts with a line containing an integer n(3n
15), which represents the size of a tuple in the Ducci sequences. In the following line, n integers are given which represents the n-tuple of integers. The range of integers are from 0 to 1,000. You may assume that the maximum number of steps of a Ducci sequence reaching zeros tuple or making a loop does not exceed 1,000.
Output
Your program is to write to standard output. Print exactly one line for each test case. Print `LOOP' if the Ducci sequence falls into a periodic loop, print `ZERO' if the Ducci sequence reaches to a zeros tuple.
The following shows sample input and output for four test cases.
Sample Input
4
4
8 11 2 7
5
4 2 0 2 0
7
0 0 0 0 0 0 0
6
1 2 3 1 2 3
Sample Output
ZERO
LOOP
ZERO
LOOP 这题的意思就是输入n个数,相邻两个数相减的绝对值赋给前一个数,最后一个数与第一个数相减。如此循环下去如果在一千次以内数列的所有数都是0了,就输出ZERO,否则输出LOOP。 代码如下:
#include <iostream>
using namespace std;
int main()
{
int T;
cin>>T;
while(T--)
{
int n,a[20];
cin>>n;
int flag=0; //每次输入完都要把flag归零
for(int i=0;i<n;i++)
cin>>a[i];
for(int j=0;j<1000;j++)
{
int f=a[0]; //因为先是a[0]与a[1]运算再赋值给a[0],a[0]的值会变,所以要先把a[0]的值赋给f。
int s=0;
for(int i=0;i<n-1;i++)
{
if(a[i]>=a[i+1])
a[i]=a[i]-a[i+1];
else
a[i]=a[i+1]-a[i];
s+=a[i]; //求数组0到n-2的和。
}
if(f>=a[n-1])
a[n-1]=f-a[n-1];
else
a[n-1]=a[n-1]-f;
s+=a[n-1]; // 再加上a[n-1]的值。
if(s==0)
{
flag=1;
break;
}
}
if(flag)
cout<<"ZERO"<<endl;
else
cout<<"LOOP"<<endl;
}
return 0;
}
第一次写博客,望各位海涵。
Ducci Sequence的更多相关文章
- UVA 1594 Ducci Sequence(两极问题)
Ducci Sequence Time Limit:3000MS Memory Limit:0KB 64bit IO Format:%lld & %llu D ...
- uva 1594 Ducci Sequence <queue,map>
Ducci Sequence Description A Ducci sequence is a sequence of n-tuples of integers. Given an n-tupl ...
- UVa----------1594(Ducci Sequence)
题目: 1594 - Ducci Sequence Asia - Seoul - 2009/2010A Ducci sequence is a sequence of n-tuples of inte ...
- [刷题]算法竞赛入门经典(第2版) 5-2/UVa1594 - Ducci Sequence
书上具体所有题目:http://pan.baidu.com/s/1hssH0KO 代码:(Accepted,20 ms) //UVa1594 - Ducci Sequence #include< ...
- UVA 1594 Ducci Sequence(紫书习题5-2 简单模拟题)
A Ducci sequence is a sequence of n-tuples of integers. Given an n-tuple of integers (a1, a2, · · · ...
- Ducci Sequence解题报告
A Ducci sequence is a sequence of n-tuples of integers. Given an n-tuple of integers (a1, a2, ... , ...
- Ducci Sequence UVA - 1594
A Ducci sequence is a sequence of n-tuples of integers. Given an n-tuple of integers (a1,a2,···,an ...
- Uva - 1594 - Ducci Sequence
水题,算出每次的结果,比较是否全0,循环1000次还不是全0则LOOP AC代码: #include <iostream> #include <cstdio> #include ...
- UVA 1594:Ducci Sequence (模拟 Grade E)
题意: 对于一个n元组(a0,a1,...),一次变换后变成(|a0-a1|,|a1-a2|,...) 问1000次变换以内是否存在循环. 思路: 模拟,map判重 代码: #include < ...
随机推荐
- Python Virtualenv 虚拟环境
在python2和python3共存的机器上做开发,要想互不干扰,虚拟环境很重要. Debian7 默认是python2.7.3,装好python3.4.1后怎么建立虚拟环境呢? $ pyvenv m ...
- sql 自定义函数--固定格式字符转时间类型
遇到一个德国的客户,他们的时间格式是JJJJ-TT-DD HH:MM:SS,程序按照这个格式将时间插入数据库,但是在sql自带的转换函数convert.cast过程中报错,网上搜了下都说用conver ...
- JPA与ORM以及Hibernate
- python(5)–sys模块
sys.argv 命令行参数list, 第一个元素是程序本身路径 sys.exit(n) 退出程序,退出时输入信息n sys.version 获取python解释程序的版本信息 sys.maxint ...
- (转)嵌入式学习准备---linux c 文件锁
(1)fcntl函数说明 前面的这5个基本函数实现了文件的打开.读写等基本操作,这一节将讨论的是,在文 件已经共享的情况下如何操作,也就是当多个用户共同使用.操作一个文件的情况,这时,Linux 通常 ...
- [改善Java代码]易变业务使用脚本语言编写
建议16: 易变业务使用脚本语言编写 Java世界一直在遭受着异种语言的入侵,比如PHP.Ruby.Groovy.JavaScript等,这些“入侵者”都有一个共同特征:全是同一类语言—脚本语言,它们 ...
- 一些xcode5.1创建的工程在xcode6.0下不能编译的问题
这是因为Xcode5.1.1自动选上了arm64架构, 建议解决办法是: Build Settings-ValidArchitectures中却掉arm64
- https抓包判断证书问题
openssl s_client -connect 61.135.250.130:443这个是reg.163.com的 tcpdump 也可
- AngularJS合集
AngularJS是Google开源的一款JavaScript MVC框架,弥补了HTML在构建应用方面的不足,其通过使用指令(directives)结构来扩展HTML词汇,使开发者可以使用HTML来 ...
- asp.net中web.config配置节点大全详解【转】
web.config 文件查找规则: (1)如果在当前页面所在目录下存在web.config文件,查看是否存在所要查找的结点名称,如果存在返回结果并停止查找. (2)如果当前页面所在目录下不存在web ...